How to Implement Internationalization and Localization in Your Software
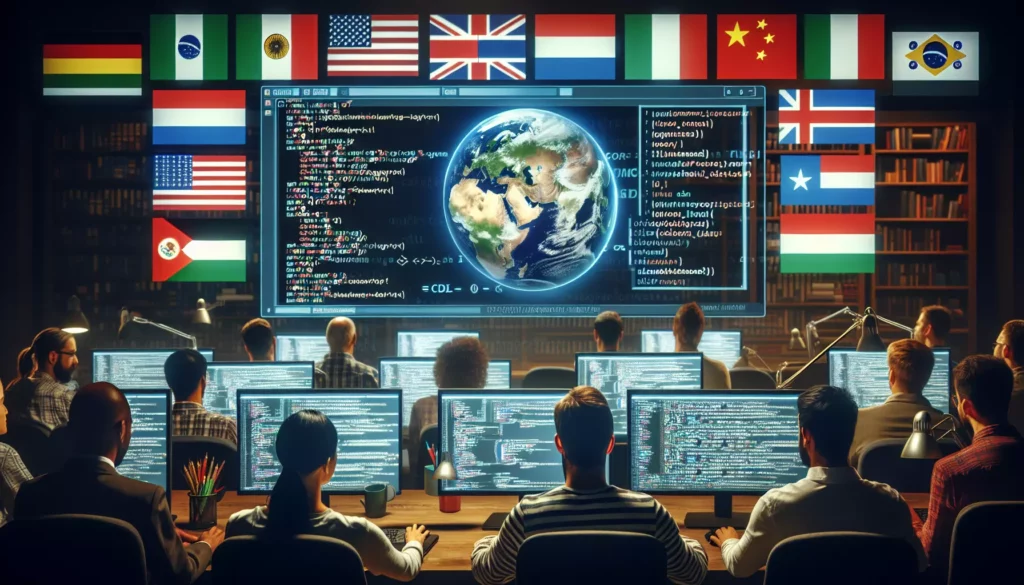
In today’s globalized digital landscape, creating software that can reach and resonate with users worldwide is more important than ever. This is where internationalization (i18n) and localization (l10n) come into play. These processes are crucial for expanding your software’s reach and ensuring a seamless user experience across different cultures and languages. In this comprehensive guide, we’ll explore the concepts of internationalization and localization, their importance, and how to implement them effectively in your software projects.
Table of Contents
- Understanding Internationalization and Localization
- The Importance of i18n and l10n
- Planning for Internationalization and Localization
- Implementing Internationalization
- Implementing Localization
- Best Practices for i18n and l10n
- Testing Internationalized and Localized Software
- Tools and Resources
- Common Challenges and Solutions
- Conclusion
1. Understanding Internationalization and Localization
Before diving into the implementation details, it’s crucial to understand what internationalization and localization mean and how they differ.
Internationalization (i18n)
Internationalization is the process of designing and developing software in a way that makes it capable of adapting to various languages and regions without requiring engineering changes. The term “i18n” is derived from the first and last letters of the word “internationalization,” with 18 representing the number of letters between them.
Key aspects of internationalization include:
- Separating user-facing text from the code
- Supporting multiple character encodings (e.g., UTF-8)
- Handling different date, time, and number formats
- Allowing for text expansion or contraction when translated
- Supporting right-to-left (RTL) languages
Localization (l10n)
Localization is the process of adapting internationalized software for a specific region or language. This involves translating the user interface, adjusting functionality to local requirements, and ensuring cultural appropriateness. The term “l10n” follows the same naming convention as “i18n.”
Key aspects of localization include:
- Translating user interface text
- Adapting graphics and colors to local conventions
- Modifying content to suit the target audience
- Converting to local currencies and units of measurement
- Adhering to local legal requirements
2. The Importance of i18n and l10n
Implementing internationalization and localization in your software offers numerous benefits:
- Expanded market reach: By making your software accessible to users in different countries and languages, you can significantly increase your potential user base and market share.
- Improved user experience: Users are more likely to engage with and appreciate software that speaks their language and respects their cultural norms.
- Competitive advantage: In a global marketplace, offering localized versions of your software can set you apart from competitors who don’t.
- Legal compliance: Some regions have legal requirements for software localization, particularly for government or enterprise applications.
- Easier maintenance: A well-internationalized codebase is often easier to maintain and update across multiple languages and regions.
3. Planning for Internationalization and Localization
Successful implementation of i18n and l10n requires careful planning. Here are some key steps to consider:
- Identify target markets: Determine which countries and languages you want to support based on your business goals and user demographics.
- Assess current codebase: Evaluate your existing software to determine the extent of changes needed for internationalization.
- Choose a localization strategy: Decide whether to localize all content at once or prioritize certain features or languages.
- Select appropriate tools and frameworks: Choose internationalization libraries and localization management tools that suit your technology stack and project needs.
- Establish a localization workflow: Define processes for managing translations, updates, and quality assurance.
