How to Handle Tree Serialization: A Comprehensive Guide
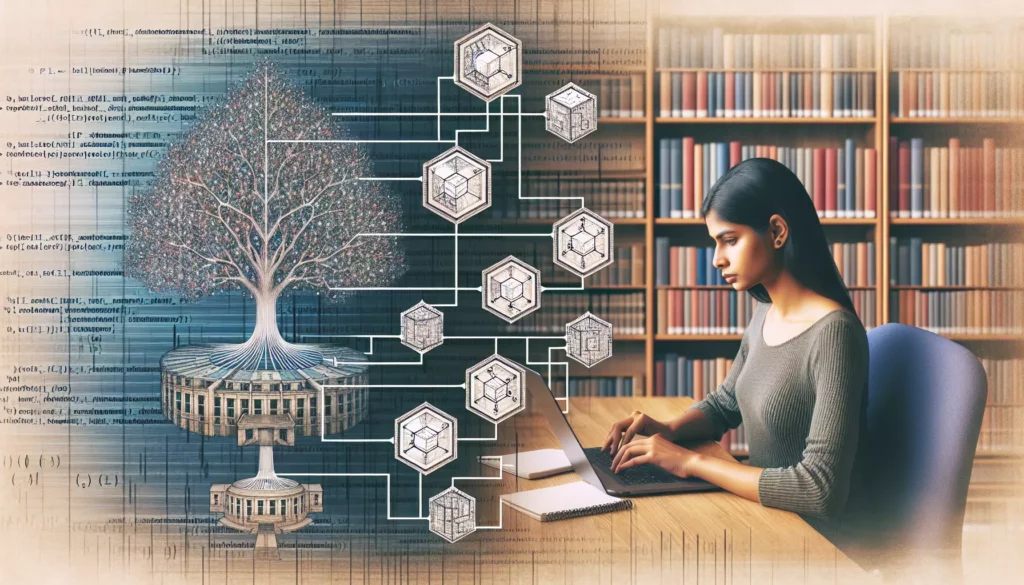
Tree serialization is a crucial concept in computer science and programming, especially when dealing with data structures and algorithms. It’s a process that converts a tree data structure into a format that can be easily stored or transmitted, and later reconstructed. This skill is particularly valuable for developers preparing for technical interviews at major tech companies, often referred to as FAANG (Facebook, Amazon, Apple, Netflix, Google).
In this comprehensive guide, we’ll explore the ins and outs of tree serialization, covering everything from basic concepts to advanced techniques. Whether you’re a beginner looking to understand the fundamentals or an experienced programmer aiming to sharpen your skills for technical interviews, this article will provide valuable insights and practical examples.
Table of Contents
- Understanding Tree Structures
- What is Tree Serialization?
- Why is Tree Serialization Important?
- Common Approaches to Tree Serialization
- Implementing Tree Serialization
- Deserializing Trees
- Handling Edge Cases
- Optimizing Serialization Techniques
- Real-World Applications of Tree Serialization
- Common Interview Questions on Tree Serialization
- Conclusion
1. Understanding Tree Structures
Before diving into serialization, it’s crucial to have a solid understanding of tree structures. A tree is a hierarchical data structure consisting of nodes connected by edges. Each node in a tree can have zero or more child nodes, and exactly one parent node (except for the root node, which has no parent).
Here’s a simple representation of a binary tree node in Python:
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
Trees are used in various applications, including file systems, organization charts, and hierarchical data representation. They provide an efficient way to store and retrieve data, making them a popular choice in many algorithms and data structures.
2. What is Tree Serialization?
Tree serialization is the process of converting a tree data structure into a linear sequence of data that can be easily stored or transmitted. This sequence should contain enough information to reconstruct the original tree structure when needed. The goal is to create a format that is both compact and easily parseable.
For example, a simple binary tree serialization might look like this:
"1,2,3,null,null,4,5"
In this representation, each node’s value is listed in a level-order traversal, with “null” representing absent children. This string represents a binary tree where the root has value 1, its left child is 2, its right child is 3, and 3 has left and right children 4 and 5 respectively.
