How to Learn Data Structures and Algorithms for Interviews: A Comprehensive Guide
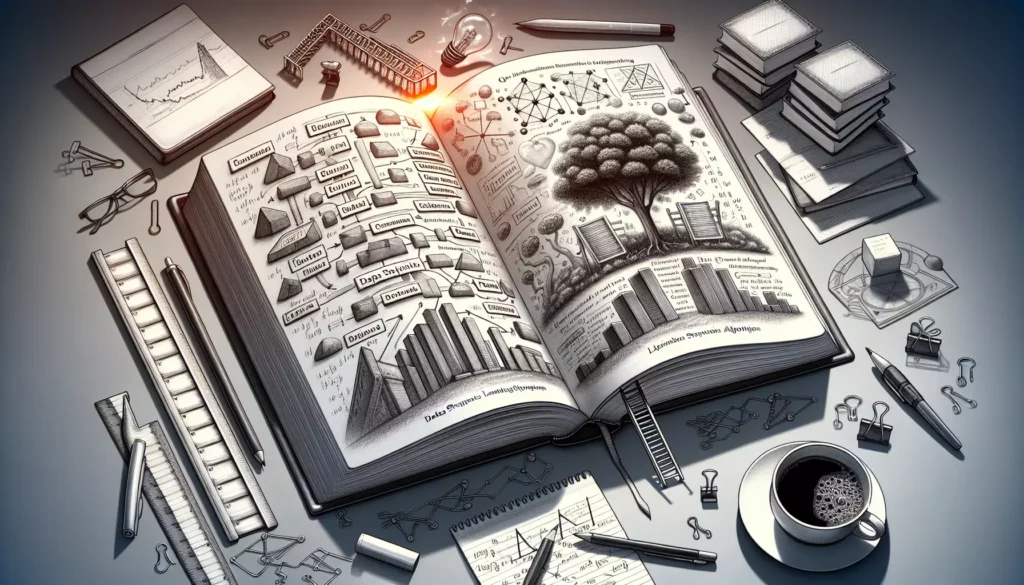
In the competitive world of tech interviews, particularly for positions at major companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), mastering data structures and algorithms (DSA) is crucial. This comprehensive guide will walk you through the process of learning DSA effectively, helping you prepare for technical interviews and boost your problem-solving skills.
Table of Contents
- Understanding the Importance of DSA
- Getting Started with DSA
- Essential Data Structures to Learn
- Key Algorithms to Master
- Problem-Solving Strategies
- Practice Resources and Platforms
- Interview-Specific Preparation
- Common Mistakes to Avoid
- Advanced Topics for Further Study
- Maintaining and Improving Your Skills
1. Understanding the Importance of DSA
Before diving into the learning process, it’s crucial to understand why Data Structures and Algorithms are so important in the tech industry, especially for interviews:
- Problem-Solving Skills: DSA forms the foundation of efficient problem-solving in computer science.
- Code Optimization: Knowledge of DSA helps in writing optimized and efficient code.
- Industry Standard: Major tech companies use DSA questions to assess candidates’ analytical and coding abilities.
- Versatility: DSA concepts are applicable across various programming languages and domains.
- Career Growth: Proficiency in DSA can lead to better job opportunities and career advancement.
