How to Learn Mobile App Development for Android and iOS: A Comprehensive Guide
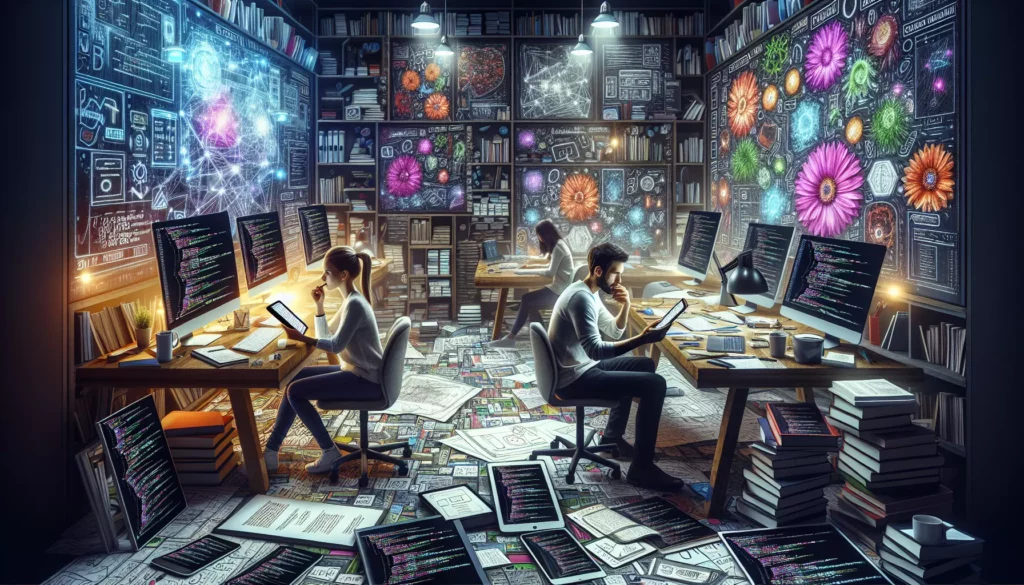
In today’s digital age, mobile applications have become an integral part of our daily lives. From social media and productivity tools to entertainment and e-commerce platforms, mobile apps are everywhere. As a result, the demand for skilled mobile app developers continues to grow. If you’re interested in joining this exciting field, you’re in the right place. This comprehensive guide will walk you through the process of learning mobile app development for both Android and iOS platforms.
Table of Contents
- Introduction to Mobile App Development
- Choosing Your Platform: Android vs. iOS
- Prerequisites and Foundational Knowledge
- Getting Started with Android Development
- Getting Started with iOS Development
- Cross-Platform Development Options
- Learning Resources and Tools
- Best Practices and Tips
- Building Your Portfolio
- Staying Updated in the Mobile App Development World
- Conclusion
1. Introduction to Mobile App Development
Mobile app development is the process of creating software applications that run on mobile devices such as smartphones and tablets. These apps can be pre-installed on phones, downloaded from app stores, or accessed through mobile web browsers. The two dominant platforms in the mobile app market are Android and iOS, which together account for over 99% of the global mobile operating system market share.
Learning mobile app development opens up a world of opportunities. You can create apps for personal use, start a business, or pursue a career in tech companies ranging from startups to giants like Google, Apple, or Facebook. The skills you acquire in mobile app development are also transferable to other areas of software development, making it a valuable addition to your skill set.
2. Choosing Your Platform: Android vs. iOS
Before diving into mobile app development, you need to decide which platform you want to focus on initially. Both Android and iOS have their unique characteristics:
Android Development:
- Larger global market share
- More diverse range of devices
- Lower barrier to entry for publishing apps
- Primary programming language: Java or Kotlin
- Development environment: Android Studio
iOS Development:
- Higher revenue potential per user
- More standardized ecosystem
- Stricter app store guidelines
- Primary programming language: Swift (or Objective-C)
- Development environment: Xcode
While it’s beneficial to eventually learn both platforms, starting with one allows you to focus your learning and become proficient more quickly. Choose based on your personal preferences, target audience, or career goals.
3. Prerequisites and Foundational Knowledge
Before jumping into mobile app development, it’s crucial to have a solid foundation in programming concepts. Here are some prerequisites that will make your learning journey smoother:
- Basic programming concepts: Variables, data types, control structures (if/else, loops), functions, and object-oriented programming principles.
