How to Implement Logging in Your Applications: A Comprehensive Guide
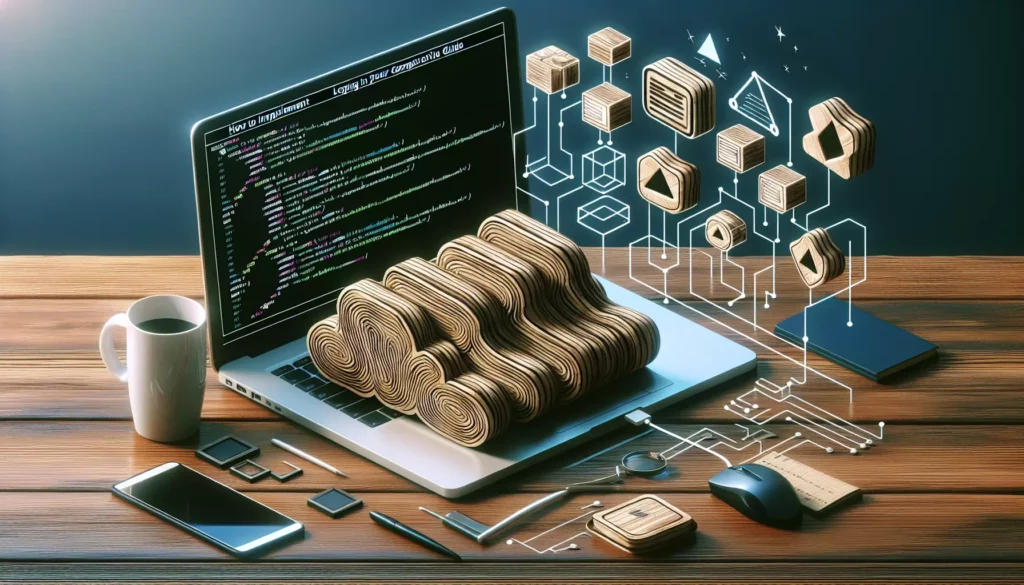
Logging is a crucial aspect of application development that often gets overlooked, especially by beginners. However, implementing a robust logging system can significantly improve your ability to debug, maintain, and monitor your applications. In this comprehensive guide, we’ll explore the ins and outs of logging, why it’s important, and how to implement it effectively in your applications.
Table of Contents
- Why Logging is Important
- Understanding Logging Levels
- Logging Best Practices
- Popular Logging Frameworks
- Implementing Logging in Different Languages
- Structured Logging
- Log Management and Analysis
- Security Considerations in Logging
- Performance Impact of Logging
- Conclusion
1. Why Logging is Important
Logging serves several crucial purposes in application development:
- Debugging: Logs provide valuable information about the application’s state and behavior, making it easier to identify and fix bugs.
- Monitoring: Logs help in monitoring the health and performance of applications in production environments.
- Auditing: Logs can serve as an audit trail for security-related events and user actions.
- Performance Analysis: By logging execution times and resource usage, you can identify performance bottlenecks.
- User Behavior Analysis: Logs can provide insights into how users interact with your application.
Implementing logging early in the development process can save countless hours of debugging and troubleshooting later on. It’s an investment that pays off in the long run, especially as your application grows in complexity and scale.
2. Understanding Logging Levels
Logging levels help categorize log messages based on their severity and importance. Common logging levels include:
- TRACE: The most detailed level, used for fine-grained debugging.
- DEBUG: Detailed information, typically useful for debugging.
- INFO: General information about the application’s state.
- WARN: Indicates potential issues that don’t prevent the application from functioning.
- ERROR: Indicates errors that need attention but don’t crash the application.
- FATAL: Severe errors that cause the application to crash or become unusable.
Using appropriate logging levels helps in filtering and managing logs effectively. For example, you might use DEBUG level during development and switch to INFO or WARN in production to reduce noise and improve performance.
3. Logging Best Practices
To make the most of your logging implementation, follow these best practices:
- Be Consistent: Use a consistent format for log messages across your application.
- Include Contextual Information: Log relevant details like timestamps, user IDs, and transaction IDs.
- Use Appropriate Logging Levels: Choose the right level for each log message based on its importance.
- Log Exceptions Properly: Include stack traces and relevant details when logging exceptions.
- Avoid Sensitive Information: Never log sensitive data like passwords or personal information.
- Use Structured Logging: Consider using structured logging formats like JSON for easier parsing and analysis.
- Implement Log Rotation: Set up log rotation to manage file sizes and prevent disk space issues.
- Configure Logging Dynamically: Allow logging levels to be changed at runtime without restarting the application.
- Use Meaningful Log Messages: Write clear, concise log messages that provide valuable information.
- Consider Performance: Be mindful of the performance impact, especially for high-volume logging.
4. Popular Logging Frameworks
Many programming languages have popular logging frameworks that provide robust features and make implementation easier. Here are some examples:
- Java: Log4j, SLF4J, Logback
- Python: logging (built-in), loguru
- JavaScript: Winston, Bunyan
- C#: NLog, Serilog
- Ruby: Logger (built-in), Log4r
- Go: log (built-in), zerolog, logrus
These frameworks often provide additional features like log formatting, output to multiple destinations, and integration with other tools and services.
