How to Handle Tree Balance Problems: A Comprehensive Guide
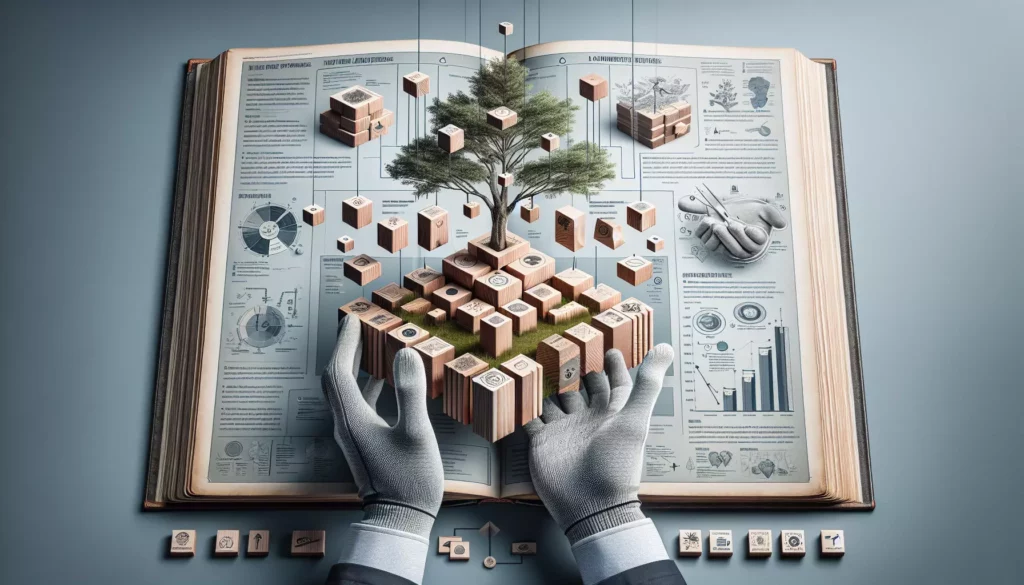
In the world of data structures and algorithms, balanced trees play a crucial role in optimizing search, insertion, and deletion operations. However, maintaining tree balance can be a challenging task, especially when dealing with dynamic data. In this comprehensive guide, we’ll explore various techniques to handle tree balance problems, focusing on popular balanced tree structures and their implementation.
Table of Contents
- Introduction to Tree Balance
- AVL Trees
- Red-Black Trees
- B-Trees
- Splay Trees
- Treap (Tree + Heap)
- Comparison of Balanced Tree Structures
- Implementing Balanced Trees
- Common Tree Balance Problems and Solutions
- Conclusion
1. Introduction to Tree Balance
Tree balance is a critical concept in computer science that ensures efficient operations on tree data structures. A balanced tree is one where the height of the left and right subtrees of every node differs by at most one. This balance property guarantees that operations like search, insertion, and deletion can be performed in logarithmic time complexity.
Unbalanced trees can lead to performance degradation, with operations potentially taking linear time in the worst case. This is particularly problematic when dealing with large datasets or in applications where quick access and modifications are crucial.
2. AVL Trees
AVL trees, named after their inventors Adelson-Velsky and Landis, are self-balancing binary search trees. They maintain balance by ensuring that the height difference between left and right subtrees (balance factor) of any node is at most 1.
Key Properties of AVL Trees:
- Balance Factor: For each node, |height(left subtree) – height(right subtree)| ≤ 1
- Height-balance property ensures O(log n) time complexity for search, insert, and delete operations
- Rebalancing is performed through rotations after insertions and deletions
AVL Tree Rotations:
- Left Rotation
- Right Rotation
- Left-Right Rotation
- Right-Left Rotation
Here’s a simple implementation of an AVL tree node in Python:
class AVLNode:
def __init__(self, key):
self.key = key
self.left = None
self.right = None
self.height = 1
class AVLTree:
def __init__(self):
self.root = None
def height(self, node):
if not node:
return 0
return node.height
def balance_factor(self, node):
if not node:
return 0
return self.height(node.left) - self.height(node.right)
def update_height(self, node):
if not node:
return
node.height = 1 + max(self.height(node.left), self.height(node.right))
# ... (rotation and insertion methods would follow)
3. Red-Black Trees
Red-Black trees are another type of self-balancing binary search tree. They use a coloring scheme to maintain balance, with each node colored either red or black.
Properties of Red-Black Trees:
- Every node is either red or black
- The root is always black
- Every leaf (NIL) is black
- If a node is red, then both its children are black
- For each node, all simple paths from the node to descendant leaves contain the same number of black nodes
These properties ensure that the tree remains approximately balanced, with no path being more than twice as long as any other path.