- Plan for ongoing maintenance: Consider how you’ll handle updates and new feature releases across all supported languages.
4. Implementing Internationalization
Implementing internationalization involves preparing your codebase to support multiple languages and regions. Here are some key steps and best practices:
Externalize Strings
One of the most crucial steps in internationalization is separating user-facing text from the code. This typically involves using string externalization techniques:
// Instead of:
console.log("Welcome to our app!");
// Use:
console.log(i18n.translate("welcome_message"));
Use Unicode for Character Encoding
Ensure your application uses Unicode (preferably UTF-8) to support a wide range of characters from different languages:
// In HTML:
<meta charset="UTF-8">
// In Python:
# -*- coding: utf-8 -*-
// In Java:
String text = new String(bytes, StandardCharsets.UTF_8);
Handle Date, Time, and Number Formats
Use locale-aware functions for formatting dates, times, and numbers:
// JavaScript example using Intl API
const date = new Date();
const formatter = new Intl.DateTimeFormat('de-DE', {
year: 'numeric',
month: 'long',
day: 'numeric'
});
console.log(formatter.format(date)); // Output: 15. April 2023
Support Text Directionality
Ensure your UI can handle both left-to-right (LTR) and right-to-left (RTL) text:
<html dir="rtl" lang="ar">
<!-- Arabic content here -->
</html>
Use ICU Message Format
The ICU (International Components for Unicode) Message Format is a powerful way to handle complex translations with pluralization and gender:
const messages = {
inbox: '{count, plural, =0 {No messages} one {1 message} other {# messages}}'
};
const formatter = new Intl.MessageFormat(messages.inbox, 'en-US');
console.log(formatter.format({ count: 0 })); // Output: No messages
console.log(formatter.format({ count: 1 })); // Output: 1 message
console.log(formatter.format({ count: 5 })); // Output: 5 messages
5. Implementing Localization
Once your software is internationalized, you can proceed with localization. Here are the key steps:
Prepare Resource Files
Create separate resource files for each supported language. These files typically contain key-value pairs for translations:
// en.json
{
"welcome_message": "Welcome to our app!",
"user_greeting": "Hello, {name}!"
}
// es.json
{
"welcome_message": "¡Bienvenido a nuestra aplicación!",
"user_greeting": "¡Hola, {name}!"
}
Implement Language Selection
Allow users to choose their preferred language, and implement logic to load the appropriate resource file:
function setLanguage(lang) {
// Load language file
fetch(`/lang/${lang}.json`)
.then(response => response.json())
.then(data => {
i18n.translations = data;
updateUI();
});
}
function updateUI() {
document.getElementById('welcome').textContent = i18n.translate("welcome_message");
// Update other UI elements...
}
Adapt UI Layout
Ensure your UI can accommodate varying text lengths and directionality:
.flexible-container {
display: flex;
flex-wrap: wrap;
justify-content: space-between;
}
[dir="rtl"] .flexible-container {
flex-direction: row-reverse;
}
Localize Images and Media
Replace or adapt images, icons, and other media that contain text or culturally specific content:
<img src="/images/{{ language }}/logo.png" alt="{{ i18n.translate('logo_alt_text') }}">
Handle Currency and Measurement Units
Convert currencies and measurement units based on the user’s locale:
function formatCurrency(amount, locale, currency) {
return new Intl.NumberFormat(locale, {
style: 'currency',
currency: currency
}).format(amount);
}
console.log(formatCurrency(1000, 'en-US', 'USD')); // $1,000.00
console.log(formatCurrency(1000, 'de-DE', 'EUR')); // 1.000,00 €
6. Best Practices for i18n and l10n
To ensure successful implementation of internationalization and localization, consider these best practices:
- Start early: Implement i18n from the beginning of your project to avoid costly refactoring later.
- Use meaningful keys: Choose descriptive keys for your translations to provide context for translators.
- Avoid string concatenation: Use placeholders instead of concatenating strings to allow for different word orders in translations.
- Provide context for translators: Include comments or descriptions for strings to ensure accurate translations.
- Use pseudo-localization: Test your internationalized app with pseudo-translations to catch potential issues early.
- Implement fallback mechanisms: Provide fallback translations for missing strings to ensure a smooth user experience.
- Keep translations updated: Regularly update and synchronize translations across all supported languages.
- Consider cultural sensitivities: Be aware of cultural differences and adapt content accordingly.
