How to Learn Mobile App Development for Android and iOS: A Comprehensive Guide
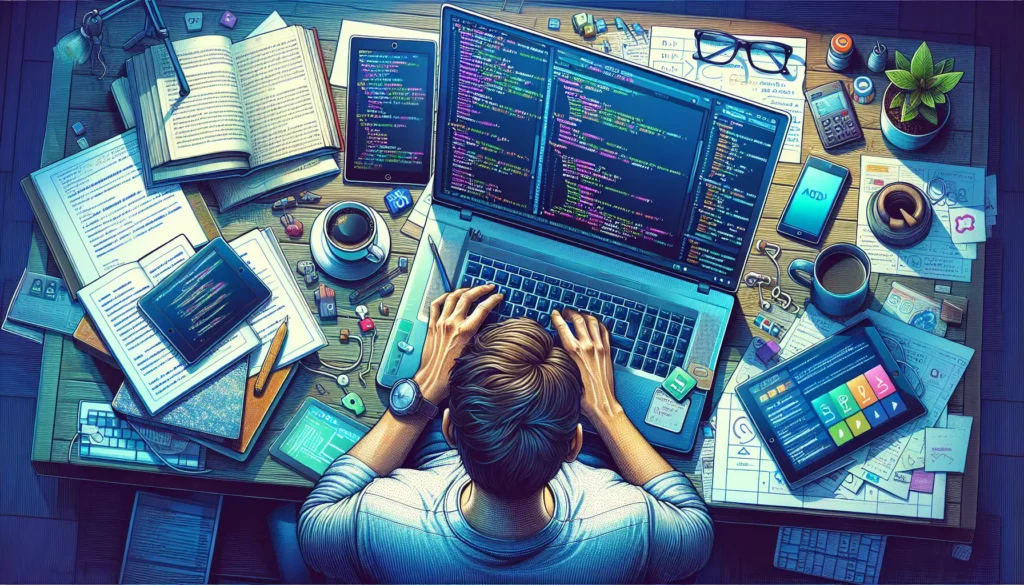
In today’s digital age, mobile applications have become an integral part of our daily lives. From social media and productivity tools to entertainment and e-commerce platforms, mobile apps are everywhere. As a result, the demand for skilled mobile app developers continues to grow, making it an attractive career path for many aspiring programmers. If you’re interested in learning mobile app development for Android and iOS, you’ve come to the right place. This comprehensive guide will walk you through the steps to become proficient in mobile app development, regardless of your current skill level.
Table of Contents
- Understanding the Basics
- Choosing Your Platform: Android vs. iOS
- Learning the Essential Programming Languages
- Familiarizing Yourself with Development Tools
- Mastering UI/UX Design Principles
- Learning App Architecture and Design Patterns
- Working with APIs and Third-party Libraries
- Database Management and Data Storage
- Testing and Debugging Your Apps
- Publishing Your Apps
- Staying Updated with the Latest Trends
- Building Your Portfolio
- Joining the Developer Community
- Continuous Learning and Improvement
- Conclusion
1. Understanding the Basics
Before diving into mobile app development, it’s crucial to have a solid foundation in programming concepts. If you’re new to coding, start by learning the basics of computer science and programming logic. Some key concepts to understand include:
- Variables and data types
- Control structures (if-else statements, loops)
- Functions and methods
- Object-oriented programming (OOP) principles
- Data structures and algorithms
Platforms like AlgoCademy offer interactive coding tutorials and resources that can help you grasp these fundamental concepts. Their focus on algorithmic thinking and problem-solving skills will provide you with a strong foundation for mobile app development.
2. Choosing Your Platform: Android vs. iOS
When it comes to mobile app development, you have two primary platforms to choose from: Android and iOS. Each platform has its own set of development tools, programming languages, and design guidelines. Here’s a brief overview of both:
Android Development
- Primary programming languages: Java and Kotlin
- Development environment: Android Studio
- Larger market share globally
- More diverse device ecosystem
iOS Development
- Primary programming languages: Swift and Objective-C
- Development environment: Xcode
- Higher revenue potential per user
- More standardized device ecosystem
While it’s beneficial to have knowledge of both platforms, you may want to start with one and expand your skills later. Consider factors such as your personal interest, target market, and career goals when making your decision.
