How to Learn Programming Logic and Fundamentals: A Comprehensive Guide
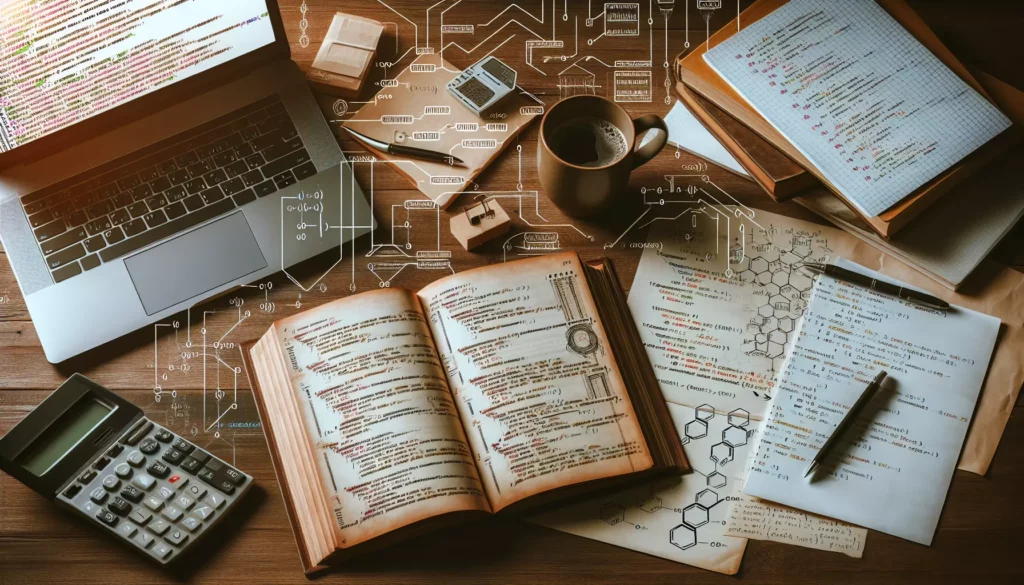
In today’s digital age, programming has become an essential skill across various industries. Whether you’re aspiring to become a software developer, data scientist, or simply want to enhance your problem-solving abilities, understanding programming logic and fundamentals is crucial. This comprehensive guide will walk you through the process of learning these vital skills, providing you with a solid foundation for your coding journey.
Table of Contents
- Understanding Programming Logic
- Key Programming Fundamentals
- Starting Your Learning Journey
- Mastering Basic Concepts
- Practicing Problem-Solving
- Exploring Advanced Topics
- Leveraging Online Resources
- Joining Coding Communities
- Building Projects
- Preparing for Technical Interviews
- Conclusion
Understanding Programming Logic
Programming logic is the foundation of all coding skills. It refers to the ability to think systematically and solve problems step-by-step using a structured approach. Understanding programming logic helps you break down complex problems into smaller, manageable parts and develop efficient solutions.
Key aspects of programming logic include:
- Algorithmic thinking: The ability to create step-by-step procedures for solving problems.
- Logical reasoning: Using if-then statements and boolean logic to make decisions in your code.
- Pattern recognition: Identifying recurring patterns and applying reusable solutions.
- Abstraction: Simplifying complex systems by focusing on essential details.
To develop your programming logic skills, start by solving simple puzzles and brain teasers. Gradually move on to more complex problems, always focusing on breaking them down into smaller, manageable steps.
Key Programming Fundamentals
Before diving into any specific programming language, it’s essential to understand the core concepts that are common across most languages. These fundamentals will help you grasp new languages more quickly and write more efficient code.
Some key programming fundamentals include:
- Variables and data types: Understanding how to store and manipulate different types of data.
- Control structures: Using conditional statements (if-else) and loops (for, while) to control program flow.
- Functions and methods: Creating reusable blocks of code to perform specific tasks.
- Arrays and data structures: Organizing and storing collections of data efficiently.
- Object-oriented programming (OOP): Understanding concepts like classes, objects, inheritance, and polymorphism.
- Algorithms and complexity: Learning common algorithms and analyzing their efficiency.
- Version control: Using tools like Git to manage and track changes in your code.
