How to Leverage Git Blame to Track Code History
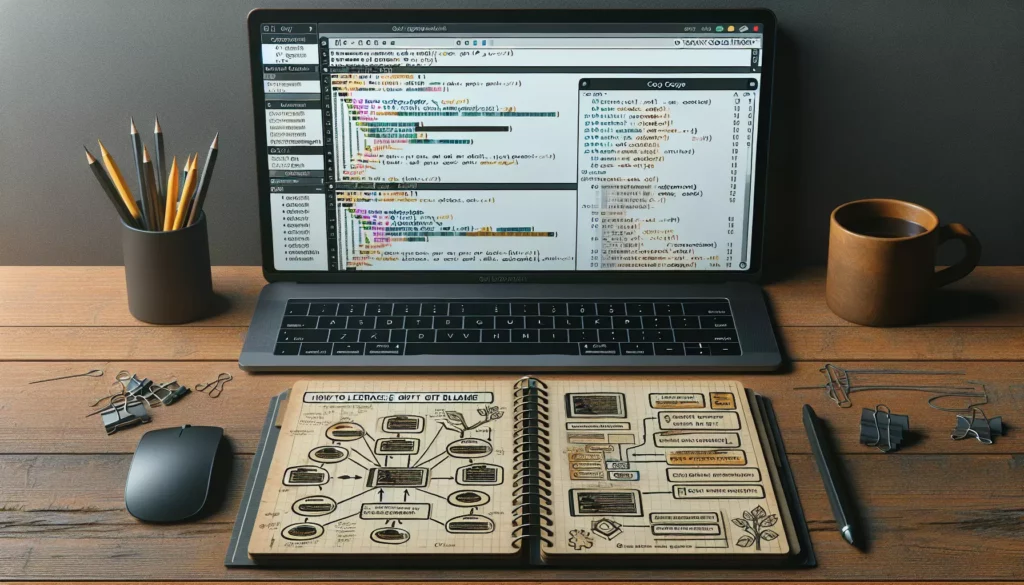
In the world of software development, understanding the history and evolution of code is crucial for maintaining, debugging, and improving projects. One powerful tool that developers have at their disposal is Git blame. This feature allows you to trace the origin of each line of code in a file, providing valuable insights into who made specific changes, when they were made, and why. In this comprehensive guide, we’ll explore how to leverage Git blame effectively to track code history and enhance your development workflow.
What is Git Blame?
Git blame is a command in the Git version control system that shows what revision and author last modified each line of a file. It’s an invaluable tool for understanding the context behind code changes, identifying potential bugs, and collaborating with team members.
The name “blame” might sound negative, but it’s not about pointing fingers. Instead, it’s about attributing changes to their authors and understanding the evolution of code over time.
Why Use Git Blame?
Before diving into the how-to, let’s explore some reasons why Git blame is an essential tool in a developer’s arsenal:
- Code Understanding: When working on unfamiliar code, Git blame helps you understand why certain decisions were made and who might have more context.
- Debugging: If you encounter a bug, Git blame can help you identify when the problematic code was introduced and by whom.
- Code Review: During code reviews, Git blame provides context about recent changes and their authors.
- Collaboration: It helps team members understand who to reach out to for questions about specific parts of the codebase.
- Historical Context: Git blame allows you to see the evolution of code over time, providing insights into the project’s history.
Basic Usage of Git Blame
Let’s start with the basic usage of Git blame. The simplest way to use Git blame is by running the following command in your terminal:
git blame <filename>
This command will display the specified file with annotations for each line, showing the commit hash, author, date, and line number. Here’s an example of what the output might look like:
^5d830c4 (John Doe 2023-03-15 10:30:45 -0700 1) def calculate_sum(a, b):
^5d830c4 (John Doe 2023-03-15 10:30:45 -0700 2) return a + b
94f85cf6 (Jane Smith 2023-04-02 14:22:30 -0700 3)
94f85cf6 (Jane Smith 2023-04-02 14:22:30 -0700 4) def calculate_product(a, b):
94f85cf6 (Jane Smith 2023-04-02 14:22:30 -0700 5) return a * b
In this example, we can see that John Doe introduced the calculate_sum
function on March 15, 2023, while Jane Smith added the calculate_product
function on April 2, 2023.
Advanced Git Blame Techniques
While the basic Git blame command is useful, there are several advanced techniques and options that can make it even more powerful:
1. Limiting the Range of Lines
If you’re interested in a specific portion of a file, you can limit the blame output to a range of lines:
git blame -L 10,20 <filename>
This command will show blame information only for lines 10 through 20 of the specified file.
2. Ignoring Whitespace Changes
Sometimes, you want to focus on substantial changes and ignore minor whitespace modifications. Use the -w
option to achieve this:
git blame -w <filename>
3. Showing Long Format
For more detailed information, including the full commit hash and author email, use the -l
option:
git blame -l <filename>
4. Detecting Moved or Copied Lines
Git blame can detect lines that were moved or copied from other files. Use the -C
option to enable this feature:
git blame -C <filename>
5. Blaming a Specific Revision
You can see how a file looked at a specific point in history by specifying a revision:
git blame <revision> -- <filename>
Replace <revision>
with a commit hash, branch name, or tag.
