How to Learn React.js for Front-End Development: A Comprehensive Guide
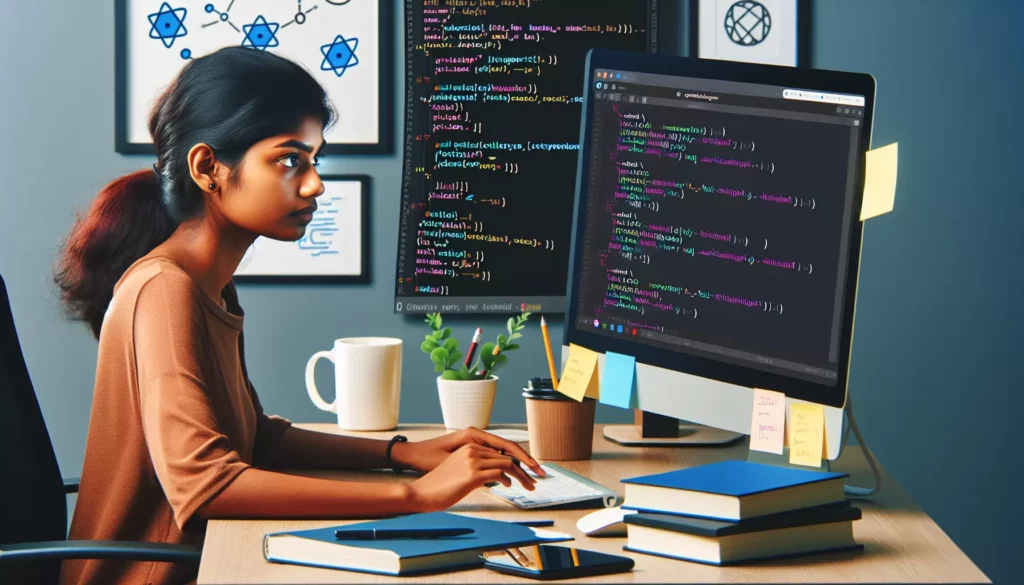
In today’s dynamic world of web development, React.js has emerged as a powerhouse for building interactive and efficient user interfaces. Whether you’re a beginner looking to break into front-end development or an experienced developer aiming to expand your skill set, learning React.js can significantly boost your career prospects. This comprehensive guide will walk you through the process of learning React.js, from the basics to advanced concepts, and provide you with the resources and strategies to become proficient in this popular JavaScript library.
Table of Contents
- Understanding React.js: The Basics
- Prerequisites for Learning React.js
- Getting Started with React.js
- Core Concepts of React.js
- Advanced Topics in React.js
- Best Practices and Design Patterns
- Tools and Ecosystem
- Building Projects and Practice
- Learning Resources and Communities
- Career Growth and Opportunities
- Staying Updated with React.js
- Conclusion
1. Understanding React.js: The Basics
React.js, often simply called React, is an open-source JavaScript library for building user interfaces. Developed and maintained by Facebook, React has gained immense popularity due to its efficiency, flexibility, and component-based architecture. Before diving into the learning process, it’s crucial to understand what makes React unique:
- Component-Based Architecture: React allows you to build encapsulated components that manage their own state, then compose them to make complex UIs.
- Virtual DOM: React uses a virtual DOM (Document Object Model) to improve performance by minimizing direct manipulation of the actual DOM.
- Declarative Nature: With React, you describe what you want to render, and it takes care of updating the UI when the underlying data changes.
- Unidirectional Data Flow: React follows a unidirectional data flow, making it easier to understand how data changes affect the application.
2. Prerequisites for Learning React.js
Before you start learning React.js, it’s important to have a solid foundation in the following areas:
- HTML and CSS: A good understanding of HTML structure and CSS styling is essential for creating React components.
- JavaScript: Proficiency in modern JavaScript (ES6+) is crucial. You should be comfortable with concepts like:
- Variables and data types
- Functions and arrow functions
- Arrays and objects
- ES6 features (let, const, destructuring, spread operator, etc.)
- Promises and async/await
- Modules (import/export)
- DOM Manipulation: Understanding how to interact with the Document Object Model is helpful, although React abstracts much of this away.
- Node.js and npm: Familiarity with Node.js and the npm package manager will be beneficial for setting up React projects and managing dependencies.
