How to Handle String Pattern Matching: A Comprehensive Guide
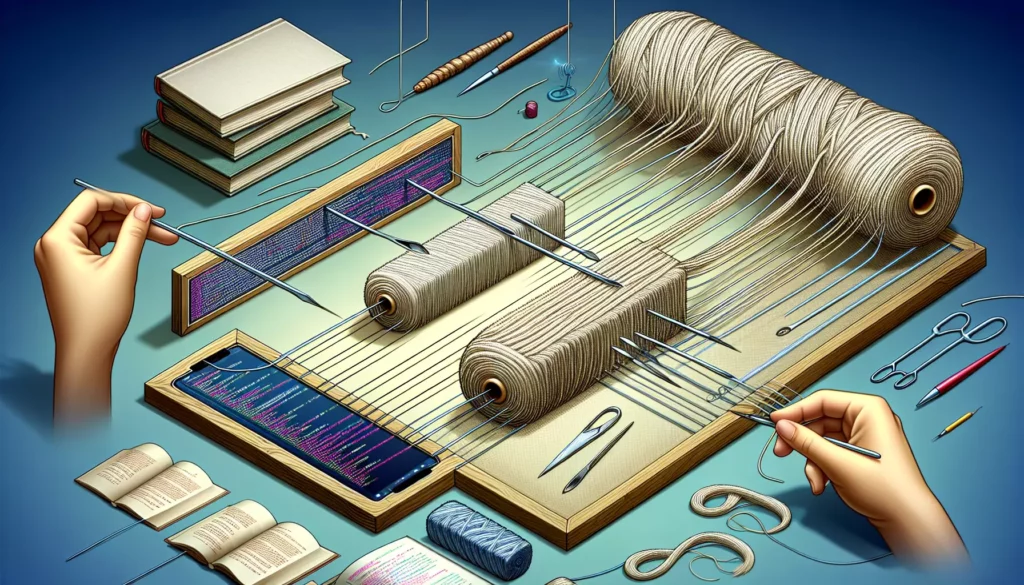
String pattern matching is a fundamental concept in computer science and programming that plays a crucial role in various applications, from text processing to data analysis. As an essential skill for developers, mastering string pattern matching can significantly enhance your problem-solving abilities and coding efficiency. In this comprehensive guide, we’ll explore different techniques and algorithms for handling string pattern matching, providing you with the knowledge and tools to tackle complex string-related problems.
Table of Contents
- Introduction to String Pattern Matching
- Basic Techniques for String Pattern Matching
- Advanced Algorithms for Efficient Pattern Matching
- Regular Expressions: A Powerful Tool for Pattern Matching
- Practical Applications of String Pattern Matching
- Best Practices and Performance Considerations
- Conclusion
1. Introduction to String Pattern Matching
String pattern matching is the process of finding occurrences of a specific pattern within a larger text or string. This fundamental operation is used in various scenarios, such as:
- Searching for keywords in a document
- Validating user input
- Parsing and processing structured data
- Implementing search functionality in applications
- Analyzing DNA sequences in bioinformatics
Understanding and implementing efficient string pattern matching algorithms is crucial for developers, as it can significantly impact the performance and functionality of their applications. Let’s dive into the various techniques and algorithms used for string pattern matching.
2. Basic Techniques for String Pattern Matching
Before we explore advanced algorithms, let’s start with some basic techniques for string pattern matching. These methods are straightforward and easy to implement, making them suitable for simple use cases or as a starting point for more complex solutions.
2.1. Brute Force Method
The brute force method is the simplest approach to string pattern matching. It involves comparing the pattern with every possible substring of the text, character by character. While this method is easy to implement, it can be inefficient for large texts or patterns.
Here’s a simple implementation of the brute force method in Python:
def brute_force_search(text, pattern):
n = len(text)
m = len(pattern)
for i in range(n - m + 1):
j = 0
while j < m and text[i + j] == pattern[j]:
j += 1
if j == m:
return i # Pattern found at index i
return -1 # Pattern not found
# Example usage
text = "Hello, world! This is a test string."
pattern = "test"
result = brute_force_search(text, pattern)
print(f"Pattern found at index: {result}")
This method has a time complexity of O(n * m), where n is the length of the text and m is the length of the pattern. While it works well for small inputs, it becomes inefficient for larger texts or patterns.
2.2. Two-Pointer Technique
The two-pointer technique is another simple approach that can be more efficient than the brute force method in certain scenarios. This technique involves using two pointers to compare the pattern with the text, moving them in a coordinated manner to avoid unnecessary comparisons.