As you learn these fundamentals, focus on understanding the underlying concepts rather than memorizing syntax. This approach will make it easier to adapt to different programming languages in the future.
Starting Your Learning Journey
Now that you understand the importance of programming logic and fundamentals, it’s time to start your learning journey. Here are some steps to get you started:
- Choose a beginner-friendly language: Python, JavaScript, or Ruby are excellent choices for beginners due to their readable syntax and extensive learning resources.
- Set up your development environment: Install the necessary tools and software for your chosen language.
- Find learning resources: Look for online courses, tutorials, and books that cater to beginners.
- Start with the basics: Begin with simple concepts like printing “Hello, World!” and gradually progress to more complex topics.
- Practice regularly: Consistency is key when learning to code. Aim to practice for at least 30 minutes every day.
- Join a community: Connect with other learners through online forums, local meetups, or coding bootcamps.
Remember, learning to code is a journey, not a destination. Be patient with yourself and celebrate small victories along the way.
Mastering Basic Concepts
As you begin your coding journey, focus on mastering these essential concepts:
1. Variables and Data Types
Learn how to declare variables and work with different data types such as integers, floating-point numbers, strings, and booleans. Understand how to perform operations on these data types and convert between them when necessary.
2. Control Structures
Master the use of if-else statements for conditional execution and loops (for and while) for repetitive tasks. These structures are fundamental to controlling the flow of your programs.
3. Functions
Learn how to define and call functions, pass arguments, and return values. Understanding functions is crucial for writing modular and reusable code.
4. Basic Input/Output
Practice reading input from users and displaying output in various formats. This skill is essential for creating interactive programs.
5. Error Handling
Learn about common errors and exceptions, and how to handle them gracefully in your code.
Here’s a simple Python example that incorporates some of these basic concepts:
def calculate_area(length, width):
if length <= 0 or width <= 0:
raise ValueError("Length and width must be positive numbers")
return length * width
try:
length = float(input("Enter the length: "))
width = float(input("Enter the width: "))
area = calculate_area(length, width)
print(f"The area of the rectangle is: {area}")
except ValueError as e:
print(f"Error: {e}")
except Exception as e:
print(f"An unexpected error occurred: {e}")
This example demonstrates variables, functions, input/output, error handling, and conditional statements. Practice writing similar programs to reinforce your understanding of these concepts.
Practicing Problem-Solving
Problem-solving is at the heart of programming. To improve your skills, engage in regular coding exercises and challenges. Here are some ways to practice:
- Coding challenges: Websites like LeetCode, HackerRank, and CodeWars offer a wide range of programming problems to solve.
- Project Euler: This website provides a series of challenging mathematical/computer programming problems.
- Coding competitions: Participate in online coding contests to test your skills against others and learn new techniques.
- Daily coding problems: Subscribe to newsletters or services that send daily coding problems to your inbox.
- Reverse engineering: Try to recreate simple games or applications to understand how they work.
When solving problems, follow these steps:
- Understand the problem thoroughly
- Plan your approach (pseudocode can be helpful)
- Implement your solution
- Test and debug your code
- Optimize and refactor if necessary
- Reflect on what you’ve learned
Remember, the goal is not just to solve the problem but to understand the underlying concepts and improve your problem-solving skills.
Exploring Advanced Topics
As you become more comfortable with the basics, start exploring more advanced programming concepts. These topics will help you write more efficient, maintainable, and scalable code:
1. Data Structures
Learn about arrays, linked lists, stacks, queues, trees, and graphs. Understanding these structures is crucial for efficient data management and algorithm implementation.
2. Algorithms
Study common algorithms for searching, sorting, and graph traversal. Analyze their time and space complexity to understand when to use each algorithm.
3. Object-Oriented Programming (OOP)
Dive deeper into OOP concepts such as encapsulation, inheritance, and polymorphism. Learn how to design and implement classes effectively.
4. Design Patterns
Familiarize yourself with common design patterns like Singleton, Factory, and Observer. These patterns provide reusable solutions to common software design problems.
5. Concurrency and Parallelism
Learn about threads, processes, and asynchronous programming to write more efficient and responsive applications.
6. Database Management
Understand how to work with databases, including SQL and NoSQL options. Learn about database design, querying, and optimization.