7. Testing Internationalized and Localized Software
Thorough testing is crucial to ensure your internationalized and localized software functions correctly. Consider the following testing approaches:
Automated Testing
Implement automated tests to check for internationalization issues:
describe('Internationalization', () => {
it('should display the correct welcome message', () => {
setLanguage('es');
expect(getWelcomeMessage()).toBe('¡Bienvenido a nuestra aplicación!');
setLanguage('en');
expect(getWelcomeMessage()).toBe('Welcome to our app!');
});
});
describe('Date Formatting', () => {
it('should format dates correctly for different locales', () => {
const date = new Date('2023-04-15');
expect(formatDate(date, 'en-US')).toBe('4/15/2023');
expect(formatDate(date, 'de-DE')).toBe('15.4.2023');
});
});
Manual Testing
Perform manual testing to catch issues that automated tests might miss:
- Test UI layout in different languages, especially those with longer or shorter translations
- Verify RTL layout for languages like Arabic and Hebrew
- Check cultural appropriateness of images and icons
- Test currency conversions and measurement unit displays
- Verify date, time, and number formats across different locales
Localization Testing
Engage native speakers or professional translators to review localized versions:
- Check for grammatical and spelling errors
- Ensure translations are contextually appropriate
- Verify that idiomatic expressions are correctly translated
- Check for consistency in terminology across the application
8. Tools and Resources
Several tools and libraries can help streamline the internationalization and localization process:
Internationalization Libraries
- React-Intl: For React applications
- i18next: A popular JavaScript internationalization framework
- Angular i18n: Built-in internationalization support for Angular
- Django Internationalization: For Python Django projects
- Rails I18n: Internationalization support for Ruby on Rails
Localization Management Platforms
- Crowdin: Collaborative translation management platform
- Lokalise: Translation management system with integrations for various platforms
- Transifex: Localization platform with support for multiple file formats
- POEditor: Web-based localization management tool
Testing Tools
- Pseudo Localization Tools: To test internationalization without actual translations
- BrowserStack: For testing on different devices and browsers
- Applanga: In-context translation and testing tool
9. Common Challenges and Solutions
Implementing internationalization and localization can present various challenges. Here are some common issues and their solutions:
Challenge: Text Expansion
Solution: Design flexible layouts that can accommodate text expansion. Use CSS techniques like flexbox or grid to create responsive designs. Implement text truncation or dynamic font sizing for critical UI elements.
Challenge: Maintaining Context for Translators
Solution: Provide detailed comments and descriptions for translatable strings. Use translation management systems that allow you to add context and screenshots for each string.
// Provide context for translators
i18n.translate('button_label', 'Label for the submit button on the contact form')
Challenge: Handling Plurals
Solution: Use ICU message format or similar systems that support plural rules for different languages.
const messages = {
items: '{count, plural, =0 {No items} one {1 item} other {# items}}'
};
const formatter = new Intl.MessageFormat(messages.items, 'en-US');
console.log(formatter.format({ count: 0 })); // No items
console.log(formatter.format({ count: 1 })); // 1 item
console.log(formatter.format({ count: 5 })); // 5 items
Challenge: Right-to-Left (RTL) Layout
Solution: Use CSS logical properties and values, and test thoroughly with RTL languages. Consider using RTL-specific stylesheets or CSS frameworks that support RTL layouts.
/* Use logical properties for RTL support */
.container {
margin-inline-start: 10px;
padding-inline-end: 20px;
}
/* RTL-specific styles */
[dir="rtl"] .icon {
transform: scaleX(-1);
}
Challenge: Date and Time Formatting
Solution: Use locale-aware date and time formatting libraries or built-in language features. Avoid hardcoding date formats.
const date = new Date('2023-04-15T15:30:00Z');
// US English uses month-day-year order and 12-hour time
console.log(date.toLocaleString('en-US'));
// Output: 4/15/2023, 11:30:00 AM
// British English uses day-month-year order and 24-hour time
console.log(date.toLocaleString('en-GB'));
// Output: 15/04/2023, 15:30:00
// German uses day.month.year order and 24-hour time
console.log(date.toLocaleString('de-DE'));
// Output: 15.4.2023, 15:30:00
Challenge: Maintaining Translation Consistency
Solution: Use translation memory tools and glossaries to ensure consistency across your application. Implement a review process involving native speakers or professional translators.
Challenge: Performance Impact
Solution: Implement lazy loading for language resources, loading only the required language pack based on the user’s preference. Use efficient data structures for storing and retrieving translations.
async function loadLanguage(lang) {
const response = await fetch(`/lang/${lang}.json`);
const translations = await response.json();
i18n.setTranslations(translations);
updateUI();
}
// Load language on demand
document.getElementById('language-selector').addEventListener('change', (event) => {
loadLanguage(event.target.value);
});
10. Conclusion
Implementing internationalization and localization is a crucial step in creating software that can reach a global audience. By following the best practices and techniques outlined in this guide, you can create a more inclusive and user-friendly application that resonates with users across different languages and cultures.
Remember that i18n and l10n are ongoing processes. As your software evolves, you’ll need to continue updating and refining your internationalization and localization efforts. Regular testing, user feedback, and collaboration with translators and native speakers will help ensure that your software remains accessible and relevant to users worldwide.
By investing in internationalization and localization, you’re not just translating your software; you’re opening doors to new markets, improving user experiences, and creating a truly global product. As the digital world continues to shrink borders, the ability to speak to users in their own language and respect their cultural norms will become increasingly important for software success.
Start implementing these practices in your projects today, and watch as your software’s reach and impact grow across the globe. Happy coding, and may your software speak the language of success in every corner of the world!