How to Handle User Input Safely and Effectively: A Comprehensive Guide
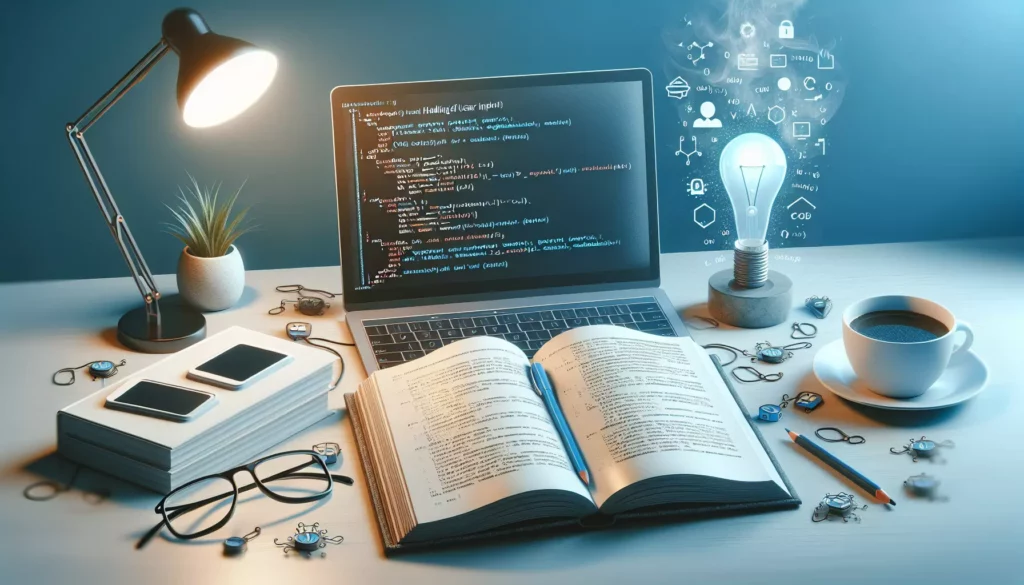
In the world of programming, handling user input is a fundamental skill that every developer must master. Whether you’re building a simple command-line application or a complex web service, the ability to process and validate user input safely and effectively is crucial. This skill is not only essential for creating user-friendly applications but also for maintaining the security and integrity of your systems. In this comprehensive guide, we’ll explore various techniques and best practices for handling user input, with a focus on safety, efficiency, and robustness.
1. Understanding the Importance of User Input Handling
Before diving into the specifics of how to handle user input, it’s crucial to understand why this topic is so important in software development:
- Security: Improper handling of user input can lead to vulnerabilities such as SQL injection, cross-site scripting (XSS), and buffer overflow attacks.
- Data Integrity: Ensuring that the data entered by users is in the correct format and within acceptable ranges helps maintain the integrity of your application’s data.
- User Experience: Proper input handling, including clear error messages and intuitive validation, contributes to a better user experience.
- Application Stability: Robust input handling prevents unexpected crashes and errors that could result from invalid or malicious input.
2. Input Validation Techniques
Input validation is the process of ensuring that the data provided by users meets the expected criteria. Here are some key techniques for input validation:
2.1. Type Checking
Always verify that the input is of the expected data type. For example, if you’re expecting a number, make sure the input can be converted to a numeric type without errors.
def get_age():
while True:
try:
age = int(input("Enter your age: "))
if age < 0 or age > 150:
print("Please enter a valid age between 0 and 150.")
else:
return age
except ValueError:
print("Invalid input. Please enter a number.")
2.2. Range Checking
For numeric inputs, ensure that the value falls within an acceptable range.
def get_percentage():
while True:
try:
percentage = float(input("Enter a percentage (0-100): "))
if 0 <= percentage <= 100:
return percentage
else:
print("Percentage must be between 0 and 100.")
except ValueError:
print("Invalid input. Please enter a number.")
2.3. Length Validation
For string inputs, check that the length is within acceptable limits.
def get_username():
while True:
username = input("Enter a username (3-20 characters): ")
if 3 <= len(username) <= 20:
return username
else:
print("Username must be between 3 and 20 characters long.")
2.4. Pattern Matching
Use regular expressions to validate input that should conform to a specific pattern, such as email addresses or phone numbers.
import re
def get_email():
email_pattern = r'^[\w\.-]+@[\w\.-]+\.\w+$'
while True:
email = input("Enter your email address: ")
if re.match(email_pattern, email):
return email
else:
print("Invalid email format. Please try again.")
3. Sanitizing User Input
Input sanitization is the process of cleaning user input to remove or escape potentially harmful content. This is particularly important when dealing with data that will be displayed on web pages or stored in databases.
