How to Implement Simple Algorithms: Sorting and Searching
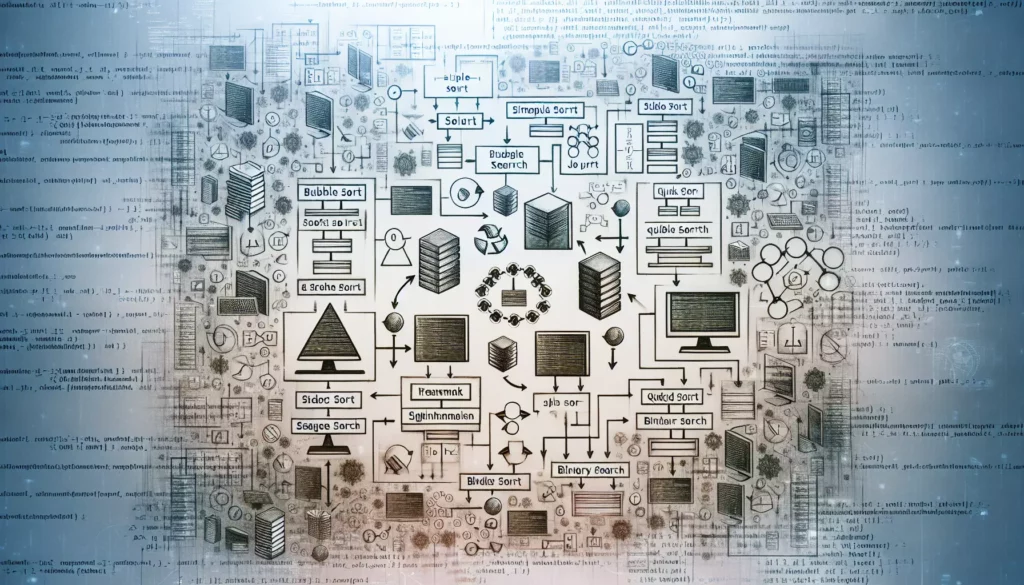
In the world of programming and computer science, algorithms are the backbone of efficient problem-solving. Among the most fundamental and widely used algorithms are those for sorting and searching. These algorithms form the basis for more complex operations and are essential skills for any programmer, from beginners to those preparing for technical interviews at top tech companies. In this comprehensive guide, we’ll explore how to implement simple sorting and searching algorithms, providing you with the knowledge and practical skills to enhance your coding abilities.
Understanding the Importance of Sorting and Searching Algorithms
Before we dive into the implementation details, it’s crucial to understand why sorting and searching algorithms are so important:
- Efficiency: Proper implementation of these algorithms can significantly improve the performance of your programs, especially when dealing with large datasets.
- Foundation for Complex Problems: Many advanced algorithms and data structures build upon the principles of basic sorting and searching techniques.
- Interview Preparation: These algorithms are frequently asked about in technical interviews, particularly for positions at major tech companies.
- Real-world Applications: From organizing data in databases to finding information quickly in applications, sorting and searching are ubiquitous in software development.
Sorting Algorithms
Sorting algorithms arrange elements in a specific order, typically ascending or descending. Let’s explore three fundamental sorting algorithms: Bubble Sort, Selection Sort, and Insertion Sort.
1. Bubble Sort
Bubble Sort is one of the simplest sorting algorithms. It repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order.
How it works:
- Start with the first element.
- Compare it with the next element.
- If the first element is greater than the second, swap them.
- Move to the next pair of adjacent elements and repeat steps 2-3.
- Continue this process until you reach the end of the list.
- Repeat steps 1-5 for each pass through the list until no more swaps are needed.
Python Implementation:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
return arr
# Example usage
unsorted_list = [64, 34, 25, 12, 22, 11, 90]
sorted_list = bubble_sort(unsorted_list)
print("Sorted array:", sorted_list)
Bubble Sort has a time complexity of O(n²) in the worst and average cases, making it inefficient for large datasets. However, it’s easy to understand and implement, making it a good starting point for learning sorting algorithms.
2. Selection Sort
Selection Sort divides the input list into two parts: a sorted portion at the left end and an unsorted portion at the right end. It repeatedly selects the smallest element from the unsorted portion and moves it to the sorted portion.
How it works:
- Find the minimum element in the unsorted portion of the list.
- Swap it with the first element of the unsorted portion.
- Move the boundary between the sorted and unsorted portions one element to the right.
- Repeat steps 1-3 until the entire list is sorted.
Python Implementation:
def selection_sort(arr):
n = len(arr)
for i in range(n):
min_idx = i
for j in range(i + 1, n):
if arr[j] < arr[min_idx]:
min_idx = j
arr[i], arr[min_idx] = arr[min_idx], arr[i]
return arr
# Example usage
unsorted_list = [64, 25, 12, 22, 11]
sorted_list = selection_sort(unsorted_list)
print("Sorted array:", sorted_list)
Selection Sort also has a time complexity of O(n²) for all cases. While it’s not efficient for large lists, it has the advantage of minimizing the number of swaps.