Here’s an example implementation of the two-pointer technique in Python:
def two_pointer_search(text, pattern):
i = 0 # Pointer for text
j = 0 # Pointer for pattern
while i < len(text) and j < len(pattern):
if text[i] == pattern[j]:
i += 1
j += 1
else:
i = i - j + 1
j = 0
if j == len(pattern):
return i - j # Pattern found
else:
return -1 # Pattern not found
# Example usage
text = "Hello, world! This is a test string."
pattern = "test"
result = two_pointer_search(text, pattern)
print(f"Pattern found at index: {result}")
The two-pointer technique can be more efficient than the brute force method in cases where the pattern has repeated characters or when partial matches are common. However, its worst-case time complexity is still O(n * m).
3. Advanced Algorithms for Efficient Pattern Matching
While basic techniques can work well for simple cases, more advanced algorithms are needed for efficient pattern matching in larger texts or when dealing with complex patterns. Let’s explore some of the most popular and efficient string pattern matching algorithms.
3.1. Knuth-Morris-Pratt (KMP) Algorithm
The Knuth-Morris-Pratt (KMP) algorithm is an efficient string matching algorithm that improves upon the basic techniques by utilizing information about the pattern itself. It precomputes a failure function (also known as the prefix function) to avoid unnecessary comparisons when a mismatch occurs.
Here’s an implementation of the KMP algorithm in Python:
def kmp_search(text, pattern):
def compute_prefix_function(pattern):
m = len(pattern)
pi = [0] * m
k = 0
for q in range(1, m):
while k > 0 and pattern[k] != pattern[q]:
k = pi[k - 1]
if pattern[k] == pattern[q]:
k += 1
pi[q] = k
return pi
n = len(text)
m = len(pattern)
pi = compute_prefix_function(pattern)
q = 0
for i in range(n):
while q > 0 and pattern[q] != text[i]:
q = pi[q - 1]
if pattern[q] == text[i]:
q += 1
if q == m:
return i - m + 1 # Pattern found
return -1 # Pattern not found
# Example usage
text = "Hello, world! This is a test string."
pattern = "test"
result = kmp_search(text, pattern)
print(f"Pattern found at index: {result}")
The KMP algorithm has a time complexity of O(n + m), where n is the length of the text and m is the length of the pattern. This makes it significantly more efficient than the brute force method, especially for large inputs.
3.2. Boyer-Moore Algorithm
The Boyer-Moore algorithm is another efficient string matching algorithm that improves performance by using information gathered from the pattern. It uses two heuristics: the bad character heuristic and the good suffix heuristic. These heuristics allow the algorithm to skip unnecessary comparisons, making it particularly efficient for large alphabets and long patterns.
Here’s a simplified implementation of the Boyer-Moore algorithm using only the bad character heuristic:
def boyer_moore_search(text, pattern):
def build_bad_char_heuristic(pattern):
bad_char = {}
for i in range(len(pattern)):
bad_char[pattern[i]] = i
return bad_char
m = len(pattern)
n = len(text)
bad_char = build_bad_char_heuristic(pattern)
i = 0
while i <= n - m:
j = m - 1
while j >= 0 and pattern[j] == text[i + j]:
j -= 1
if j < 0:
return i # Pattern found
else:
i += max(1, j - bad_char.get(text[i + j], -1))
return -1 # Pattern not found
# Example usage
text = "Hello, world! This is a test string."
pattern = "test"
result = boyer_moore_search(text, pattern)
print(f"Pattern found at index: {result}")
The Boyer-Moore algorithm has a best-case time complexity of O(n/m) and a worst-case time complexity of O(n*m). In practice, it often outperforms other algorithms, especially for larger alphabets and longer patterns.
3.3. Rabin-Karp Algorithm
The Rabin-Karp algorithm is a string matching algorithm that uses hashing to find patterns in text. It calculates hash values for the pattern and substrings of the text, comparing them to identify potential matches. This approach is particularly useful when searching for multiple patterns simultaneously.