3.1. HTML Escaping
When displaying user input on web pages, always escape HTML special characters to prevent XSS attacks.
import html
def sanitize_html(input_string):
return html.escape(input_string)
3.2. SQL Injection Prevention
When working with databases, use parameterized queries or prepared statements to prevent SQL injection attacks.
import sqlite3
def safe_insert(name, age):
conn = sqlite3.connect('users.db')
cursor = conn.cursor()
cursor.execute("INSERT INTO users (name, age) VALUES (?, ?)", (name, age))
conn.commit()
conn.close()
3.3. Stripping Unwanted Characters
Remove or replace characters that are not allowed in certain contexts.
def sanitize_filename(filename):
return ''.join(char for char in filename if char.isalnum() or char in ('_', '-', '.'))
4. Handling Different Types of User Input
Different applications require different types of user input. Let’s explore how to handle various common input types:
4.1. Command-Line Arguments
When building command-line tools, it’s important to properly parse and validate command-line arguments.
import argparse
def parse_arguments():
parser = argparse.ArgumentParser(description='Example script with command-line arguments')
parser.add_argument('--name', type=str, required=True, help='User name')
parser.add_argument('--age', type=int, required=True, help='User age')
return parser.parse_args()
args = parse_arguments()
print(f"Name: {args.name}, Age: {args.age}")
4.2. File Input
When reading data from files, always handle potential errors and validate the file contents.
def read_user_file(filename):
try:
with open(filename, 'r') as file:
data = file.read().strip()
if not data:
raise ValueError("File is empty")
return data
except FileNotFoundError:
print(f"Error: File '{filename}' not found.")
except ValueError as e:
print(f"Error: {str(e)}")
except Exception as e:
print(f"An unexpected error occurred: {str(e)}")
4.3. GUI Input
For graphical user interfaces, use appropriate widgets and validation techniques to ensure correct input.
import tkinter as tk
from tkinter import messagebox
def validate_input():
try:
age = int(age_entry.get())
if age < 0 or age > 150:
raise ValueError("Age must be between 0 and 150")
messagebox.showinfo("Success", f"Age {age} is valid!")
except ValueError as e:
messagebox.showerror("Error", str(e))
root = tk.Tk()
age_entry = tk.Entry(root)
age_entry.pack()
submit_button = tk.Button(root, text="Submit", command=validate_input)
submit_button.pack()
root.mainloop()
5. Error Handling and User Feedback
Proper error handling and clear user feedback are crucial for a good user experience. Here are some best practices:
5.1. Use Try-Except Blocks
Wrap potentially error-prone code in try-except blocks to catch and handle exceptions gracefully.
def divide_numbers():
try:
num1 = float(input("Enter the first number: "))
num2 = float(input("Enter the second number: "))
result = num1 / num2
print(f"Result: {result}")
except ValueError:
print("Error: Please enter valid numbers.")
except ZeroDivisionError:
print("Error: Cannot divide by zero.")
except Exception as e:
print(f"An unexpected error occurred: {str(e)}")
5.2. Provide Clear Error Messages
Error messages should be informative and guide the user on how to correct the input.
def get_positive_number():
while True:
try:
num = float(input("Enter a positive number: "))
if num <= 0:
raise ValueError("The number must be greater than zero.")
return num
except ValueError as e:
print(f"Error: {str(e)}. Please try again.")
5.3. Implement Input Retry Logic
Allow users to retry input when they make mistakes, rather than terminating the program.
def get_valid_input(prompt, validator):
while True:
user_input = input(prompt)
try:
validated_input = validator(user_input)
return validated_input
except ValueError as e:
print(f"Error: {str(e)}. Please try again.")
def validate_age(age_str):
age = int(age_str)
if age < 0 or age > 150:
raise ValueError("Age must be between 0 and 150")
return age
age = get_valid_input("Enter your age: ", validate_age)
print(f"Valid age entered: {age}")
6. Advanced Input Handling Techniques
As you become more proficient in handling user input, you may encounter more complex scenarios that require advanced techniques:
6.1. Input Throttling
For applications that accept frequent user input, implement throttling to prevent abuse or overload.
import time
class InputThrottler:
def __init__(self, max_calls, time_frame):
self.max_calls = max_calls
self.time_frame = time_frame
self.calls = []
def throttle(self):
current_time = time.time()
self.calls = [call for call in self.calls if current_time - call < self.time_frame]
if len(self.calls) >= self.max_calls:
return False
self.calls.append(current_time)
return True
throttler = InputThrottler(max_calls=5, time_frame=60)
def handle_user_input():
if throttler.throttle():
# Process user input
print("Input processed")
else:
print("Too many requests. Please try again later.")
