How to Handle String Reversal Problems: A Comprehensive Guide
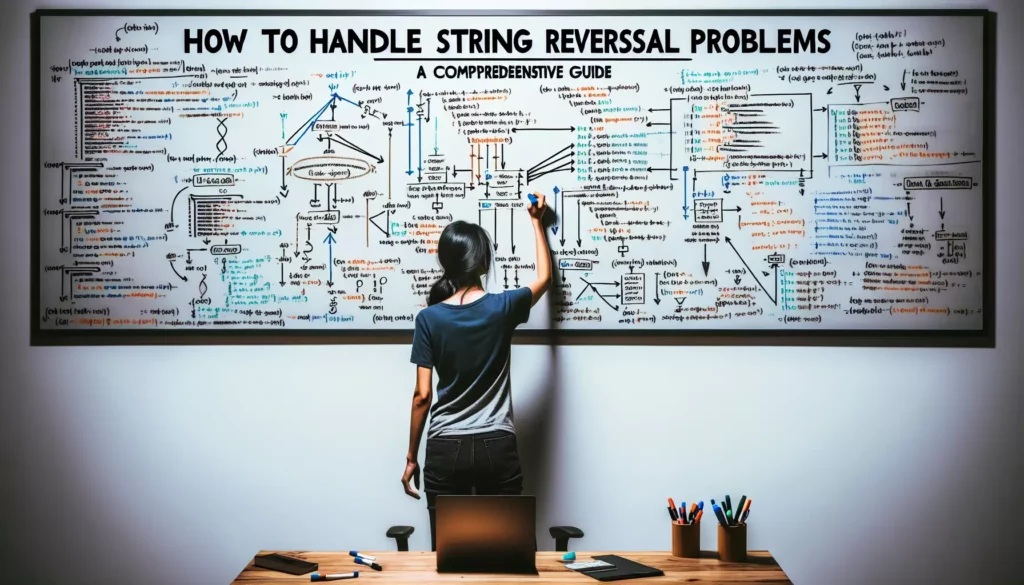
String reversal is a classic programming problem that often appears in coding interviews and serves as a fundamental exercise for beginners learning about string manipulation. In this comprehensive guide, we’ll explore various approaches to string reversal, discuss their time and space complexities, and provide practical examples to help you master this essential skill.
Table of Contents
- Introduction to String Reversal
- The Importance of String Reversal in Programming
- Different Approaches to String Reversal
- Language-Specific Considerations
- Handling Edge Cases and Special Characters
- Optimization Techniques
- Interview Tips for String Reversal Problems
- Practice Problems and Variations
- Conclusion
1. Introduction to String Reversal
String reversal is the process of changing the order of characters in a string so that the last character becomes the first, the second-to-last becomes the second, and so on. For example, reversing the string “hello” would result in “olleh”.
While it may seem simple at first glance, string reversal can be approached in various ways, each with its own advantages and trade-offs. Understanding these different methods can help you choose the most appropriate solution based on the specific requirements of the problem at hand.
2. The Importance of String Reversal in Programming
String reversal is more than just a common interview question; it’s a fundamental operation that has practical applications in various programming scenarios:
- Palindrome checking: Reversing a string is often used as part of algorithms to determine if a word or phrase is a palindrome (reads the same forwards and backwards).
- Text processing: In text editors or word processors, reversing strings can be useful for implementing undo/redo functionality or for creating mirror effects in text art.
- Cryptography: Some simple encryption techniques involve reversing parts of a string as one step in the encryption process.
- Algorithm building blocks: String reversal often serves as a component in more complex string manipulation algorithms.
- Learning tool: For beginners, it’s an excellent exercise to practice string manipulation, loops, and basic algorithmic thinking.
Moreover, string reversal problems help assess a programmer’s ability to work with strings efficiently, handle edge cases, and optimize for performance – skills that are crucial in many programming tasks.
3. Different Approaches to String Reversal
Let’s explore various methods to reverse a string, discussing the implementation, time complexity, and space complexity of each approach.
Using Built-in Functions
Many programming languages provide built-in functions or methods to reverse strings. While these are often the most straightforward to use, it’s important to understand their limitations and the underlying mechanisms.
Python Example:
def reverse_string(s):
return s[::-1]
# Usage
original = "Hello, World!"
reversed = reverse_string(original)
print(reversed) # Output: !dlroW ,olleH
Time Complexity: O(n), where n is the length of the string
Space Complexity: O(n) for creating a new string
While this method is concise and readable, it may not be available in all languages, and interviewers might expect a more manual implementation to assess your understanding of string manipulation.
