How to Implement Caching Mechanisms in Applications
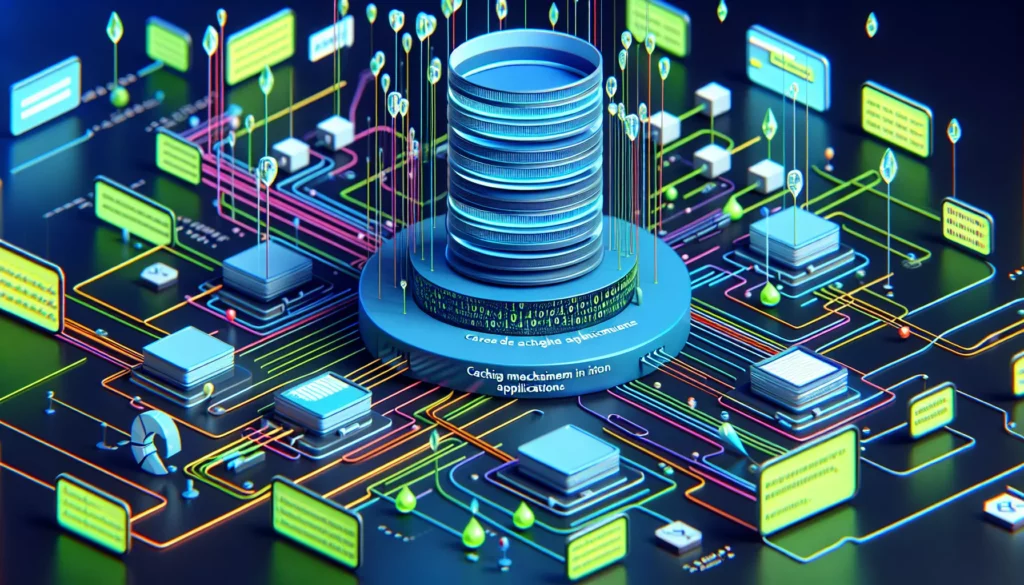
In the world of software development, performance is key. As applications grow in complexity and user bases expand, the need for efficient data retrieval and processing becomes increasingly critical. This is where caching mechanisms come into play. Caching is a technique that stores frequently accessed data in a faster storage medium, allowing subsequent requests for that data to be served more quickly. In this comprehensive guide, we’ll explore various caching mechanisms and how to implement them in your applications.
Table of Contents
- Understanding Caching
- Types of Caching
- In-Memory Caching
- Distributed Caching
- Database Caching
- Content Delivery Network (CDN) Caching
- Browser Caching
- API Response Caching
- Cache Invalidation Strategies
- Best Practices for Implementing Caching
- Conclusion
1. Understanding Caching
Before diving into the implementation details, it’s crucial to understand what caching is and why it’s important. Caching is a technique used to store copies of frequently accessed data in a location that allows for faster retrieval. The primary goals of caching are:
- Reduce latency: By serving data from a cache, you can significantly decrease the time it takes to retrieve information.
- Decrease network traffic: Caching reduces the number of requests made to the original data source, lowering network congestion.
- Improve application performance: Faster data retrieval leads to quicker response times and a better user experience.
- Reduce load on backend systems: By serving requests from the cache, you can alleviate the pressure on your databases and application servers.
2. Types of Caching
There are several types of caching mechanisms, each suited for different scenarios and requirements. Let’s explore the most common types:
- In-Memory Caching
- Distributed Caching
- Database Caching
- Content Delivery Network (CDN) Caching
- Browser Caching
- API Response Caching
We’ll delve into each of these types and provide examples of how to implement them in various programming languages and frameworks.
3. In-Memory Caching
In-memory caching is one of the simplest and most effective forms of caching. It involves storing frequently accessed data in the application’s memory, allowing for extremely fast data retrieval. This type of caching is particularly useful for applications that run on a single server or have a relatively small dataset that can fit in memory.