7. Web Development
Explore front-end and back-end web development technologies, including HTML, CSS, JavaScript, and popular frameworks like React or Django.
Here’s an example of a more advanced concept, demonstrating a simple implementation of the Observer design pattern in Python:
from abc import ABC, abstractmethod
class Subject:
def __init__(self):
self._observers = []
self._state = None
def attach(self, observer):
self._observers.append(observer)
def detach(self, observer):
self._observers.remove(observer)
def notify(self):
for observer in self._observers:
observer.update(self._state)
def set_state(self, state):
self._state = state
self.notify()
class Observer(ABC):
@abstractmethod
def update(self, state):
pass
class ConcreteObserverA(Observer):
def update(self, state):
print(f"ConcreteObserverA: Reacted to the state change: {state}")
class ConcreteObserverB(Observer):
def update(self, state):
print(f"ConcreteObserverB: Reacted to the state change: {state}")
# Usage
subject = Subject()
observer_a = ConcreteObserverA()
subject.attach(observer_a)
observer_b = ConcreteObserverB()
subject.attach(observer_b)
subject.set_state("New State")
subject.detach(observer_a)
subject.set_state("Another New State")
This example demonstrates the Observer pattern, which is commonly used in event-driven programming and user interface design. As you explore these advanced topics, try to implement them in your own projects to solidify your understanding.
Leveraging Online Resources
The internet is a treasure trove of resources for learning programming. Here are some valuable online platforms and tools to aid your learning:
1. Online Courses and MOOCs
- Coursera: Offers courses from top universities on various programming topics.
- edX: Provides free courses from institutions like MIT and Harvard.
- Udacity: Offers nanodegree programs focused on specific tech skills.
- freeCodeCamp: Provides a comprehensive, project-based curriculum for web development.
2. Interactive Coding Platforms
- Codecademy: Offers interactive coding lessons in various languages.
- DataCamp: Focuses on data science and analytics programming.
- AlgoCademy: Provides interactive coding tutorials and AI-powered assistance for algorithmic problem-solving.
3. Video Tutorials
- YouTube: Channels like Traversy Media, The Net Ninja, and CS Dojo offer free programming tutorials.
- Pluralsight: Provides in-depth video courses on various programming topics.
4. Documentation and Reference Materials
- Official language documentation: Always refer to the official docs for your chosen language.
- MDN Web Docs: Excellent resource for web development technologies.
- Stack Overflow: A community-driven Q&A platform for programming-related questions.
5. Coding Challenge Websites
- LeetCode: Offers a wide range of coding challenges, often used for interview preparation.
- HackerRank: Provides challenges in various domains and languages.
- CodeWars: Gamifies the learning process with coding challenges called “katas”.
When using these resources, create a structured learning plan. Combine theoretical learning with practical coding exercises and projects to reinforce your understanding.
Joining Coding Communities
Connecting with other programmers can significantly enhance your learning experience. Here are some ways to engage with coding communities:
1. Online Forums and Discussion Boards
- Reddit: Join programming-related subreddits like r/learnprogramming or language-specific communities.
- Stack Overflow: Participate in discussions and help others to reinforce your own knowledge.
- Dev.to: A community of developers sharing ideas and helping each other.
2. Social Media
- Twitter: Follow developers, join coding-related discussions using hashtags like #CodeNewbie or #100DaysOfCode.
- LinkedIn: Connect with professionals in the tech industry and join programming-related groups.
3. Local Meetups and Events
- Meetup.com: Find local coding meetups and tech events in your area.
- Hackathons: Participate in coding competitions to challenge yourself and network with others.
4. Open Source Contributions
- GitHub: Contribute to open-source projects to gain real-world experience and collaborate with other developers.
- Open Source Initiative: Find open-source projects that align with your interests and skills.
5. Online Coding Communities
- Discord: Join programming-related Discord servers to chat with other developers in real-time.
- Slack: Many coding communities have Slack channels for discussions and support.
Engaging with these communities can provide support, motivation, and valuable insights from experienced developers. Don’t be afraid to ask questions and share your own knowledge as you progress in your learning journey.
Building Projects
One of the most effective ways to learn programming is by building your own projects. Projects allow you to apply your knowledge, explore new concepts, and create something tangible. Here are some tips for project-based learning:
1. Start Small
Begin with simple projects that you can complete in a few hours or days. As you gain confidence, gradually increase the complexity of your projects.
