How to Learn Data Structures and Algorithms for Interviews: A Comprehensive Guide
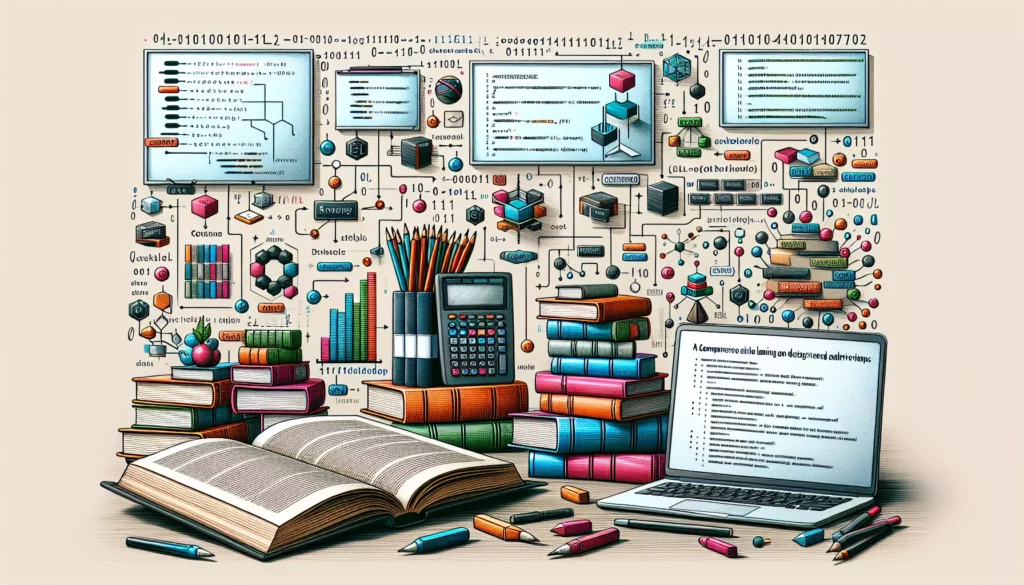
In the competitive world of tech interviews, particularly for positions at major companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), mastering data structures and algorithms is crucial. This comprehensive guide will walk you through the process of learning these essential concepts, helping you prepare effectively for technical interviews and boost your overall programming skills.
Table of Contents
- Understanding the Importance of Data Structures and Algorithms
- Setting a Strong Foundation
- Key Data Structures to Master
- Essential Algorithms to Learn
- Problem-Solving Strategies
- Practice Resources and Coding Platforms
- Interview-Specific Preparation
- Time Management and Study Techniques
- Common Mistakes to Avoid
- Beyond Interviews: Long-term Benefits
1. Understanding the Importance of Data Structures and Algorithms
Before diving into the learning process, it’s crucial to understand why data structures and algorithms are so important in the tech industry, especially for interviews:
- Problem-Solving Skills: They form the foundation of efficient problem-solving in computer science.
- Code Optimization: Knowledge of data structures and algorithms helps in writing optimized and efficient code.
- Industry Standard: Most tech companies, especially FAANG, consider this knowledge as a baseline for software engineering roles.
- Scalability: Understanding these concepts is crucial for building scalable software systems.
- Analytical Thinking: They enhance your ability to think analytically and approach problems systematically.
2. Setting a Strong Foundation
Before jumping into complex algorithms, ensure you have a solid grasp of the basics:
2.1 Programming Language Proficiency
Choose a programming language and become proficient in it. Popular choices include:
- Python: Known for its simplicity and readability
- Java: Widely used in enterprise environments
- C++: Preferred for its performance in competitive programming
- JavaScript: Essential for web development roles
2.2 Basic Computer Science Concepts
Ensure you understand:
- Time and space complexity (Big O notation)
- Memory management
- Recursion
- Object-Oriented Programming principles
2.3 Mathematics for Computer Science
Brush up on:
- Discrete mathematics
- Basic probability and statistics
- Logic and proofs
3. Key Data Structures to Master
Focus on understanding and implementing these fundamental data structures:
3.1 Arrays and Strings
Start with the basics:
- One-dimensional and multi-dimensional arrays
- Dynamic arrays (e.g., ArrayList in Java, List in Python)
- String manipulation techniques
3.2 Linked Lists
Understand:
- Singly linked lists
- Doubly linked lists
- Circular linked lists
3.3 Stacks and Queues
Learn about:
- LIFO (Last In, First Out) principle for stacks
- FIFO (First In, First Out) principle for queues
- Implementations using arrays and linked lists
3.4 Trees
Master various tree structures:
- Binary trees
- Binary search trees (BST)
- Balanced trees (AVL, Red-Black)
- Heap (min-heap and max-heap)
- Trie (prefix tree)
3.5 Graphs
Understand different types and representations:
- Directed and undirected graphs
- Weighted graphs
- Adjacency matrix and adjacency list representations
3.6 Hash Tables
Learn about:
- Hash functions
- Collision resolution techniques
- Implementation of hash tables
4. Essential Algorithms to Learn
After mastering data structures, focus on these key algorithmic concepts:
4.1 Sorting Algorithms
Understand and implement:
- Bubble Sort
- Selection Sort
- Insertion Sort
- Merge Sort
- Quick Sort
- Heap Sort
4.2 Searching Algorithms
Master these searching techniques:
- Linear Search
- Binary Search
- Depth-First Search (DFS)
- Breadth-First Search (BFS)
4.3 Graph Algorithms
Learn these important graph algorithms:
- Dijkstra’s Algorithm
- Bellman-Ford Algorithm
- Floyd-Warshall Algorithm
- Kruskal’s and Prim’s Algorithms for Minimum Spanning Trees
- Topological Sorting
4.4 Dynamic Programming
Understand the concept and practice problems like:
- Fibonacci sequence
- Longest Common Subsequence
- Knapsack problem
- Matrix Chain Multiplication
4.5 Greedy Algorithms
Learn about greedy approach and its applications:
- Activity Selection
- Huffman Coding
- Fractional Knapsack
5. Problem-Solving Strategies
Develop effective problem-solving strategies to tackle algorithmic challenges:
5.1 Understand the Problem
- Read the problem statement carefully
- Identify the input and expected output
- Look for any constraints or special conditions
5.2 Plan Your Approach
- Break down the problem into smaller sub-problems
- Consider multiple approaches before settling on one
- Think about edge cases and potential pitfalls
5.3 Implement the Solution
- Start with a brute-force approach if needed
- Optimize your solution step by step