3. Learning the Essential Programming Languages
Once you’ve chosen your platform, it’s time to learn the programming languages specific to that platform:
For Android Development
Java: Java has been the traditional language for Android development. It’s object-oriented, widely used, and has a large community of developers.
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, Android World!");
}
}
Kotlin: Kotlin is a more modern language that’s fully interoperable with Java. It’s concise, expressive, and is now Google’s preferred language for Android development.
fun main() {
println("Hello, Android World!")
}
For iOS Development
Swift: Swift is Apple’s modern programming language for iOS development. It’s designed to be safe, fast, and expressive.
import Foundation
print("Hello, iOS World!")
Objective-C: While less common for new projects, Objective-C is still used in many existing iOS apps and is worth learning for maintenance and legacy code.
#import <Foundation/Foundation.h>
int main(int argc, const char * argv[]) {
@autoreleasepool {
NSLog(@"Hello, iOS World!");
}
return 0;
}
Regardless of the language you choose, focus on mastering the syntax, understanding the language’s features, and practicing writing clean, efficient code.
4. Familiarizing Yourself with Development Tools
To develop mobile apps efficiently, you need to become proficient with the development tools for your chosen platform:
For Android Development
Android Studio: This is the official Integrated Development Environment (IDE) for Android app development. It provides a comprehensive set of tools for coding, debugging, and testing Android apps.
Key features to learn in Android Studio include:
- Layout Editor for designing user interfaces
- Emulator for testing apps on various virtual devices
- Gradle build system for managing dependencies and building your app
- Android Debug Bridge (ADB) for debugging and device communication
For iOS Development
Xcode: This is Apple’s IDE for developing iOS apps. It offers a suite of tools for writing code, designing interfaces, and testing your applications.
Important features to master in Xcode include:
- Interface Builder for creating user interfaces visually
- Simulator for testing your app on various iOS devices
- Instruments for performance analysis and debugging
- Swift Playgrounds for experimenting with Swift code
Both Android Studio and Xcode offer powerful features that can streamline your development process. Spend time exploring these tools and learning their shortcuts and capabilities to boost your productivity.
5. Mastering UI/UX Design Principles
Creating a successful mobile app isn’t just about writing good code; it’s also about designing an intuitive and visually appealing user interface. To create apps that users love, you need to understand the principles of UI/UX design:
- Learn the design guidelines for your chosen platform:
- Android: Material Design
- iOS: Human Interface Guidelines
- Understand user behavior and expectations on mobile devices
- Study color theory and typography for mobile interfaces
- Learn to create responsive layouts that work on different screen sizes
- Practice creating wireframes and prototypes before coding
Tools like Sketch, Adobe XD, or Figma can be helpful for creating mockups and prototypes of your app’s interface. Familiarize yourself with at least one of these tools to improve your design skills.
6. Learning App Architecture and Design Patterns
As you progress in your mobile app development journey, it’s crucial to understand app architecture and design patterns. These concepts help you structure your code in a way that’s maintainable, scalable, and easier to test.
Common Architectural Patterns
- Model-View-Controller (MVC)
- Model-View-ViewModel (MVVM)
- Model-View-Presenter (MVP)
- Clean Architecture
Important Design Patterns
- Singleton
- Observer
- Factory
- Dependency Injection
Here’s a simple example of the Singleton pattern in Swift:
class DatabaseManager {
static let shared = DatabaseManager()
private init() {}
func saveData(_ data: String) {
// Implementation
}
}
// Usage
DatabaseManager.shared.saveData("Some data")
Understanding these patterns will help you write more organized and efficient code, making your apps easier to maintain and scale as they grow in complexity.
7. Working with APIs and Third-party Libraries
Most modern mobile apps interact with web services and incorporate functionality from third-party libraries. Learning how to work with APIs (Application Programming Interfaces) and integrate external libraries is crucial for developing feature-rich applications.
