How to Learn Python Programming Quickly: A Comprehensive Guide
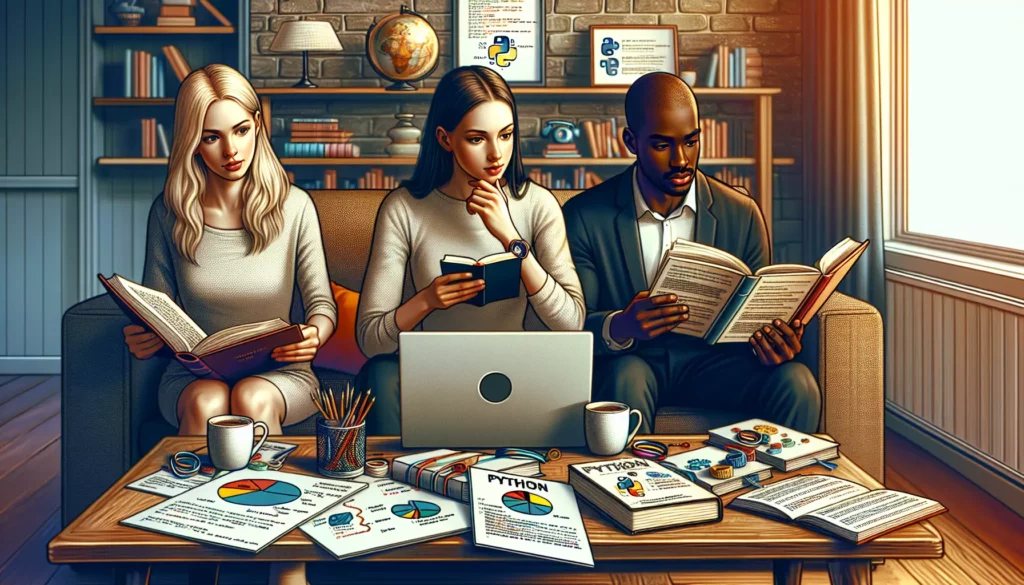
Python has become one of the most popular programming languages in the world, and for good reason. Its simplicity, versatility, and powerful libraries make it an excellent choice for beginners and experienced developers alike. Whether you’re looking to start a career in tech, enhance your data analysis skills, or simply learn a new hobby, mastering Python can open up a world of opportunities. In this comprehensive guide, we’ll explore effective strategies and resources to help you learn Python programming quickly and efficiently.
Table of Contents
- Why Learn Python?
- Set Clear Learning Goals
- Start with the Basics
- Leverage Interactive Tutorials
- Practice with Real Projects
- Enroll in Online Courses
- Tackle Coding Challenges
- Read Python Documentation
- Join a Python Community
- Utilize AI-Powered Learning Tools
- Dive into Advanced Concepts
- Stay Motivated and Consistent
- Prepare for Technical Interviews
- Conclusion
1. Why Learn Python?
Before diving into the learning process, it’s essential to understand why Python is worth your time and effort:
- Simplicity and Readability: Python’s clean syntax makes it easy to read and write, reducing the learning curve for beginners.
- Versatility: Python is used in various fields, including web development, data science, artificial intelligence, and automation.
- Large Community and Resources: With a vast community, you’ll find abundant learning resources and support.
- Career Opportunities: Python skills are in high demand across various industries, offering excellent job prospects.
- Powerful Libraries: Python’s extensive library ecosystem allows you to tackle complex tasks with ease.
2. Set Clear Learning Goals
To learn Python quickly, it’s crucial to define your objectives. Ask yourself:
- What do you want to achieve with Python?
- Are you learning for a specific job role or project?
- How much time can you dedicate to learning each day or week?
Setting clear goals will help you stay focused and measure your progress. For example, you might aim to:
- Build a personal website using Django within two months
- Create a data analysis project using pandas and matplotlib in six weeks
- Automate a repetitive task at work within a month
3. Start with the Basics
Begin your Python journey by mastering the fundamentals. Focus on:
- Variables and data types
- Basic operators and expressions
- Control structures (if statements, loops)
- Functions and modules
- Lists, tuples, and dictionaries
- File handling
Here’s a simple example of a Python function that demonstrates some of these basics:
def greet_user(name):
"""A simple function to greet the user."""
print(f"Hello, {name}! Welcome to Python programming.")