Integrating Git Blame with Your Development Workflow
Now that we’ve covered the basics and some advanced techniques, let’s explore how to integrate Git blame effectively into your development workflow:
1. Use Git Blame in Your IDE
Most modern Integrated Development Environments (IDEs) have Git blame functionality built-in or available through plugins. For example:
- Visual Studio Code: Use the “Git Lens” extension for advanced Git blame features.
- IntelliJ IDEA: Right-click on a file and select “Annotate” to see Git blame information.
- Sublime Text: Install the “Git Blame” package for inline blame information.
Using Git blame directly in your IDE can save time and provide instant context as you work.
2. Combine with Git Log
Once you’ve identified an interesting commit using Git blame, you can use Git log to get more context about that specific change:
git log -1 <commit-hash>
This command will show you the full commit message, which often contains valuable information about why a change was made.
3. Use Git Blame for Code Reviews
When reviewing code, use Git blame to understand recent changes and their context. This can help you ask more informed questions and provide better feedback during the review process.
4. Identify Code Hotspots
By analyzing Git blame data over time, you can identify parts of your codebase that change frequently. These “hotspots” might be candidates for refactoring or closer scrutiny.
5. Track Down Bugs
When you encounter a bug, use Git blame to identify when the problematic code was introduced. This can help you understand the context of the change and potentially why the bug occurred.
Best Practices for Using Git Blame
To get the most out of Git blame, consider the following best practices:
1. Write Meaningful Commit Messages
Since Git blame shows commit information, writing clear and descriptive commit messages becomes even more important. A good commit message should explain why a change was made, not just what was changed.
2. Use Atomic Commits
Make small, focused commits that address a single concern. This makes it easier to understand the purpose of each change when using Git blame.
3. Regularly Update Your Working Copy
Keep your local repository up-to-date with the remote to ensure you’re working with the latest blame information.
4. Combine with Other Git Tools
Use Git blame in conjunction with other Git commands like git log
, git show
, and git diff
for a more comprehensive understanding of code history.
5. Be Mindful of Code Formatting Changes
Large-scale code formatting changes can make Git blame less useful. Consider using options like -w
to ignore whitespace changes, or use tools like git-hyper-blame
that can see through these types of changes.
Common Pitfalls and How to Avoid Them
While Git blame is a powerful tool, there are some potential pitfalls to be aware of:
1. Overreliance on Blame Information
Pitfall: Assuming that the person who last modified a line is always the best person to ask about it.
Solution: Use Git blame as a starting point, but consider the overall context and history of the code. The original author or someone who has worked extensively on the module might be a better resource.
2. Misinterpreting Code Evolution
Pitfall: Failing to consider that code might have been refactored or moved from another file.
Solution: Use the -C
option to detect moved or copied lines, and combine Git blame with git log
to get a more complete picture of code evolution.
3. Ignoring Merge Commits
Pitfall: Merge commits can sometimes obscure the true origin of code changes.
Solution: Use the --first-parent
option with Git blame to follow only the first parent of merge commits, which can provide a clearer history in some cases.
4. Performance Issues with Large Files
Pitfall: Git blame can be slow on very large files or repositories with extensive history.
Solution: Use options like -L
to limit the range of lines, or consider using GUI tools that can cache blame information for better performance.
Advanced Git Blame Techniques for Power Users
For those looking to take their Git blame skills to the next level, here are some advanced techniques:
1. Using Git Blame with Aliases
Create Git aliases to streamline your blame commands. For example, you could create an alias for blame with ignored whitespace:
git config --global alias.blamew 'blame -w'
Now you can use git blamew <filename>
as a shortcut.
2. Combining Git Blame with Grep
Use Git blame in combination with grep to find specific changes:
git blame <filename> | grep "<search-term>"
This can help you quickly locate relevant changes in a file.
3. Using Git Blame Ignore Revisions
If you have commits that you want to ignore in blame (like large reformatting commits), you can create a file called .git-blame-ignore-revs
in your repository root. List the commit hashes to ignore, one per line. Then use:
git blame --ignore-revs-file .git-blame-ignore-revs <filename>
4. Visualizing Blame Information
For a more visual representation of blame data, consider using tools like git-heat
or gource
, which can provide graphical representations of code ownership and change frequency.
Conclusion
Git blame is a powerful tool that, when used effectively, can significantly enhance your understanding of code history and improve your development workflow. By leveraging Git blame, you can track down bugs more efficiently, understand the context behind code changes, and collaborate more effectively with your team.
Remember that Git blame is just one tool in the Git ecosystem. Combining it with other Git commands and best practices will give you a more comprehensive view of your project’s history and evolution.
As you continue to develop your skills in software development, mastering tools like Git blame will become increasingly valuable. Whether you’re a beginner just starting to explore version control or an experienced developer looking to refine your workflow, understanding and effectively using Git blame can be a game-changer in your coding journey.
Keep practicing, exploring, and pushing the boundaries of what you can do with Git. The more you use these tools, the more proficient you’ll become, and the more value you’ll be able to extract from your version control system. Happy coding!