How to Improve Your Ability to Give and Receive Feedback: A Comprehensive Guide for Programmers
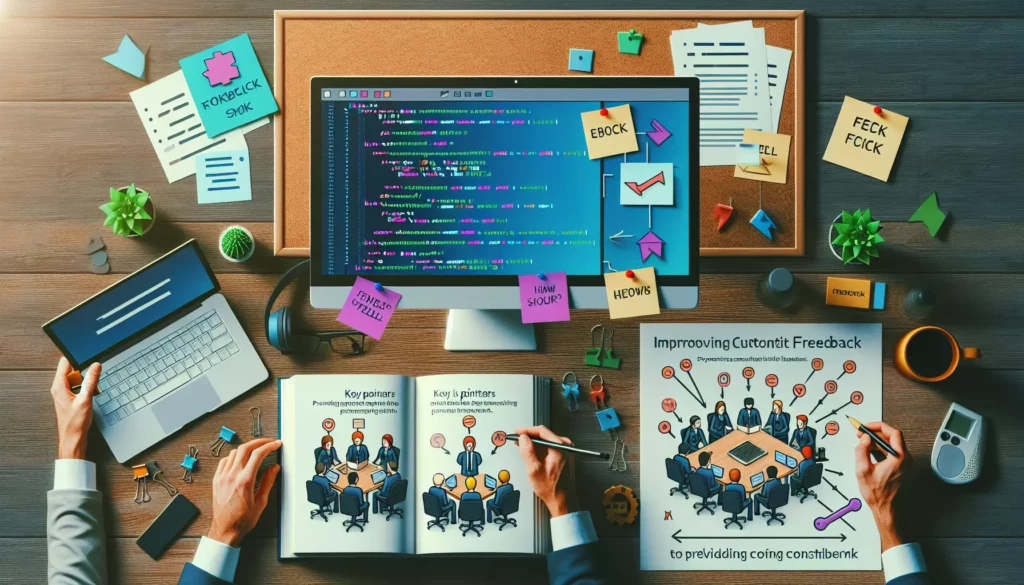
In the fast-paced world of software development, the ability to give and receive feedback effectively is a crucial skill that can significantly impact your career growth and the success of your projects. Whether you’re a beginner coder or an experienced developer preparing for technical interviews at major tech companies, mastering the art of feedback can enhance your problem-solving abilities, improve your code quality, and foster better collaboration within your team.
At AlgoCademy, we understand the importance of feedback in coding education and programming skills development. This comprehensive guide will explore various strategies to improve your ability to give and receive feedback, with a special focus on how these skills apply to the world of coding and algorithmic thinking.
1. Understanding the Importance of Feedback in Programming
Before diving into the specifics of improving your feedback skills, it’s essential to recognize why feedback is so crucial in the programming world:
- Code Quality: Feedback helps identify bugs, inefficiencies, and areas for improvement in your code.
- Skill Development: Constructive feedback accelerates learning and helps you become a better programmer.
- Collaboration: Effective feedback fosters better teamwork and communication among developers.
- Problem-Solving: Feedback can provide new perspectives on tackling complex algorithmic challenges.
- Career Growth: The ability to give and receive feedback is highly valued in technical interviews and professional settings.
2. Giving Effective Feedback in Programming Contexts
2.1. Be Specific and Actionable
When providing feedback on code or problem-solving approaches, always aim to be specific and actionable. Instead of making general statements, point out exact lines of code or specific algorithmic choices that could be improved.
Example of vague feedback:
Your code could be more efficient.
Example of specific, actionable feedback:
Consider using a hash table instead of nested loops in the function findDuplicates(). This could reduce the time complexity from O(n^2) to O(n), significantly improving efficiency for large input sizes.
2.2. Use the Sandwich Method
The sandwich method involves structuring your feedback with positive comments at the beginning and end, with constructive criticism in the middle. This approach can make the feedback more palatable and encourage the recipient to be more receptive.
Example:
Great job implementing the binary search algorithm! The code is well-commented and easy to follow.
One area for improvement could be the handling of edge cases. Consider what happens when the array is empty or contains only one element. Adding checks for these scenarios would make your implementation more robust.