Here’s an implementation of the Rabin-Karp algorithm in Python:
def rabin_karp_search(text, pattern):
d = 256 # Number of characters in the input alphabet
q = 101 # A prime number
m = len(pattern)
n = len(text)
p = 0 # Hash value for pattern
t = 0 # Hash value for text
h = 1
# Calculate h = d^(m-1) % q
for i in range(m - 1):
h = (h * d) % q
# Calculate initial hash values
for i in range(m):
p = (d * p + ord(pattern[i])) % q
t = (d * t + ord(text[i])) % q
# Slide the pattern over text one by one
for i in range(n - m + 1):
if p == t:
# Check for characters one by one
if text[i:i+m] == pattern:
return i
if i < n - m:
t = (d * (t - ord(text[i]) * h) + ord(text[i + m])) % q
if t < 0:
t += q
return -1 # Pattern not found
# Example usage
text = "Hello, world! This is a test string."
pattern = "test"
result = rabin_karp_search(text, pattern)
print(f"Pattern found at index: {result}")
The Rabin-Karp algorithm has an average and best-case time complexity of O(n + m), but its worst-case time complexity is O(n*m). It’s particularly useful when searching for multiple patterns simultaneously, as the hash function can be computed once for each text position.
4. Regular Expressions: A Powerful Tool for Pattern Matching
Regular expressions (regex) provide a powerful and flexible way to perform pattern matching in strings. While not a specific algorithm, regex is a language for describing patterns that can be used to search, match, and manipulate text. Most programming languages have built-in support for regex, making it a versatile tool for developers.
Here are some key concepts and examples of using regex for pattern matching:
4.1. Basic Regex Patterns
.
– Matches any single character except newline*
– Matches zero or more occurrences of the previous character+
– Matches one or more occurrences of the previous character?
– Matches zero or one occurrence of the previous character^
– Matches the start of a line$
– Matches the end of a line[]
– Matches any single character within the brackets[^]
– Matches any single character not within the brackets
4.2. Regex in Python
Python provides the re
module for working with regular expressions. Here’s an example of using regex for pattern matching in Python:
import re
text = "Hello, world! This is a test string. Test is important."
pattern = r"test"
# Case-insensitive search
matches = re.finditer(pattern, text, re.IGNORECASE)
for match in matches:
print(f"Found '{match.group()}' at position {match.start()}")
# Output:
# Found 'test' at position 24
# Found 'Test' at position 40
This example demonstrates how to use regex to find all occurrences of a pattern in a text, ignoring case sensitivity.
4.3. Common Regex Use Cases
Regular expressions are useful for various pattern matching tasks, including:
- Validating email addresses or phone numbers
- Extracting specific information from structured text
- Parsing and manipulating log files
- Implementing search functionality with advanced options
- Cleaning and preprocessing text data
While regex is powerful, it’s important to note that complex regex patterns can be difficult to read and maintain. For simple pattern matching tasks, using built-in string methods or the algorithms discussed earlier may be more appropriate.
5. Practical Applications of String Pattern Matching
String pattern matching has numerous practical applications across various domains of software development and data analysis. Let’s explore some common use cases where pattern matching skills can be applied:
5.1. Text Editors and IDEs
Text editors and Integrated Development Environments (IDEs) heavily rely on string pattern matching for features such as:
- Find and replace functionality
- Syntax highlighting
- Code refactoring
- Auto-completion suggestions
Implementing these features often involves efficient pattern matching algorithms to provide a smooth user experience, especially when working with large codebases.
5.2. Data Validation and Parsing
Pattern matching is crucial for validating and parsing various types of data, including:
- Email addresses
- Phone numbers
- Dates and times
- IP addresses
- URLs
Regular expressions are commonly used for these tasks, as they provide a concise way to define complex patterns. Here’s an example of validating an email address using regex in Python:
import re
def is_valid_email(email):
pattern = r"^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$"
return re.match(pattern, email) is not None
# Example usage
emails = ["user@example.com", "invalid.email@", "another.user@domain.co.uk"]
for email in emails:
print(f"{email}: {'Valid' if is_valid_email(email) else 'Invalid'}")
# Output:
# user@example.com: Valid
# invalid.email@: Invalid
# another.user@domain.co.uk: Valid
5.3. Log Analysis and System Monitoring
System administrators and DevOps engineers often use pattern matching techniques to analyze log files and monitor system health. This involves tasks such as:
- Identifying error messages or specific events in log files
- Extracting relevant information from structured log entries
- Detecting patterns that indicate potential security threats
Here’s an example of parsing a simple log file to extract information using regex:
import re
log_entry = "2023-04-15 14:30:22 [INFO] User john.doe@example.com logged in from 192.168.1.100"
pattern = r"(\d{4}-\d{2}-\d{2} \d{2}:\d{2}:\d{2}) \[(\w+)\] User (\S+) logged in from (\d+\.\d+\.\d+\.\d+)"
match = re.match(pattern, log_entry)
if match:
timestamp, log_level, user_email, ip_address = match.groups()
print(f"Timestamp: {timestamp}")
print(f"Log Level: {log_level}")
print(f"User Email: {user_email}")
print(f"IP Address: {ip_address}")
else:
print("Log entry does not match the expected format")
# Output:
# Timestamp: 2023-04-15 14:30:22
# Log Level: INFO
# User Email: john.doe@example.com
# IP Address: 192.168.1.100
5.4. Natural Language Processing (NLP)
In the field of Natural Language Processing, pattern matching plays a crucial role in various tasks, including:
- Tokenization: Breaking text into individual words or phrases
- Named Entity Recognition (NER): Identifying and classifying named entities in text
- Part-of-speech tagging: Assigning grammatical categories to words
- Information extraction: Extracting structured information from unstructured text
While more advanced NLP techniques often involve machine learning models, pattern matching remains an important tool for many text processing tasks.
