How to Handle String Concatenation Efficiently: A Comprehensive Guide
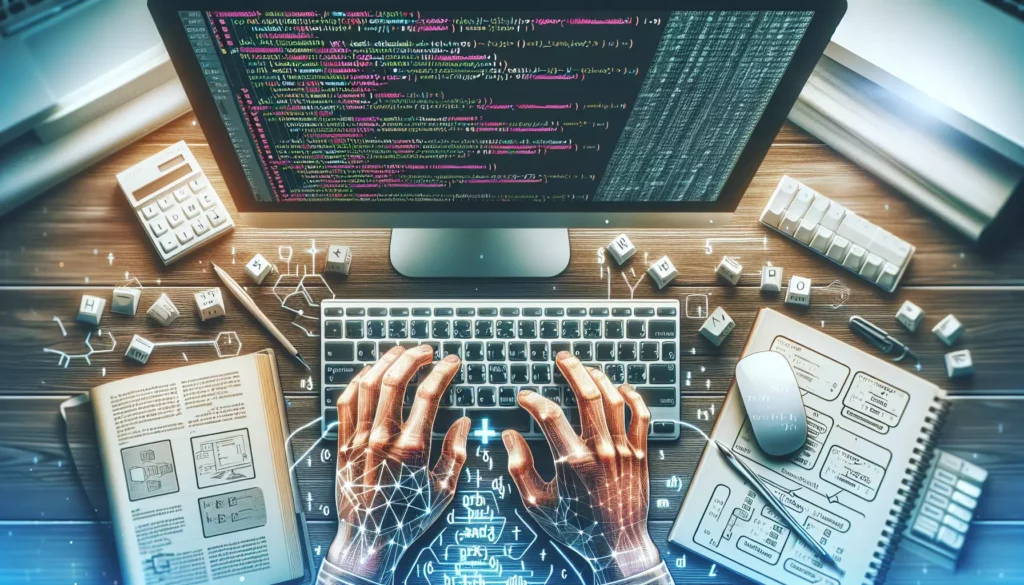
String concatenation is a fundamental operation in programming that involves combining two or more strings to create a new string. While it may seem like a simple task, the way you handle string concatenation can significantly impact your program’s performance, especially when dealing with large amounts of data or performing frequent concatenation operations. In this comprehensive guide, we’ll explore various methods of string concatenation across different programming languages, discuss their efficiency, and provide best practices for optimal performance.
Table of Contents
- Understanding String Concatenation
- String Concatenation in Different Programming Languages
- Efficient String Concatenation Techniques
- Performance Considerations
- Best Practices for Efficient String Concatenation
- Common Pitfalls to Avoid
- Advanced Techniques for Large-Scale String Operations
- Conclusion
1. Understanding String Concatenation
Before diving into the specifics of efficient string concatenation, it’s essential to understand what string concatenation is and why it’s important in programming.
String concatenation is the process of combining two or more strings to create a new string. This operation is used in various programming tasks, such as:
- Building dynamic text output
- Constructing SQL queries
- Formatting data for display or storage
- Creating URLs or file paths
- Manipulating text data in data processing applications
While string concatenation is a simple concept, its implementation can vary greatly in terms of efficiency, depending on the programming language and the specific method used.
2. String Concatenation in Different Programming Languages
Different programming languages handle string concatenation in various ways. Let’s look at some common approaches in popular languages:
Python
In Python, you can concatenate strings using the ‘+’ operator or the join() method:
# Using the '+' operator
result = "Hello, " + "world!"
# Using the join() method
words = ["Hello", "world!"]
