How to Implement Simple Encryption and Decryption
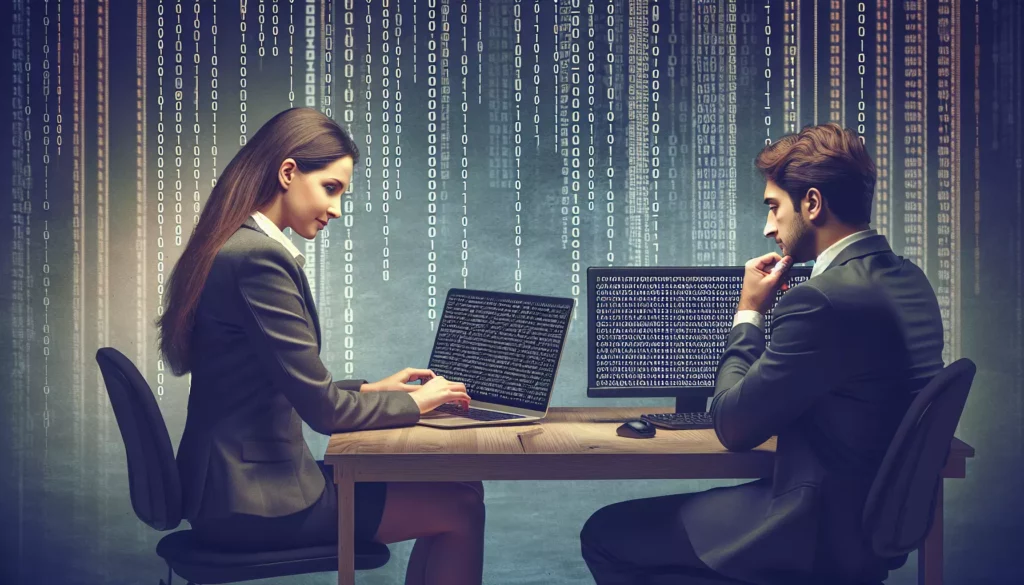
Encryption is a fundamental concept in computer science and cybersecurity, playing a crucial role in protecting sensitive information from unauthorized access. In this comprehensive guide, we’ll explore the basics of encryption and decryption, and walk through the implementation of simple encryption algorithms. Whether you’re a beginner programmer or preparing for technical interviews at top tech companies, understanding these concepts will enhance your coding skills and deepen your knowledge of data security.
Table of Contents
- Understanding Encryption and Decryption
- The Caesar Cipher: A Classic Example
- Implementing the Caesar Cipher in Python
- XOR Encryption: A Simple Symmetric Algorithm
- Implementing XOR Encryption in Python
- Base64 Encoding: Not Encryption, But Useful
- Implementing Base64 Encoding in Python
- Advanced Encryption Concepts
- Best Practices for Encryption in Real-World Applications
- Conclusion
1. Understanding Encryption and Decryption
Encryption is the process of converting plaintext (readable data) into ciphertext (scrambled data) using an algorithm and a key. Decryption is the reverse process, converting ciphertext back into plaintext. The main goal of encryption is to ensure data confidentiality, making it unreadable to unauthorized parties.
There are two main types of encryption:
- Symmetric Encryption: Uses the same key for both encryption and decryption.
- Asymmetric Encryption: Uses a pair of keys – a public key for encryption and a private key for decryption.
In this tutorial, we’ll focus on implementing simple symmetric encryption algorithms to help you grasp the fundamental concepts.
2. The Caesar Cipher: A Classic Example
The Caesar Cipher is one of the oldest and simplest encryption techniques. Named after Julius Caesar, who allegedly used it to communicate with his generals, this cipher involves shifting each letter in the plaintext by a fixed number of positions in the alphabet.
For example, with a shift of 3:
- A becomes D
- B becomes E
- C becomes F
- … and so on
The shift value serves as the encryption key. To decrypt, you simply shift the letters back by the same number of positions.
3. Implementing the Caesar Cipher in Python
Let’s implement the Caesar Cipher in Python. We’ll create functions for both encryption and decryption:
def caesar_encrypt(plaintext, shift):
ciphertext = ""
for char in plaintext:
if char.isalpha():
ascii_offset = 65 if char.isupper() else 97
shifted = (ord(char) - ascii_offset + shift) % 26 + ascii_offset
ciphertext += chr(shifted)
else:
ciphertext += char
return ciphertext
def caesar_decrypt(ciphertext, shift):
return caesar_encrypt(ciphertext, -shift)
# Example usage
message = "Hello, World!"
shift = 3
encrypted = caesar_encrypt(message, shift)
decrypted = caesar_decrypt(encrypted, shift)
print(f"Original: {message}")
print(f"Encrypted: {encrypted}")
print(f"Decrypted: {decrypted}")
This implementation handles both uppercase and lowercase letters while preserving non-alphabetic characters. The caesar_encrypt
function shifts each letter by the specified amount, wrapping around the alphabet if necessary. The caesar_decrypt
function simply calls caesar_encrypt
with a negative shift to reverse the process.
When you run this code, you’ll see output similar to:
Original: Hello, World!
Encrypted: Khoor, Zruog!
Decrypted: Hello, World!
While the Caesar Cipher is easy to understand and implement, it’s not secure for modern use as it can be easily broken through frequency analysis or brute-force attempts.
4. XOR Encryption: A Simple Symmetric Algorithm
XOR (exclusive or) encryption is a simple yet powerful technique used in many cryptographic operations. It’s based on the XOR logical operation, which has an interesting property: when you XOR a value with a key and then XOR the result with the same key, you get the original value back.
This property makes XOR encryption both simple to implement and symmetric (the same operation is used for encryption and decryption).