Overall, your solution shows a solid understanding of divide-and-conquer strategies. Keep up the good work!
2.3. Focus on the Code, Not the Coder
When giving feedback, it’s crucial to separate the code from the person who wrote it. Focus on the technical aspects and avoid making personal comments. This approach helps maintain a professional atmosphere and prevents defensive reactions.
Instead of saying:
You always forget to handle null pointer exceptions.
Try:
The function calculateAverage() could benefit from null checks to prevent potential NullPointerExceptions.
2.4. Provide Examples and Alternatives
When suggesting improvements, it’s helpful to provide concrete examples or alternative approaches. This not only clarifies your feedback but also serves as a learning opportunity for the recipient.
Example:
Instead of using a recursive approach for the Fibonacci sequence, consider using dynamic programming. Here's an example of how you could implement it:
public int fibDP(int n) {
if (n <= 1) return n;
int[] dp = new int[n + 1];
dp[0] = 0;
dp[1] = 1;
for (int i = 2; i <= n; i++) {
dp[i] = dp[i-1] + dp[i-2];
}
return dp[n];
}
2.5. Ask Questions
Incorporating questions into your feedback can encourage dialogue and help you understand the reasoning behind certain coding decisions. This approach can lead to more meaningful discussions and potentially uncover alternative solutions.
Example:
I noticed you used a bubble sort algorithm for this problem. What was your thought process behind choosing this sorting method? Have you considered using quicksort or mergesort for potentially better performance on larger datasets?
3. Receiving Feedback Effectively in Programming
3.1. Maintain an Open Mindset
Approach feedback with an open mind, viewing it as an opportunity to learn and improve rather than as criticism. Remember that even experienced programmers continually learn and refine their skills.
3.2. Listen Actively
When receiving feedback, practice active listening. Pay attention to the specifics of the feedback, ask clarifying questions, and take notes if necessary. This demonstrates your engagement and helps ensure you don’t miss important points.
3.3. Avoid Defensive Reactions
It’s natural to feel defensive when receiving criticism about your code, but try to resist this urge. Instead of immediately justifying your choices, take a moment to consider the feedback objectively.
3.4. Ask for Clarification
If you don’t fully understand the feedback or need more information, don’t hesitate to ask for clarification. This shows your commitment to improvement and can lead to more in-depth discussions about coding practices.
Example:
You mentioned that my implementation of the depth-first search algorithm could be more memory-efficient. Could you elaborate on specific areas where I could optimize memory usage?
3.5. Follow Up and Implement Changes
After receiving feedback, make an effort to implement the suggested changes or explore the recommended alternatives. This demonstrates your willingness to learn and improve. If you decide not to implement certain suggestions, be prepared to explain your reasoning.
4. Leveraging Feedback in Coding Education and Skill Development
4.1. Code Reviews
Participating in code reviews, both as a reviewer and as the person whose code is being reviewed, is an excellent way to improve your feedback skills. Code reviews provide opportunities to practice giving constructive feedback and to learn from others’ perspectives on your code.
4.2. Pair Programming
Pair programming sessions offer real-time opportunities to give and receive feedback. As you work together to solve problems, you can practice providing immediate, constructive feedback and learn to receive and act on suggestions quickly.
4.3. Online Coding Platforms
Platforms like AlgoCademy offer interactive coding tutorials and AI-powered assistance. These tools often provide immediate feedback on your code, helping you identify areas for improvement and learn best practices.
4.4. Open Source Contributions
Contributing to open source projects exposes you to feedback from a diverse community of developers. This can be an excellent way to improve your ability to handle various types of feedback and collaborate with others on complex coding projects.
4.5. Mock Technical Interviews
Participating in mock technical interviews, either as an interviewer or interviewee, can help you practice giving and receiving feedback in a high-pressure situation similar to what you might encounter when interviewing for major tech companies.
