How to Improve Coding Speed and Efficiency: A Comprehensive Guide
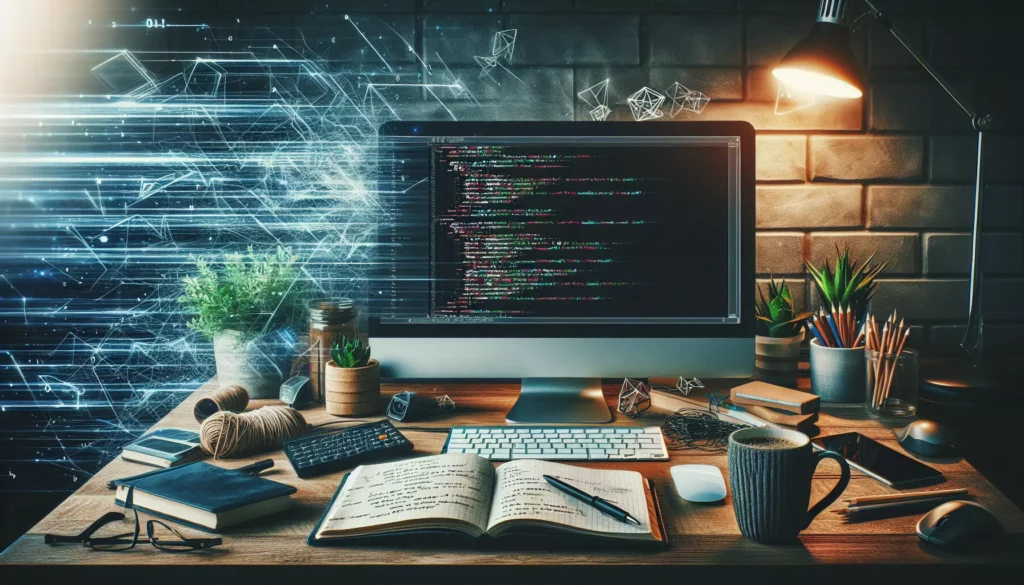
In today’s fast-paced tech world, being able to code quickly and efficiently is a valuable skill that can set you apart from other developers. Whether you’re a beginner looking to enhance your coding abilities or an experienced programmer aiming to boost your productivity, this guide will provide you with practical strategies to improve your coding speed and efficiency.
Table of Contents
- Understanding the Importance of Coding Speed and Efficiency
- Mastering the Fundamentals
- Practice Regularly
- Learn Keyboard Shortcuts and IDE Features
- Use Version Control Effectively
- Implement Design Patterns and Best Practices
- Optimize Your Workflow
- Leverage Coding Tools and Libraries
- Write Clean and Maintainable Code
- Embrace Continuous Learning
- Improve Time Management Skills
- Engage in Collaborative Coding
- Participate in Code Reviews
- Automate Repetitive Tasks
- Enhance Problem-Solving Skills
- Conclusion
1. Understanding the Importance of Coding Speed and Efficiency
Before diving into specific techniques, it’s crucial to understand why coding speed and efficiency matter. In the world of software development, time is often of the essence. Faster coding can lead to:
- Increased productivity
- Quicker project completion
- More time for testing and refinement
- Ability to take on more projects
- Improved job prospects and career advancement
However, it’s important to note that speed should never come at the cost of code quality. The goal is to find the right balance between speed and maintaining clean, efficient, and bug-free code.
2. Mastering the Fundamentals
To code quickly and efficiently, you need a solid foundation in programming basics. This includes:
- Understanding data structures and algorithms
- Mastering the syntax and features of your primary programming language(s)
- Familiarity with common programming paradigms (e.g., object-oriented, functional)
- Knowledge of software design principles
Platforms like AlgoCademy offer interactive coding tutorials and resources that can help you strengthen your fundamental skills. Make sure to invest time in building a strong foundation, as it will pay dividends in the long run.
3. Practice Regularly
As with any skill, regular practice is key to improving your coding speed and efficiency. Here are some ways to incorporate practice into your routine:
- Solve coding challenges on platforms like LeetCode, HackerRank, or CodeWars
- Participate in coding competitions or hackathons
- Work on personal projects or contribute to open-source projects
- Set aside dedicated time each day for coding practice
AlgoCademy’s focus on algorithmic thinking and problem-solving can be particularly helpful in honing your skills through regular practice.
4. Learn Keyboard Shortcuts and IDE Features
Mastering your development environment can significantly boost your coding speed. Invest time in learning:
- Keyboard shortcuts for your preferred IDE or text editor
- Advanced features like code completion, refactoring tools, and debugging capabilities
- Customization options to tailor your environment to your needs
For example, in Visual Studio Code, you can use the following shortcuts to speed up your coding:
Ctrl + Space: Trigger suggestions
Ctrl + Shift + P: Open command palette
Ctrl + /: Toggle line comment
Alt + Up/Down: Move line up/down
Ctrl + D: Select next occurrence
Ctrl + F2: Select all occurrences
Take the time to explore and memorize these shortcuts, as they can save you countless hours in the long run.
5. Use Version Control Effectively
Version control systems like Git not only help you manage your code but can also improve your coding efficiency. Learn to:
- Use branching strategies effectively
- Write meaningful commit messages
- Utilize Git commands and features to streamline your workflow
Here’s an example of how to create a new branch and switch to it in one command:
git checkout -b feature/new-feature
By mastering version control, you’ll be able to manage your projects more efficiently and collaborate more effectively with other developers.
