How to Identify When a Hash Table Will Optimize Your Solution
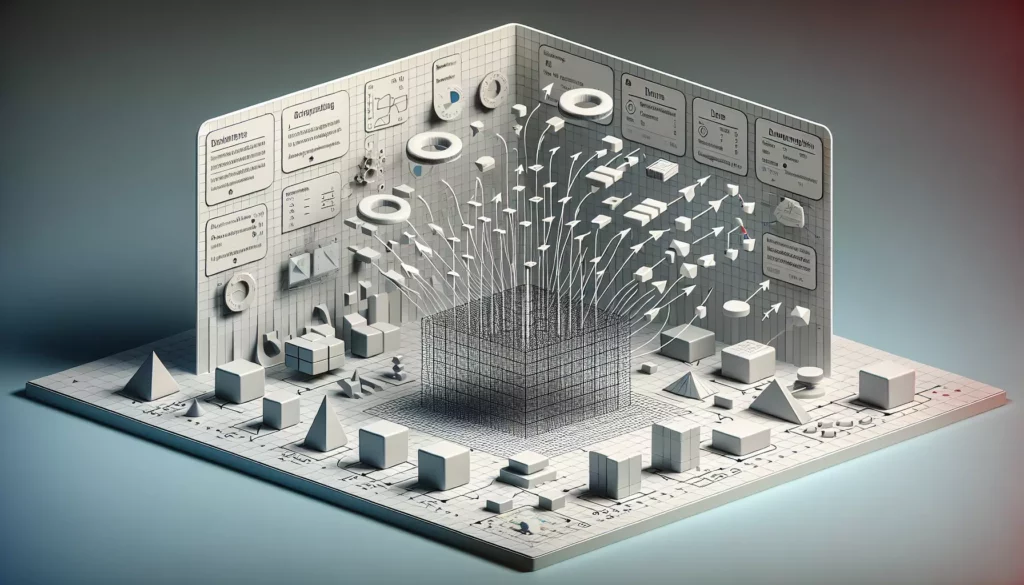
In the world of coding and algorithm design, efficiency is key. As you progress from beginner-level coding to preparing for technical interviews at major tech companies, understanding when and how to use data structures like hash tables can significantly improve your problem-solving skills. This article will guide you through the process of identifying situations where a hash table can optimize your solution, providing you with a powerful tool in your algorithmic toolkit.
Understanding Hash Tables
Before we dive into identifying when to use hash tables, let’s briefly review what they are and how they work.
A hash table, also known as a hash map, is a data structure that implements an associative array abstract data type, a structure that can map keys to values. It uses a hash function to compute an index into an array of buckets or slots, from which the desired value can be found.
The primary operations of a hash table are:
- Insert: Add a new key-value pair to the hash table
- Delete: Remove a key-value pair from the hash table
- Search: Look up the value associated with a particular key
The main advantage of hash tables is their efficiency: on average, the time complexity for insert, delete, and search operations is O(1), or constant time. This makes hash tables extremely fast for these operations, especially when compared to other data structures like arrays or linked lists, which may require O(n) time for similar operations.
Identifying Hash Table Opportunities
Now that we’ve refreshed our understanding of hash tables, let’s explore how to identify situations where they can optimize your solution. Here are some key indicators:
1. Frequent Lookups
If your algorithm requires frequent lookups of values based on keys, a hash table might be the perfect solution. This is especially true when you have a large dataset and need to perform many lookups quickly.
Example scenario: You’re implementing a spell checker that needs to quickly verify if a word exists in a dictionary.
def is_word_in_dictionary(word, dictionary):
return word in dictionary
# Usage
dictionary = set(["apple", "banana", "cherry", ...]) # Using a set (hash table) for O(1) lookups
print(is_word_in_dictionary("apple", dictionary)) # True
print(is_word_in_dictionary("grape", dictionary)) # False
2. Duplicate Detection
When you need to identify duplicates in a dataset quickly, hash tables are an excellent choice. They allow you to check for the existence of an element in constant time.
Example scenario: Finding the first recurring character in a string.
def first_recurring_char(s):
char_set = set()
for char in s:
if char in char_set:
return char
char_set.add(char)
return None
# Usage
print(first_recurring_char("ABCDEBC")) # B
3. Counting Occurrences
Hash tables are ideal for counting the occurrences of elements in a dataset. This is particularly useful in problems involving frequency analysis.
Example scenario: Counting the frequency of words in a text.
from collections import Counter
def word_frequency(text):
words = text.split()
return Counter(words)
# Usage
text = "the quick brown fox jumps over the lazy dog"
print(word_frequency(text))
# Counter({'the': 2, 'quick': 1, 'brown': 1, 'fox': 1, 'jumps': 1, 'over': 1, 'lazy': 1, 'dog': 1})
4. Caching
Hash tables are excellent for implementing caches. If you find yourself repeatedly computing the same values, storing them in a hash table can significantly speed up your algorithm.