result = " ".join(words)
Java
Java offers multiple ways to concatenate strings:
// Using the '+' operator
String result = "Hello, " + "world!";
// Using StringBuilder
StringBuilder sb = new StringBuilder();
sb.append("Hello, ");
sb.append("world!");
String result = sb.toString();
// Using String.concat()
String result = "Hello, ".concat("world!");
JavaScript
JavaScript provides several methods for string concatenation:
// Using the '+' operator
let result = "Hello, " + "world!";
// Using template literals
let greeting = "Hello";
let subject = "world";
let result = `${greeting}, ${subject}!`;
// Using the concat() method
let result = "Hello, ".concat("world!");
C#
C# offers multiple ways to concatenate strings:
// Using the '+' operator
string result = "Hello, " + "world!";
// Using string interpolation
string greeting = "Hello";
string subject = "world";
string result = $"{greeting}, {subject}!";
// Using StringBuilder
StringBuilder sb = new StringBuilder();
sb.Append("Hello, ");
sb.Append("world!");
string result = sb.ToString();
3. Efficient String Concatenation Techniques
Now that we’ve seen how different languages handle string concatenation, let’s explore some efficient techniques that can be applied across various programming languages:
3.1 Using StringBuilder or StringBuffer
Many languages provide a mutable string class (e.g., StringBuilder in Java and C#, or StringIO in Python) that allows for efficient string concatenation, especially when dealing with multiple concatenations in a loop.
// Java example
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 1000; i++) {
sb.append("Number: ").append(i).append("\n");
}
String result = sb.toString();
3.2 Join Method
Many languages offer a join method that efficiently concatenates an array or list of strings:
# Python example
words = ["Hello", "world", "How", "are", "you?"]
result = " ".join(words)
3.3 String Interpolation or Formatting
String interpolation or formatting can be more readable and sometimes more efficient than concatenation:
// C# example
string name = "Alice";
int age = 30;
string result = $"{name} is {age} years old.";
3.4 Array Join (for JavaScript)
In JavaScript, using array join can be more efficient than repeated concatenation:
let parts = ["Hello", "world", "How", "are", "you?"];
let result = parts.join(" ");
4. Performance Considerations
When it comes to string concatenation, performance can vary significantly depending on the method used and the specific use case. Here are some key performance considerations:
4.1 Immutability vs. Mutability
In many languages, strings are immutable, meaning that each concatenation operation creates a new string object. This can lead to performance issues, especially in loops. Using mutable string builders (like StringBuilder in Java or C#) can significantly improve performance in these cases.
4.2 Memory Allocation
Repeated string concatenations can lead to multiple memory allocations and deallocations, which can be costly in terms of performance. Methods like StringBuilder or StringBuffer pre-allocate memory, reducing the overhead of memory management.
4.3 Compile-Time vs. Runtime Concatenation
Some compilers optimize simple string concatenations at compile-time, especially when concatenating string literals. However, for dynamic strings or concatenations in loops, runtime performance becomes crucial.
4.4 Large-Scale Concatenations
For large-scale concatenations (e.g., building very large strings or processing large amounts of text data), consider using specialized techniques like memory-mapped files or streaming approaches.
5. Best Practices for Efficient String Concatenation
To ensure efficient string concatenation in your code, consider the following best practices:
5.1 Use StringBuilder for Multiple Concatenations
When concatenating strings in a loop or performing multiple concatenations, use a StringBuilder or equivalent mutable string class:
// Java example
StringBuilder sb = new StringBuilder();
for (String item : items) {
sb.append(item).append(", ");
}
String result = sb.toString().trim();
5.2 Prefer Join Method for Array-Like Structures
When concatenating an array or list of strings, use the join method provided by your language:
# Python example
items = ["apple", "banana", "cherry"]
result = ", ".join(items)
5.3 Use String Interpolation for Readability
When combining strings with variables, consider using string interpolation or formatting for better readability and potentially better performance:
// C# example
string name = "Bob";
int score = 95;
string result = $"{name} scored {score} points.";
5.4 Preallocate StringBuilder Capacity
If you know the approximate final size of your string, preallocate the capacity of your StringBuilder to avoid resizing:
// Java example
StringBuilder sb = new StringBuilder(1000); // Preallocate 1000 characters
// ... append operations ...