2. Choose Projects That Interest You
Select project ideas that align with your interests or solve a problem you’ve encountered. This will keep you motivated throughout the development process.
3. Explore Different Domains
Try projects in various areas such as web development, mobile apps, data analysis, or game development to broaden your skills.
4. Use Version Control
Practice using Git and GitHub to manage your project’s code. This is an essential skill for collaborative development.
5. Document Your Work
Write clear documentation for your projects, including setup instructions and usage guidelines. This is valuable practice for real-world development.
6. Seek Feedback
Share your projects with peers or mentors and ask for constructive feedback to improve your code and design.
Project Ideas for Beginners:
- To-do list application
- Simple calculator
- Weather app using an API
- Basic blog system
- Tic-tac-toe game
Intermediate Project Ideas:
- Social media dashboard
- E-commerce website
- Chat application
- Data visualization tool
- Simple mobile app
Remember, the goal is not just to complete the project but to learn and grow throughout the development process. Don’t be afraid to tackle challenges that seem slightly beyond your current skill level – this is how you’ll improve fastest.
Preparing for Technical Interviews
As you progress in your programming journey, you may start considering career opportunities in the tech industry. Technical interviews are a common part of the hiring process for programming roles, especially at major tech companies (often referred to as FAANG – Facebook, Amazon, Apple, Netflix, Google). Here are some tips to help you prepare:
1. Master Data Structures and Algorithms
Focus on understanding and implementing common data structures (arrays, linked lists, trees, graphs) and algorithms (sorting, searching, dynamic programming). Practice solving problems that involve these concepts.
2. Practice Coding Challenges
Use platforms like LeetCode, HackerRank, or AlgoCademy to solve coding challenges regularly. Focus on medium to hard difficulty problems as you improve.
3. Study System Design
For more senior roles, understand how to design scalable systems. Study topics like load balancing, caching, database sharding, and microservices architecture.
4. Mock Interviews
Practice with friends or use platforms like Pramp or InterviewBit that offer mock interview sessions with peers.
5. Behavioral Questions
Prepare for non-technical questions about your experience, problem-solving approach, and teamwork skills. Use the STAR method (Situation, Task, Action, Result) to structure your responses.
6. Learn About the Company
Research the company you’re interviewing with. Understand their products, culture, and recent news. This shows genuine interest and helps you ask informed questions.
7. Improve Your Communication Skills
Practice explaining your thought process clearly while solving problems. This is crucial during technical interviews.
8. Review Your Projects
Be prepared to discuss projects you’ve worked on in detail, including challenges faced and how you overcame them.
9. Stay Updated with Industry Trends
Keep up with the latest developments in programming languages, frameworks, and technologies relevant to your field.
10. Don’t Neglect Soft Skills
Develop skills like problem-solving, teamwork, and adaptability. These are highly valued in the tech industry.
Remember, interview preparation is an ongoing process. Start early and make it a part of your regular learning routine. With consistent practice and a solid understanding of programming fundamentals, you’ll be well-prepared to tackle technical interviews at top tech companies.
Conclusion
Learning programming logic and fundamentals is a journey that requires dedication, patience, and consistent practice. By following the steps outlined in this guide – from understanding basic concepts to building projects and preparing for technical interviews – you’ll be well on your way to becoming a proficient programmer.
Remember these key points as you continue your learning journey:
- Focus on understanding core concepts rather than memorizing syntax.
- Practice regularly through coding challenges and personal projects.
- Engage with coding communities for support and knowledge sharing.
- Stay curious and keep learning about new technologies and best practices.
- Don’t be afraid to make mistakes – they’re an essential part of the learning process.
With the right mindset and approach, you can master programming logic and fundamentals, opening up a world of opportunities in the ever-evolving field of technology. Whether your goal is to become a professional software developer, enhance your problem-solving skills, or simply explore the world of coding, the skills you develop will be invaluable in today’s digital landscape.
Remember, every expert was once a beginner. Stay persistent, embrace the challenges, and enjoy the process of becoming a skilled programmer. The journey of learning to code is as rewarding as the destination itself. Happy coding!