How to Handle Tree Path Problems: A Comprehensive Guide
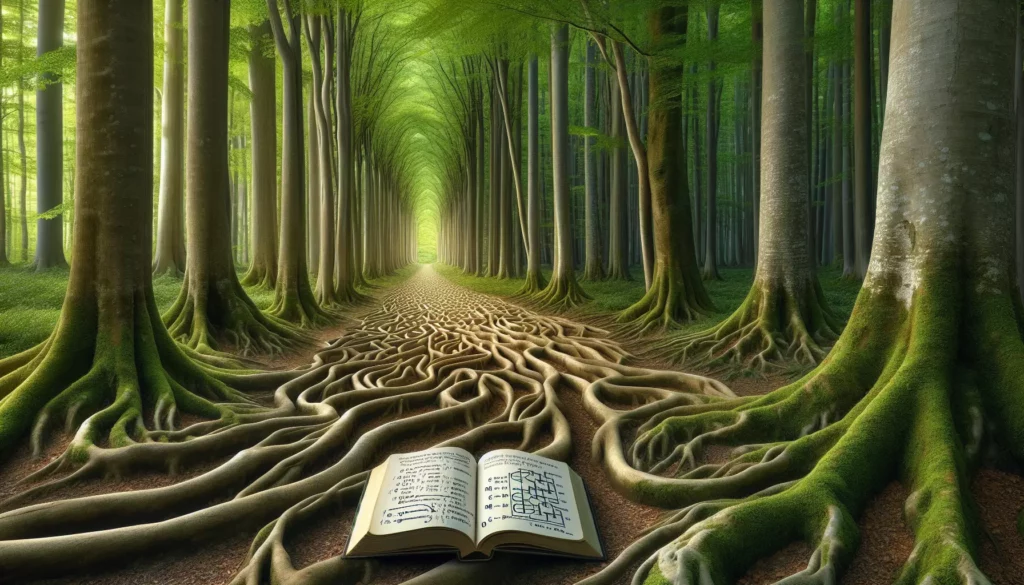
Tree path problems are a common type of coding challenge that often appear in technical interviews, especially for positions at major tech companies. These problems involve navigating and manipulating tree data structures, which are fundamental in computer science and have wide-ranging applications in real-world scenarios. In this comprehensive guide, we’ll explore various strategies and techniques to effectively handle tree path problems, providing you with the skills needed to excel in your coding interviews and beyond.
Understanding Tree Data Structures
Before diving into specific problem-solving techniques, it’s crucial to have a solid understanding of tree data structures. A tree is a hierarchical data structure consisting of nodes connected by edges. Each node can have multiple child nodes, but only one parent node (except for the root node, which has no parent).
Here’s a basic implementation of a binary tree node in Python:
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
This simple structure forms the foundation for many tree-related problems. Now, let’s explore some common types of tree path problems and how to approach them.
1. Path Sum Problems
Path sum problems involve finding paths in a tree that sum up to a specific value. These problems test your ability to traverse a tree and keep track of cumulative sums along the way.
Example: Path Sum
Given a binary tree and a target sum, determine if the tree has a root-to-leaf path such that adding up all the values along the path equals the target sum.
Here’s a recursive solution to this problem:
def hasPathSum(root, targetSum):
if not root:
return False
if not root.left and not root.right:
return root.val == targetSum
return hasPathSum(root.left, targetSum - root.val) or hasPathSum(root.right, targetSum - root.val)
This solution uses a recursive approach, subtracting the current node’s value from the target sum as it traverses down the tree. If it reaches a leaf node and the remaining sum equals the leaf’s value, it returns True.
Variations and Extensions
- Find all paths that sum to a target value
- Find the maximum path sum in the tree
- Count the number of paths with a given sum
These variations often require similar techniques but may involve additional data structures like lists to keep track of paths or global variables to store results across recursive calls.
2. Lowest Common Ancestor (LCA) Problems
Finding the lowest common ancestor of two nodes in a tree is another common type of tree path problem. The LCA is the deepest node in the tree that is an ancestor of both given nodes.
Example: Lowest Common Ancestor in a Binary Tree
Given a binary tree and two nodes p and q, find their lowest common ancestor.
