How to Learn Programming Through Building Games: A Comprehensive Guide
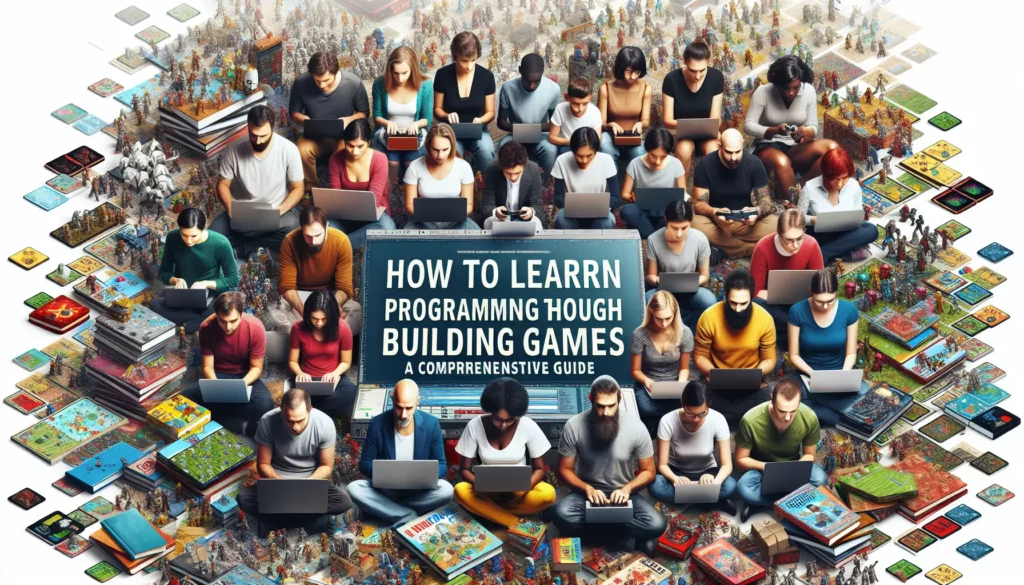
Learning to program can be an exciting journey, and what better way to embark on this adventure than by building games? Game development not only makes the learning process more engaging but also provides a practical application for various programming concepts. In this comprehensive guide, we’ll explore how you can master programming skills by creating games, from simple text-based adventures to more complex graphical experiences.
Table of Contents
- Why Learn Programming Through Games?
- Getting Started: Choosing Your Path
- Beginning with Text-Based Games
- Moving to Simple Graphical Games
- Advancing to More Complex Concepts
- Exploring Game Engines
- Incorporating AI in Games
- Venturing into Multiplayer Games
- Mobile Game Development
- Resources and Communities
- Conclusion
1. Why Learn Programming Through Games?
Game development offers a unique and engaging way to learn programming for several reasons:
- Motivation: Creating games is fun and rewarding, which can keep you motivated throughout the learning process.
- Practical Application: Games provide a tangible output for your code, allowing you to see the results of your work in action.
- Diverse Skill Set: Game development covers a wide range of programming concepts, from basic logic to complex algorithms.
- Problem-Solving: Games often present unique challenges that require creative problem-solving skills.
- Interdisciplinary Learning: Game development can introduce you to other fields like graphics, sound design, and user experience.
2. Getting Started: Choosing Your Path
Before diving into game development, you need to choose a programming language and development environment. Here are some popular options for beginners:
- Python: Known for its simplicity and readability, Python is an excellent choice for beginners. Libraries like Pygame make it easy to create simple games.
- JavaScript: With HTML5 and Canvas, JavaScript allows you to create browser-based games without additional software.
- C#: If you’re interested in using Unity, one of the most popular game engines, C# is the primary language you’ll need to learn.
- Lua: Often used in game modding and in game engines like LÖVE, Lua is another beginner-friendly option.
Choose a language based on your goals, the types of games you want to create, and the resources available to you.
3. Beginning with Text-Based Games
Text-based games are an excellent starting point for beginners. They allow you to focus on core programming concepts without worrying about graphics or complex user interfaces. Here’s a simple example of a text-based game in Python:
def guessing_game():
import random
number = random.randint(1, 100)
attempts = 0
print("Welcome to the Guessing Game!")
print("I'm thinking of a number between 1 and 100.")
while True:
guess = int(input("Enter your guess: "))
attempts += 1
if guess < number:
print("Too low! Try again.")
elif guess > number:
print("Too high! Try again.")
else:
print(f"Congratulations! You guessed the number in {attempts} attempts!")
break
guessing_game()
This simple game introduces concepts like:
- Variables and data types
- Input/output operations
- Conditional statements
- Loops
- Functions
- Importing and using modules
As you become more comfortable with these basics, you can expand your text-based games to include more complex features like inventory systems, multiple endings, or even simple combat mechanics.
