How to Learn Programming Through Building Games: A Comprehensive Guide
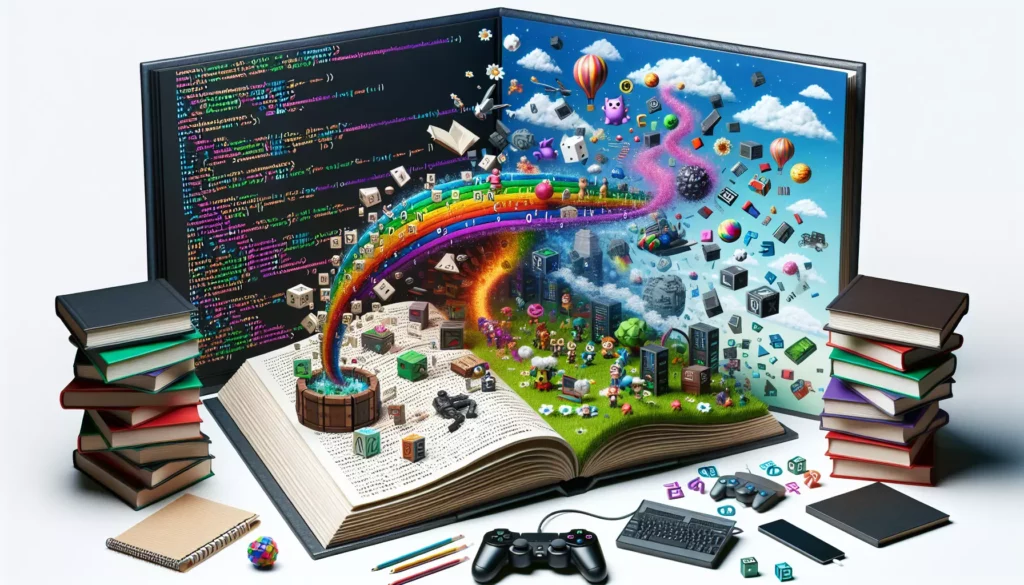
Learning programming can be a challenging but rewarding journey. One of the most engaging and effective ways to master coding skills is through game development. This approach not only makes the learning process more enjoyable but also provides practical experience in applying programming concepts. In this comprehensive guide, we’ll explore how you can learn programming by building games, covering various aspects from choosing the right programming language to advanced game development techniques.
Table of Contents
- Why Learn Programming Through Games?
- Getting Started: Choosing Your Path
- Programming Languages for Game Development
- Popular Game Engines for Beginners
- Basic Programming Concepts Through Simple Games
- Intermediate Game Projects to Enhance Your Skills
- Advanced Game Development Techniques
- Resources and Communities for Game Development
- Common Challenges and How to Overcome Them
- Career Paths in Game Development
- Conclusion: Your Journey Ahead
1. Why Learn Programming Through Games?
Learning programming through game development offers numerous advantages:
- Engagement: Games are inherently fun and interactive, making the learning process more enjoyable.
- Practical Application: Game development allows you to apply programming concepts in real-world scenarios.
- Visual Feedback: Immediate visual results help reinforce learning and motivate progress.
- Problem-Solving Skills: Game development challenges you to think creatively and solve complex problems.
- Multidisciplinary Learning: It combines various aspects of computer science, including graphics, AI, and user interface design.
By choosing to learn programming through game development, you’re setting yourself up for an exciting and rewarding educational journey.
2. Getting Started: Choosing Your Path
Before diving into game development, it’s essential to determine your goals and choose the right path:
Define Your Objectives
- Are you learning for fun or aiming for a career in game development?
- Do you want to focus on mobile games, web games, or desktop games?
- Are you interested in 2D or 3D game development?
Assess Your Current Skills
- Complete beginner: Start with basic programming concepts and simple game logic.
- Some programming experience: Jump into game-specific frameworks and engines.
- Experienced programmer: Focus on game design patterns and advanced techniques.
Choose Your Learning Style
- Self-paced online courses
- Structured bootcamps
- University programs in computer science or game development
- Hands-on learning through personal projects
By clearly defining your path, you can focus your efforts and make steady progress in your game development journey.
3. Programming Languages for Game Development
Choosing the right programming language is crucial for your game development journey. Here are some popular options:
Python
Python is an excellent choice for beginners due to its simplicity and readability. It’s great for creating simple 2D games and learning basic programming concepts.