- Understanding of algorithms and data structures: Knowledge of common algorithms and data structures will help you write more efficient code.
- Familiarity with version control systems: Git is widely used in the industry for collaboration and code management.
- Basic understanding of databases: Many apps require data storage and retrieval.
- HTML, CSS, and JavaScript: Useful for creating hybrid apps or understanding web views within native apps.
If you’re new to programming or need to brush up on these concepts, platforms like AlgoCademy offer interactive coding tutorials and resources to help you build a strong foundation in algorithmic thinking and problem-solving skills.
4. Getting Started with Android Development
If you’ve chosen to start with Android development, here’s a step-by-step guide to get you started:
Step 1: Learn Java or Kotlin
Java has been the traditional language for Android development, but Kotlin is now the preferred language by Google. Both are valid choices:
- Java: A widely-used, object-oriented language with a large community and extensive resources.
- Kotlin: A more modern language that’s interoperable with Java, offering more concise syntax and additional features.
Step 2: Set Up Your Development Environment
Download and install Android Studio, the official Integrated Development Environment (IDE) for Android development. It includes everything you need to start building Android apps.
Step 3: Learn Android Fundamentals
Familiarize yourself with key Android concepts:
- Activity and Fragment lifecycles
- Layouts and UI design
- Intents and navigation
- Android Jetpack libraries
- Data storage options
Step 4: Build Your First Android App
Start with a simple app, like a to-do list or a calculator, to apply what you’ve learned. Here’s a basic example of creating a “Hello World” app in Kotlin:
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
import android.widget.TextView
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val textView = findViewById<TextView>(R.id.textView)
textView.text = "Hello, World!"
}
}
Step 5: Explore Advanced Topics
As you progress, delve into more advanced areas:
- Material Design principles
- Networking and APIs
- Multithreading and background processing
- Testing and debugging
- Publishing to the Google Play Store
5. Getting Started with iOS Development
If you’ve decided to focus on iOS development, follow these steps to begin your journey:
Step 1: Learn Swift
Swift is the modern programming language for iOS development. While Objective-C is still used in some legacy projects, Swift is the recommended language for new iOS apps.
Step 2: Set Up Your Development Environment
You’ll need a Mac computer to develop iOS apps. Download and install Xcode, Apple’s IDE for iOS development, from the Mac App Store.
Step 3: Learn iOS Fundamentals
Familiarize yourself with key iOS concepts:
- UIKit framework
- View controllers and the view hierarchy
- Auto Layout for responsive UI design
- Swift UI (for modern UI development)
- Core Data for local data storage
Step 4: Build Your First iOS App
Start with a simple app to apply your knowledge. Here’s a basic example of creating a “Hello World” app in Swift:
import UIKit
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
let label = UILabel(frame: CGRect(x: 0, y: 0, width: 200, height: 21))
label.center = CGPoint(x: 160, y: 285)
label.textAlignment = .center
label.text = "Hello, World!"
self.view.addSubview(label)
}
}
Step 5: Explore Advanced Topics
As you become more comfortable, explore advanced areas:
- Networking with URLSession
- Concurrency and Grand Central Dispatch
- Core Animation for advanced UI effects
- Unit testing and UI testing
- Publishing to the App Store
6. Cross-Platform Development Options
While native development for Android and iOS offers the best performance and access to platform-specific features, cross-platform development frameworks allow you to write code once and deploy to multiple platforms. Some popular options include:
- React Native: Developed by Facebook, uses JavaScript and React.
- Flutter: Created by Google, uses the Dart programming language.
- Xamarin: A Microsoft-owned framework that uses C#.
- Ionic: Uses web technologies like HTML, CSS, and JavaScript.
These frameworks can be a good choice if you want to develop for both Android and iOS simultaneously or if you have prior experience with web development.
7. Learning Resources and Tools
To support your learning journey, take advantage of these resources:
- Official Documentation: Android Developers website and Apple Developer website provide comprehensive guides and API references.
- Online Courses: Platforms like Coursera, Udacity, and edX offer structured courses on mobile app development.
- YouTube Tutorials: Channels like Coding with Mitch, Ray Wenderlich, and Stanford’s CS193p course offer free video tutorials.