Two-Pointer Technique
The two-pointer technique is an efficient and widely applicable method for string reversal. It involves using two pointers, one starting from the beginning of the string and the other from the end, swapping characters as they move towards the center.
C++ Example:
#include <string>
#include <iostream>
void reverseString(std::string &s) {
int left = 0;
int right = s.length() - 1;
while (left < right) {
std::swap(s[left], s[right]);
left++;
right--;
}
}
int main() {
std::string str = "Hello, World!";
reverseString(str);
std::cout << str << std::endl; // Output: !dlroW ,olleH
return 0;
}
Time Complexity: O(n), where n is the length of the string
Space Complexity: O(1), as it reverses the string in-place
This approach is memory-efficient and works well for languages that allow in-place string modification. For immutable strings (like in Java or Python), you’d need to convert the string to a mutable data structure first.
Stack-based Approach
Using a stack is another common method for string reversal. This approach leverages the Last-In-First-Out (LIFO) property of stacks to naturally reverse the order of characters.
Java Example:
import java.util.Stack;
public class StringReversal {
public static String reverseString(String s) {
Stack<Character> stack = new Stack<>();
for (char c : s.toCharArray()) {
stack.push(c);
}
StringBuilder reversed = new StringBuilder();
while (!stack.isEmpty()) {
reversed.append(stack.pop());
}
return reversed.toString();
}
public static void main(String[] args) {
String original = "Hello, World!";
String reversed = reverseString(original);
System.out.println(reversed); // Output: !dlroW ,olleH
}
}
Time Complexity: O(n), where n is the length of the string
Space Complexity: O(n) for the stack and the new string
While this method is not as memory-efficient as the two-pointer approach, it can be useful in scenarios where you need to reverse only a portion of a string or when working with streams of characters.
Recursive Approach
Recursion offers an elegant solution to string reversal, though it may not be the most efficient for very long strings due to the overhead of function calls.
JavaScript Example:
function reverseString(s) {
// Base case: if the string is empty or has only one character
if (s.length <= 1) return s;
// Recursive case: reverse the substring excluding the first character
// and append the first character at the end
return reverseString(s.slice(1)) + s[0];
}
// Usage
const original = "Hello, World!";
const reversed = reverseString(original);
console.log(reversed); // Output: !dlroW ,olleH
Time Complexity: O(n^2) due to string concatenation in each recursive call
Space Complexity: O(n) for the call stack
While this recursive approach is conceptually simple, it’s important to note that it’s not the most efficient method, especially for large strings. The repeated string concatenation can lead to quadratic time complexity in languages with immutable strings.
String Slicing (Python-specific)
Python offers a unique and concise way to reverse strings using slicing with a step of -1. While we mentioned this briefly in the built-in functions section, it’s worth exploring in more detail due to its elegance and efficiency in Python.
def reverse_string(s):
return s[::-1]
# Usage
original = "Hello, World!"
reversed = reverse_string(original)
print(reversed) # Output: !dlroW ,olleH
Time Complexity: O(n), where n is the length of the string
Space Complexity: O(n) for creating a new string
This method is highly efficient in Python and is often the preferred way to reverse strings. However, it’s important to understand that this is a Python-specific feature and may not be available or as efficient in other languages.
4. Language-Specific Considerations
When working with string reversal, it’s crucial to consider the specific characteristics and limitations of the programming language you’re using:
- String Mutability: In languages like Python and Java, strings are immutable, meaning you can’t modify them in-place. You’ll need to create a new string or convert to a mutable data structure first.
- Character Encoding: Be aware of how your language handles Unicode characters. Some methods might not correctly reverse strings containing multi-byte characters or combining characters.
- Performance Considerations: In languages like C++, using std::reverse() from the <algorithm> library can be more efficient than implementing your own reversal function.
- Built-in Methods: Some languages provide built-in methods for string reversal (e.g., StringBuilder.reverse() in Java). While these are convenient, interviewers might expect you to implement the reversal manually.
5. Handling Edge Cases and Special Characters
When implementing string reversal, it’s important to consider various edge cases and special scenarios:
- Empty Strings: Your function should handle empty strings gracefully, typically by returning an empty string.
- Single-Character Strings: These should remain unchanged after reversal.
- Unicode and Multi-byte Characters: Ensure your reversal method correctly handles strings with Unicode characters, especially those represented by multiple bytes.
- Whitespace: Consider how your function should handle leading, trailing, or multiple spaces within the string.
- Special Characters and Punctuation: Decide whether these should be treated differently or simply reversed along with the rest of the string.