Pros:
- Easy to learn and read
- Large community and extensive libraries (e.g., Pygame)
- Suitable for rapid prototyping
Cons:
- Not as performant as lower-level languages for complex games
- Limited options for advanced 3D game development
JavaScript
JavaScript is ideal for creating web-based games and is a great entry point for aspiring web developers.
Pros:
- Runs in web browsers, making games easily accessible
- Large ecosystem of game development libraries (e.g., Phaser, Three.js)
- Useful for both front-end and back-end development
Cons:
- Performance limitations compared to compiled languages
- Browser compatibility issues can be challenging
C#
C# is widely used in game development, especially with the Unity game engine.
Pros:
- Powerful and versatile language
- Excellent documentation and community support
- Used in Unity, one of the most popular game engines
Cons:
- Steeper learning curve compared to Python or JavaScript
- Primarily used with Unity, which may limit flexibility
C++
C++ is the industry standard for high-performance game development and is used in many AAA game engines.
Pros:
- Excellent performance and low-level control
- Used in popular engines like Unreal Engine
- Valuable skill for professional game development
Cons:
- Complex syntax and steep learning curve
- Memory management can be challenging for beginners
Choose a language that aligns with your goals and comfort level. Remember, you can always learn additional languages as you progress in your game development journey.
4. Popular Game Engines for Beginners
Game engines provide a framework and tools to streamline the game development process. Here are some popular options for beginners:
Unity
Unity is one of the most widely used game engines, suitable for both 2D and 3D game development.
Pros:
- Extensive documentation and tutorials
- Large asset store with pre-made models and scripts
- Cross-platform development capabilities
- Free for personal use and small studios
Cons:
- Can be overwhelming for absolute beginners
- Requires learning C# programming
Godot
Godot is an open-source game engine that’s gaining popularity among indie developers.
Pros:
- Completely free and open-source
- Lightweight and easy to use
- Supports both 2D and 3D game development
- Uses a Python-like scripting language (GDScript)
Cons:
- Smaller community compared to Unity or Unreal
- Fewer learning resources available
Phaser
Phaser is a popular HTML5 game framework for creating browser-based games.
Pros:
- Easy to learn for those familiar with JavaScript
- Great for creating 2D games quickly
- Games can be played directly in web browsers
Cons:
- Limited to 2D game development
- Performance may be an issue for complex games
Pygame
Pygame is a set of Python modules designed for writing video games.
Pros:
- Excellent for learning game development basics
- Simple and straightforward for Python beginners
- Good documentation and community support
Cons:
- Limited to 2D game development
- Lacks advanced features of more robust game engines
Choose an engine that matches your programming language preference and the type of games you want to create. Starting with a simpler engine like Pygame or Phaser can be beneficial for absolute beginners before moving on to more complex options like Unity or Godot.
5. Basic Programming Concepts Through Simple Games
Learning programming concepts through simple games is an effective way to build a strong foundation. Here are some basic concepts and game ideas to help you get started:
Variables and Data Types
Concept: Understanding how to store and manipulate different types of data.
Game Idea: Create a simple quiz game where you store questions and answers as variables.
// Example in JavaScript
let question = "What is the capital of France?";
let correctAnswer = "Paris";
let userAnswer = prompt(question);
if (userAnswer.toLowerCase() === correctAnswer.toLowerCase()) {
console.log("Correct!");
} else {
console.log("Sorry, that's incorrect.");
}
Conditional Statements
Concept: Making decisions in your code based on certain conditions.
Game Idea: Develop a simple “Guess the Number” game where the program compares the user’s guess to a random number.
// Example in Python
import random
secret_number = random.randint(1, 100)
guess = int(input("Guess a number between 1 and 100: "))
if guess == secret_number:
print("Congratulations! You guessed it!")
elif guess < secret_number:
print("Too low. Try again!")
else:
print("Too high. Try again!")
Loops
Concept: Repeating a set of instructions multiple times.
Game Idea: Create a simple “Hangman” game where the player has a limited number of attempts to guess a word.