3. Why is Tree Serialization Important?
Tree serialization is important for several reasons:
- Data Storage: It allows complex tree structures to be stored in databases or files efficiently.
- Data Transmission: Serialized trees can be easily sent over networks or between different systems.
- Caching: Serialization enables caching of tree structures for quick retrieval and reconstruction.
- Interoperability: It allows trees to be shared between different programming languages or systems that may have different internal representations.
- Versioning and Persistence: Serialization is crucial for saving the state of tree structures, enabling features like undo/redo or saving application states.
Understanding and implementing tree serialization is often a key component in technical interviews, especially for roles involving data structures and algorithms.
4. Common Approaches to Tree Serialization
There are several approaches to serializing trees, each with its own advantages and use cases:
1. Level-Order Traversal (Breadth-First)
This approach visits nodes level by level, from left to right. It’s intuitive and preserves the structure of the tree well.
"1,2,3,null,null,4,5"
2. Preorder Traversal (Depth-First)
In this method, we visit the root, then the left subtree, then the right subtree. It’s efficient for recreating the tree and works well with recursive algorithms.
"1,2,null,null,3,4,null,null,5,null,null"
3. Parenthesis Representation
This method uses parentheses to denote the structure of the tree. It’s very readable but can be verbose for large trees.
"1(2()())(3(4()())(5()()))"
4. Nested List Representation
Similar to the parenthesis method, but using lists or arrays. It’s particularly useful in languages with good list manipulation capabilities.
[1, [2, [], []], [3, [4, [], []], [5, [], []]]]
5. Implementing Tree Serialization
Let’s implement a simple tree serialization using the level-order traversal approach in Python:
from collections import deque
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def serialize(root):
if not root:
return "null"
queue = deque([root])
result = []
while queue:
node = queue.popleft()
if node:
result.append(str(node.val))
queue.append(node.left)
queue.append(node.right)
else:
result.append("null")
while result[-1] == "null":
result.pop()
return ",".join(result)
# Example usage
root = TreeNode(1)
root.left = TreeNode(2)
root.right = TreeNode(3)
root.right.left = TreeNode(4)
root.right.right = TreeNode(5)
serialized = serialize(root)
print(serialized) # Output: 1,2,3,null,null,4,5
This implementation uses a breadth-first search approach to traverse the tree level by level. It handles null nodes and trims trailing nulls for a more compact representation.
6. Deserializing Trees
Deserialization is the process of reconstructing the tree from its serialized form. Here’s how we can implement deserialization for our level-order serialization:
def deserialize(data):
if data == "null":
return None
nodes = data.split(",")
root = TreeNode(int(nodes[0]))
queue = deque([root])
i = 1
while queue and i < len(nodes):
node = queue.popleft()
if i < len(nodes) and nodes[i] != "null":
node.left = TreeNode(int(nodes[i]))
queue.append(node.left)
i += 1
if i < len(nodes) and nodes[i] != "null":
node.right = TreeNode(int(nodes[i]))
queue.append(node.right)
i += 1
return root
# Example usage
serialized = "1,2,3,null,null,4,5"
root = deserialize(serialized)
# Function to print the tree (for verification)
def print_tree(node):
if not node:
return
print(node.val, end=" ")
print_tree(node.left)
print_tree(node.right)
print_tree(root) # Output: 1 2 3 4 5
This deserialization function recreates the tree by processing the serialized string level by level, creating nodes as it goes and linking them appropriately.
7. Handling Edge Cases
When implementing tree serialization and deserialization, it’s crucial to handle various edge cases:
- Empty Trees: Ensure your functions can handle null or empty trees correctly.
- Single Node Trees: The serialization should work for trees with just one node.
- Unbalanced Trees: Your implementation should handle trees that are heavily skewed to one side.
- Large Trees: Consider memory efficiency for very large trees.
- Trees with Duplicate Values: Make sure your serialization can distinguish between different nodes with the same value.
