How to Improve Decision-Making Skills as a Developer
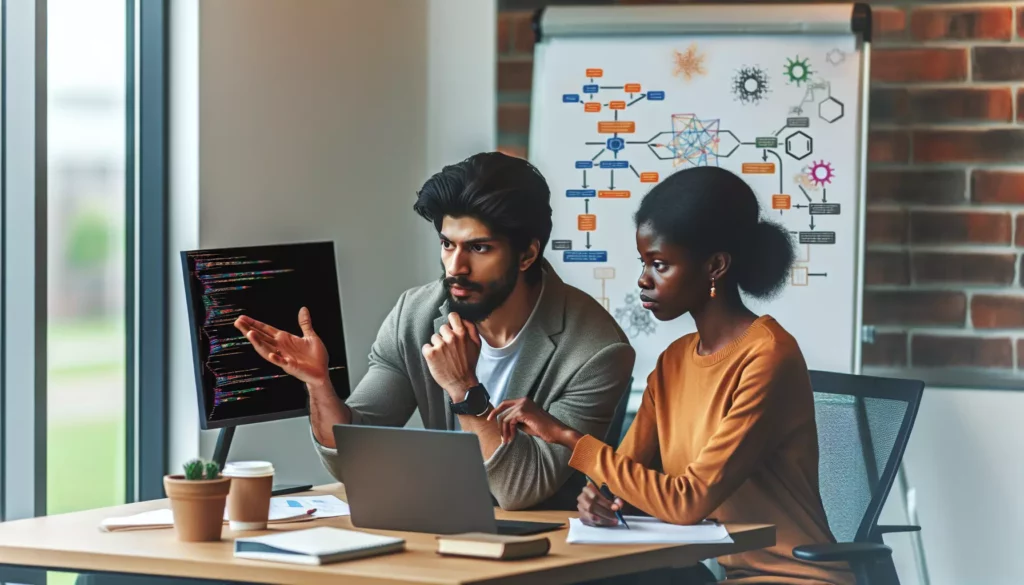
In the fast-paced world of software development, the ability to make sound decisions quickly and effectively is a crucial skill that can set you apart from your peers. As developers, we are constantly faced with choices that can impact the success of our projects, the efficiency of our code, and the overall quality of our work. Whether you’re a beginner just starting your journey in coding or an experienced developer looking to refine your skills, improving your decision-making abilities is essential for career growth and success in the tech industry.
In this comprehensive guide, we’ll explore various strategies and techniques to enhance your decision-making skills as a developer. We’ll delve into the importance of this skill in the context of coding education and programming skills development, and how it relates to preparing for technical interviews at major tech companies. By the end of this article, you’ll have a solid foundation for making better choices in your development work and advancing your career.
1. Understand the Importance of Decision-Making in Development
Before we dive into specific techniques, it’s crucial to recognize why decision-making skills are so vital in the field of software development:
- Problem-solving: Development is essentially about solving problems, and each problem requires multiple decisions to reach a solution.
- Code quality: The choices you make directly impact the quality, maintainability, and efficiency of your code.
- Project management: Decisions about timelines, resource allocation, and priorities can make or break a project.
- Technical debt: Poor decisions can lead to technical debt, which can hinder future development and scalability.
- Career advancement: Strong decision-making skills are highly valued by employers and can lead to leadership roles.
2. Cultivate Algorithmic Thinking
Algorithmic thinking is at the core of effective decision-making in programming. It involves breaking down complex problems into smaller, manageable steps and considering various approaches to solve them. Here’s how you can improve your algorithmic thinking:
- Practice problem-solving: Regularly engage in coding challenges and algorithmic puzzles on platforms like AlgoCademy.
- Analyze existing algorithms: Study well-known algorithms and understand the decision-making process behind their design.
- Implement algorithms from scratch: Try implementing common algorithms without referencing existing code to deepen your understanding.
- Optimize solutions: After solving a problem, look for ways to optimize your solution, considering time and space complexity.