- Write clean, readable code
5.4 Test and Debug
- Test your solution with various inputs, including edge cases
- Use debugging techniques to identify and fix errors
- Analyze the time and space complexity of your solution
6. Practice Resources and Coding Platforms
Utilize these resources to enhance your skills:
6.1 Online Platforms
- LeetCode: Offers a wide range of problems with difficulty levels
- HackerRank: Provides coding challenges and competitions
- CodeSignal: Features interview practice and coding challenges
- AlgoExpert: Offers curated list of common interview questions
6.2 Books
- “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “Algorithm Design Manual” by Steven Skiena
6.3 Online Courses
- Coursera: “Algorithms Specialization” by Stanford University
- edX: “Algorithm Design and Analysis” by PennX
- Udacity: “Data Structures and Algorithms Nanodegree”
6.4 Interactive Learning Platforms
- AlgoCademy: Offers AI-powered assistance and step-by-step guidance
- InterviewBit: Provides a structured learning path for interview preparation
7. Interview-Specific Preparation
As you approach your interviews, focus on these aspects:
7.1 Mock Interviews
- Practice with peers or use platforms like Pramp for mock interviews
- Get comfortable explaining your thought process out loud
- Learn to handle pressure and time constraints
7.2 Company-Specific Preparation
- Research the company’s interview process and typical questions
- Focus on areas that are particularly important to the company (e.g., scalability for Google)
- Understand the company’s products and technologies
7.3 Behavioral Questions
- Prepare stories about your past projects and experiences
- Practice answering questions about teamwork, conflict resolution, and leadership
- Be ready to discuss your passion for technology and learning
7.4 System Design (for Senior Roles)
- Study distributed systems concepts
- Practice designing scalable architectures
- Understand trade-offs in system design decisions
8. Time Management and Study Techniques
Effective time management is crucial for successful interview preparation:
8.1 Create a Study Schedule
- Set realistic goals and deadlines
- Allocate specific time slots for different topics
- Include regular review sessions in your schedule
8.2 Use Spaced Repetition
- Review concepts at increasing intervals
- Use flashcards or apps like Anki for efficient review
- Focus more on areas where you struggle
8.3 Active Learning Techniques
- Implement algorithms from scratch
- Explain concepts to others (rubber duck debugging)
- Participate in coding competitions or hackathons
8.4 Take Care of Your Well-being
- Maintain a healthy sleep schedule
- Include physical exercise in your routine
- Practice stress-management techniques like meditation
9. Common Mistakes to Avoid
Be aware of these common pitfalls in interview preparation:
9.1 Overemphasis on Memorization
Don’t just memorize solutions. Focus on understanding the underlying principles and problem-solving approaches.
9.2 Neglecting Time Complexity Analysis
Always analyze and optimize the time and space complexity of your solutions.
9.3 Ignoring the Basics
Don’t overlook fundamental concepts while chasing complex algorithms.
9.4 Lack of Real-world Context
Try to understand how these concepts apply to real-world software development scenarios.
9.5 Not Practicing Communication
Practice explaining your thought process clearly. Communication is as important as coding in interviews.
10. Beyond Interviews: Long-term Benefits
Remember that learning data structures and algorithms has benefits beyond just acing interviews:
10.1 Improved Problem-Solving Skills
These skills will help you approach complex problems in your day-to-day work more effectively.
10.2 Better Code Quality
Understanding algorithms leads to writing more efficient and maintainable code.
10.3 Foundation for Advanced Topics
This knowledge forms the basis for learning advanced topics like machine learning and artificial intelligence.
10.4 Career Growth
Strong algorithmic skills can open doors to more challenging and rewarding roles in your career.
Conclusion
Learning data structures and algorithms for interviews is a challenging but rewarding journey. It requires dedication, consistent practice, and a strategic approach. By following this comprehensive guide, you’ll not only prepare yourself for technical interviews at top companies but also develop skills that will benefit your entire programming career.
Remember, the key to success lies in consistent practice, understanding core concepts deeply, and applying your knowledge to solve diverse problems. Embrace the learning process, stay curious, and don’t be discouraged by initial difficulties. With persistence and the right approach, you’ll be well-equipped to tackle even the most challenging technical interviews.
Happy coding and best of luck with your interview preparation!