# Call the function
greet_user("Alice")
Understanding these core concepts will provide a solid foundation for more advanced topics.
4. Leverage Interactive Tutorials
Interactive tutorials offer a hands-on approach to learning Python. They allow you to write and execute code in real-time, providing immediate feedback. Some popular platforms include:
- Codecademy: Offers a comprehensive Python course with interactive lessons.
- Python.org: The official Python website provides a browser-based interactive tutorial.
- AlgoCademy: Provides interactive coding tutorials with a focus on algorithmic thinking and problem-solving.
- Jupyter Notebooks: Allow you to create and share documents containing live code, equations, visualizations, and narrative text.
These platforms can help you quickly grasp Python concepts through practical application.
5. Practice with Real Projects
Applying your knowledge to real-world projects is crucial for rapid learning. Start with small projects and gradually increase complexity. Some ideas include:
- Building a simple calculator
- Creating a to-do list application
- Developing a basic web scraper
- Implementing a text-based adventure game
Here’s a snippet of code for a simple calculator function:
def calculator():
"""A basic calculator function."""
num1 = float(input("Enter the first number: "))
operator = input("Enter the operator (+, -, *, /): ")
num2 = float(input("Enter the second number: "))
if operator == "+":
result = num1 + num2
elif operator == "-":
result = num1 - num2
elif operator == "*":
result = num1 * num2
elif operator == "/":
if num2 != 0:
result = num1 / num2
else:
return "Error: Division by zero"
else:
return "Error: Invalid operator"
return f"Result: {result}"
print(calculator())
Working on projects helps reinforce your learning and builds your portfolio, which can be valuable when seeking job opportunities.
6. Enroll in Online Courses
Structured online courses can provide a comprehensive learning path. Popular platforms offering Python courses include:
- Coursera: Offers courses from top universities, including the popular “Python for Everybody” specialization.
- edX: Provides Python courses from institutions like MIT and Harvard.
- Udemy: Offers a wide range of Python courses for different skill levels and applications.
- AlgoCademy: Provides structured learning paths with a focus on algorithmic thinking and interview preparation.
Many of these courses offer certificates upon completion, which can be added to your resume or LinkedIn profile.
7. Tackle Coding Challenges
Coding challenges and problems are excellent for honing your skills and learning new concepts. Platforms like LeetCode, HackerRank, and Project Euler offer a variety of Python challenges. Start with easier problems and progressively move to more complex ones.
Here’s an example of a simple coding challenge: reversing a string in Python.
def reverse_string(s):
"""Reverse a given string."""
return s[::-1]
# Test the function
print(reverse_string("Hello, World!")) # Output: !dlroW ,olleH
Regularly solving coding challenges improves your problem-solving skills and prepares you for technical interviews.
8. Read Python Documentation
The official Python documentation (docs.python.org) is an invaluable resource. It provides detailed information about Python’s syntax, built-in functions, and standard libraries. Make it a habit to refer to the documentation when you encounter new concepts or need clarification.
For example, if you’re learning about Python’s built-in sorted()
function, the documentation provides comprehensive information about its usage and parameters:
numbers = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5]
sorted_numbers = sorted(numbers, reverse=True)
print(sorted_numbers) # Output: [9, 6, 5, 5, 5, 4, 3, 3, 2, 1, 1]
Understanding how to read and utilize documentation is a crucial skill for any programmer.
9. Join a Python Community
Engaging with the Python community can accelerate your learning process. Join forums, social media groups, and local meetups to connect with other Python enthusiasts. Some popular platforms include:
- Reddit’s r/learnpython subreddit
- Stack Overflow for asking and answering questions
- Python Discord servers
- Local Python user groups or meetups
Participating in these communities allows you to ask questions, share knowledge, and stay updated with the latest Python trends and best practices.