// Example in C#
string word = "programming";
char[] guessedLetters = new char[word.Length];
int attempts = 6;
while (attempts > 0)
{
Console.WriteLine($"Word: {string.Join(" ", guessedLetters)}");
Console.WriteLine($"Attempts left: {attempts}");
char guess = Console.ReadKey().KeyChar;
bool correctGuess = false;
for (int i = 0; i < word.Length; i++)
{
if (word[i] == guess)
{
guessedLetters[i] = guess;
correctGuess = true;
}
}
if (!correctGuess)
{
attempts--;
}
if (new string(guessedLetters) == word)
{
Console.WriteLine("\nCongratulations! You guessed the word!");
break;
}
}
if (attempts == 0)
{
Console.WriteLine("\nGame over! The word was: " + word);
}
Functions
Concept: Organizing code into reusable blocks.
Game Idea: Build a simple “Rock, Paper, Scissors” game using functions for game logic and user input.
// Example in JavaScript
function getComputerChoice() {
const choices = ['rock', 'paper', 'scissors'];
return choices[Math.floor(Math.random() * choices.length)];
}
function determineWinner(playerChoice, computerChoice) {
if (playerChoice === computerChoice) {
return "It's a tie!";
}
if ((playerChoice === 'rock' && computerChoice === 'scissors') ||
(playerChoice === 'paper' && computerChoice === 'rock') ||
(playerChoice === 'scissors' && computerChoice === 'paper')) {
return "You win!";
}
return "Computer wins!";
}
function playGame() {
const playerChoice = prompt("Enter rock, paper, or scissors:").toLowerCase();
const computerChoice = getComputerChoice();
console.log(`You chose ${playerChoice}`);
console.log(`Computer chose ${computerChoice}`);
console.log(determineWinner(playerChoice, computerChoice));
}
playGame();
Arrays and Lists
Concept: Storing and manipulating collections of data.
Game Idea: Develop a simple “Memory” game where players need to match pairs of cards.
// Example in Python
import random
def create_board(size):
symbols = list("AABBCCDDEE"[:size])
board = symbols * 2
random.shuffle(board)
return board
def print_board(board, revealed):
for i in range(len(board)):
if revealed[i]:
print(board[i], end=" ")
else:
print("*", end=" ")
print()
def play_memory_game():
board = create_board(5)
revealed = [False] * len(board)
pairs_found = 0
while pairs_found < len(board) // 2:
print_board(board, revealed)
choice1 = int(input("Enter the first card (0-9): "))
choice2 = int(input("Enter the second card (0-9): "))
if board[choice1] == board[choice2]:
print("Match found!")
revealed[choice1] = True
revealed[choice2] = True
pairs_found += 1
else:
print("No match. Try again.")
print("Congratulations! You've found all the pairs.")
play_memory_game()
By implementing these simple games, you’ll gain hands-on experience with fundamental programming concepts. As you become more comfortable with these basics, you can start to combine them in more complex ways to create more sophisticated games.
6. Intermediate Game Projects to Enhance Your Skills
Once you’ve mastered the basics, it’s time to tackle more challenging projects. These intermediate game ideas will help you apply your skills in more complex scenarios and introduce you to new programming concepts.
Platformer Game
Creating a platformer game introduces concepts like physics, collision detection, and level design.
Key Concepts:
- Gravity and jumping mechanics
- Collision detection with platforms and obstacles
- Character animation
- Level design and scrolling backgrounds
Example Implementation (using Pygame):
import pygame
pygame.init()
# Set up the display
WIDTH, HEIGHT = 800, 600
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("Simple Platformer")
# Colors
WHITE = (255, 255, 255)
BLUE = (0, 0, 255)
# Player properties
player_x = 50
player_y = HEIGHT - 100
player_width = 40
player_height = 60
player_velocity_y = 0
player_speed = 5
jump_strength = -15
gravity = 0.8
# Platform properties
platform_width = 200
platform_height = 20
platforms = [
pygame.Rect(100, HEIGHT - 50, platform_width, platform_height),
pygame.Rect(400, HEIGHT - 150, platform_width, platform_height),
pygame.Rect(700, HEIGHT - 250, platform_width, platform_height)
]
clock = pygame.time.Clock()
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_SPACE and player_y == HEIGHT - player_height:
player_velocity_y = jump_strength
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
player_x -= player_speed
if keys[pygame.K_RIGHT]:
player_x += player_speed
# Apply gravity
player_velocity_y += gravity
player_y += player_velocity_y
# Check for collisions with platforms
player_rect = pygame.Rect(player_x, player_y, player_width, player_height)
for platform in platforms:
if player_rect.colliderect(platform):
if player_velocity_y > 0: # Falling
player_y = platform.top - player_height
player_velocity_y = 0
# Keep player on screen
if player_y > HEIGHT - player_height:
player_y = HEIGHT - player_height
player_velocity_y = 0
# Draw everything
screen.fill(WHITE)
pygame.draw.rect(screen, BLUE, (player_x, player_y, player_width, player_height))
for platform in platforms:
pygame.draw.rect(screen, BLUE, platform)
pygame.display.flip()
clock.tick(60)
pygame.quit()
Puzzle Game
Developing a puzzle game helps improve your problem-solving skills and introduces concepts like game state management and user interface design.
