How to Implement Basic Networking Concepts in Code
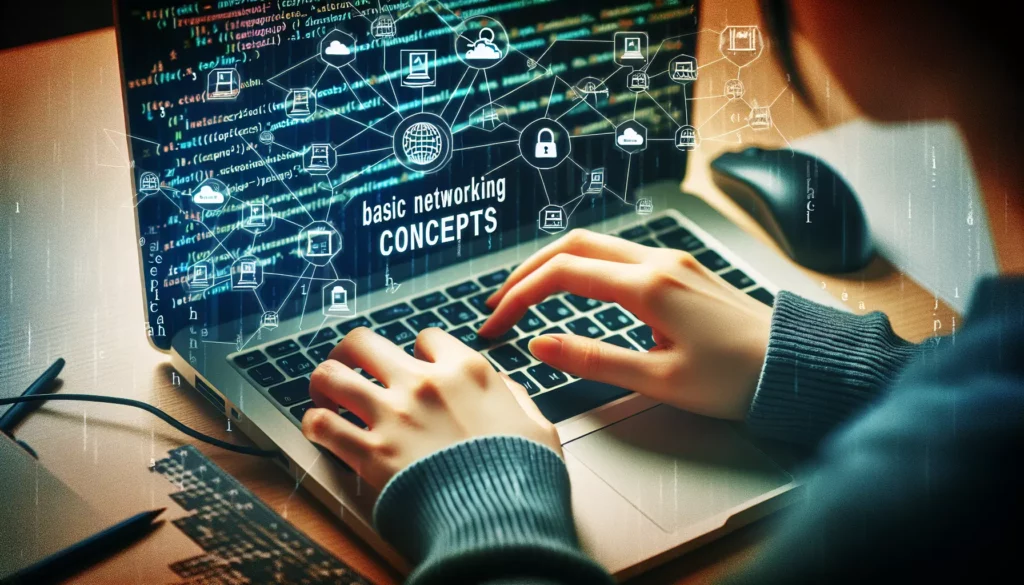
Networking is a fundamental aspect of modern computing, enabling communication between devices and systems across the globe. As a programmer, understanding and implementing basic networking concepts is crucial for developing robust and interconnected applications. In this comprehensive guide, we’ll explore how to implement essential networking concepts in code, providing you with practical examples and explanations along the way.
1. Understanding the OSI Model
Before diving into code implementation, it’s important to have a solid grasp of the OSI (Open Systems Interconnection) model. This conceptual framework describes how data is transmitted between two points in a network. The OSI model consists of seven layers:
- Physical Layer
- Data Link Layer
- Network Layer
- Transport Layer
- Session Layer
- Presentation Layer
- Application Layer
As we implement networking concepts in code, we’ll primarily focus on the higher layers, particularly the Transport and Application layers.
2. Socket Programming
Sockets are the foundation of network programming, providing a way for applications to communicate over a network. Let’s implement a simple client-server application using Python to demonstrate socket programming.
Server-side implementation:
import socket
def start_server():
host = '127.0.0.1' # localhost
port = 12345
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_socket.bind((host, port))
server_socket.listen(1)
print(f"Server listening on {host}:{port}")
while True:
client_socket, address = server_socket.accept()
print(f"Connection from {address} has been established!")
client_socket.send(bytes("Welcome to the server!", "utf-8"))
client_socket.close()
if __name__ == "__main__":
start_server()
Client-side implementation:
import socket
def connect_to_server():
host = '127.0.0.1' # localhost
port = 12345
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
client_socket.connect((host, port))
message = client_socket.recv(1024)
print(message.decode("utf-8"))
client_socket.close()
if __name__ == "__main__":
connect_to_server()
In this example, we’ve created a simple server that listens for incoming connections and sends a welcome message to clients. The client connects to the server and receives the message.
3. HTTP Requests and Responses
HTTP (Hypertext Transfer Protocol) is the foundation of data communication on the World Wide Web. Let’s implement a basic HTTP client and server using Python.