10. Utilize AI-Powered Learning Tools
AI-powered coding assistants and learning platforms can significantly enhance your Python learning experience. These tools can provide personalized feedback, suggest improvements, and even generate code snippets. Some popular AI-powered tools include:
- GitHub Copilot: An AI pair programmer that suggests code completions.
- AlgoCademy’s AI Assistant: Provides step-by-step guidance and explanations for coding problems.
- Tabnine: An AI code completion tool that works with various IDEs.
While these tools can be incredibly helpful, it’s important to use them as learning aids rather than relying on them entirely. Understanding the code you write is crucial for your growth as a programmer.
11. Dive into Advanced Concepts
Once you’ve mastered the basics, explore more advanced Python concepts to deepen your knowledge:
- Object-Oriented Programming (OOP)
- Decorators and generators
- Context managers
- Multithreading and multiprocessing
- Web frameworks like Django or Flask
- Data analysis with pandas and NumPy
Here’s a simple example of a class in Python, demonstrating basic OOP concepts:
class Car:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
self.odometer_reading = 0
def get_descriptive_name(self):
long_name = f"{self.year} {self.make} {self.model}"
return long_name.title()
def read_odometer(self):
print(f"This car has {self.odometer_reading} miles on it.")
def update_odometer(self, mileage):
if mileage >= self.odometer_reading:
self.odometer_reading = mileage
else:
print("You can't roll back an odometer!")
# Create an instance of the Car class
my_car = Car('audi', 'a4', 2019)
print(my_car.get_descriptive_name())
my_car.update_odometer(23500)
my_car.read_odometer()
Understanding these advanced concepts will make you a more versatile Python programmer and open up new possibilities for your projects.
12. Stay Motivated and Consistent
Learning Python quickly requires dedication and consistency. Here are some tips to stay motivated:
- Set realistic goals: Break your learning journey into smaller, achievable milestones.
- Track your progress: Use tools like GitHub to document your learning and projects.
- Celebrate small wins: Acknowledge your achievements, no matter how small.
- Find a study buddy: Partner with someone who’s also learning Python to keep each other accountable.
- Take breaks: Regular breaks can help prevent burnout and improve retention.
Remember, consistency is key. Even 30 minutes of focused learning each day can lead to significant progress over time.
13. Prepare for Technical Interviews
If your goal is to land a Python-related job, prepare for technical interviews by:
- Practicing common Python interview questions
- Reviewing data structures and algorithms
- Participating in mock interviews
- Solving problems on platforms like LeetCode or HackerRank
AlgoCademy’s focus on preparing for technical interviews, particularly for major tech companies, can be especially helpful in this regard. Their AI-powered assistance and step-by-step guidance can help you tackle complex algorithmic problems and improve your problem-solving skills.
Here’s an example of a common interview question: checking if a string is a palindrome.
def is_palindrome(s):
"""Check if a string is a palindrome."""
# Remove non-alphanumeric characters and convert to lowercase
s = ''.join(char.lower() for char in s if char.isalnum())
# Compare the string with its reverse
return s == s[::-1]
# Test the function
print(is_palindrome("A man, a plan, a canal: Panama")) # Output: True
print(is_palindrome("race a car")) # Output: False
Practice implementing and explaining such solutions to prepare for technical interviews effectively.
14. Conclusion
Learning Python quickly is an achievable goal with the right approach and resources. By following this comprehensive guide, you can accelerate your Python learning journey and develop valuable programming skills. Remember to:
- Start with the basics and gradually move to more advanced topics
- Practice consistently with real projects and coding challenges
- Utilize interactive tutorials and online courses
- Engage with the Python community and leverage AI-powered learning tools
- Prepare for technical interviews if seeking a Python-related job
With dedication and the right resources, you can quickly become proficient in Python programming. Whether you’re aiming to enhance your career prospects, tackle data analysis projects, or simply explore the world of coding, Python offers a versatile and powerful toolset to achieve your goals.
Remember that learning to code is a journey, and every programmer continues to learn and grow throughout their career. Embrace the process, stay curious, and don’t be afraid to experiment with your newfound Python skills. Happy coding!