Key Concepts:
- Game state management
- Grid-based gameplay
- Scoring systems
- User interface design
Example Implementation (using JavaScript and HTML5 Canvas):
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Simple Match-3 Puzzle</title>
<style>
canvas { border: 1px solid black; }
</style>
</head>
<body>
<canvas id="gameCanvas" width="400" height="400"></canvas>
<script>
const canvas = document.getElementById('gameCanvas');
const ctx = canvas.getContext('2d');
const GRID_SIZE = 8;
const CELL_SIZE = canvas.width / GRID_SIZE;
const COLORS = ['red', 'blue', 'green', 'yellow', 'purple'];
let grid = [];
let score = 0;
function initializeGrid() {
for (let i = 0; i < GRID_SIZE; i++) {
grid[i] = [];
for (let j = 0; j < GRID_SIZE; j++) {
grid[i][j] = COLORS[Math.floor(Math.random() * COLORS.length)];
}
}
}
function drawGrid() {
for (let i = 0; i < GRID_SIZE; i++) {
for (let j = 0; j < GRID_SIZE; j++) {
ctx.fillStyle = grid[i][j];
ctx.fillRect(i * CELL_SIZE, j * CELL_SIZE, CELL_SIZE, CELL_SIZE);
ctx.strokeRect(i * CELL_SIZE, j * CELL_SIZE, CELL_SIZE, CELL_SIZE);
}
}
}
function checkMatches() {
let matched = false;
// Check horizontal matches
for (let i = 0; i < GRID_SIZE; i++) {
for (let j = 0; j < GRID_SIZE - 2; j++) {
if (grid[i][j] === grid[i][j+1] && grid[i][j] === grid[i][j+2]) {
grid[i][j] = grid[i][j+1] = grid[i][j+2] = null;
score += 3;
matched = true;
}
}
}
// Check vertical matches
for (let i = 0; i < GRID_SIZE - 2; i++) {
for (let j = 0; j < GRID_SIZE; j++) {
if (grid[i][j] && grid[i][j] === grid[i+1][j] && grid[i][j] === grid[i+2][j]) {
grid[i][j] = grid[i+1][j] = grid[i+2][j] = null;
score += 3;
matched = true;
}
}
}
return matched;
}
function fillEmptyCells() {
for (let i = 0; i < GRID_SIZE; i++) {
for (let j = GRID_SIZE - 1; j >= 0; j--) {
if (!grid[i][j]) {
for (let k = j - 1; k >= 0; k--) {
if (grid[i][k]) {
grid[i][j] = grid[i][k];
grid[i][k] = null;
break;
}
}
if (!grid[i][j]) {
grid[i][j] = COLORS[Math.floor(Math.random() * COLORS.length)];
}
}
}
}
}
function gameLoop() {
if (checkMatches()) {
fillEmptyCells();
}
drawGrid();
ctx.fillStyle = 'black';
ctx.font = '20px Arial';
ctx.fillText(`Score: ${score}`, 10, 30);
requestAnimationFrame(gameLoop);
}
canvas.addEventListener('click', (event) => {
const rect = canvas.getBoundingClientRect();
const x = Math.floor((event.clientX - rect.left) / CELL_SIZE);
const y = Math.floor((event.clientY - rect.top) / CELL_SIZE);
// Swap with adjacent cell (right)
if (x < GRID_SIZE - 1) {
[grid[x][y], grid[x+1][y]] = [grid[x+1][y], grid[x][y]];
if (!checkMatches()) {
[grid[x][y], grid[x+1][y]] = [grid[x+1][y], grid[x][y]]; // Swap back if no match
}
}
});
initializeGrid();
gameLoop();
</script>
</body>
</html>
Tower Defense Game
Creating a tower defense game introduces concepts like path finding, resource management, and wave-based gameplay.