5.5. Bioinformatics
In bioinformatics, string pattern matching algorithms are used to analyze DNA, RNA, and protein sequences. Applications include:
- Identifying specific gene sequences or motifs
- Aligning multiple sequences to find similarities
- Searching for patterns associated with genetic diseases
The efficiency of pattern matching algorithms is particularly important in this field due to the large size of genomic datasets.
6. Best Practices and Performance Considerations
When working with string pattern matching, it’s important to consider best practices and performance implications to ensure efficient and maintainable code. Here are some key points to keep in mind:
6.1. Choose the Right Algorithm
Select the appropriate pattern matching algorithm based on your specific use case:
- For simple, short patterns and small texts, basic techniques like the two-pointer method may be sufficient.
- For longer patterns or larger texts, consider using more efficient algorithms like KMP or Boyer-Moore.
- When searching for multiple patterns simultaneously, the Rabin-Karp algorithm or Aho-Corasick algorithm may be more suitable.
- For complex pattern matching needs with flexibility, regular expressions are often the best choice.
6.2. Optimize for Performance
Consider the following performance optimizations:
- Precompute any necessary data structures (e.g., the failure function in KMP) to avoid redundant calculations.
- Use efficient data structures like hash tables for quick lookups when appropriate.
- For repeated searches in the same text, consider building an index or suffix array to speed up subsequent queries.
- When using regex, compile patterns in advance if they will be used multiple times.
6.3. Handle Edge Cases
Ensure your pattern matching code handles various edge cases, such as:
- Empty strings (both for the text and the pattern)
- Patterns longer than the text
- Special characters or Unicode symbols
- Case sensitivity (when relevant)
6.4. Write Clear and Maintainable Code
Follow these guidelines to improve code readability and maintainability:
- Use meaningful variable and function names that describe their purpose.
- Add comments to explain complex logic or algorithm implementations.
- Break down complex pattern matching tasks into smaller, reusable functions.
- Use consistent formatting and follow language-specific style guides.
6.5. Test Thoroughly
Implement comprehensive test cases to ensure your pattern matching code works correctly:
- Test with various input sizes, from small to very large texts and patterns.
- Include edge cases and corner cases in your test suite.
- Use property-based testing to generate random inputs and verify algorithm correctness.
- Benchmark your implementation against built-in functions or libraries to ensure competitive performance.
7. Conclusion
String pattern matching is a fundamental skill for developers, with applications spanning various domains of computer science and software engineering. By understanding and implementing efficient pattern matching algorithms, you can significantly improve the performance and functionality of your applications.
In this comprehensive guide, we’ve covered:
- Basic techniques like the brute force method and two-pointer technique
- Advanced algorithms such as Knuth-Morris-Pratt, Boyer-Moore, and Rabin-Karp
- The power and flexibility of regular expressions
- Practical applications of string pattern matching in various fields
- Best practices and performance considerations
As you continue to develop your programming skills, remember that pattern matching is not just about implementing algorithms – it’s about choosing the right tool for the job and applying it effectively. Practice implementing these algorithms and using regex in your projects to gain hands-on experience and deepen your understanding of string pattern matching.
By mastering string pattern matching techniques, you’ll be better equipped to tackle complex problems in text processing, data analysis, and algorithm design. This knowledge will prove invaluable as you progress in your coding journey and prepare for technical interviews at top tech companies.