5. Implementing Logging in Different Languages
Let’s look at how to implement basic logging in some popular programming languages:
Python
Python’s built-in logging module provides a flexible framework for generating log messages:
import logging
# Configure the logging system
logging.basicConfig(level=logging.INFO,
format='%(asctime)s - %(name)s - %(levelname)s - %(message)s',
filename='app.log')
# Create a logger object
logger = logging.getLogger(__name__)
# Log messages
logger.debug('This is a debug message')
logger.info('This is an info message')
logger.warning('This is a warning message')
logger.error('This is an error message')
logger.critical('This is a critical message')
Java
In Java, you can use the java.util.logging package or more popular frameworks like Log4j:
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class LoggingExample {
private static final Logger logger = LogManager.getLogger(LoggingExample.class);
public static void main(String[] args) {
logger.trace("This is a trace message");
logger.debug("This is a debug message");
logger.info("This is an info message");
logger.warn("This is a warning message");
logger.error("This is an error message");
logger.fatal("This is a fatal message");
}
}
JavaScript (Node.js)
For Node.js applications, you can use the Winston library:
const winston = require('winston');
const logger = winston.createLogger({
level: 'info',
format: winston.format.json(),
defaultMeta: { service: 'user-service' },
transports: [
new winston.transports.File({ filename: 'error.log', level: 'error' }),
new winston.transports.File({ filename: 'combined.log' })
]
});
logger.info('This is an info message');
logger.warn('This is a warning message');
logger.error('This is an error message');
6. Structured Logging
Structured logging is an approach where log entries are recorded in a structured format, typically JSON, instead of plain text. This approach offers several advantages:
- Easier to parse and analyze logs programmatically
- Consistent format across different parts of the application
- Ability to include rich metadata with each log entry
- Better integration with log management and analysis tools
Here’s an example of structured logging in Python using the structlog library:
import structlog
log = structlog.get_logger()
log.info("user logged in",
user_id=user.id,
username=user.username,
login_method="password")
This would produce a log entry like:
{
"event": "user logged in",
"user_id": 123,
"username": "johndoe",
"login_method": "password",
"timestamp": "2023-06-15T10:30:45Z",
"level": "info"
}
7. Log Management and Analysis
As your application grows, managing and analyzing logs becomes increasingly important. Here are some strategies and tools to consider:
Centralized Logging
For distributed systems or microservices architectures, centralized logging is crucial. It involves collecting logs from multiple sources into a single system for easier management and analysis. Popular centralized logging solutions include:
- ELK Stack (Elasticsearch, Logstash, Kibana)
- Splunk
- Graylog
- Papertrail
- Loggly
Log Analysis
Log analysis tools help you make sense of your logs, identify patterns, and extract valuable insights. Some features to look for in log analysis tools include:
- Full-text search
- Real-time alerting
- Visualization and dashboards
- Machine learning-based anomaly detection
- Custom metrics and KPIs
Log Retention and Archiving
Implement a log retention policy that balances storage costs with the need to retain historical data. Consider:
- Rotating logs based on size or time
- Compressing older logs
- Archiving logs to long-term storage (e.g., Amazon S3)
- Implementing a tiered storage strategy
8. Security Considerations in Logging
While logging is essential for application monitoring and debugging, it’s crucial to consider security implications:
Protecting Sensitive Information
- Never log passwords, API keys, or other secrets
- Be cautious with personally identifiable information (PII)
- Use data masking techniques for sensitive fields
- Implement proper access controls for log files and log management systems
Logging for Security Events
Use logging to track security-relevant events:
- Authentication attempts (successful and failed)
- Authorization checks
- Data access and modifications
- System configuration changes
Secure Log Transmission
When transmitting logs over a network:
- Use encrypted connections (e.g., TLS)
- Implement authentication for log ingestion endpoints
- Consider using VPNs or private networks for log transmission
9. Performance Impact of Logging
While logging is crucial, it’s important to be mindful of its performance impact, especially in high-traffic applications:
Asynchronous Logging
Use asynchronous logging to minimize the impact on application performance. This involves writing log messages to a buffer or queue, which is then processed by a separate thread.
Logging Levels in Production
In production environments, consider using higher logging levels (e.g., WARN or ERROR) by default to reduce the volume of logs and improve performance. Implement the ability to dynamically change log levels for troubleshooting when needed.
Sampling
For high-volume events, consider implementing sampling to log only a percentage of occurrences. This can significantly reduce the volume of logs while still providing valuable insights.
Buffering and Batching
Implement buffering and batching of log messages to reduce I/O operations. This is especially important when logging to remote systems.
Monitoring Logging Performance
Regularly monitor the performance impact of your logging implementation. Look for metrics like:
- Logging latency
- CPU and memory usage of logging operations
- Disk I/O for log files
- Network bandwidth used for log transmission
10. Conclusion
Implementing effective logging in your applications is a crucial skill for any developer. It not only aids in debugging and troubleshooting but also provides valuable insights into application behavior, performance, and user interactions. By following best practices, choosing appropriate tools, and considering factors like security and performance, you can create a robust logging system that enhances the overall quality and maintainability of your applications.
Remember, logging is not a one-time setup; it’s an ongoing process that should evolve with your application. Regularly review and refine your logging strategy to ensure it continues to meet your needs as your application grows and changes.
As you progress in your coding journey, whether you’re preparing for technical interviews or building complex applications, effective logging will be an invaluable tool in your developer toolkit. It’s a skill that distinguishes experienced developers and contributes significantly to creating robust, maintainable, and production-ready applications.