4. Moving to Simple Graphical Games
Once you’ve mastered the basics with text-based games, it’s time to add some visual elements. Libraries like Pygame for Python or the HTML5 Canvas with JavaScript can help you create simple 2D games. Here’s a basic example of a moving rectangle in Pygame:
import pygame
import sys
# Initialize Pygame
pygame.init()
# Set up the display
width, height = 640, 480
screen = pygame.display.set_mode((width, height))
pygame.display.set_caption("Moving Rectangle")
# Set up the rectangle
rect_x, rect_y = 300, 200
rect_width, rect_height = 50, 50
rect_speed = 5
# Main game loop
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# Move the rectangle based on key presses
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
rect_x -= rect_speed
if keys[pygame.K_RIGHT]:
rect_x += rect_speed
if keys[pygame.K_UP]:
rect_y -= rect_speed
if keys[pygame.K_DOWN]:
rect_y += rect_speed
# Clear the screen
screen.fill((255, 255, 255))
# Draw the rectangle
pygame.draw.rect(screen, (255, 0, 0), (rect_x, rect_y, rect_width, rect_height))
# Update the display
pygame.display.flip()
# Control the frame rate
pygame.time.Clock().tick(60)
This example introduces new concepts such as:
- Game loops
- Event handling
- Drawing shapes and managing screen updates
- Controlling frame rate
From here, you can start adding more elements like collision detection, sprite animations, and simple physics to create more engaging games.
5. Advancing to More Complex Concepts
As you progress, you’ll want to incorporate more advanced programming concepts into your games. Some areas to explore include:
- Object-Oriented Programming (OOP): OOP is crucial for organizing code in larger games. It allows you to create reusable game objects with their own properties and behaviors.
- Data Structures: Efficient use of data structures like arrays, lists, dictionaries, and trees can significantly improve your game’s performance.
- Algorithms: Understanding and implementing algorithms for pathfinding, sorting, and searching can enhance your game’s functionality.
- Design Patterns: Familiarize yourself with common game design patterns like the game loop, component pattern, and observer pattern.
Here’s an example of how you might use OOP to create a simple spaceship class in Python:
import pygame
import random
class Spaceship:
def __init__(self, x, y):
self.x = x
self.y = y
self.speed = 5
self.health = 100
self.image = pygame.image.load("spaceship.png")
def move(self, direction):
if direction == "left":
self.x -= self.speed
elif direction == "right":
self.x += self.speed
elif direction == "up":
self.y -= self.speed
elif direction == "down":
self.y += self.speed
def draw(self, screen):
screen.blit(self.image, (self.x, self.y))
def take_damage(self, amount):
self.health -= amount
if self.health < 0:
self.health = 0
# Usage in a game loop
player_ship = Spaceship(300, 400)
while True:
# ... (event handling and other game logic)
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
player_ship.move("left")
if keys[pygame.K_RIGHT]:
player_ship.move("right")
player_ship.draw(screen)
# ... (screen updates and frame rate control)
6. Exploring Game Engines
As your projects become more complex, you might want to explore game engines. These provide a framework for game development, handling many low-level details and providing tools for graphics, sound, physics, and more. Some popular game engines include:
- Unity: A versatile engine that uses C# for scripting. It’s great for both 2D and 3D games and has a large community.
- Unreal Engine: Known for its high-quality graphics, it uses C++ or Blueprint (a visual scripting language).
- Godot: An open-source engine that uses its own scripting language (GDScript) or C#.
- Phaser: A JavaScript framework for creating HTML5 games, ideal for web-based game development.
Learning a game engine involves understanding its architecture, workflow, and specific scripting language. Here’s a simple example of a Unity script in C# that makes an object move:
using UnityEngine;
public class Mover : MonoBehaviour
{
public float speed = 5f;
void Update()
{
float horizontalInput = Input.GetAxis("Horizontal");
float verticalInput = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(horizontalInput, 0f, verticalInput);
transform.Translate(movement * speed * Time.deltaTime);
}
}
7. Incorporating AI in Games
Artificial Intelligence can add depth and challenge to your games. Some AI concepts you might want to explore include:
- Pathfinding: Algorithms like A* for navigating game worlds.