Red-Black Tree Operations:
- Insertion: O(log n) time complexity
- Deletion: O(log n) time complexity
- Search: O(log n) time complexity
Here’s a basic structure for a Red-Black tree node in C++:
enum Color { RED, BLACK };
struct Node {
int key;
Color color;
Node *left, *right, *parent;
Node(int k) : key(k), color(RED), left(nullptr), right(nullptr), parent(nullptr) {}
};
class RedBlackTree {
private:
Node *root;
// Helper functions
void leftRotate(Node *x);
void rightRotate(Node *y);
void insertFixup(Node *z);
void deleteFixup(Node *x);
public:
RedBlackTree() : root(nullptr) {}
void insert(int key);
void remove(int key);
Node* search(int key);
};
4. B-Trees
B-Trees are self-balancing tree data structures that maintain sorted data and allow searches, sequential access, insertions, and deletions in logarithmic time. They are particularly useful in storage systems that read and write large blocks of data, such as databases and file systems.
Key Characteristics of B-Trees:
- All leaf nodes must be at the same depth
- All internal nodes (except root) have between t-1 and 2t-1 keys, where t is the minimum degree of the B-tree
- The root has at least 2 children if it’s not a leaf
- All nodes (except root) have at least t children
- All keys within a node are sorted in ascending order
B-Tree Operations:
- Search: O(log n)
- Insert: O(log n)
- Delete: O(log n)
Here’s a simplified structure for a B-Tree node in Java:
public class BTreeNode {
private int[] keys; // An array of keys
private int t; // Minimum degree (defines the range for number of keys)
private BTreeNode[] children; // An array of child pointers
private int n; // Current number of keys
private boolean leaf; // Is true when node is leaf. Otherwise false
public BTreeNode(int t, boolean leaf) {
this.t = t;
this.leaf = leaf;
this.keys = new int[2*t - 1];
this.children = new BTreeNode[2*t];
this.n = 0;
}
// Other methods like insertNonFull, splitChild, etc. would be implemented here
}
public class BTree {
private BTreeNode root;
private int t; // Minimum degree
public BTree(int t) {
this.root = null;
this.t = t;
}
// Methods for search, insert, and delete would be implemented here
}
5. Splay Trees
Splay trees are self-adjusting binary search trees with the additional property that recently accessed elements are quick to access again. They perform basic operations such as insertion, look-up and removal in O(log n) amortized time.
Key Features of Splay Trees:
- No explicit balance condition
- Recently accessed elements move closer to the root
- Good performance in practice for many applications
- Simple implementation compared to other self-balancing trees
Splay Tree Operations:
- Splay: Moves a given element to the root
- Insert: O(log n) amortized time
- Delete: O(log n) amortized time
- Search: O(log n) amortized time
Here’s a basic implementation of a Splay tree node in Python:
class SplayNode:
def __init__(self, key):
self.key = key
self.left = None
self.right = None
class SplayTree:
def __init__(self):
self.root = None
def splay(self, key):
if not self.root:
return None
dummy = SplayNode(None)
left = right = dummy
current = self.root
while True:
if key < current.key:
if not current.left:
break
if key < current.left.key:
# Rotate right
y = current.left
current.left = y.right
y.right = current
current = y
if not current.left:
break
# Link right
right.left = current
right = current
current = current.left
elif key > current.key:
if not current.right:
break
if key > current.right.key:
# Rotate left
y = current.right
current.right = y.left
y.left = current
current = y
if not current.right:
break
# Link left
left.right = current
left = current
current = current.right
else:
break
# Assemble
left.right = current.left
right.left = current.right
current.left = dummy.right
current.right = dummy.left
self.root = current
return self.root
# Other methods (insert, delete, search) would be implemented here
6. Treap (Tree + Heap)
A Treap is a randomized binary search tree that combines the properties of a binary search tree and a heap. Each node in a Treap has two values: a key and a priority. The tree is structured so that it’s a valid binary search tree with respect to the keys and a max-heap with respect to the priorities.