Here’s an example of how to handle some of these edge cases:
def serialize_with_edge_cases(root):
if not root:
return "null"
# Rest of the serialization logic...
def deserialize_with_edge_cases(data):
if data == "null":
return None
# Rest of the deserialization logic...
# Test edge cases
print(serialize_with_edge_cases(None)) # Empty tree
print(serialize_with_edge_cases(TreeNode(1))) # Single node tree
# Unbalanced tree
unbalanced_root = TreeNode(1)
unbalanced_root.left = TreeNode(2)
unbalanced_root.left.left = TreeNode(3)
print(serialize_with_edge_cases(unbalanced_root))
8. Optimizing Serialization Techniques
As you become more comfortable with basic serialization, consider these optimization techniques:
1. Compression
For large trees, you can implement compression algorithms to reduce the size of the serialized data. For example, you could use run-length encoding for sequences of null values.
2. Custom Encoding
Instead of using strings, you could encode the tree structure using bits or custom byte representations for more compact storage.
3. Partial Serialization
In some cases, you might only need to serialize part of a tree. Implementing partial serialization can save space and time.
4. Streaming Serialization
For very large trees, consider implementing a streaming approach where you serialize and deserialize the tree in chunks, reducing memory usage.
Here’s a simple example of run-length encoding for null values:
def serialize_compressed(root):
if not root:
return "null"
result = []
queue = deque([root])
null_count = 0
while queue:
node = queue.popleft()
if node:
if null_count > 0:
result.append(f"n{null_count}")
null_count = 0
result.append(str(node.val))
queue.append(node.left)
queue.append(node.right)
else:
null_count += 1
if null_count > 0:
result.append(f"n{null_count}")
return ",".join(result)
# Example usage
root = TreeNode(1)
root.left = TreeNode(2)
root.right = TreeNode(3)
root.right.right = TreeNode(4)
print(serialize_compressed(root)) # Output: 1,2,3,n1,4,n2
9. Real-World Applications of Tree Serialization
Tree serialization finds applications in various domains:
1. Database Systems
Many databases use tree structures (like B-trees or B+ trees) for indexing. Serialization is crucial for storing these structures on disk.
2. File Systems
Directory structures in file systems are essentially trees. Serialization is used to store and retrieve these structures efficiently.
3. Game Development
Game state, including scene graphs or decision trees, often needs to be serialized for saving and loading game progress.
4. Network Protocols
Tree structures are sometimes used in network protocols, and serialization is necessary for transmitting these structures over the network.
5. Machine Learning
Decision trees and random forests in machine learning models often need to be serialized for storage or deployment.
10. Common Interview Questions on Tree Serialization
Here are some common interview questions related to tree serialization, along with brief solutions:
Q1: How would you serialize a binary tree to a file and deserialize it back?
A: Use a preorder traversal to serialize the tree, writing each node’s value and a marker for null children. For deserialization, read the file and reconstruct the tree using the same preorder approach.
Q2: How can you serialize an N-ary tree?
A: One approach is to use a format like “value(child1)(child2)…(childN)” for each node, recursively applying this to all children.
Q3: How would you handle serialization of a tree with cycles?
A: Use a hash map to keep track of already serialized nodes. When encountering a cycle, use a special marker to indicate a reference to an existing node.
Q4: Design a serialization method that is space-efficient for sparse trees.
A: Use a depth-first approach and only store the path to non-null nodes. For example, “1L2R3” could represent a tree where 2 is the left child of 1, and 3 is the right child of 1.
Q5: How would you modify your serialization method to handle binary trees with parent pointers?
A: Serialize the tree structure without parent pointers first. During deserialization, maintain a stack or map of parent nodes to reconstruct the parent pointers.
11. Conclusion
Tree serialization is a fundamental concept in computer science with wide-ranging applications. Mastering this skill not only prepares you for technical interviews but also equips you with knowledge applicable to various real-world scenarios in software development.
Remember, the key to excelling in tree serialization problems is to:
- Understand the tree structure thoroughly
- Choose the appropriate serialization method based on the problem requirements
- Handle edge cases carefully
- Consider optimization techniques for large-scale applications
- Practice with diverse tree structures and serialization scenarios
As you continue your journey in coding education and skill development, keep exploring different tree structures and serialization techniques. The more you practice, the more comfortable you’ll become with these concepts, setting you up for success in your programming career and technical interviews.