Key Concepts:
- Enemy path finding
- Tower placement and upgrading
- Wave management
- Resource economy
These intermediate projects will challenge you to apply your programming skills in more complex scenarios. They introduce new game mechanics and design patterns that are common in many popular games. As you work on these projects, you’ll gain a deeper understanding of game development principles and improve your problem-solving abilities.
7. Advanced Game Development Techniques
As you progress in your game development journey, you’ll encounter more advanced techniques that can take your games to the next level. Here are some advanced concepts to explore:
Artificial Intelligence (AI)
Implementing AI in your games can create more challenging and dynamic gameplay experiences. Some AI techniques to explore include:
- Pathfinding algorithms (e.g., A* algorithm)
- Finite State Machines for enemy behavior
- Decision trees for complex decision-making
- Machine learning for adaptive difficulty
Example: Simple Pathfinding with A* Algorithm
import heapq
def heuristic(a, b):
return abs(b[0] - a[0]) + abs(b[1] - a[1])
def a_star(grid, start, goal):
neighbors = [(0,1), (0,-1), (1,0), (-1,0)]
close_set = set()
came_from = {}
gscore = {start:0}
fscore = {start:heuristic(start, goal)}
oheap = []
heapq.heappush(oheap, (fscore[start], start))
while oheap:
current = heapq.heappop(oheap)[1]
if current == goal:
path = []
while current in came_from:
path.append(current)
current = came_from[current]
path.append(start)
path.reverse()
return path
close_set.add(current)
for i, j in neighbors:
neighbor = current[0] + i, current[1] + j
tentative_g_score = gscore[current] + 1
if 0 <= neighbor[0] < len(grid) and 0 <= neighbor[1] < len(grid[0]):
if grid[neighbor[0]][neighbor[1]] == 1:
continue
if neighbor in close_set and tentative_g_score >= gscore.get(neighbor, 0):
continue
if tentative_g_score < gscore.get(neighbor, 0) or neighbor not in [i[1]for i in oheap]:
came_from[neighbor] = current
gscore[neighbor] = tentative_g_score
fscore[neighbor] = gscore[neighbor] + heuristic(neighbor, goal)
heapq.heappush(oheap, (fscore[neighbor], neighbor))
return False
# Example usage
grid = [
[0, 0, 0, 0, 1],
[1, 1, 0, 1, 0],
[0, 0, 0, 0, 0],
[0, 1, 1, 1, 0],
[0, 0, 0, 1, 0]
]
start = (0, 0)
goal = (4, 4)
path = a_star(grid, start, goal)
print(path)
Procedural Content Generation
Procedural generation allows you to create vast amounts of content algorithmically, which can lead to more varied and replayable games. Areas to explore include:
- Random level generation
- Procedural terrain creation
- Dynamic quest generation
- Procedural texture and model creation
Example: Simple Dungeon Room Generator
import random
def generate_room(width, height):
room = [["#" for _ in range(width)] for _ in range(height)]
# Create floor
for y in range(1, height-1):
for x in range(1, width-1):
room[y][x] = "."
# Add random obstacles
for _ in range(int((width * height) * 0.1)): # 10% of room filled with obstacles
x = random.randint(1, width-2)
y = random.randint(1, height-2)
room[y][x] = "O"
# Add entrance and exit
room[height//2][0] = "E"
room[height//2][width-1] = "X"
return room
def print_room(room):
for row in room:
print(" ".join(row))
# Generate and print a room
room = generate_room(20, 10)
print_room(room)
Networking and Multiplayer
Adding multiplayer capabilities to your games introduces a whole new level of complexity. Key areas to focus on include:
- Client-server architecture
- Network synchronization
- Latency compensation techniques
- Multiplayer game design considerations
Shaders and Advanced Graphics
For visually impressive games, learning about shaders and advanced graphics techniques is crucial:
- Vertex and fragment shaders
- Post-processing effects
- Particle systems
- Physically-based rendering
Example: Simple Vertex Shader in GLSL
#version 330 core
layout (location = 0) in vec3 aPos;
layout (location = 1) in vec3 aColor;
out vec3 vertexColor;
uniform mat4 model;
uniform mat4 view;
uniform mat4 projection;
void main()
{
gl_Position = projection * view * model * vec4(aPos, 1.0);
vertexColor = aColor;
}
Physics Simulation
Implementing realistic physics can greatly enhance gameplay. Areas to explore include: