How to Learn HTML and CSS for Web Design: A Comprehensive Guide
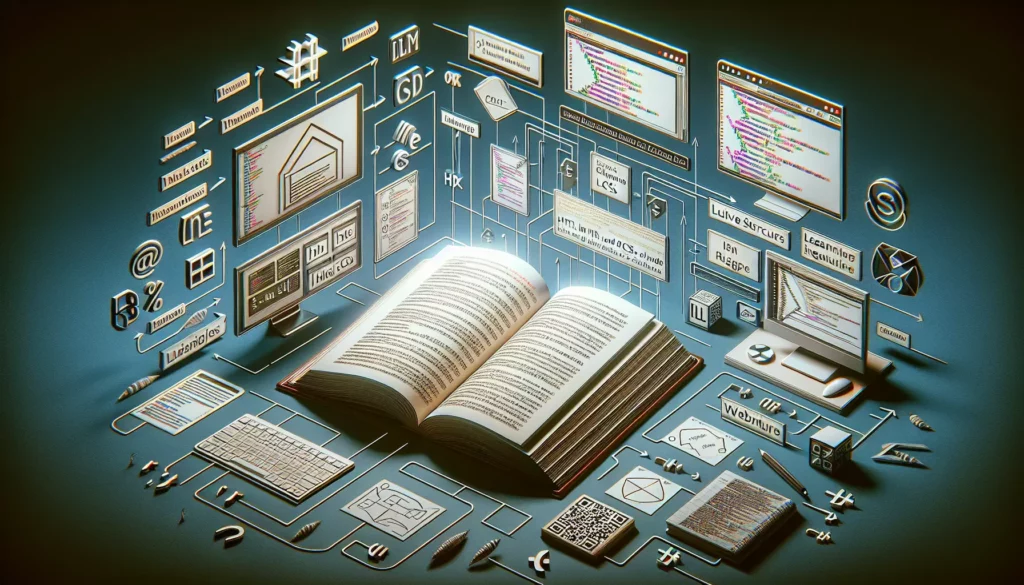
In today’s digital age, web design skills are more valuable than ever. Whether you’re looking to launch a career in web development, enhance your current skill set, or simply create your own personal website, learning HTML and CSS is the perfect place to start. These two fundamental languages form the backbone of web design and are essential for anyone looking to create visually appealing and functional websites. In this comprehensive guide, we’ll walk you through the process of learning HTML and CSS, providing you with the knowledge and resources you need to kickstart your web design journey.
Understanding the Basics: What are HTML and CSS?
Before diving into the learning process, it’s crucial to understand what HTML and CSS are and how they work together to create web pages.
HTML: The Structure of the Web
HTML stands for Hypertext Markup Language. It’s the standard markup language used to create the structure and content of web pages. HTML uses a system of tags to define different elements on a page, such as headings, paragraphs, images, and links.
Here’s a simple example of HTML code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My First Web Page</title>
</head>
<body>
<h1>Welcome to My Website</h1>
<p>This is a paragraph of text.</p>
</body>
</html>
CSS: The Style of the Web
CSS, or Cascading Style Sheets, is a stylesheet language used to describe the presentation of a document written in HTML. CSS defines how HTML elements should be displayed on screen, paper, or in other media. It controls the layout, colors, fonts, and overall visual appearance of web pages.
Here’s a basic example of CSS:
body {
font-family: Arial, sans-serif;
background-color: #f0f0f0;
}
h1 {
color: #333333;
text-align: center;
}
p {
font-size: 16px;
line-height: 1.5;
}
Getting Started with HTML and CSS
Now that you understand the basics, let’s explore how to start learning HTML and CSS effectively.
1. Set Up Your Development Environment
To begin coding, you’ll need a text editor and a web browser. Here are some popular options:
- Text Editors: Visual Studio Code, Sublime Text, Atom
- Web Browsers: Google Chrome, Mozilla Firefox, Microsoft Edge
Visual Studio Code is a fantastic choice for beginners due to its user-friendly interface and extensive plugin ecosystem. It also includes features like syntax highlighting and auto-completion, which can be incredibly helpful when learning.
2. Learn the Basics of HTML
Start by learning the fundamental HTML tags and structure. Focus on understanding:
- Document structure (<!DOCTYPE>, <html>, <head>, <body>)
- Text elements (<h1> to <h6>, <p>, <span>)
- Links (<a>)
- Images (<img>)
- Lists (<ul>, <ol>, <li>)
- Tables (<table>, <tr>, <td>)
- Forms (<form>, <input>, <button>)
3. Dive into CSS Basics
Once you’re comfortable with HTML, start exploring CSS. Key concepts to focus on include:
- Selectors (element, class, ID)
- Properties and values
- The box model (margin, border, padding, content)
- Colors and backgrounds
- Typography
- Layout (display, position, float)
4. Practice, Practice, Practice
The best way to learn HTML and CSS is through hands-on practice. Start by creating simple web pages and gradually increase the complexity as you become more comfortable. Try recreating existing websites or design mockups to challenge yourself.
Learning Resources for HTML and CSS
There are numerous resources available to help you learn HTML and CSS. Here are some of the best options:
1. Online Courses and Tutorials
- freeCodeCamp: Offers a comprehensive curriculum covering HTML, CSS, and more, completely free.
- Codecademy: Provides interactive courses on HTML and CSS with hands-on coding exercises.
- MDN Web Docs: Mozilla’s excellent documentation for web technologies, including detailed guides on HTML and CSS.
- W3Schools: Offers tutorials and references for HTML, CSS, and other web technologies.
2. Books
- “HTML and CSS: Design and Build Websites” by Jon Duckett
- “CSS: The Definitive Guide” by Eric A. Meyer and Estelle Weyl
- “Learning Web Design” by Jennifer Niederst Robbins
3. YouTube Channels
- Traversy Media
- The Net Ninja
- freeCodeCamp
- Kevin Powell (CSS-focused)
4. Coding Challenges and Projects
- Frontend Mentor: Provides real-world projects to improve your frontend skills.
- CSS Battle: Offers fun CSS coding challenges to test and improve your skills.
- 100 Days of CSS: A creative project that challenges you to create something new with CSS every day.
Advanced Topics in HTML and CSS
As you progress in your learning journey, you’ll want to explore more advanced topics in HTML and CSS. Here are some areas to focus on:
1. Responsive Web Design
Learn how to create websites that look great on all devices, from mobile phones to desktop computers. Key concepts include:
- Media queries
- Flexible grids and layouts
- Responsive images
- Mobile-first design approach
2. CSS Flexbox and Grid
Master these powerful layout systems to create complex and flexible page structures:
- Flexbox for one-dimensional layouts
- CSS Grid for two-dimensional layouts
- Combining Flexbox and Grid for optimal layouts
3. CSS Preprocessors
Explore CSS preprocessors like Sass or Less to write more efficient and maintainable CSS:
- Variables
- Nesting
- Mixins
- Functions
4. CSS Animations and Transitions
Learn how to create engaging user experiences with CSS animations:
- Keyframe animations
- Transitions
- Transform properties
5. Semantic HTML5
Understand the importance of semantic markup and how to use HTML5 structural elements:
- <header>, <nav>, <main>, <article>, <section>, <aside>, <footer>
- Accessibility considerations
- SEO benefits of semantic HTML
Best Practices for Learning HTML and CSS
To make the most of your learning experience, consider the following best practices:
1. Code Every Day
Consistency is key when learning any new skill. Try to code for at least an hour every day, even if it’s just working on small projects or exercises.
2. Build Real Projects
Apply your knowledge by building real websites or web applications. This hands-on experience is invaluable for reinforcing your skills and understanding how different concepts work together.
3. Read and Analyze Other People’s Code
Study well-designed websites and examine their source code. This can give you insights into best practices and new techniques you might not have discovered on your own.
4. Stay Updated
Web technologies are constantly evolving. Follow industry blogs, attend webinars, and participate in online communities to stay up-to-date with the latest trends and best practices in HTML and CSS.
5. Seek Feedback
Share your work with others and ask for constructive criticism. Platforms like GitHub, CodePen, or web development forums can be great places to get feedback from more experienced developers.
6. Embrace the Developer Tools
Learn to use your browser’s developer tools. These can be incredibly helpful for debugging, testing different styles, and understanding how websites are built.
Integrating HTML and CSS Learning with AlgoCademy
While AlgoCademy primarily focuses on algorithmic thinking and problem-solving for technical interviews, the skills you develop learning HTML and CSS can complement your coding education in several ways:
1. Visualizing Data Structures
As you progress in your algorithmic studies with AlgoCademy, you can use your HTML and CSS skills to create visual representations of data structures like arrays, linked lists, trees, and graphs. This can help reinforce your understanding of these concepts.
2. Building Interactive Coding Challenges
Combine your HTML, CSS, and JavaScript skills to create interactive coding challenges similar to those you might encounter on AlgoCademy. This can be an excellent way to practice both your web development and problem-solving skills.
3. Creating a Portfolio
Use your HTML and CSS skills to build a personal portfolio website showcasing the projects and coding challenges you’ve completed on AlgoCademy. This can be a valuable asset when applying for jobs or internships.
4. Understanding Full-Stack Development
While AlgoCademy focuses on algorithmic thinking, learning HTML and CSS gives you insight into the frontend aspect of web development. This broader understanding can be beneficial when working on full-stack projects or preparing for technical interviews that cover a wide range of topics.
Conclusion
Learning HTML and CSS is an exciting journey that opens up a world of possibilities in web design and development. By following this guide and leveraging the resources provided, you’ll be well on your way to creating beautiful, functional websites.
Remember, the key to mastering HTML and CSS is consistent practice and a willingness to experiment. Don’t be afraid to try new things, make mistakes, and learn from them. As you progress, you’ll find that your skills improve rapidly, and you’ll be able to bring your creative visions to life on the web.
While platforms like AlgoCademy focus on algorithmic thinking and problem-solving for technical interviews, the skills you develop in HTML and CSS can complement this knowledge, giving you a well-rounded understanding of web technologies. This combination of frontend skills and algorithmic thinking can make you a more versatile and valuable developer in the long run.
So, roll up your sleeves, fire up your text editor, and start coding! The world of web design is waiting for you to make your mark. Happy coding!