Here’s a simple example of how algorithmic thinking can improve decision-making in code:
// Inefficient approach
function findMaxNumber(numbers) {
return Math.max(...numbers);
}
// More efficient approach using algorithmic thinking
function findMaxNumber(numbers) {
let max = numbers[0];
for (let i = 1; i < numbers.length; i++) {
if (numbers[i] > max) {
max = numbers[i];
}
}
return max;
}
In this example, the second approach demonstrates better decision-making by considering performance implications and avoiding the spread operator for large arrays.
3. Develop a Strong Foundation in Data Structures
A solid understanding of data structures is crucial for making informed decisions about how to organize and manipulate data efficiently. Familiarize yourself with common data structures such as:
- Arrays and Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
Knowing when to use each data structure can significantly impact the performance and readability of your code. For instance, consider the following scenario:
// Inefficient approach using an array
const dictionary = [];
function addWord(word, definition) {
dictionary.push({ word, definition });
}
function findDefinition(word) {
return dictionary.find(entry => entry.word === word)?.definition;
}
// More efficient approach using a hash table (object in JavaScript)
const dictionary = {};
function addWord(word, definition) {
dictionary[word] = definition;
}
function findDefinition(word) {
return dictionary[word];
}
The second approach demonstrates better decision-making by using an appropriate data structure (hash table) for faster lookups.
4. Learn from Code Reviews and Collaborate
Code reviews are an excellent opportunity to improve your decision-making skills. By examining others’ code and having your code reviewed, you can:
- Gain exposure to different problem-solving approaches
- Learn best practices and industry standards
- Understand the rationale behind certain decisions
- Receive constructive feedback on your own decision-making process
To make the most of code reviews:
- Approach reviews with an open mind and a willingness to learn
- Ask questions about decisions you don’t understand
- Explain your own decision-making process when presenting your code
- Implement feedback and track your progress over time
5. Embrace Design Patterns and Architectural Principles
Design patterns and architectural principles provide tested solutions to common software design problems. By studying and applying these concepts, you can make more informed decisions about code structure and organization. Some important patterns and principles to learn include:
- SOLID principles
- Creational patterns (e.g., Singleton, Factory)
- Structural patterns (e.g., Adapter, Decorator)
- Behavioral patterns (e.g., Observer, Strategy)
- Architectural patterns (e.g., MVC, MVVM)
Here’s an example of how applying a design pattern can lead to better decision-making:
// Without design pattern
class UserService {
constructor(database) {
this.database = database;
}
getUser(id) {
return this.database.query(`SELECT * FROM users WHERE id = ${id}`);
}
}
// With Repository pattern
class UserRepository {
constructor(database) {
this.database = database;
}
getUser(id) {
return this.database.query(`SELECT * FROM users WHERE id = ${id}`);
}
}
class UserService {
constructor(userRepository) {
this.userRepository = userRepository;
}
getUser(id) {
return this.userRepository.getUser(id);
}
}
The second approach demonstrates better decision-making by separating concerns and improving testability and maintainability.
6. Stay Updated with Technology Trends
The tech industry evolves rapidly, and staying informed about new technologies, frameworks, and best practices is crucial for making relevant decisions. To stay up-to-date:
- Follow tech blogs and news sites
- Attend conferences and meetups
- Participate in online developer communities
- Experiment with new technologies in side projects
- Read books and take online courses on emerging topics
Being aware of the latest trends will help you make informed decisions about which technologies to learn and adopt in your projects.
7. Develop Critical Thinking Skills
Critical thinking is the foundation of good decision-making. To improve your critical thinking skills:
- Question assumptions and biases
- Analyze problems from multiple perspectives
- Consider long-term implications of decisions
- Practice logical reasoning
- Seek evidence to support or refute ideas
Apply critical thinking to your coding decisions by asking questions like:
- Is this the most efficient solution?
- How will this code scale as the project grows?
- What are the potential edge cases or failure points?
- Are there any security implications to consider?
- How does this decision align with the project’s overall architecture?
