How to Learn from Your Mistakes and Improve with Every Failed Solution
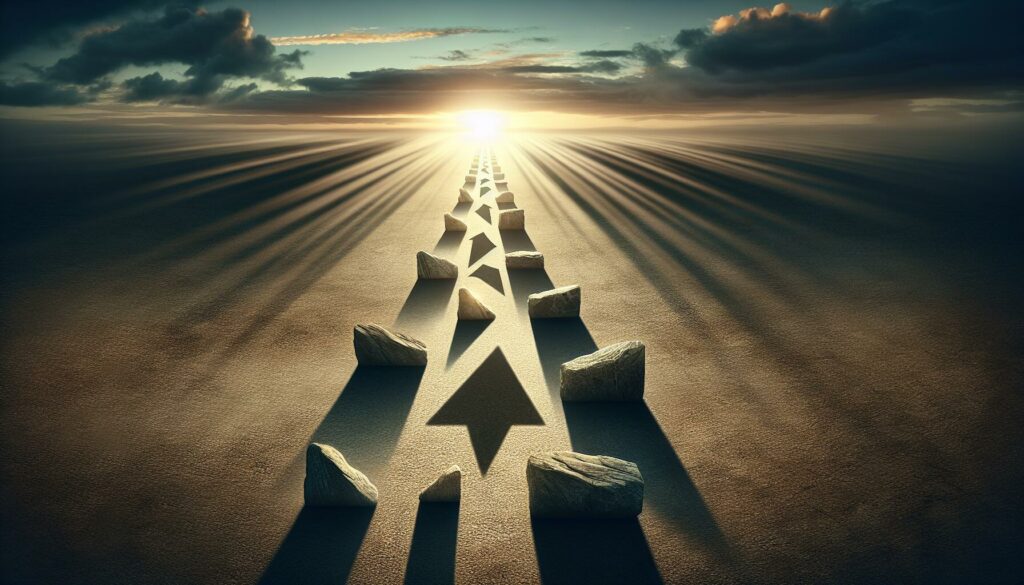
In the world of coding and software development, mistakes are not just inevitable—they’re a crucial part of the learning process. As you embark on your journey to become a proficient programmer, whether you’re a beginner or preparing for technical interviews at major tech companies, understanding how to learn from your mistakes and grow from failed solutions is an invaluable skill. This article will guide you through the process of turning your coding errors into stepping stones for success.
The Importance of Embracing Mistakes in Coding
Before we dive into the strategies for learning from mistakes, it’s essential to understand why errors are so important in the coding process:
- Mistakes reveal gaps in knowledge: When you make an error, it often highlights areas where your understanding is incomplete or incorrect.
- Errors enhance problem-solving skills: Debugging and fixing mistakes improve your analytical abilities and teach you to approach problems from different angles.
- Failures build resilience: Learning to cope with and overcome coding challenges prepares you for the realities of professional software development.
- Mistakes lead to deeper understanding: When you correct an error, you often gain a more profound comprehension of the underlying concepts.
Strategies for Learning from Coding Mistakes
1. Analyze the Error Message
When you encounter an error, the first step is to carefully read and understand the error message. Error messages are not just roadblocks; they’re valuable pieces of information that can guide you to the solution.
Here’s how to approach error messages effectively:
- Read the entire message carefully, don’t just glance at it.
- Identify the type of error (syntax error, runtime error, logical error, etc.).
- Note the line number or location where the error occurred.
- Look for any specific details about what might be causing the error.
For example, consider this Python error message:
Traceback (most recent call last):
File "example.py", line 5, in <module>
result = 10 / 0
ZeroDivisionError: division by zero
This error message tells us:
- The error occurred in the file “example.py” on line 5
- The specific operation that caused the error (division by zero)
- The type of error (ZeroDivisionError)
2. Reproduce the Error
Once you understand the error message, try to reproduce the error consistently. This step is crucial for several reasons:
- It confirms that you’ve identified the correct issue
- It allows you to test potential solutions systematically
- It helps in creating a minimal example of the problem, which is useful if you need to seek help
To reproduce the error effectively:
- Isolate the problematic code
- Create a simple test case that triggers the error
- Document the steps to reproduce the error
3. Understand the Root Cause
After reproducing the error, dive deep to understand why it’s occurring. This often involves:
- Reviewing the relevant code carefully
- Checking your assumptions about how the code should work
- Researching the specific error type and common causes
- Using debugging tools to step through the code
Let’s say you’re working on a sorting algorithm and encountering unexpected results. You might use a debugger to step through the code, examining variable values at each step to identify where the logic deviates from your expectations.
4. Implement a Solution
Once you understand the root cause, it’s time to implement a solution. Remember these points:
- Start with the simplest possible fix
- Test your solution thoroughly
- Ensure your fix doesn’t introduce new problems
- Consider alternative solutions and their trade-offs
For instance, if you’re fixing a bug in a recursive function that’s causing a stack overflow, you might consider:
- Adding a base case to ensure termination
- Rewriting the function iteratively
- Optimizing the recursion to reduce stack usage
5. Reflect on the Learning Experience
After successfully resolving the issue, take time to reflect on what you’ve learned. Ask yourself:
- What was the fundamental concept I misunderstood?
- How can I prevent similar errors in the future?
- What new skills or knowledge did I gain from this experience?
- How can I apply this learning to other areas of my coding?
This reflection process is crucial for cementing your learning and preventing similar mistakes in the future.