5. Implementing XOR Encryption in Python
Let’s implement a simple XOR encryption function in Python:
def xor_encrypt_decrypt(data, key):
# Convert data and key to bytearray for byte-level operations
data = bytearray(data.encode())
key = bytearray(key.encode())
# XOR each byte of data with the corresponding byte of the key
for i in range(len(data)):
data[i] ^= key[i % len(key)]
return data.decode()
# Example usage
message = "XOR encryption is simple but effective!"
key = "SECRET"
encrypted = xor_encrypt_decrypt(message, key)
decrypted = xor_encrypt_decrypt(encrypted, key)
print(f"Original: {message}")
print(f"Encrypted: {encrypted}")
print(f"Decrypted: {decrypted}")
In this implementation:
- We convert both the data and key to bytearrays to perform byte-level XOR operations.
- We iterate through each byte of the data, XORing it with the corresponding byte of the key.
- If the key is shorter than the data, we cycle through the key using the modulo operator.
- The same function is used for both encryption and decryption due to the nature of XOR.
When you run this code, you’ll see output similar to:
Original: XOR encryption is simple but effective!
Encrypted: (garbled text)
Decrypted: XOR encryption is simple but effective!
The encrypted text will appear garbled because it contains non-printable characters. This simple XOR encryption is more secure than the Caesar Cipher but still not suitable for serious cryptographic applications.
6. Base64 Encoding: Not Encryption, But Useful
While not an encryption method, Base64 encoding is a useful technique often used in conjunction with encryption. It’s a way of encoding binary data into ASCII string format, which is helpful when you need to transmit binary data over text-based protocols.
Base64 uses a set of 64 characters (A-Z, a-z, 0-9, + and /) to represent binary data. It’s commonly used in email attachments, storing complex data in JSON, and as part of more complex encryption schemes.
7. Implementing Base64 Encoding in Python
Python provides a built-in base64
module for Base64 encoding and decoding. Here’s how to use it:
import base64
def base64_encode(data):
# Convert string to bytes and then to base64
return base64.b64encode(data.encode()).decode()
def base64_decode(encoded_data):
# Decode base64 to bytes and then to string
return base64.b64decode(encoded_data).decode()
# Example usage
message = "Base64 is not encryption, but it's useful!"
encoded = base64_encode(message)
decoded = base64_decode(encoded)
print(f"Original: {message}")
print(f"Encoded: {encoded}")
print(f"Decoded: {decoded}")
When you run this code, you’ll see output similar to:
Original: Base64 is not encryption, but it's useful!
Encoded: QmFzZTY0IGlzIG5vdCBlbmNyeXB0aW9uLCBidXQgaXQncyB1c2VmdWwh
Decoded: Base64 is not encryption, but it's useful!
Remember, Base64 encoding is reversible and does not provide any security. It’s primarily used for data encoding, not encryption.
8. Advanced Encryption Concepts
While the simple encryption methods we’ve covered are great for learning, real-world applications require more sophisticated approaches. Here are some advanced concepts you should be aware of:
- Block Ciphers: Algorithms that encrypt fixed-size blocks of data, such as AES (Advanced Encryption Standard).
- Stream Ciphers: Algorithms that encrypt data bit by bit or byte by byte, like ChaCha20.
- Public Key Cryptography: Asymmetric encryption systems like RSA that use separate keys for encryption and decryption.
- Hash Functions: One-way functions that generate a fixed-size output from variable-size input, used for data integrity checks and password storage.
- Digital Signatures: Techniques to verify the authenticity and integrity of digital messages or documents.
- Key Exchange Protocols: Methods for securely exchanging cryptographic keys over a public channel, such as Diffie-Hellman.
These advanced topics are crucial for building secure systems and are often the subject of technical interviews at top tech companies.
9. Best Practices for Encryption in Real-World Applications
When implementing encryption in real-world applications, keep these best practices in mind:
- Never Invent Your Own Cryptography: Always use well-established, peer-reviewed encryption algorithms and libraries.
- Keep Keys Secure: Protect encryption keys with strong access controls and consider using key management systems.
- Use Strong Random Number Generators: For generating keys and initialization vectors, use cryptographically secure random number generators.
- Implement Proper Key Rotation: Regularly update and rotate encryption keys to limit the impact of potential breaches.
- Encrypt Data in Transit and at Rest: Use protocols like TLS for data in transit and encrypt sensitive data before storing it.
- Be Aware of Regulations: Understand and comply with relevant data protection regulations in your jurisdiction.
- Regularly Update and Patch: Keep your cryptographic libraries and systems up to date to address known vulnerabilities.
- Use Authenticated Encryption: When possible, use encryption modes that provide both confidentiality and authenticity, like AES-GCM.
- Implement Proper Error Handling: Avoid leaking sensitive information through error messages or logs.
- Conduct Security Audits: Regularly review and test your encryption implementations for vulnerabilities.
10. Conclusion
Understanding encryption and decryption is crucial for any programmer, especially those aspiring to work at top tech companies. While we’ve covered simple implementations like the Caesar Cipher and XOR encryption, it’s important to remember that these are primarily educational tools. Real-world applications require more robust, standardized encryption methods.
As you continue your journey in coding education and skills development, consider exploring more advanced topics in cryptography. Practice implementing various encryption algorithms, study their strengths and weaknesses, and understand how they fit into larger security systems. This knowledge will not only prepare you for technical interviews but also make you a more well-rounded and security-conscious developer.
Remember, the field of cryptography is vast and constantly evolving. Stay curious, keep learning, and always prioritize security in your coding practices. Whether you’re building a small personal project or working on enterprise-level applications, the principles of secure encryption will always be relevant and valuable.