2. Getting Started with DSA
Starting your journey into DSA can seem daunting, but with the right approach, it can be an exciting learning experience:
Choose a Programming Language
Select a language you’re comfortable with or one that’s commonly used in interviews. Popular choices include:
- Python (beginner-friendly and widely used)
- Java (common in many tech companies)
- C++ (efficient for competitive programming)
- JavaScript (useful for web development roles)
Brush Up on Basic Programming Concepts
Ensure you have a solid grasp of fundamental programming concepts:
- Variables and data types
- Control structures (if-else, loops)
- Functions and methods
- Object-Oriented Programming basics
Start with Simple Data Structures
Begin with basic data structures to build a strong foundation:
- Arrays
- Strings
- Linked Lists
- Stacks and Queues
Learn Time and Space Complexity
Understanding Big O notation is crucial for analyzing algorithm efficiency:
- Learn to calculate time complexity
- Understand space complexity
- Practice analyzing different algorithms
3. Essential Data Structures to Learn
After mastering the basics, focus on these essential data structures:
Arrays and Strings
Fundamental structures used in many algorithms:
- 1D and 2D arrays
- Dynamic arrays (e.g., ArrayList in Java, List in Python)
- String manipulation techniques
Linked Lists
Important for understanding memory management and pointer manipulation:
- Singly linked lists
- Doubly linked lists
- Circular linked lists
Stacks and Queues
Crucial for understanding LIFO and FIFO operations:
- Implementation using arrays and linked lists
- Applications in problem-solving
Trees
Essential for hierarchical data representation:
- Binary trees
- Binary search trees (BST)
- Balanced trees (AVL, Red-Black)
- Tries
Graphs
Important for representing complex relationships:
- Graph representation (adjacency list, adjacency matrix)
- Graph traversals (BFS, DFS)
- Shortest path algorithms
Hash Tables
Crucial for efficient data retrieval:
- Hash functions
- Collision resolution techniques
- Applications in problem-solving
Heaps
Important for priority queue implementations:
- Min heaps and max heaps
- Heap operations
4. Key Algorithms to Master
Once you’re comfortable with data structures, focus on these essential algorithms:
Sorting Algorithms
- Bubble Sort
- Selection Sort
- Insertion Sort
- Merge Sort
- Quick Sort
- Heap Sort
Searching Algorithms
- Linear Search
- Binary Search
- Depth-First Search (DFS)
- Breadth-First Search (BFS)
Graph Algorithms
- Dijkstra’s Algorithm
- Bellman-Ford Algorithm
- Floyd-Warshall Algorithm
- Kruskal’s Algorithm
- Prim’s Algorithm
Dynamic Programming
A powerful technique for solving optimization problems:
- Memoization
- Tabulation
- Common DP problems (e.g., Fibonacci, Knapsack)
Greedy Algorithms
Useful for optimization problems:
- Activity Selection
- Huffman Coding
- Fractional Knapsack
String Algorithms
- KMP Algorithm
- Rabin-Karp Algorithm
- Z Algorithm
5. Problem-Solving Strategies
Developing effective problem-solving strategies is crucial for tackling DSA questions in interviews:
Understand the Problem
- Read the problem statement carefully
- Identify the input and expected output
- Look for any constraints or special cases
Plan Your Approach
- Break down the problem into smaller steps
- Consider multiple approaches
- Think about the appropriate data structures and algorithms
Implement the Solution
- Start with a basic implementation
- Focus on correctness before optimization
- Use meaningful variable names and add comments
Test and Debug
- Test with sample inputs
- Consider edge cases and boundary conditions
- Use debugging techniques to fix issues
Optimize and Refine
- Analyze the time and space complexity
- Look for opportunities to optimize
- Consider trade-offs between time and space
6. Practice Resources and Platforms
Regular practice is key to mastering DSA. Here are some excellent resources and platforms to help you practice:
Online Coding Platforms
- LeetCode: Offers a wide range of problems with difficulty levels and company tags
- HackerRank: Provides structured learning paths and coding challenges
- CodeForces: Great for competitive programming and advanced problem-solving
- AlgoExpert: Curated list of common interview questions with video explanations
Books
- “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “Algorithms” by Robert Sedgewick and Kevin Wayne
Online Courses
- Coursera’s “Algorithms Specialization” by Stanford University
- edX’s “Algorithm Design and Analysis” by PennX
- Udacity’s “Data Structures and Algorithms Nanodegree”
YouTube Channels
- MIT OpenCourseWare
- mycodeschool
- Back To Back SWE
Interactive Coding Platforms
- AlgoCademy: Offers interactive coding tutorials and AI-powered assistance
- CodeSignal: Provides coding assessments and interview practice
7. Interview-Specific Preparation
As you approach your interview date, focus on these interview-specific preparation strategies:
Mock Interviews
- Practice with a friend or use platforms like Pramp for mock interviews
- Get comfortable explaining your thought process out loud
- Learn to handle hints and feedback during the interview
Time Management
- Practice solving problems within time constraints
- Learn to allocate time for understanding, coding, and testing
- Develop strategies for when you’re stuck on a problem
Company-Specific Preparation
- Research the company’s interview process and common questions
- Focus on problems tagged for your target company on platforms like LeetCode
- Understand the company’s tech stack and prepare relevant examples
Behavioral Preparation
- Prepare answers to common behavioral questions
- Have examples ready to demonstrate your problem-solving skills
- Practice explaining technical concepts in a clear, concise manner
System Design (for Senior Roles)
- Study basic system design principles
- Practice designing scalable systems
- Understand trade-offs in system design decisions
8. Common Mistakes to Avoid
Be aware of these common pitfalls when learning DSA and preparing for interviews:
Neglecting the Basics
- Don’t skip fundamental concepts in favor of advanced topics
- Ensure a strong understanding of core data structures and algorithms
Memorizing Solutions
- Focus on understanding patterns and approaches rather than memorizing specific solutions
- Learn to apply concepts to new problems
Ignoring Time and Space Complexity
- Always analyze and optimize your solutions
- Be prepared to discuss trade-offs between different approaches
Not Practicing Communication
- Practice explaining your thought process clearly
- Learn to discuss technical concepts in a non-technical manner
Overlooking Edge Cases
- Always consider boundary conditions and special cases
- Test your code with various inputs, including edge cases
Rushing to Code
- Take time to understand the problem and plan your approach
- Don’t start coding until you have a clear strategy
9. Advanced Topics for Further Study
Once you’re comfortable with the basics, consider exploring these advanced topics:
Advanced Data Structures
- Segment Trees
- Fenwick Trees (Binary Indexed Trees)
- Disjoint Set Union (Union-Find)
- Suffix Arrays and Suffix Trees
Advanced Algorithms
- Network Flow Algorithms
- Linear Programming
- Advanced Dynamic Programming Techniques
- Approximation Algorithms
Computational Geometry
- Convex Hull Algorithms
- Line Intersection Problems
- Polygon Algorithms
Parallel and Distributed Algorithms
- MapReduce
- Parallel Sorting Algorithms
- Distributed Hash Tables
Machine Learning Algorithms
- Basic Clustering Algorithms
- Decision Trees
- Neural Network Basics
10. Maintaining and Improving Your Skills
Continuous learning and practice are essential to maintain and improve your DSA skills:
Regular Practice
- Set aside time each week for coding practice
- Participate in coding contests or challenges
- Revisit and optimize your old solutions
Stay Updated
- Follow tech blogs and forums for new developments in algorithms
- Attend coding workshops or webinars
- Explore new problem-solving techniques
Teach Others
- Explaining concepts to others reinforces your own understanding
- Consider mentoring junior developers or students
- Contribute to open-source projects or write technical blogs
Apply Skills in Real Projects
- Look for opportunities to apply DSA in your work or personal projects
- Analyze and optimize existing codebases
- Develop tools or applications that utilize advanced data structures or algorithms
Continuous Learning
- Explore new programming languages and paradigms
- Study emerging technologies and their algorithmic challenges
- Read research papers on cutting-edge algorithms and data structures
Conclusion
Learning data structures and algorithms for interviews is a journey that requires dedication, consistent practice, and a strategic approach. By following this comprehensive guide, you’ll be well-equipped to tackle technical interviews at top tech companies. Remember, the key to success lies not just in memorizing solutions, but in developing a deep understanding of fundamental concepts and honing your problem-solving skills.
Start with the basics, gradually build your knowledge, and consistently practice solving diverse problems. Utilize the wealth of resources available, from online platforms to books and courses. As you progress, focus on developing your communication skills and learning to approach problems systematically.
Keep in mind that mastering DSA is not just about acing interviews; it’s about becoming a better programmer and problem solver. These skills will serve you well throughout your career in technology. Stay curious, embrace challenges, and enjoy the learning process. With persistence and the right approach, you’ll be well-prepared to showcase your skills in technical interviews and excel in your programming career.