Implementing In-Memory Caching in Python
Let’s look at a simple example of in-memory caching in Python using a dictionary:
class SimpleCache:
def __init__(self):
self.cache = {}
def get(self, key):
return self.cache.get(key)
def set(self, key, value):
self.cache[key] = value
# Usage
cache = SimpleCache()
cache.set("user_1", {"name": "John Doe", "age": 30})
user = cache.get("user_1")
print(user) # Output: {'name': 'John Doe', 'age': 30}
This simple implementation provides basic caching functionality. However, for more advanced features like expiration and size limits, you might want to use a library like cachetools
:
from cachetools import TTLCache
import time
# Create a cache with a maximum of 100 items and a 10-second time-to-live
cache = TTLCache(maxsize=100, ttl=10)
# Set a value in the cache
cache["key"] = "value"
# Get the value from the cache
print(cache["key"]) # Output: value
# Wait for 11 seconds
time.sleep(11)
# Try to get the expired value
print(cache.get("key")) # Output: None
Implementing In-Memory Caching in Java
For Java applications, you can use libraries like Guava or Caffeine for in-memory caching. Here’s an example using Caffeine:
import com.github.benmanes.caffeine.cache.*;
LoadingCache<String, DataObject> cache = Caffeine.newBuilder()
.maximumSize(100)
.expireAfterWrite(Duration.ofMinutes(5))
.build(key -> getDataFromDatabase(key));
// Usage
DataObject result = cache.get("myKey");
4. Distributed Caching
Distributed caching is essential for applications that run on multiple servers or in a cloud environment. It allows you to share cached data across multiple application instances, ensuring consistency and improving scalability.
Implementing Distributed Caching with Redis
Redis is a popular choice for distributed caching due to its speed and versatility. Here’s an example of how to use Redis for caching in Python:
import redis
import json
class RedisCache:
def __init__(self, host='localhost', port=6379):
self.redis_client = redis.Redis(host=host, port=port)
def get(self, key):
value = self.redis_client.get(key)
if value:
return json.loads(value)
return None
def set(self, key, value, expiration=None):
self.redis_client.set(key, json.dumps(value), ex=expiration)
# Usage
cache = RedisCache()
cache.set("user_1", {"name": "John Doe", "age": 30}, expiration=3600) # Cache for 1 hour
user = cache.get("user_1")
print(user) # Output: {'name': 'John Doe', 'age': 30}
Implementing Distributed Caching in .NET
For .NET applications, you can use the built-in IDistributedCache
interface, which can be backed by various providers like Redis or SQL Server. Here’s an example using Redis:
using Microsoft.Extensions.Caching.Distributed;
using System.Text.Json;
public class CacheService
{
private readonly IDistributedCache _cache;
public CacheService(IDistributedCache cache)
{
_cache = cache;
}
public async Task<T> GetOrSetAsync<T>(string key, Func<Task<T>> getDataFunc, TimeSpan? absoluteExpiration = null)
{
var cachedData = await _cache.GetStringAsync(key);
if (cachedData != null)
{
return JsonSerializer.Deserialize<T>(cachedData);
}
var data = await getDataFunc();
var options = new DistributedCacheEntryOptions();
if (absoluteExpiration.HasValue)
{
options.AbsoluteExpirationRelativeToNow = absoluteExpiration;
}
await _cache.SetStringAsync(key, JsonSerializer.Serialize(data), options);
return data;
}
}
// Usage
var cacheService = new CacheService(distributedCache);
var user = await cacheService.GetOrSetAsync<User>("user_1", async () => await GetUserFromDatabase(1), TimeSpan.FromHours(1));
5. Database Caching
Database caching involves storing frequently accessed database query results in memory to reduce the load on the database and improve response times. This can be implemented at various levels, including the application level, database level, or using a separate caching layer.