Here’s an example that addresses some of these considerations:
def reverse_string(s):
if not s: # Handle empty string
return ""
# Convert to list for easier manipulation of Unicode characters
chars = list(s)
left, right = 0, len(chars) - 1
while left < right:
chars[left], chars[right] = chars[right], chars[left]
left += 1
right -= 1
return ''.join(chars)
# Test cases
print(reverse_string("")) # Empty string
print(reverse_string("a")) # Single character
print(reverse_string("Hello, World!")) # With punctuation
print(reverse_string(" spaces ")) # With leading/trailing spaces
print(reverse_string("😊ðŸŒ")) # Unicode characters
6. Optimization Techniques
While basic string reversal is relatively straightforward, there are several optimization techniques you can apply to improve performance or handle specific scenarios more efficiently:
- In-place Reversal: For languages that allow it, reversing the string in-place (like the two-pointer method) is memory-efficient.
- Avoiding Unnecessary Allocations: In languages with immutable strings, consider using mutable data structures like character arrays or StringBuilder (in Java) to avoid creating multiple string objects.
- Chunking for Long Strings: For very long strings, you can reverse chunks of the string separately and then combine them, which can be more cache-friendly.
- Parallel Processing: For extremely large strings, you could potentially use parallel processing to reverse different segments simultaneously, then combine the results.
Here’s an example of a chunk-based reversal in Python:
def reverse_string_chunked(s, chunk_size=1000):
if len(s) <= chunk_size:
return s[::-1]
chunks = []
for i in range(0, len(s), chunk_size):
chunk = s[i:i+chunk_size]
chunks.append(chunk[::-1])
return ''.join(reversed(chunks))
# Usage
long_string = "a" * 1000000 + "b" * 1000000
reversed_string = reverse_string_chunked(long_string)
print(reversed_string[:10], reversed_string[-10:]) # Check first and last 10 characters
This chunked approach can be more efficient for very long strings, as it works on smaller pieces of the string at a time, which can be more cache-friendly and reduce memory pressure.
7. Interview Tips for String Reversal Problems
When tackling string reversal problems in coding interviews, keep these tips in mind:
- Clarify the Requirements: Ask about any specific constraints or expectations, such as in-place reversal or handling of special characters.
- Start with a Simple Approach: Begin with a straightforward solution, then optimize if needed. This shows you can quickly produce working code.
- Discuss Trade-offs: Explain the pros and cons of different approaches, especially regarding time and space complexity.
- Consider Edge Cases: Mention and handle edge cases like empty strings or single-character strings.
- Think About Scalability: If asked, discuss how your solution would handle very long strings or how it could be optimized for large-scale applications.
- Test Your Code: Write a few test cases to verify your solution, including edge cases.
- Optimize if Time Allows: If you implement a basic solution quickly, consider optimizing it or discussing potential optimizations.
8. Practice Problems and Variations
To further hone your skills in string manipulation and reversal, try these practice problems and variations:
- Reverse Words in a String: Given a string of words, reverse the order of the words while keeping the words themselves intact.
- Reverse Only Alphabetical Characters: Reverse a string, but keep non-alphabetical characters in their original positions.
- Palindrome Check: Determine if a given string is a palindrome (reads the same forwards and backwards), ignoring spaces and punctuation.
- Reverse String in Groups: Reverse every k characters in a given string, where k is an input parameter.
- Reverse Vowels: Reverse only the vowels in a given string, keeping all other characters in their original positions.
Here’s an example solution for reversing words in a string:
def reverse_words(s):
# Split the string into words
words = s.split()
# Reverse the list of words and join them back into a string
return ' '.join(reversed(words))
# Test the function
sentence = "Hello World! How are you?"
reversed_sentence = reverse_words(sentence)
print(reversed_sentence) # Output: "you? are How World! Hello"
Practicing these variations will help you become more comfortable with string manipulation and prepare you for a wide range of potential interview questions.
9. Conclusion
String reversal is a fundamental problem in computer programming that serves as a gateway to more complex string manipulation tasks. By mastering various approaches to string reversal, you’ll not only be better prepared for coding interviews but also develop a deeper understanding of string handling in your chosen programming language.
Remember that the “best” method for reversing a string can vary depending on the specific requirements of your task, the programming language you’re using, and the constraints of your system. Always consider factors like time complexity, space complexity, and the specific needs of your project when choosing an approach.
As you continue to practice and refine your skills, you’ll find that the principles you learn from string reversal problems – such as efficient iteration, in-place manipulation, and handling of edge cases – will serve you well in tackling a wide variety of programming challenges. Keep practicing, stay curious, and always look for ways to optimize and improve your code!