Here’s an efficient solution to this problem:
def lowestCommonAncestor(root, p, q):
if not root or root == p or root == q:
return root
left = lowestCommonAncestor(root.left, p, q)
right = lowestCommonAncestor(root.right, p, q)
if left and right:
return root
return left if left else right
This solution uses a bottom-up approach. It recursively searches for p and q in the left and right subtrees. If both are found in different subtrees, the current node is the LCA. If one is found, that node is returned up the recursion chain.
Variations and Extensions
- Find LCA in a binary search tree
- Find LCA of multiple nodes
- Find the distance between two nodes in a tree
These variations may require modifications to the basic LCA algorithm or additional traversal techniques.
3. Tree Serialization and Deserialization
Serialization is the process of converting a data structure into a format that can be easily stored or transmitted. Deserialization is the reverse process. These problems test your ability to represent tree structures in different formats and reconstruct them.
Example: Serialize and Deserialize Binary Tree
Design an algorithm to serialize and deserialize a binary tree.
Here’s a solution using preorder traversal and string manipulation:
class Codec:
def serialize(self, root):
if not root:
return "null"
return str(root.val) + "," + self.serialize(root.left) + "," + self.serialize(root.right)
def deserialize(self, data):
def dfs():
val = next(values)
if val == "null":
return None
node = TreeNode(int(val))
node.left = dfs()
node.right = dfs()
return node
values = iter(data.split(","))
return dfs()
# Your Codec object will be instantiated and called as such:
# codec = Codec()
# codec.deserialize(codec.serialize(root))
This solution uses a preorder traversal to serialize the tree into a string, with “null” representing null nodes. The deserialization process uses a depth-first approach to reconstruct the tree from the serialized string.
Variations and Extensions
- Serialize and deserialize N-ary trees
- Compress the serialized representation
- Handle special characters in node values
These variations may require different traversal methods or encoding techniques to efficiently represent the tree structure.
4. Tree Construction Problems
Tree construction problems involve building a tree from given information, such as traversal sequences or level order representations. These problems test your understanding of tree properties and traversal algorithms.
Example: Construct Binary Tree from Preorder and Inorder Traversal
Given two integer arrays preorder and inorder where preorder is the preorder traversal of a binary tree and inorder is the inorder traversal of the same tree, construct and return the binary tree.
Here’s a solution to this problem:
def buildTree(preorder, inorder):
if not preorder or not inorder:
return None
root = TreeNode(preorder[0])
mid = inorder.index(preorder[0])
root.left = buildTree(preorder[1:mid+1], inorder[:mid])
root.right = buildTree(preorder[mid+1:], inorder[mid+1:])
return root
This solution uses the property that the first element in preorder traversal is always the root. It then finds this element in the inorder traversal to determine the sizes of left and right subtrees, and recursively constructs them.
Variations and Extensions
- Construct tree from postorder and inorder traversal
- Construct binary search tree from preorder traversal
- Construct complete binary tree from level order traversal
These variations may require different techniques to determine the root and subtree structures based on the given information.
5. Tree Path Printing Problems
These problems involve finding and printing specific paths in a tree, often with certain constraints or conditions.
Example: Binary Tree Paths
Given a binary tree, return all root-to-leaf paths in any order.
Here’s a solution using depth-first search:
def binaryTreePaths(root):
def dfs(node, path):
if not node:
return
path += str(node.val)
if not node.left and not node.right:
result.append(path)
return
path += "->"
dfs(node.left, path)
dfs(node.right, path)
result = []
dfs(root, "")
return result
This solution uses a helper function to perform a depth-first search, building the path string as it goes. When it reaches a leaf node, it adds the complete path to the result list.
Variations and Extensions
- Print all paths with a given sum
- Find the longest path in the tree
- Print paths in a specific order (e.g., lexicographically)
These variations may require additional logic to filter paths based on certain criteria or to order the paths in a specific way.
Advanced Techniques for Tree Path Problems
As you progress in your problem-solving skills, you’ll encounter more complex tree path problems that require advanced techniques. Here are some strategies to tackle these challenges:
1. Dynamic Programming on Trees
Dynamic programming can be applied to tree problems to optimize solutions, especially when dealing with subtree properties or path optimizations.