Query Result Caching in SQL Server
SQL Server provides a built-in query result cache that can be enabled at the database level. Here’s how you can enable it:
ALTER DATABASE YourDatabaseName
SET MEMORY_OPTIMIZED_ELEVATE_TO_SNAPSHOT = ON;
ALTER DATABASE SCOPED CONFIGURATION
SET QUERY_STORE = ON;
ALTER DATABASE SCOPED CONFIGURATION
SET QUERY_STORE_CAPTURE_MODE = ALL;
ALTER DATABASE SCOPED CONFIGURATION
SET AUTOMATIC_TUNING (QUERY_STORE_QUERY_HINTS = ON);
Once enabled, SQL Server will automatically cache and reuse query results when appropriate.
Implementing Database Caching in Django
Django provides a caching framework that can be used to cache database query results. Here’s an example of how to use it:
from django.core.cache import cache
from django.db import models
class User(models.Model):
name = models.CharField(max_length=100)
email = models.EmailField()
@classmethod
def get_user_by_email(cls, email):
cache_key = f"user_email_{email}"
user = cache.get(cache_key)
if user is None:
try:
user = cls.objects.get(email=email)
cache.set(cache_key, user, timeout=3600) # Cache for 1 hour
except cls.DoesNotExist:
return None
return user
# Usage
user = User.get_user_by_email("john@example.com")
6. Content Delivery Network (CDN) Caching
CDN caching involves storing static assets (like images, CSS, and JavaScript files) on geographically distributed servers to reduce latency for users around the world. While implementing a CDN itself is beyond the scope of most applications, integrating with a CDN service is relatively straightforward.
Implementing CDN Caching with Amazon CloudFront
Here’s an example of how to configure Amazon CloudFront to cache your static assets:
- Create a CloudFront distribution in the AWS Management Console.
- Set your origin (e.g., your S3 bucket or web server).
- Configure caching behaviors:
{ "DefaultCacheBehavior": { "MinTTL": 0, "DefaultTTL": 86400, "MaxTTL": 31536000, "ForwardedValues": { "QueryString": false, "Cookies": { "Forward": "none" } }, "ViewerProtocolPolicy": "redirect-to-https", "Compress": true } }
- Update your application to use the CloudFront URL for static assets.
7. Browser Caching
Browser caching allows web browsers to store static assets locally, reducing the number of requests made to the server and improving page load times for returning visitors.
Implementing Browser Caching with HTTP Headers
You can control browser caching behavior by setting appropriate HTTP headers. Here’s an example of how to set cache headers in an Express.js application:
const express = require('express');
const app = express();
app.use((req, res, next) => {
// Cache static assets for 1 year
if (req.url.match(/^\/static\//)) {
res.setHeader('Cache-Control', 'public, max-age=31536000');
} else {
// For other routes, cache for 1 hour
res.setHeader('Cache-Control', 'public, max-age=3600');
}
next();
});
app.use(express.static('public'));
app.listen(3000, () => {
console.log('Server running on port 3000');
});
8. API Response Caching
Caching API responses can significantly reduce the load on your backend services and improve response times for clients. This is particularly useful for APIs that serve relatively static data or have high read-to-write ratios.
Implementing API Caching in Express.js
Here’s an example of how to implement API response caching using Redis in an Express.js application:
const express = require('express');
const redis = require('redis');
const { promisify } = require('util');
const app = express();
const client = redis.createClient();
const getAsync = promisify(client.get).bind(client);
const setAsync = promisify(client.set).bind(client);
const cacheMiddleware = async (req, res, next) => {
const key = `api-cache:${req.originalUrl}`;
const cachedResponse = await getAsync(key);
if (cachedResponse) {
return res.json(JSON.parse(cachedResponse));
}
res.sendResponse = res.json;
res.json = (body) => {
setAsync(key, JSON.stringify(body), 'EX', 3600); // Cache for 1 hour
res.sendResponse(body);
};
next();
};
app.get('/api/data', cacheMiddleware, (req, res) => {
// Simulate a slow database query
setTimeout(() => {
res.json({ data: 'Some API response' });
}, 2000);
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
9. Cache Invalidation Strategies
Cache invalidation is the process of removing or updating cached data when it becomes stale. Implementing an effective cache invalidation strategy is crucial to ensure that users always see up-to-date information. There are several common cache invalidation strategies:
Time-based Expiration
This is the simplest strategy, where cached items are given a time-to-live (TTL) and automatically expire after a set duration. This approach is easy to implement but may result in serving stale data if not carefully tuned.