Advanced Techniques for Learning from Mistakes
1. Keep a Coding Journal
Maintaining a coding journal can be an excellent way to track your progress and learn from your mistakes. In your journal, you can:
- Document challenging problems you’ve encountered
- Record solutions you’ve implemented and their outcomes
- Note any patterns in the types of errors you frequently make
- Reflect on your problem-solving process and how it evolves over time
A coding journal entry might look something like this:
Date: 2023-05-15
Problem: Implementing QuickSort algorithm
Error encountered: Infinite recursion causing stack overflow
Root cause: Incorrect partitioning logic leading to no progress in divide step
Solution: Fixed partitioning function to ensure proper element distribution
Lessons learned:
- Always consider edge cases (e.g., all elements equal)
- Importance of choosing a good pivot for efficient QuickSort
- How to use recursion safely to avoid stack overflow
2. Participate in Code Reviews
Code reviews, whether giving or receiving them, are excellent opportunities to learn from mistakes. When participating in code reviews:
- Pay attention to common patterns of errors or inefficiencies
- Ask questions to understand the reasoning behind certain implementations
- Be open to constructive criticism and alternative approaches
- Learn from others’ mistakes as well as your own
For example, during a code review, you might notice that a colleague consistently forgets to free allocated memory in C programs. This observation can remind you to be vigilant about memory management in your own code.
3. Analyze Open Source Projects
Studying open source projects can provide valuable insights into how experienced developers handle errors and improve their code. When analyzing open source projects:
- Look at the project’s issue tracker to see common problems and their solutions
- Examine pull requests to understand how code improvements are proposed and implemented
- Study the project’s error handling and logging mechanisms
- Consider contributing to fix bugs or improve error handling
For instance, by examining the Node.js GitHub repository, you might learn about best practices for asynchronous error handling in JavaScript:
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
const data = await response.json();
return data;
} catch (error) {
console.error('Failed to fetch data:', error);
// Optionally, rethrow or return a default value
}
}
4. Practice Deliberate Error Introduction
While it might seem counterintuitive, deliberately introducing errors into your code can be a powerful learning tool. This technique, sometimes called “error seeding” or “fault injection,” can help you:
- Understand how different types of errors manifest
- Improve your debugging skills
- Develop more robust error handling mechanisms
- Prepare for unexpected scenarios in your code
Here’s an example of how you might practice this technique:
- Write a simple function, such as a binary search algorithm
- Introduce various errors (off-by-one errors, incorrect comparisons, etc.)
- Try to identify and fix these errors using debugging tools and techniques
- Reflect on how each type of error affects the algorithm’s behavior
5. Engage in Coding Challenges and Competitions
Participating in coding challenges and competitions exposes you to a wide range of problems and pushes you to write code under pressure. This environment is ripe for making mistakes and learning from them. When engaging in these activities:
- Attempt to solve problems even if you’re unsure of the solution
- Review your submissions, especially for problems you couldn’t solve
- Study other participants’ solutions to learn alternative approaches
- Reflect on the mistakes you made and how you can improve
Platforms like LeetCode, HackerRank, and Codeforces offer a wealth of coding challenges that can help you hone your skills and learn from your mistakes.
Applying Your Learning to Technical Interviews
As you prepare for technical interviews, especially for major tech companies, the ability to learn from your mistakes becomes even more crucial. Here’s how you can apply these learning strategies to your interview preparation:
1. Mock Interviews and Post-Interview Analysis
Conduct mock interviews with peers or use online platforms that offer this service. After each mock interview:
- Review your performance critically
- Identify areas where you struggled or made mistakes
- Research and implement better solutions for problems you couldn’t solve
- Practice explaining your thought process, including how you recognize and correct mistakes
2. Time-Boxed Problem Solving
Practice solving coding problems within strict time limits, similar to real interview conditions. This approach will:
- Help you identify which types of errors you’re prone to making under pressure
- Improve your ability to quickly recognize and correct mistakes
- Enhance your time management skills during problem-solving
3. Develop a Mistake Mitigation Strategy
Based on your experiences and the mistakes you’ve learned from, develop a personal strategy for minimizing errors during interviews. This might include:
- A checklist of common pitfalls to avoid (e.g., off-by-one errors, failing to handle edge cases)
- A structured approach to problem-solving that includes error checking at each step
- Techniques for gracefully handling and recovering from mistakes during the interview
4. Build a Personal Error Database
Create a database of common errors you’ve encountered in your preparation, along with their solutions. This resource can serve as a quick reference for last-minute review before interviews. Your database might include:
- Syntax errors specific to languages commonly used in interviews
- Logical errors in common algorithms and data structures
- Conceptual misunderstandings in key computer science topics
Conclusion: Embracing the Learning Process
Learning from your mistakes and improving with every failed solution is a fundamental skill for any programmer, whether you’re just starting out or preparing for technical interviews at top tech companies. By embracing errors as learning opportunities, systematically analyzing and reflecting on your mistakes, and continuously applying the lessons learned, you can accelerate your growth as a developer.
Remember, every experienced programmer has faced countless errors and setbacks. What sets successful developers apart is not an absence of mistakes, but rather their ability to learn, adapt, and grow from these experiences. As you continue your coding journey, maintain a growth mindset, stay curious about the root causes of errors, and never stop learning from your mistakes.
By following the strategies outlined in this article and consistently applying them to your coding practice, you’ll not only become a more skilled and resilient programmer but also be better prepared to tackle the challenges of technical interviews and real-world software development. Embrace your mistakes, learn from them, and watch as they transform from obstacles into opportunities for growth and improvement.