5. Overcoming Common Challenges in Giving and Receiving Feedback
5.1. Dealing with Negative Feedback
Receiving negative feedback can be challenging, but it’s often the most valuable for growth. Try to:
- Take a step back and evaluate the feedback objectively
- Focus on the specific points rather than taking it personally
- Use it as motivation to improve your skills
5.2. Handling Disagreements
When you disagree with feedback you’ve received:
- Politely explain your reasoning
- Be open to discussing alternative viewpoints
- If appropriate, suggest running benchmarks or tests to compare different approaches
5.3. Giving Feedback to More Experienced Developers
When providing feedback to senior developers:
- Be respectful and humble
- Back up your suggestions with evidence or reputable sources
- Frame your feedback as questions or observations rather than direct criticism
5.4. Balancing Positive and Negative Feedback
Strive to maintain a balance between positive and negative feedback:
- Acknowledge good coding practices and clever solutions
- Provide constructive criticism for areas of improvement
- Ensure your feedback is comprehensive, covering both strengths and weaknesses
6. Cultivating a Feedback-Friendly Culture in Development Teams
6.1. Establish Clear Feedback Guidelines
Work with your team to create guidelines for giving and receiving feedback. These could include:
- Best practices for code reviews
- Preferred communication channels for different types of feedback
- Expectations for response times and follow-ups
6.2. Lead by Example
If you’re in a leadership position, model good feedback behavior:
- Regularly seek feedback on your own work
- Respond graciously to criticism
- Provide thoughtful, constructive feedback to others
6.3. Create Opportunities for Feedback
Integrate feedback into your team’s regular workflow:
- Schedule regular code review sessions
- Implement a system for peer evaluations
- Encourage informal feedback sharing during team meetings
6.4. Recognize and Reward Good Feedback Practices
Acknowledge team members who excel at giving or implementing feedback:
- Highlight valuable feedback contributions in team meetings
- Consider feedback skills in performance evaluations
- Offer opportunities for growth to those who demonstrate strong feedback skills
7. Leveraging Technology for Better Feedback in Coding
7.1. Static Code Analysis Tools
Utilize static code analysis tools to provide automated feedback on code quality, potential bugs, and adherence to coding standards. Examples include:
- SonarQube
- ESLint for JavaScript
- PyLint for Python
7.2. Code Review Platforms
Use dedicated code review platforms to streamline the feedback process:
- GitHub Pull Requests
- Gerrit
- Crucible
7.3. AI-Powered Coding Assistants
Explore AI-powered tools that can provide real-time feedback and suggestions as you code:
- GitHub Copilot
- Tabnine
- Kite
8. Preparing for Technical Interviews: Feedback in Action
As you prepare for technical interviews, especially for positions at major tech companies, your ability to give and receive feedback becomes crucial. Here’s how to apply your improved feedback skills in this context:
8.1. Mock Interviews
Participate in mock interviews where you can practice:
- Receiving feedback on your problem-solving approach
- Giving feedback to your interviewer on the clarity of the problem statement
- Implementing feedback in real-time as you work through coding challenges
8.2. Collaborative Problem Solving
Many technical interviews involve collaborative problem-solving. Practice:
- Clearly communicating your thought process
- Incorporating suggestions from the interviewer
- Providing constructive feedback on alternative approaches suggested by the interviewer
8.3. Code Review Simulation
Some interviews may include a code review component. Prepare by:
- Reviewing sample code and practicing giving constructive feedback
- Learning to quickly identify and articulate potential improvements in code efficiency, readability, and best practices
- Practicing how to receive and respond to feedback on your own code in a professional manner
Conclusion
Improving your ability to give and receive feedback is a valuable investment in your programming career. It enhances your technical skills, fosters better collaboration, and prepares you for success in technical interviews and professional settings.
Remember that developing these skills is an ongoing process. Continue to practice, seek out feedback opportunities, and reflect on your experiences. As you progress in your coding journey, whether you’re working through AlgoCademy’s interactive tutorials or preparing for interviews at top tech companies, your improved feedback skills will be a powerful asset in your continuous growth as a programmer.
By mastering the art of feedback, you’ll not only become a better coder but also a more valuable team member and a more competitive candidate in the job market. Embrace feedback as a tool for growth, and watch as it transforms your approach to problem-solving, collaboration, and career development in the exciting world of programming.