Working with APIs
- Understand RESTful API concepts
- Learn to make HTTP requests (GET, POST, PUT, DELETE)
- Parse JSON and XML responses
- Implement error handling and network connectivity checks
Here’s a simple example of making an API request in Kotlin using the OkHttp library:
import okhttp3.*
import java.io.IOException
val client = OkHttpClient()
val request = Request.Builder()
.url("https://api.example.com/data")
.build()
client.newCall(request).enqueue(object : Callback {
override fun onFailure(call: Call, e: IOException) {
e.printStackTrace()
}
override fun onResponse(call: Call, response: Response) {
response.use {
if (!response.isSuccessful) throw IOException("Unexpected code $response")
for ((name, value) in response.headers) {
println("$name: $value")
}
println(response.body!!.string())
}
}
})
Using Third-party Libraries
Familiarize yourself with popular libraries in your platform’s ecosystem. For Android, this might include libraries like Retrofit for networking, Glide for image loading, or Room for database management. For iOS, you might use Alamofire for networking, SDWebImage for image handling, or Core Data for local data storage.
Learn how to integrate these libraries into your projects using dependency management tools like Gradle for Android or CocoaPods for iOS.
8. Database Management and Data Storage
Most apps need to store data locally on the device. Understanding how to work with databases and manage local storage is essential for creating robust mobile applications.
For Android
- SQLite: Android’s built-in relational database
- Room: A part of Android Jetpack that provides an abstraction layer over SQLite
- Shared Preferences: For storing key-value pairs
Here’s a simple example of using Room in Android:
@Entity
data class User(
@PrimaryKey val uid: Int,
@ColumnInfo(name = "first_name") val firstName: String?,
@ColumnInfo(name = "last_name") val lastName: String?
)
@Dao
interface UserDao {
@Query("SELECT * FROM user")
fun getAll(): List<User>
@Insert
fun insertAll(vararg users: User)
@Delete
fun delete(user: User)
}
@Database(entities = [User::class], version = 1)
abstract class AppDatabase : RoomDatabase() {
abstract fun userDao(): UserDao
}
For iOS
- Core Data: Apple’s framework for managing the model layer objects in your application
- SQLite: Can be used directly, though Core Data is generally preferred
- UserDefaults: For storing small amounts of data as key-value pairs
Here’s a basic example of using Core Data in iOS:
import CoreData
class CoreDataManager {
static let shared = CoreDataManager()
lazy var persistentContainer: NSPersistentContainer = {
let container = NSPersistentContainer(name: "MyDataModel")
container.loadPersistentStores { (storeDescription, error) in
if let error = error as NSError? {
fatalError("Unresolved error \(error), \(error.userInfo)")
}
}
return container
}()
func saveContext () {
let context = persistentContainer.viewContext
if context.hasChanges {
do {
try context.save()
} catch {
let nserror = error as NSError
fatalError("Unresolved error \(nserror), \(nserror.userInfo)")
}
}
}
}
Learning how to effectively manage data storage will help you create apps that provide a smooth user experience, even when offline.
9. Testing and Debugging Your Apps
Creating a robust and reliable app requires thorough testing and effective debugging. As you develop your mobile app, it’s crucial to implement various testing strategies and become proficient in debugging techniques.
Types of Testing
- Unit Testing: Testing individual components or functions of your app
- Integration Testing: Testing how different parts of your app work together
- UI Testing: Automating user interface interactions to ensure the app behaves correctly
- Performance Testing: Evaluating the app’s responsiveness and resource usage
For Android
Android Studio provides a suite of testing tools, including JUnit for unit tests and Espresso for UI tests. Here’s a simple example of a JUnit test in Android:
import org.junit.Test
import org.junit.Assert.*
class CalculatorTest {
@Test
fun addition_isCorrect() {
assertEquals(4, 2 + 2)
}
}
For iOS
Xcode includes XCTest framework for writing and running tests. Here’s a basic unit test example in Swift:
import XCTest
@testable import YourAppModule
class CalculatorTests: XCTestCase {
func testAddition() {
XCTAssertEqual(Calculator.add(2, 2), 4)
}
}
Debugging Techniques
- Using breakpoints to pause execution at specific points in your code
- Inspecting variable values during runtime
- Analyzing stack traces to understand the flow of execution
- Using logging to track the app’s behavior
Both Android Studio and Xcode offer powerful debugging tools. Familiarize yourself with these tools to efficiently identify and fix issues in your code.