Example: House Robber III
The thief has found himself a new place for his thievery again. There is only one entrance to this area, called the “root.” Besides the root, each house has one and only one parent house. After a tour, the smart thief realized that “all houses in this place forms a binary tree”. It will automatically contact the police if two directly-linked houses were broken into on the same night.
Determine the maximum amount of money the thief can rob tonight without alerting the police.
Here’s a dynamic programming solution:
def rob(root):
def dfs(node):
if not node:
return 0, 0
left = dfs(node.left)
right = dfs(node.right)
rob_root = node.val + left[1] + right[1]
not_rob_root = max(left) + max(right)
return rob_root, not_rob_root
return max(dfs(root))
This solution uses a bottom-up approach, where each node returns two values: the maximum amount if we rob this node, and the maximum amount if we don’t rob this node.
2. Tree Diameter Problems
Tree diameter problems involve finding the longest path between any two nodes in a tree. These problems often require a two-pass approach or clever use of depth-first search.
Example: Diameter of Binary Tree
Given a binary tree, you need to compute the length of the diameter of the tree. The diameter of a binary tree is the length of the longest path between any two nodes in a tree. This path may or may not pass through the root.
Here’s an efficient solution:
def diameterOfBinaryTree(root):
diameter = 0
def dfs(node):
nonlocal diameter
if not node:
return 0
left = dfs(node.left)
right = dfs(node.right)
diameter = max(diameter, left + right)
return max(left, right) + 1
dfs(root)
return diameter
This solution uses a single depth-first search pass, updating the global diameter variable as it goes and returning the height of each subtree.
3. Lowest Common Ancestor Variations
More complex variations of the LCA problem can involve finding LCA in different types of trees or with additional constraints.
Example: Lowest Common Ancestor of Deepest Leaves
Given the root of a binary tree, return the lowest common ancestor of its deepest leaves.
Here’s a solution that combines finding the tree height with LCA:
def lcaDeepestLeaves(root):
def dfs(node, depth):
if not node:
return None, depth
left, left_depth = dfs(node.left, depth + 1)
right, right_depth = dfs(node.right, depth + 1)
if left_depth > right_depth:
return left, left_depth
elif right_depth > left_depth:
return right, right_depth
else:
return node, left_depth
return dfs(root, 0)[0]
This solution uses a single depth-first search to find both the deepest leaves and their LCA simultaneously.
Practical Tips for Solving Tree Path Problems
As you practice and encounter more tree path problems, keep these tips in mind:
- Visualize the tree: Always start by drawing out the tree structure. This helps in understanding the problem and coming up with a solution strategy.
- Choose the right traversal: Different problems may be more suited to different traversal methods (preorder, inorder, postorder, or level-order). Choose the one that best fits the problem requirements.
- Consider recursive vs. iterative approaches: While recursive solutions are often more intuitive for tree problems, iterative solutions using stacks or queues can sometimes be more efficient or easier to implement.
- Use helper functions: Breaking down the problem into smaller helper functions can make your code more readable and easier to debug.
- Handle edge cases: Always consider edge cases like empty trees, single-node trees, or trees with specific structures (e.g., skewed trees).
- Optimize space usage: For large trees, consider solutions that use constant extra space (e.g., Morris traversal) or optimize recursion to reduce stack space.
- Practice, practice, practice: The more tree problems you solve, the more patterns you’ll recognize, making it easier to tackle new challenges.
Conclusion
Tree path problems are a fundamental aspect of computer science and a common feature in coding interviews. By understanding the various types of tree path problems and the techniques to solve them, you’ll be well-equipped to handle a wide range of challenges in your coding journey.
Remember that mastering these problems takes time and practice. Start with simpler problems and gradually work your way up to more complex ones. As you gain experience, you’ll develop an intuition for approaching tree problems and be able to recognize patterns that can be applied across different problem types.
Keep exploring, practicing, and refining your skills. With dedication and consistent effort, you’ll be well on your way to becoming proficient in handling tree path problems and excelling in your coding interviews.