Key Properties of Treaps:
- Binary Search Tree property for keys
- Max-heap property for priorities
- Priorities are randomly assigned, which helps in maintaining balance
Treap Operations:
- Insert: O(log n) expected time
- Delete: O(log n) expected time
- Search: O(log n) expected time
Here’s a simple implementation of a Treap node in C++:
class TreapNode {
public:
int key;
int priority;
TreapNode* left;
TreapNode* right;
TreapNode(int k) : key(k), priority(rand()), left(nullptr), right(nullptr) {}
};
class Treap {
private:
TreapNode* root;
TreapNode* rotateRight(TreapNode* y) {
TreapNode* x = y->left;
TreapNode* T2 = x->right;
x->right = y;
y->left = T2;
return x;
}
TreapNode* rotateLeft(TreapNode* x) {
TreapNode* y = x->right;
TreapNode* T2 = y->left;
y->left = x;
x->right = T2;
return y;
}
TreapNode* insert(TreapNode* root, int key) {
if (!root)
return new TreapNode(key);
if (key <= root->key) {
root->left = insert(root->left, key);
if (root->left->priority > root->priority)
root = rotateRight(root);
}
else {
root->right = insert(root->right, key);
if (root->right->priority > root->priority)
root = rotateLeft(root);
}
return root;
}
public:
Treap() : root(nullptr) {}
void insert(int key) {
root = insert(root, key);
}
// Other methods (search, delete) would be implemented here
};
7. Comparison of Balanced Tree Structures
Each balanced tree structure has its own strengths and is suited for different scenarios. Here’s a brief comparison:
Tree Type | Time Complexity (Average) | Space Complexity | Balancing Mechanism | Best Use Case |
---|---|---|---|---|
AVL Trees | O(log n) for all operations | O(n) | Strict balancing with rotations | Frequent lookups, less frequent updates |
Red-Black Trees | O(log n) for all operations | O(n) | Color-based balancing with rotations | Frequent insertions and deletions |
B-Trees | O(log n) for all operations | O(n) | Multiple keys per node, splitting and merging | Database systems, file systems |
Splay Trees | O(log n) amortized for all operations | O(n) | Move-to-root heuristic | Caching, frequently accessed elements |
Treap | O(log n) expected for all operations | O(n) | Randomized priorities | Simple implementation, good overall performance |
8. Implementing Balanced Trees
When implementing balanced trees, there are several key considerations:
1. Choose the Right Structure:
Select the balanced tree structure that best fits your use case. Consider factors like the frequency of different operations, the nature of your data, and the specific requirements of your application.
2. Implement Basic Operations:
Start with implementing the fundamental operations: insertion, deletion, and search. These form the core of any tree structure.
3. Balance Maintenance:
Implement the balancing mechanisms specific to your chosen tree structure. This might involve rotations, color changes, or other rebalancing operations.
4. Testing:
Thoroughly test your implementation with various scenarios, including edge cases. Ensure that the tree maintains its balance properties after multiple operations.
5. Performance Optimization:
Look for opportunities to optimize your implementation, such as using iterative approaches instead of recursive ones where appropriate.
6. Memory Management:
In languages without automatic garbage collection, ensure proper memory management to avoid leaks, especially during node deletion.
9. Common Tree Balance Problems and Solutions
Problem 1: Skewed Trees
Issue: Inserting elements in sorted order can lead to a skewed tree, degrading performance to O(n).
Solution: Use self-balancing trees like AVL or Red-Black trees, which automatically rebalance after insertions and deletions.
Problem 2: Frequent Rebalancing Overhead
Issue: Some balanced trees (like AVL) may require frequent rebalancing, adding overhead to insertions and deletions.
Solution: Consider using Red-Black trees, which require fewer rotations on average, or Splay trees for scenarios with localized access patterns.
Problem 3: Large Node Size in Memory-Constrained Environments
Issue: Some balanced trees require additional information per node (like color or balance factor), which can be problematic in memory-constrained environments.
Solution: Use space-efficient implementations or consider alternatives like B-trees, which can store multiple keys per node.
Problem 4: Performance Degradation with Duplicate Keys
Issue: Some balanced tree implementations may not handle duplicate keys efficiently.
Solution: Modify the tree implementation to allow duplicate keys, possibly by using a linked list for equal keys or by storing a count with each key.
Problem 5: Complexity of Implementation
Issue: Implementing and maintaining complex balanced tree structures can be error-prone and time-consuming.
Solution: Consider using library implementations when available, or opt for simpler structures like Treaps for prototyping and small-scale applications.
10. Conclusion
Handling tree balance problems is crucial for maintaining efficient data structures in many applications. By understanding the various balanced tree structures and their properties, you can choose the most appropriate solution for your specific needs.
Remember that each balanced tree structure has its own strengths and weaknesses. AVL trees offer strict balance but with higher maintenance costs, Red-Black trees provide a good balance between performance and complexity, B-trees excel in systems with block-based storage, Splay trees are great for scenarios with localized access patterns, and Treaps offer a simple randomized approach to balance.
When implementing balanced trees, focus on correctly handling the core operations and balance maintenance. Always test thoroughly, considering edge cases and performance under various scenarios. With practice and experience, you’ll become proficient at recognizing and solving tree balance problems, a valuable skill for any software developer or computer scientist.
As you continue your journey in algorithm and data structure mastery, remember that balanced trees are just one piece of the puzzle. Keep exploring other advanced data structures and algorithms to broaden your problem-solving toolkit and enhance your coding skills.