10. Publishing Your Apps
Once you’ve developed and thoroughly tested your app, it’s time to publish it to the app store. The process differs slightly between Android and iOS:
Publishing Android Apps
- Create a developer account on Google Play Console
- Prepare your app’s store listing (title, description, screenshots, etc.)
- Upload your APK or Android App Bundle
- Set pricing and distribution options
- Submit for review
Publishing iOS Apps
- Enroll in the Apple Developer Program
- Create your app in App Store Connect
- Prepare your app’s metadata and screenshots
- Upload your build using Xcode or Application Loader
- Submit for App Review
Remember to follow the guidelines provided by Google and Apple to ensure your app meets their requirements and has the best chance of being approved.
11. Staying Updated with the Latest Trends
Mobile app development is a rapidly evolving field. To stay competitive and create cutting-edge apps, you need to keep up with the latest trends and technologies. Some areas to watch include:
- Artificial Intelligence and Machine Learning integration in mobile apps
- Augmented Reality (AR) and Virtual Reality (VR) applications
- Internet of Things (IoT) and mobile app connectivity
- Progressive Web Apps (PWAs)
- Cross-platform development frameworks like React Native or Flutter
Regularly read tech blogs, attend conferences, and participate in online communities to stay informed about new developments in the mobile app world.
12. Building Your Portfolio
As you learn and grow as a mobile app developer, it’s important to build a portfolio that showcases your skills and projects. Here are some tips for creating an impressive portfolio:
- Develop a variety of apps that demonstrate different skills and features
- Include both personal projects and any professional work you’ve done
- Provide detailed descriptions of each project, including the technologies used and your role
- If possible, include links to live apps on the app stores
- Showcase your code on platforms like GitHub to demonstrate your coding practices
- Keep your portfolio updated with your latest and best work
A strong portfolio can be a powerful tool in job interviews or when seeking freelance opportunities in mobile app development.
13. Joining the Developer Community
Becoming part of the developer community can greatly accelerate your learning and provide valuable networking opportunities. Here are some ways to get involved:
- Join online forums and communities (e.g., Stack Overflow, Reddit’s r/androiddev or r/iOSProgramming)
- Participate in local meetups or tech events
- Contribute to open-source projects
- Attend hackathons to challenge yourself and meet other developers
- Share your knowledge through blog posts or tutorials
Engaging with the community not only helps you learn from others but also allows you to give back and establish yourself as a knowledgeable developer.
14. Continuous Learning and Improvement
The journey of becoming a proficient mobile app developer doesn’t end with publishing your first app. It’s a continuous process of learning and improvement. Here are some strategies for ongoing growth:
- Set personal coding challenges to push your skills further
- Regularly review and refactor your old code to improve its quality
- Explore advanced topics in mobile development, such as:
- Performance optimization
- Security best practices
- Accessibility features
- Localization and internationalization
- Learn about software development methodologies (e.g., Agile, Scrum) to improve your project management skills
- Consider learning cross-platform development to broaden your skill set
Remember, the best developers are those who are always eager to learn and adapt to new technologies and methodologies.
15. Conclusion
Learning mobile app development for Android and iOS is an exciting journey that opens up a world of opportunities. By following this comprehensive guide, you’ll be well on your way to becoming a skilled mobile app developer. Remember that the key to success is consistent practice, continuous learning, and a passion for creating great user experiences.
Start with the basics, choose your platform, master the essential programming languages and tools, and gradually build up your skills in areas like UI/UX design, app architecture, and working with APIs. Don’t forget to test your apps thoroughly, stay updated with the latest trends, and engage with the developer community.
As you progress, build a strong portfolio that showcases your skills and creativity. Keep pushing yourself to learn new technologies and improve your existing knowledge. With dedication and perseverance, you’ll be creating amazing mobile apps in no time.
Remember, platforms like AlgoCademy can be valuable resources in your learning journey, offering interactive tutorials and tools to help you develop your programming skills and prepare for technical interviews. Embrace the challenges, enjoy the process, and happy coding!