HTTP Server implementation:
from http.server import HTTPServer, BaseHTTPRequestHandler
class SimpleHTTPRequestHandler(BaseHTTPRequestHandler):
def do_GET(self):
self.send_response(200)
self.send_header('Content-type', 'text/html')
self.end_headers()
self.wfile.write(b"<html><body><h1>Hello, World!</h1></body></html>")
def run_server(port=8000):
server_address = ('', port)
httpd = HTTPServer(server_address, SimpleHTTPRequestHandler)
print(f"Server running on port {port}")
httpd.serve_forever()
if __name__ == '__main__':
run_server()
HTTP Client implementation:
import requests
def make_http_request(url):
response = requests.get(url)
print(f"Status Code: {response.status_code}")
print(f"Content: {response.text}")
if __name__ == '__main__':
make_http_request('http://localhost:8000')
This example demonstrates a simple HTTP server that responds to GET requests with a “Hello, World!” message, and a client that sends a GET request to the server and prints the response.
4. Working with APIs
APIs (Application Programming Interfaces) are crucial for allowing different software systems to communicate with each other. Let’s implement a simple example of working with a RESTful API using Python and the requests library.
import requests
import json
def get_user_data(user_id):
url = f"https://jsonplaceholder.typicode.com/users/{user_id}"
response = requests.get(url)
if response.status_code == 200:
user_data = json.loads(response.text)
print(f"User: {user_data['name']}")
print(f"Email: {user_data['email']}")
print(f"Company: {user_data['company']['name']}")
else:
print(f"Error: Unable to fetch user data. Status code: {response.status_code}")
if __name__ == '__main__':
get_user_data(1)
This example demonstrates how to make a GET request to a RESTful API, parse the JSON response, and extract relevant information.
5. Implementing a Basic Chat Application
Let’s combine our knowledge of socket programming and create a simple chat application that allows multiple clients to communicate with each other through a central server.
Chat Server implementation:
import socket
import threading
class ChatServer:
def __init__(self, host='127.0.0.1', port=12345):
self.host = host
self.port = port
self.server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
self.clients = []
def start(self):
self.server_socket.bind((self.host, self.port))
self.server_socket.listen(5)
print(f"Chat server started on {self.host}:{self.port}")
while True:
client_socket, address = self.server_socket.accept()
print(f"New connection from {address}")
self.clients.append(client_socket)
client_thread = threading.Thread(target=self.handle_client, args=(client_socket,))
client_thread.start()
def handle_client(self, client_socket):
while True:
try:
message = client_socket.recv(1024).decode('utf-8')
if message:
print(f"Received: {message}")
self.broadcast(message, client_socket)
else:
self.remove_client(client_socket)
break
except:
self.remove_client(client_socket)
break
def broadcast(self, message, sender_socket):
for client in self.clients:
if client != sender_socket:
try:
client.send(message.encode('utf-8'))
except:
self.remove_client(client)
def remove_client(self, client_socket):
if client_socket in self.clients:
self.clients.remove(client_socket)
client_socket.close()
if __name__ == '__main__':
server = ChatServer()
server.start()
Chat Client implementation:
import socket
import threading
class ChatClient:
def __init__(self, host='127.0.0.1', port=12345):
self.host = host
self.port = port
self.client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
def connect(self):
self.client_socket.connect((self.host, self.port))
receive_thread = threading.Thread(target=self.receive_messages)
receive_thread.start()
while True:
message = input()
self.client_socket.send(message.encode('utf-8'))
def receive_messages(self):
while True:
try:
message = self.client_socket.recv(1024).decode('utf-8')
print(message)
except:
print("An error occurred!")
self.client_socket.close()
break
if __name__ == '__main__':
client = ChatClient()
client.connect()
This chat application demonstrates how to implement a multi-client chat system using socket programming and threading. The server manages multiple client connections and broadcasts messages to all connected clients, while each client can send and receive messages concurrently.
6. Understanding and Implementing TCP vs UDP
TCP (Transmission Control Protocol) and UDP (User Datagram Protocol) are two of the most common transport layer protocols. Let’s implement simple examples of both to understand their differences.
TCP Server and Client:
import socket
# TCP Server
def tcp_server():
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_socket.bind(('localhost', 12345))
server_socket.listen(1)
print("TCP Server is waiting for a connection...")
client_socket, address = server_socket.accept()
print(f"Connected to {address}")
data = client_socket.recv(1024).decode()
print(f"Received: {data}")
client_socket.send("Message received".encode())
client_socket.close()
server_socket.close()
# TCP Client
def tcp_client():
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
client_socket.connect(('localhost', 12345))
client_socket.send("Hello, TCP Server!".encode())
response = client_socket.recv(1024).decode()
print(f"Server response: {response}")
client_socket.close()
# Run these functions in separate terminal windows
# tcp_server()
# tcp_client()
UDP Server and Client:
import socket
# UDP Server
def udp_server():
server_socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
server_socket.bind(('localhost', 12346))
print("UDP Server is waiting for a message...")