8. Use Decision-Making Frameworks
Adopting structured decision-making frameworks can help you approach complex problems systematically. Some useful frameworks include:
- SWOT Analysis: Evaluate Strengths, Weaknesses, Opportunities, and Threats
- Decision Matrix: Compare options based on weighted criteria
- Pros and Cons List: List advantages and disadvantages of each option
- Six Thinking Hats: Consider decisions from different perspectives (facts, emotions, risks, benefits, creativity, process)
For example, when deciding on a new technology stack for a project, you might use a decision matrix:
Criteria | Weight | React | Vue | Angular |
---|---|---|---|---|
Learning Curve | 0.3 | 8 | 9 | 6 |
Performance | 0.2 | 9 | 8 | 7 |
Community Support | 0.2 | 10 | 8 | 9 |
Ecosystem | 0.3 | 9 | 7 | 8 |
Total Score | 8.9 | 8.0 | 7.4 |
This framework helps you make a more objective decision based on weighted criteria.
9. Practice Time Management and Prioritization
Effective decision-making often involves managing time and priorities. Improve these skills by:
- Using time management techniques like the Pomodoro Technique
- Prioritizing tasks using methods like the Eisenhower Matrix
- Setting SMART (Specific, Measurable, Achievable, Relevant, Time-bound) goals
- Learning to say no to low-priority tasks or requests
- Regularly reviewing and adjusting your priorities
Good time management allows you to allocate sufficient time for important decisions and avoid rushed judgments.
10. Develop Emotional Intelligence
Emotional intelligence plays a crucial role in decision-making, especially when working in teams or dealing with stakeholders. To improve your emotional intelligence:
- Practice self-awareness and recognize your own emotions
- Develop empathy and consider others’ perspectives
- Learn to manage stress and maintain composure under pressure
- Improve your communication skills
- Work on conflict resolution techniques
Emotional intelligence can help you navigate complex decision-making scenarios, especially when there are conflicting opinions or high-stakes outcomes.
11. Learn from Your Mistakes
Making mistakes is an inevitable part of the learning process. To improve your decision-making skills, it’s crucial to learn from your errors:
- Conduct post-mortems after project completions or major milestones
- Keep a decision journal to track your choices and their outcomes
- Analyze what went wrong and why when faced with unfavorable results
- Seek feedback from peers and mentors on your decision-making process
- Be open to admitting mistakes and taking responsibility for your decisions
Remember, the goal is not to avoid mistakes entirely but to learn from them and make better decisions in the future.
12. Simulate Real-World Scenarios
To prepare for technical interviews and real-world development challenges, practice making decisions in simulated scenarios:
- Participate in coding competitions that require quick problem-solving
- Work on open-source projects to gain experience in real-world codebases
- Create mock projects that mimic complex business requirements
- Practice whiteboard coding and system design exercises
- Engage in pair programming sessions to discuss decision-making in real-time
These simulations will help you develop the ability to make decisions under pressure and with limited information, which is often the case in technical interviews and real-world development scenarios.
13. Leverage AI and Tools for Decision Support
While it’s important to develop your own decision-making skills, don’t hesitate to leverage AI and tools for support. Many platforms, including AlgoCademy, offer AI-powered assistance that can help you:
- Analyze code for potential improvements
- Suggest optimizations and best practices
- Provide insights into algorithm complexity and performance
- Offer step-by-step guidance for problem-solving
- Compare different approaches to solving a problem
Use these tools to supplement your decision-making process, but always strive to understand the reasoning behind the suggestions.
Conclusion
Improving your decision-making skills as a developer is a continuous journey that requires dedication, practice, and a willingness to learn from both successes and failures. By focusing on algorithmic thinking, understanding data structures, collaborating with others, staying updated with technology trends, and developing critical thinking skills, you’ll be well-equipped to make better choices in your development work.
Remember that good decision-making is not just about technical knowledge; it also involves time management, emotional intelligence, and the ability to learn from mistakes. As you progress in your career, these skills will become increasingly valuable, helping you tackle complex problems, lead teams, and excel in technical interviews at top tech companies.
Embrace the challenge of improving your decision-making skills, and you’ll find that it not only enhances your coding abilities but also opens up new opportunities for growth and success in the ever-evolving world of software development. Keep practicing, stay curious, and don’t be afraid to make decisions – even if they’re not always perfect. With time and experience, you’ll develop the confidence and expertise to make sound choices that drive your projects and career forward.