3. Insertion Sort
Insertion Sort builds the final sorted array one item at a time. It’s much less efficient on large lists than more advanced algorithms like QuickSort, HeapSort, or MergeSort. However, it’s efficient for small datasets and is often used as part of more sophisticated algorithms.
How it works:
- Start with the second element of the array.
- Compare it with the elements to its left.
- If it’s smaller than an element to its left, shift that element to the right.
- Continue shifting elements until you find the correct position for the current element.
- Insert the current element into its correct position.
- Move to the next unsorted element and repeat steps 2-5.
Python Implementation:
def insertion_sort(arr):
for i in range(1, len(arr)):
key = arr[i]
j = i - 1
while j >= 0 and key < arr[j]:
arr[j + 1] = arr[j]
j -= 1
arr[j + 1] = key
return arr
# Example usage
unsorted_list = [12, 11, 13, 5, 6]
sorted_list = insertion_sort(unsorted_list)
print("Sorted array:", sorted_list)
Insertion Sort has a time complexity of O(n²) in the worst and average cases, but it can perform well on small datasets or partially sorted arrays.
Searching Algorithms
Searching algorithms are used to find a specific element within a collection of data. We’ll explore two fundamental searching algorithms: Linear Search and Binary Search.
1. Linear Search
Linear Search is the simplest searching algorithm. It sequentially checks each element in the list until a match is found or the end of the list is reached.
How it works:
- Start from the first element of the list.
- Compare the current element with the target value.
- If they match, return the index of the current element.
- If they don’t match, move to the next element.
- Repeat steps 2-4 until a match is found or the end of the list is reached.
- If the end of the list is reached without finding a match, return -1 or a similar indicator that the element wasn’t found.
Python Implementation:
def linear_search(arr, target):
for i in range(len(arr)):
if arr[i] == target:
return i
return -1
# Example usage
my_list = [5, 2, 8, 1, 9, 3]
target = 8
result = linear_search(my_list, target)
if result != -1:
print(f"Element {target} found at index {result}")
else:
print(f"Element {target} not found in the list")
Linear Search has a time complexity of O(n) in the worst case, where n is the number of elements in the list. While it’s not efficient for large datasets, it’s simple to implement and works well for small lists or unsorted data.
2. Binary Search
Binary Search is a much more efficient algorithm for searching in sorted arrays. It repeatedly divides the search interval in half, significantly reducing the number of comparisons needed.
How it works:
- Ensure the array is sorted.
- Set two pointers: one at the start of the array and one at the end.
- Find the middle element of the current range.
- If the middle element is the target, return its index.
- If the target is less than the middle element, repeat the search on the left half of the array.
- If the target is greater than the middle element, repeat the search on the right half of the array.
- Repeat steps 3-6 until the element is found or it’s determined that the element is not in the array.
Python Implementation:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
# Example usage
sorted_list = [1, 3, 5, 7, 9, 11, 13, 15]
target = 7
result = binary_search(sorted_list, target)
if result != -1:
print(f"Element {target} found at index {result}")
else:
print(f"Element {target} not found in the list")
Binary Search has a time complexity of O(log n), making it extremely efficient for large sorted datasets. However, it requires the data to be sorted beforehand, which may not always be feasible or efficient depending on the use case.
Practical Applications and Tips
Understanding these basic sorting and searching algorithms is crucial for several reasons:
- Problem-Solving Skills: Implementing these algorithms helps develop logical thinking and problem-solving abilities essential for programming.
- Performance Optimization: Knowing when to use each algorithm can significantly improve the efficiency of your code, especially when dealing with large datasets.
- Interview Preparation: These algorithms are often the basis for more complex interview questions, particularly at major tech companies.
- Understanding Built-in Functions: Many programming languages have built-in sorting and searching functions. Understanding the underlying algorithms helps you use these functions more effectively.
Tips for Mastering These Algorithms:
- Practice Implementation: Try coding these algorithms from scratch without referring to examples. This will reinforce your understanding and improve your coding skills.
- Analyze Time Complexity: Understand the time complexity of each algorithm and when to use one over the other based on the size and nature of your data.
- Visualize the Process: Use visualization tools or draw out the steps to better understand how each algorithm works.
- Solve Related Problems: Look for coding challenges that involve sorting or searching and apply these algorithms to solve them.
- Explore Variations: Once you’re comfortable with these basic algorithms, explore their variations and more advanced algorithms like QuickSort, MergeSort, or Hash-based searching.
Conclusion
Mastering simple sorting and searching algorithms is a fundamental step in becoming a proficient programmer. These algorithms not only form the basis for more complex problem-solving but are also crucial for optimizing code performance and preparing for technical interviews.
As you continue your journey in programming, remember that understanding these basic algorithms is just the beginning. The world of algorithms is vast and constantly evolving. Keep practicing, exploring new algorithms, and applying your knowledge to real-world problems. With persistence and dedication, you’ll develop the algorithmic thinking skills necessary to tackle even the most challenging coding tasks.
Whether you’re a beginner looking to build a strong foundation in programming or an experienced developer preparing for technical interviews at top tech companies, mastering these sorting and searching algorithms will serve you well throughout your career in software development.