data, address = server_socket.recvfrom(1024)
print(f"Received: {data.decode()} from {address}")
server_socket.sendto("Message received".encode(), address)
server_socket.close()
# UDP Client
def udp_client():
client_socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
client_socket.sendto("Hello, UDP Server!".encode(), ('localhost', 12346))
response, _ = client_socket.recvfrom(1024)
print(f"Server response: {response.decode()}")
client_socket.close()
# Run these functions in separate terminal windows
# udp_server()
# udp_client()
The key differences between TCP and UDP are:
- TCP is connection-oriented, while UDP is connectionless.
- TCP ensures reliable, ordered delivery of data, while UDP does not guarantee delivery or order.
- TCP has flow control and congestion control mechanisms, while UDP does not.
- UDP is generally faster and more efficient for applications that can tolerate some data loss, such as video streaming or online gaming.
7. Implementing a Simple Load Balancer
Load balancing is a crucial concept in networking, especially for distributing traffic across multiple servers. Let’s implement a basic round-robin load balancer using Python.
import socket
import threading
import time
class SimpleLoadBalancer:
def __init__(self, backends, host='localhost', port=8000):
self.backends = backends
self.host = host
self.port = port
self.server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
self.current_backend = 0
def start(self):
self.server_socket.bind((self.host, self.port))
self.server_socket.listen(5)
print(f"Load Balancer started on {self.host}:{self.port}")
while True:
client_socket, address = self.server_socket.accept()
print(f"New connection from {address}")
client_thread = threading.Thread(target=self.handle_client, args=(client_socket,))
client_thread.start()
def handle_client(self, client_socket):
backend = self.get_next_backend()
print(f"Forwarding request to backend: {backend}")
backend_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
backend_socket.connect(backend)
client_socket.setblocking(False)
backend_socket.setblocking(False)
while True:
try:
data = client_socket.recv(4096)
if data:
backend_socket.send(data)
else:
break
except socket.error:
pass
try:
resp = backend_socket.recv(4096)
if resp:
client_socket.send(resp)
else:
break
except socket.error:
pass
client_socket.close()
backend_socket.close()
def get_next_backend(self):
backend = self.backends[self.current_backend]
self.current_backend = (self.current_backend + 1) % len(self.backends)
return backend
if __name__ == '__main__':
backends = [
('localhost', 8001),
('localhost', 8002),
('localhost', 8003)
]
load_balancer = SimpleLoadBalancer(backends)
load_balancer.start()
This simple load balancer distributes incoming connections across multiple backend servers in a round-robin fashion. To test this, you would need to set up multiple backend servers listening on the specified ports.
8. Implementing Basic Network Security
Network security is a critical aspect of any networked application. Let’s implement a simple example of using SSL/TLS to secure our socket communication.
import socket
import ssl
def ssl_client():
hostname = 'www.python.org'
context = ssl.create_default_context()
with socket.create_connection((hostname, 443)) as sock:
with context.wrap_socket(sock, server_hostname=hostname) as secure_sock:
print(f"Connected to {hostname}")
print(f"Cipher used: {secure_sock.cipher()}")
print(f"Certificate: {secure_sock.getpeercert()}")
secure_sock.send(b"GET / HTTP/1.1\r\nHost: www.python.org\r\n\r\n")
response = secure_sock.recv(1024)
print(f"Response: {response.decode()}")
if __name__ == '__main__':
ssl_client()
This example demonstrates how to create a secure SSL/TLS connection to a website, which is essential for protecting sensitive data during transmission.
Conclusion
In this comprehensive guide, we’ve explored how to implement various basic networking concepts in code. We’ve covered socket programming, HTTP communication, API interaction, chat applications, TCP vs UDP, load balancing, and basic network security. These fundamental concepts form the backbone of network programming and are essential for developing robust, scalable, and secure networked applications.
As you continue your journey in network programming, remember that these examples are just the beginning. There’s a wealth of advanced topics to explore, such as asynchronous networking, WebSockets, peer-to-peer communication, and more complex security protocols. Keep practicing, experimenting, and building upon these concepts to enhance your skills and create more sophisticated networked applications.
Remember, the field of networking is vast and constantly evolving. Stay curious, keep learning, and don’t hesitate to dive deeper into areas that interest you. Happy coding!