- Books: “Android Programming: The Big Nerd Ranch Guide” and “iOS Programming: The Big Nerd Ranch Guide” are excellent resources for beginners.
- Coding Challenges: Websites like AlgoCademy offer coding challenges and algorithmic problems to improve your problem-solving skills.
- Community Forums: Stack Overflow, Reddit communities (r/androiddev, r/iOSProgramming), and GitHub discussions are great places to ask questions and learn from others.
8. Best Practices and Tips
As you learn mobile app development, keep these best practices in mind:
- Follow platform-specific design guidelines: Adhere to Material Design for Android and Human Interface Guidelines for iOS to create intuitive, platform-consistent user interfaces.
- Write clean, maintainable code: Follow coding standards, use meaningful variable names, and comment your code appropriately.
- Optimize for performance: Mobile devices have limited resources, so efficient code and optimized assets are crucial.
- Implement proper error handling: Anticipate and handle potential errors to create a robust, user-friendly app.
- Consider security: Implement proper data encryption, secure network communications, and follow platform security best practices.
- Test thoroughly: Conduct unit tests, integration tests, and user interface tests to ensure your app functions correctly across different devices and scenarios.
- Stay updated: Mobile platforms evolve rapidly. Keep up with the latest updates, deprecations, and new features.
9. Building Your Portfolio
As you learn and practice mobile app development, it’s crucial to build a portfolio that showcases your skills. Here are some tips for creating an impressive portfolio:
- Diverse Projects: Create a variety of apps that demonstrate different skills and features. This could include a simple utility app, a game, a social media app, or an e-commerce app.
- Polish Your Work: Even if your apps are simple, ensure they are well-designed, bug-free, and provide a good user experience.
- Open Source Contributions: Contributing to open-source projects can demonstrate your ability to work with existing codebases and collaborate with other developers.
- GitHub Profile: Maintain an active GitHub profile with your projects. Use clear README files to explain your projects, the technologies used, and any challenges you overcame.
- App Store Presence: If possible, publish your apps on the Google Play Store or Apple App Store. This shows that you can take a project from conception to completion.
- Personal Website: Create a personal website or online portfolio that showcases your projects, skills, and any relevant certifications or courses you’ve completed.
Remember, quality is more important than quantity. A few well-executed projects can be more impressive than many incomplete or poorly implemented ones.
10. Staying Updated in the Mobile App Development World
The field of mobile app development is constantly evolving. To stay competitive and up-to-date, consider the following strategies:
- Follow Industry News: Subscribe to blogs, newsletters, and YouTube channels that cover mobile development news and trends.
- Attend Conferences and Meetups: Events like Google I/O, Apple’s WWDC, and local developer meetups are great for learning about new technologies and networking.
- Experiment with New Features: When new APIs or tools are released, try incorporating them into your projects to gain hands-on experience.
- Continuous Learning: Regularly take online courses or read books to deepen your knowledge and learn about emerging technologies in mobile development.
- Engage with the Community: Participate in online forums, contribute to open-source projects, or write blog posts about your experiences and learnings.
Some emerging trends to keep an eye on include:
- Augmented Reality (AR) and Virtual Reality (VR) in mobile apps
- Internet of Things (IoT) integration
- AI and Machine Learning capabilities in mobile devices
- Progressive Web Apps (PWAs)
- 5G technology and its impact on mobile app capabilities
11. Conclusion
Learning mobile app development for Android and iOS is an exciting journey that opens up numerous opportunities in the tech industry. While the learning curve may seem steep at first, with dedication, practice, and the right resources, you can become proficient in creating powerful and user-friendly mobile applications.
Remember that becoming a skilled mobile app developer is not just about learning programming languages and frameworks. It’s also about understanding user needs, staying updated with industry trends, and continuously improving your problem-solving skills. Platforms like AlgoCademy can be invaluable in honing your algorithmic thinking and coding skills, which are fundamental to becoming a successful developer.
As you progress in your learning journey, don’t be afraid to take on challenging projects, contribute to open-source initiatives, or even start building your own app ideas. The mobile app development field is vast and varied, offering opportunities for creativity, innovation, and impactful work.
Whether you choose to specialize in Android, iOS, or cross-platform development, the skills you acquire will be highly valuable in today’s digital-first world. So, start coding, stay curious, and enjoy the process of bringing your ideas to life through mobile apps!