6.2. Asynchronous Input Handling
For applications that need to handle multiple inputs simultaneously or prevent blocking operations, consider using asynchronous input handling.
import asyncio
async def handle_input():
while True:
user_input = await asyncio.to_thread(input, "Enter a command: ")
print(f"You entered: {user_input}")
if user_input.lower() == 'quit':
break
async def main():
await asyncio.gather(
handle_input(),
some_other_async_task()
)
asyncio.run(main())
6.3. Input Caching and History
Implement input caching and history features to improve user experience, especially in command-line interfaces.
import readline
def setup_input_history():
histfile = '.input_history'
try:
readline.read_history_file(histfile)
readline.set_history_length(1000)
except FileNotFoundError:
pass
def save_history(histfile=histfile):
readline.write_history_file(histfile)
import atexit
atexit.register(save_history)
setup_input_history()
while True:
command = input("Enter a command (or 'quit' to exit): ")
if command.lower() == 'quit':
break
print(f"Executing: {command}")
7. Testing Input Handling
Thorough testing of input handling is crucial to ensure the robustness and security of your application. Here are some approaches to testing input handling:
7.1. Unit Testing
Write unit tests to verify that your input validation and sanitization functions work correctly for various input scenarios.
import unittest
def validate_age(age):
if not isinstance(age, int) or age < 0 or age > 150:
raise ValueError("Age must be an integer between 0 and 150")
return age
class TestAgeValidation(unittest.TestCase):
def test_valid_age(self):
self.assertEqual(validate_age(25), 25)
self.assertEqual(validate_age(0), 0)
self.assertEqual(validate_age(150), 150)
def test_invalid_age(self):
with self.assertRaises(ValueError):
validate_age(-1)
with self.assertRaises(ValueError):
validate_age(151)
with self.assertRaises(ValueError):
validate_age("not a number")
if __name__ == '__main__':
unittest.main()
7.2. Fuzz Testing
Use fuzz testing techniques to generate random, unexpected inputs and ensure your application handles them gracefully.
import random
import string
def fuzz_test_input_handler(input_handler, num_tests=1000):
for _ in range(num_tests):
fuzz_input = ''.join(random.choices(string.printable, k=random.randint(1, 100)))
try:
result = input_handler(fuzz_input)
print(f"Input: {fuzz_input}, Output: {result}")
except Exception as e:
print(f"Input: {fuzz_input}, Exception: {str(e)}")
def example_input_handler(input_string):
# Your input handling logic here
if len(input_string) > 50:
raise ValueError("Input too long")
return input_string.upper()
fuzz_test_input_handler(example_input_handler)
7.3. Integration Testing
Perform integration tests to ensure that your input handling works correctly within the context of your entire application.
import sys
from io import StringIO
import unittest
class TestUserInputIntegration(unittest.TestCase):
def test_user_registration(self):
# Simulate user input
user_input = StringIO("John Doe\n25\njohn@example.com\n")
sys.stdin = user_input
# Capture output
captured_output = StringIO()
sys.stdout = captured_output
# Run the user registration function
register_user()
# Reset stdin and stdout
sys.stdin = sys.__stdin__
sys.stdout = sys.__stdout__
# Check the output
output = captured_output.getvalue()
self.assertIn("Registration successful", output)
self.assertIn("Name: John Doe", output)
self.assertIn("Age: 25", output)
self.assertIn("Email: john@example.com", output)
if __name__ == '__main__':
unittest.main()
8. Best Practices for Handling User Input
To wrap up our comprehensive guide, let’s review some best practices for handling user input safely and effectively:
- Never trust user input: Always validate and sanitize all input, regardless of its source.
- Use type-specific validation: Apply appropriate validation techniques based on the expected data type (e.g., numeric range checks for numbers, pattern matching for emails).
- Implement strong input sanitization: Remove or escape potentially harmful characters, especially when dealing with data that will be displayed on web pages or stored in databases.
- Provide clear error messages: Help users understand what went wrong and how to correct their input.
- Use secure coding practices: Avoid common vulnerabilities like SQL injection and cross-site scripting by using parameterized queries and proper escaping techniques.
- Implement input throttling: Protect your application from abuse by limiting the rate of input processing.
- Use appropriate input methods: Choose the right input method for your application type (e.g., command-line arguments for scripts, form inputs for web applications).
- Handle exceptions gracefully: Use try-except blocks to catch and handle potential errors without crashing the application.
- Test thoroughly: Implement unit tests, integration tests, and fuzz testing to ensure robust input handling.
- Keep security in mind: Regularly update your input handling techniques to address new security threats and vulnerabilities.
Conclusion
Handling user input safely and effectively is a critical skill for any programmer. By implementing the techniques and best practices outlined in this guide, you can create more secure, stable, and user-friendly applications. Remember that input handling is an ongoing process, and it’s important to stay updated on the latest security threats and best practices in this area.
As you continue to develop your programming skills, consider exploring more advanced topics related to user input handling, such as natural language processing for more complex user interactions, or machine learning techniques for input prediction and auto-completion. By mastering the art of handling user input, you’ll be well-equipped to build robust and secure applications in any programming domain.