- Decision Making: Techniques like decision trees or behavior trees for NPC actions.
- Machine Learning: Using neural networks or reinforcement learning for adaptive AI.
Here’s a simple example of a basic AI that chases the player in Python:
import math
class Enemy:
def __init__(self, x, y):
self.x = x
self.y = y
self.speed = 2
def move_towards_player(self, player_x, player_y):
dx = player_x - self.x
dy = player_y - self.y
distance = math.sqrt(dx**2 + dy**2)
if distance != 0:
dx /= distance
dy /= distance
self.x += dx * self.speed
self.y += dy * self.speed
# Usage in game loop
enemy = Enemy(100, 100)
player_x, player_y = 300, 300
while True:
# ... (other game logic)
enemy.move_towards_player(player_x, player_y)
# ... (drawing and updates)
8. Venturing into Multiplayer Games
Multiplayer games introduce new challenges and concepts:
- Networking: Understanding client-server architecture or peer-to-peer connections.
- Synchronization: Keeping game states consistent across multiple clients.
- Latency Handling: Dealing with network delays and packet loss.
Here’s a basic example of a server for a simple multiplayer game using Python’s socket library:
import socket
import threading
class GameServer:
def __init__(self, host, port):
self.host = host
self.port = port
self.server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
self.clients = []
def start(self):
self.server_socket.bind((self.host, self.port))
self.server_socket.listen()
print(f"Server started on {self.host}:{self.port}")
while True:
client_socket, address = self.server_socket.accept()
print(f"New connection from {address}")
self.clients.append(client_socket)
client_thread = threading.Thread(target=self.handle_client, args=(client_socket,))
client_thread.start()
def handle_client(self, client_socket):
while True:
try:
message = client_socket.recv(1024).decode('utf-8')
if message:
print(f"Received: {message}")
self.broadcast(message, client_socket)
else:
self.remove_client(client_socket)
break
except:
self.remove_client(client_socket)
break
def broadcast(self, message, sender_socket):
for client in self.clients:
if client != sender_socket:
try:
client.send(message.encode('utf-8'))
except:
self.remove_client(client)
def remove_client(self, client_socket):
if client_socket in self.clients:
self.clients.remove(client_socket)
client_socket.close()
# Usage
server = GameServer('localhost', 5555)
server.start()
9. Mobile Game Development
Mobile game development introduces its own set of challenges and considerations:
- Touch Controls: Designing intuitive touch-based interfaces.
- Performance Optimization: Ensuring smooth performance on limited hardware.
- Cross-Platform Development: Creating games that work on both iOS and Android.
- Monetization: Implementing in-app purchases or ads effectively.
Many game engines like Unity and Unreal Engine support mobile development. Alternatively, you can use mobile-specific frameworks like React Native with a game engine like Phaser.
10. Resources and Communities
To support your learning journey, consider these resources:
- Online Courses: Platforms like Coursera, Udemy, and edX offer game development courses.
- Documentation: Official documentation for your chosen language or game engine is invaluable.
- Game Jams: Participate in game jams to practice your skills and meet other developers.
- Forums and Communities: Join communities like r/gamedev on Reddit or the GameDev.net forums.
- YouTube Tutorials: Channels like Brackeys, Sebastian Lague, and GDQuest offer excellent tutorials.
- Books: “Game Programming Patterns” by Robert Nystrom and “The Art of Game Design” by Jesse Schell are great reads.
11. Conclusion
Learning programming through game development is an exciting and rewarding journey. It offers a unique blend of creativity and technical skills, allowing you to see the immediate results of your code in action. As you progress from simple text-based games to complex multiplayer experiences, you’ll not only learn programming concepts but also develop problem-solving skills, design thinking, and project management abilities.
Remember that game development is a vast field, and it’s okay to start small. Each game you create, no matter how simple, is a stepping stone in your learning process. Don’t be afraid to experiment, make mistakes, and most importantly, have fun! The skills you develop through game programming are transferable to many other areas of software development, making this an excellent path for aspiring programmers.
As you continue your journey, consider joining game development communities, participating in game jams, and sharing your creations with others. The feedback and support from fellow developers can be invaluable in your growth as a programmer and game developer.
Whether your goal is to become a professional game developer or simply to enhance your programming skills, building games provides a fun and engaging way to learn. So, fire up your IDE, choose a project that excites you, and start coding. Your adventure in game development and programming awaits!