String result = sb.toString();
5.5 Avoid Unnecessary Concatenations
Be mindful of unnecessary concatenations, especially in loops. Try to minimize the number of concatenation operations:
// JavaScript example - Avoid this:
let result = "";
for (let i = 0; i < 1000; i++) {
result += "Number: " + i + "\n"; // Inefficient
}
// Better approach:
let parts = [];
for (let i = 0; i < 1000; i++) {
parts.push(`Number: ${i}`);
}
let result = parts.join("\n");
6. Common Pitfalls to Avoid
When working with string concatenation, be aware of these common pitfalls:
6.1 Concatenation in Tight Loops
Avoid concatenating strings inside tight loops using the ‘+’ operator, as this can lead to poor performance due to the creation of multiple intermediate string objects:
// Avoid this:
String result = "";
for (int i = 0; i < 10000; i++) {
result += "Number: " + i + "\n"; // Inefficient
}
// Better approach:
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 10000; i++) {
sb.append("Number: ").append(i).append("\n");
}
String result = sb.toString();
6.2 Overusing String Concatenation for Complex String Building
When building complex strings with multiple parts and conditions, avoid overusing concatenation. Instead, consider using string formatting or templating systems:
// Avoid this:
String message = "Dear " + title + " " + lastName + ",\n"
+ "Your account balance is $" + balance + ".\n"
+ (isPremium ? "Thank you for being a premium member!" : "");
// Better approach:
String message = String.format("Dear %s %s,\nYour account balance is $%.2f.\n%s",
title, lastName, balance,
isPremium ? "Thank you for being a premium member!" : "");
6.3 Ignoring Language-Specific Optimizations
Be aware of language-specific optimizations. For example, in Java, the ‘+’ operator is optimized for simple concatenations, but StringBuilder is still more efficient for multiple concatenations or concatenations in loops.
6.4 Unnecessary String Conversions
Avoid unnecessary conversions between strings and other data types when concatenating:
// Avoid this:
int number = 42;
String result = "" + number; // Implicit conversion
// Better approach:
String result = String.valueOf(number); // Explicit and more efficient
7. Advanced Techniques for Large-Scale String Operations
For applications dealing with very large strings or high-volume string processing, consider these advanced techniques:
7.1 Memory-Mapped Files
When working with extremely large strings that exceed available memory, consider using memory-mapped files. This technique allows you to treat a file as if it were in memory, enabling efficient random access to large amounts of data:
// Java example using memory-mapped files
import java.io.RandomAccessFile;
import java.nio.MappedByteBuffer;
import java.nio.channels.FileChannel;
RandomAccessFile file = new RandomAccessFile("largefile.txt", "rw");
FileChannel channel = file.getChannel();
MappedByteBuffer buffer = channel.map(FileChannel.MapMode.READ_WRITE, 0, channel.size());
// Now you can read and write to the buffer as if it were in memory
7.2 Streaming Approaches
For processing large amounts of text data, consider using streaming approaches that allow you to process data in chunks without loading everything into memory at once:
# Python example using streaming
def process_large_file(filename):
with open(filename, 'r') as file:
for line in file:
# Process each line
yield process_line(line)
# Usage
for processed_line in process_large_file('largefile.txt'):
print(processed_line)
7.3 Parallel Processing
For CPU-intensive string operations on large datasets, consider using parallel processing to leverage multi-core systems:
// Java example using parallel streams
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
List<String> words = Arrays.asList("Hello", "World", "Java", "Programming");
String result = words.parallelStream()
.map(String::toUpperCase)
.collect(Collectors.joining(" "));
7.4 Custom String Builders
For very specific use cases, you might consider implementing a custom string builder optimized for your particular needs. This could involve strategies like chunk-based allocation or specialized caching mechanisms.
8. Conclusion
Efficient string concatenation is a crucial skill for any programmer, especially when dealing with large-scale applications or performance-critical code. By understanding the underlying mechanics of string concatenation in your chosen programming language and applying the techniques and best practices outlined in this guide, you can significantly improve the performance and efficiency of your string operations.
Remember these key points:
- Use appropriate tools like StringBuilder for multiple concatenations
- Leverage language-specific methods like join() for array-like structures
- Consider string interpolation or formatting for better readability and performance
- Be mindful of performance in loops and large-scale operations
- Avoid common pitfalls like unnecessary conversions and concatenations
- For very large-scale operations, consider advanced techniques like memory-mapped files or streaming approaches
By mastering efficient string concatenation, you’ll not only write more performant code but also develop a deeper understanding of string handling and memory management in your programming language of choice. This knowledge will serve you well as you tackle more complex programming challenges and optimize your applications for better performance.