Example scenario: Implementing memoization in a recursive Fibonacci function.
def fibonacci(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci(n-1, memo) + fibonacci(n-2, memo)
return memo[n]
# Usage
print(fibonacci(100)) # 354224848179261915075
5. Two-Sum Type Problems
Problems that involve finding pairs of elements that satisfy certain conditions often benefit from using hash tables.
Example scenario: Finding two numbers in an array that add up to a target sum.
def two_sum(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return []
# Usage
print(two_sum([2, 7, 11, 15], 9)) # [0, 1]
6. Grouping or Categorizing Data
When you need to group or categorize data based on certain properties, hash tables can be very useful.
Example scenario: Grouping anagrams from a list of words.
from collections import defaultdict
def group_anagrams(words):
anagram_groups = defaultdict(list)
for word in words:
sorted_word = ''.join(sorted(word))
anagram_groups[sorted_word].append(word)
return list(anagram_groups.values())
# Usage
words = ["eat", "tea", "tan", "ate", "nat", "bat"]
print(group_anagrams(words))
# [['eat', 'tea', 'ate'], ['tan', 'nat'], ['bat']]
When Not to Use Hash Tables
While hash tables are powerful, they’re not always the best choice. Here are some situations where you might want to consider other data structures:
- Ordered data: If you need to maintain the order of elements, a hash table might not be suitable. Consider using an ordered dict or a tree-based structure instead.
- Memory constraints: Hash tables can consume more memory than other data structures. If memory is a concern, you might need to explore alternatives.
- Range queries: If you need to perform range queries (e.g., finding all elements between two values), hash tables are not efficient. A balanced binary search tree might be a better choice.
- Small datasets: For very small datasets, the overhead of a hash table might not be worth it. A simple list or array might suffice.
Implementing Hash Tables in Different Languages
Different programming languages have different implementations of hash tables. Here’s a quick overview of how to use hash tables in some popular languages:
Python
In Python, you can use dictionaries or the collections.defaultdict
class:
# Dictionary
my_dict = {"key1": "value1", "key2": "value2"}
# defaultdict
from collections import defaultdict
my_defaultdict = defaultdict(int)
my_defaultdict["key"] += 1 # No KeyError if key doesn't exist
Java
Java provides the HashMap class:
import java.util.HashMap;
HashMap<String, Integer> map = new HashMap<>();
map.put("key", 1);
int value = map.get("key"); // 1
JavaScript
In JavaScript, you can use objects or the Map class:
// Object
let obj = {key: "value"};
// Map
let map = new Map();
map.set("key", "value");
console.log(map.get("key")); // "value"
C++
C++ provides the unordered_map class:
#include <unordered_map>
std::unordered_map<std::string, int> myMap;
myMap["key"] = 1;
int value = myMap["key"]; // 1
Advanced Hash Table Techniques
As you become more comfortable with hash tables, you can explore more advanced techniques:
1. Custom Hash Functions
For complex objects or specific requirements, you might need to implement your own hash function. A good hash function should distribute keys uniformly across the hash table to minimize collisions.
2. Collision Resolution
There are various methods to handle collisions in hash tables, including:
- Chaining: Each bucket contains a linked list of elements
- Open addressing: Finding the next open slot in the array
Understanding these methods can help you optimize your hash table implementation for specific use cases.
3. Load Factor and Rehashing
The load factor of a hash table is the ratio of the number of stored elements to the table size. When the load factor exceeds a certain threshold, many implementations automatically increase the size of the table and rehash all elements. Understanding this process can help you optimize performance.
Hash Tables in Technical Interviews
Hash tables are a favorite topic in technical interviews, especially at major tech companies. Here are some tips for using hash tables effectively in interview situations:
- Recognize the patterns: Look for problems involving lookups, duplicates, or pair finding. These often hint at hash table solutions.
- Communicate your thought process: Explain why you’re choosing a hash table and discuss the trade-offs.
- Consider edge cases: Think about how your solution handles empty inputs, large inputs, or inputs with many duplicates.
- Analyze time and space complexity: Be prepared to discuss the efficiency of your solution in terms of both time and space.
- Practice, practice, practice: Solve many problems using hash tables to build your intuition for when they’re the right choice.
Conclusion
Hash tables are a powerful tool in any programmer’s arsenal. By understanding when and how to use them, you can significantly optimize many algorithms and solve complex problems more efficiently. As you continue your journey in coding education and prepare for technical interviews, keep an eye out for opportunities to apply hash tables in your solutions.
Remember, the key to mastering hash tables (and indeed, any programming concept) is practice. Challenge yourself with diverse problems, experiment with different implementations, and always strive to understand the underlying principles. With time and effort, identifying when a hash table will optimize your solution will become second nature, propelling you towards success in your coding journey and future technical interviews.