6. Implement Design Patterns and Best Practices
Familiarize yourself with common design patterns and best practices in software development. This knowledge will help you:
- Solve common problems more quickly
- Write more maintainable and scalable code
- Communicate more effectively with other developers
For instance, the Singleton pattern can be implemented in Python like this:
class Singleton:
_instance = None
def __new__(cls):
if cls._instance is None:
cls._instance = super().__new__(cls)
return cls._instance
# Usage
s1 = Singleton()
s2 = Singleton()
print(s1 is s2) # Output: True
By recognizing and applying these patterns, you can write more efficient code and solve problems more quickly.
7. Optimize Your Workflow
Analyze your current workflow and look for areas where you can improve efficiency. Consider:
- Using task management tools to organize your work
- Implementing the Pomodoro Technique or other time management strategies
- Creating templates for common code structures or project setups
- Setting up a comfortable and ergonomic workspace
Remember, small optimizations can add up to significant time savings over time.
8. Leverage Coding Tools and Libraries
Don’t reinvent the wheel. Make use of existing tools and libraries to speed up your development process:
- Use package managers like npm, pip, or Maven to easily install and manage dependencies
- Leverage frameworks and libraries that align with your project requirements
- Utilize code generators for boilerplate code
- Implement linters and formatters to maintain code quality and consistency
For example, you can use the Black code formatter in Python to automatically format your code:
pip install black
black your_file.py
This ensures consistent code style without manual effort, saving you time and mental energy.
9. Write Clean and Maintainable Code
While it might seem counterintuitive, writing clean code can actually improve your coding speed in the long run. Follow these principles:
- Use meaningful variable and function names
- Keep functions small and focused on a single task
- Follow the DRY (Don’t Repeat Yourself) principle
- Write self-documenting code
- Use comments judiciously to explain complex logic
Clean code is easier to understand, debug, and maintain, which saves time in the long term.
10. Embrace Continuous Learning
The tech industry is constantly evolving, and staying updated can help you code more efficiently. To keep learning:
- Follow tech blogs and newsletters
- Attend conferences and workshops
- Take online courses or participate in coding bootcamps
- Read books on programming and software development
- Explore new languages and technologies
Platforms like AlgoCademy can be valuable resources for continuous learning, offering up-to-date content and interactive learning experiences.
11. Improve Time Management Skills
Effective time management is crucial for improving coding speed and efficiency. Consider these strategies:
- Use time-tracking tools to understand how you spend your coding time
- Set realistic goals and deadlines for your tasks
- Break large projects into smaller, manageable tasks
- Minimize distractions during coding sessions
- Take regular breaks to maintain focus and prevent burnout
Remember, working smarter often leads to better results than simply working longer hours.
12. Engage in Collaborative Coding
Collaborative coding can significantly improve your efficiency and expose you to new techniques. Try:
- Pair programming sessions with colleagues or fellow developers
- Participating in coding dojos or mob programming sessions
- Collaborating on open-source projects
- Joining coding communities and forums
These experiences can help you learn from others, share knowledge, and discover new ways to approach problems.
13. Participate in Code Reviews
Actively participating in code reviews, both as a reviewer and a submitter, can improve your coding skills and efficiency:
- Learn from others’ code and feedback
- Identify common mistakes and best practices
- Improve your ability to read and understand code quickly
- Develop a critical eye for code quality
Code reviews also help maintain high code quality standards within a team or project.
14. Automate Repetitive Tasks
Identify repetitive tasks in your development process and look for ways to automate them. This could include:
- Setting up continuous integration and deployment (CI/CD) pipelines
- Creating scripts for common development tasks
- Using code generators for repetitive code patterns
- Implementing automated testing
Here’s a simple example of a bash script to automate the process of creating a new Python project structure:
#!/bin/bash
project_name=$1
mkdir $project_name
cd $project_name
mkdir src tests docs
touch src/__init__.py
touch tests/__init__.py
touch README.md
echo "# $project_name" > README.md
echo "Project structure created for $project_name"
Automation not only saves time but also reduces the likelihood of human error in repetitive tasks.
15. Enhance Problem-Solving Skills
Improving your problem-solving skills can significantly boost your coding speed and efficiency. Try these approaches:
- Practice breaking down complex problems into smaller, manageable parts
- Learn and apply various problem-solving techniques (e.g., divide and conquer, dynamic programming)
- Analyze and understand existing solutions to common problems
- Challenge yourself with algorithmic puzzles and coding challenges
AlgoCademy’s focus on algorithmic thinking and problem-solving can be particularly beneficial in developing these skills.
Conclusion
Improving your coding speed and efficiency is a continuous journey that requires dedication, practice, and a willingness to learn and adapt. By implementing the strategies outlined in this guide, you can significantly enhance your coding skills and productivity.
Remember that the goal is not just to code faster, but to produce high-quality, maintainable code more efficiently. Balance speed with good coding practices, and always prioritize writing clean, well-structured code.
As you continue to develop your skills, platforms like AlgoCademy can provide valuable resources, interactive tutorials, and AI-powered assistance to support your learning journey. Whether you’re preparing for technical interviews at major tech companies or simply looking to improve your coding abilities, consistent effort and smart practice will lead to noticeable improvements in your coding speed and efficiency.
Keep coding, keep learning, and enjoy the process of becoming a more proficient and efficient programmer!