Event-based Invalidation
In this strategy, the cache is updated or invalidated when specific events occur, such as data updates or user actions. This approach ensures that the cache always reflects the latest data but requires more complex implementation.
Version-based Invalidation
This strategy involves associating a version number with cached data and incrementing it when the data changes. Clients include the version number in their requests, allowing the server to determine if the cached data is still valid.
Implementing Cache Invalidation
Here’s an example of implementing event-based cache invalidation using Redis in Python:
import redis
import json
class CacheService:
def __init__(self):
self.redis_client = redis.Redis(host='localhost', port=6379)
def get(self, key):
value = self.redis_client.get(key)
return json.loads(value) if value else None
def set(self, key, value, expiration=None):
self.redis_client.set(key, json.dumps(value), ex=expiration)
def invalidate(self, key):
self.redis_client.delete(key)
class UserService:
def __init__(self, cache_service):
self.cache_service = cache_service
def get_user(self, user_id):
cache_key = f"user:{user_id}"
user = self.cache_service.get(cache_key)
if user is None:
user = self.get_user_from_database(user_id)
self.cache_service.set(cache_key, user, expiration=3600)
return user
def update_user(self, user_id, new_data):
self.update_user_in_database(user_id, new_data)
cache_key = f"user:{user_id}"
self.cache_service.invalidate(cache_key)
def get_user_from_database(self, user_id):
# Simulate database query
return {"id": user_id, "name": "John Doe", "email": "john@example.com"}
def update_user_in_database(self, user_id, new_data):
# Simulate database update
pass
# Usage
cache_service = CacheService()
user_service = UserService(cache_service)
user = user_service.get_user(1)
print(user) # Fetched from database and cached
user = user_service.get_user(1)
print(user) # Fetched from cache
user_service.update_user(1, {"name": "Jane Doe"})
user = user_service.get_user(1)
print(user) # Fetched from database (cache was invalidated) and cached again
10. Best Practices for Implementing Caching
When implementing caching mechanisms in your applications, consider the following best practices:
- Choose the right caching strategy: Select a caching mechanism that best fits your application’s needs, considering factors like data volatility, consistency requirements, and scalability.
- Set appropriate expiration times: Balance between keeping data fresh and reducing load on your backend systems. Adjust TTL values based on how frequently your data changes.
- Use cache keys wisely: Design a consistent and meaningful key naming convention to avoid conflicts and improve cache hit rates.
- Implement cache warming: Preload frequently accessed data into the cache to improve initial response times.
- Monitor cache performance: Keep track of cache hit rates, miss rates, and eviction rates to optimize your caching strategy.
- Handle cache failures gracefully: Implement fallback mechanisms in case the cache becomes unavailable.
- Use cache stampede prevention: Implement techniques like cache locks or sliding window algorithms to prevent multiple simultaneous requests from overwhelming your backend when cache entries expire.
- Consider cache size limits: Set appropriate size limits for your cache to prevent memory issues, especially for in-memory caches.
- Implement proper error handling: Handle cache-related errors and exceptions to ensure your application remains stable.
- Keep security in mind: Avoid caching sensitive data, and if necessary, implement encryption for cached data.
11. Conclusion
Implementing caching mechanisms in your applications can significantly improve performance, reduce latency, and enhance the overall user experience. By understanding the various types of caching and following best practices, you can effectively leverage caching to scale your applications and handle increased load.
Remember that caching is not a one-size-fits-all solution, and the best caching strategy for your application will depend on your specific requirements and constraints. Continuously monitor and optimize your caching implementation to ensure it remains effective as your application evolves.
As you continue to develop your coding skills and prepare for technical interviews, consider how caching can be applied to solve performance-related problems. Understanding caching mechanisms and their implementation details can be a valuable asset when discussing system design and optimization strategies during interviews with major tech companies.