3. Getting Started with React.js
Once you have the prerequisites covered, you can start your journey with React.js:
- Set up your development environment:
- Install Node.js and npm on your machine
- Choose a code editor (e.g., Visual Studio Code, Sublime Text, or WebStorm)
- Install React Developer Tools browser extension for debugging
- Create your first React app:
- Use Create React App (CRA) to set up a new project:
npx create-react-app my-first-react-app cd my-first-react-app npm start
- Explore the project structure and default files
- Use Create React App (CRA) to set up a new project:
- Understand JSX:
- Learn the syntax of JSX, which allows you to write HTML-like code in your JavaScript files
- Practice converting HTML to JSX and vice versa
- Create your first component:
- Start with a simple functional component
- Render the component in your app
4. Core Concepts of React.js
As you progress in your React.js learning journey, focus on mastering these core concepts:
Components and Props
- Understand the difference between functional and class components
- Learn how to pass data between components using props
- Practice creating reusable components
State and Lifecycle
- Learn about component state and how to manage it
- Understand the component lifecycle and its methods (for class components)
- Practice using the useState and useEffect hooks (for functional components)
Handling Events
- Learn how to handle user interactions in React components
- Understand the differences between handling events in React vs. vanilla JavaScript
Conditional Rendering
- Learn different techniques for conditional rendering in React
- Practice using ternary operators and logical AND (&&) for conditional rendering
Lists and Keys
- Understand how to render lists of elements in React
- Learn the importance of keys when rendering lists
Forms and Controlled Components
- Learn how to handle form inputs in React
- Understand the concept of controlled components
5. Advanced Topics in React.js
Once you’ve grasped the core concepts, dive into more advanced topics to deepen your understanding:
Hooks
- Master built-in hooks like useState, useEffect, useContext, and useRef
- Learn how to create custom hooks for reusable logic
Context API
- Understand how to use Context for global state management
- Learn when to use Context vs. props for data passing
Higher-Order Components (HOCs)
- Learn what HOCs are and how they can enhance component functionality
- Practice creating and using HOCs in your projects
Render Props
- Understand the render props pattern for sharing code between components
- Compare render props with hooks and HOCs
Error Boundaries
- Learn how to create error boundaries to handle component errors gracefully
- Understand the limitations of error boundaries
Code Splitting
- Learn how to implement code splitting using React.lazy and Suspense
- Understand the benefits of code splitting for performance optimization
6. Best Practices and Design Patterns
To become a proficient React developer, it’s essential to learn and apply best practices and common design patterns:
Component Composition
- Learn how to break down complex UIs into smaller, reusable components
- Understand the principle of composition over inheritance in React
State Management
- Learn when to use local component state vs. global state
- Explore state management libraries like Redux or MobX for larger applications
Performance Optimization
- Understand how to use React.memo for memoization
- Learn about the useCallback and useMemo hooks for optimizing performance
- Practice profiling and optimizing React applications
Code Organization
- Learn how to structure your React projects for scalability
- Understand common folder structures and file naming conventions
Testing
- Learn how to write unit tests for React components using Jest and React Testing Library
- Understand the importance of integration and end-to-end testing in React applications
7. Tools and Ecosystem
Familiarize yourself with the tools and ecosystem surrounding React.js:
Build Tools
- Learn about Webpack and how it bundles your React application
- Understand the role of Babel in transpiling modern JavaScript and JSX
State Management Libraries
- Explore Redux for global state management in large applications
- Learn about alternatives like MobX or Recoil
Routing
- Master React Router for handling navigation in single-page applications
Styling Solutions
- Learn about CSS-in-JS libraries like styled-components or Emotion
- Explore CSS Modules for scoped styling in React
Form Libraries
- Explore form management libraries like Formik or React Hook Form
UI Component Libraries
- Familiarize yourself with popular UI libraries like Material-UI or Ant Design
8. Building Projects and Practice
The best way to solidify your React.js skills is through practical application. Here are some ideas to get you started:
Beginner Projects
- Todo List App
- Weather App using a public API
- Simple Calculator
Intermediate Projects
- Blog Platform with React Router
- E-commerce Product Page
- Quiz Application
Advanced Projects
- Social Media Dashboard with Redux
- Real-time Chat Application
- Project Management Tool
Remember to incrementally increase the complexity of your projects as you learn new concepts and techniques.
9. Learning Resources and Communities
Take advantage of the wealth of resources available for learning React.js:
Official Documentation
- React Official Documentation – Always a great starting point and reference
Online Courses
- Udemy: “React – The Complete Guide” by Maximilian Schwarzmüller
- freeCodeCamp: “Introduction to the React Challenges”
- Codecademy: “Learn React”
Books
- “React Up and Running” by Stoyan Stefanov
- “Learning React” by Alex Banks and Eve Porcello
YouTube Channels
- Traversy Media
- The Net Ninja
- Academind
Communities and Forums
- Reddit: r/reactjs
- Stack Overflow: React tag
- Discord: Reactiflux
10. Career Growth and Opportunities
As you progress in your React.js journey, consider these steps for career growth:
Build a Portfolio
- Showcase your React projects on platforms like GitHub
- Create a personal website using React to demonstrate your skills
Contribute to Open Source
- Look for React-based open-source projects to contribute to
- Start with small bug fixes or documentation improvements
Network and Attend Events
- Join local React meetups or attend React conferences
- Participate in online React communities and forums
Prepare for Interviews
- Practice common React interview questions
- Be prepared to explain React concepts and solve coding challenges
Explore Related Technologies
- Learn about server-side rendering with Next.js
- Explore static site generation with Gatsby
- Consider learning React Native for mobile app development
11. Staying Updated with React.js
React.js is constantly evolving, so it’s important to stay updated:
Follow React Blogs and News Sources
- Official React Blog
- React Status Newsletter
- React Newsletter by Tyler McGinnis
Attend React Conferences
- ReactConf
- React Europe
- React Summit
Follow React Core Team Members and Influencers
- Dan Abramov (@dan_abramov)
- Kent C. Dodds (@kentcdodds)
- Sophie Alpert (@sophiebits)
Experiment with New Features
- Try out new React features in experimental versions
- Participate in discussions about upcoming React features
12. Conclusion
Learning React.js for front-end development is an exciting and rewarding journey. By following this comprehensive guide, you’ll be well on your way to mastering React and building powerful, efficient web applications. Remember that learning is an ongoing process, and the key to success is consistent practice and staying curious about new developments in the React ecosystem.
As you progress in your React.js learning journey, don’t forget to leverage platforms like AlgoCademy, which offer interactive coding tutorials and resources to help you sharpen your programming skills. AlgoCademy’s focus on algorithmic thinking and problem-solving can be particularly beneficial when working with React, especially when it comes to optimizing component logic and managing complex state interactions.
Whether you’re aiming to land your first front-end developer job or looking to upgrade your skills for more advanced positions, React.js proficiency will open up numerous opportunities in the tech industry. Stay persistent, keep building projects, and engage with the React community to accelerate your growth as a developer. Happy coding!