How to Handle Recursive Tree Problems: A Comprehensive Guide
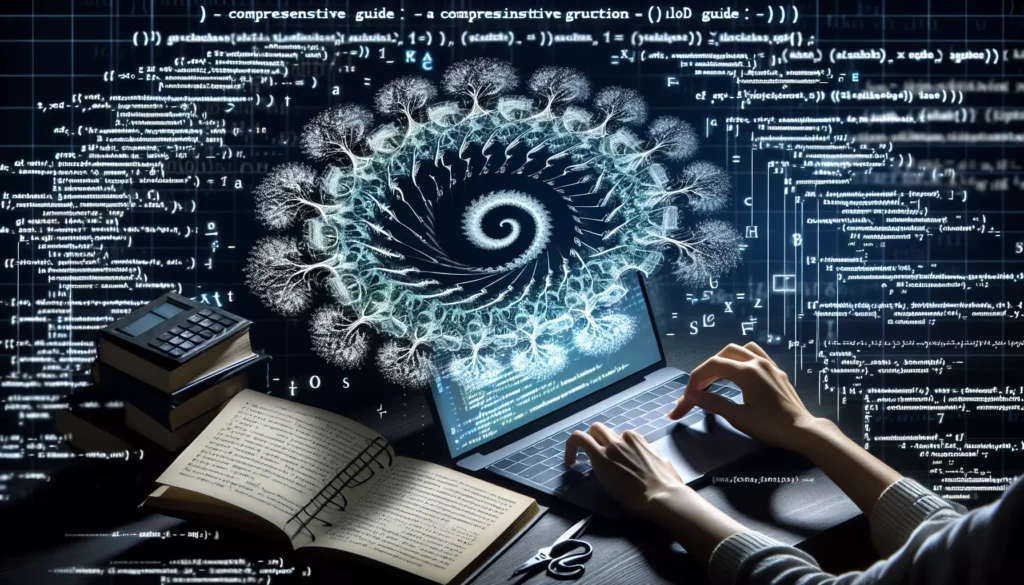
In the world of programming and computer science, tree data structures are ubiquitous. From file systems to HTML DOM, from organizational hierarchies to decision trees in machine learning, trees are everywhere. When it comes to solving problems involving trees, recursion often emerges as a powerful and elegant solution. In this comprehensive guide, we’ll explore how to handle recursive tree problems, providing you with the tools and techniques to tackle even the most complex tree-based challenges.
Table of Contents
- Understanding Trees and Their Properties
- Recursion Basics: The Foundation of Tree Traversal
- Common Recursive Tree Problems
- Advanced Techniques for Complex Tree Problems
- Optimization and Performance Considerations
- Real-World Applications of Recursive Tree Algorithms
- Conclusion
1. Understanding Trees and Their Properties
Before diving into recursive solutions for tree problems, it’s crucial to have a solid understanding of what trees are and their fundamental properties.
What is a Tree?
A tree is a hierarchical data structure consisting of nodes connected by edges. It has the following key characteristics:
- A tree has a root node, which is the topmost node in the hierarchy.
- Each node (except the root) has exactly one parent node.
- Each node can have zero or more child nodes.
- Nodes with no children are called leaf nodes.
- A tree cannot contain cycles.
Types of Trees
There are various types of trees, each with its own properties and use cases:
- Binary Trees: Each node has at most two children, typically referred to as the left and right child.
- Binary Search Trees (BST): A binary tree where the left subtree of a node contains only nodes with keys less than the node’s key, and the right subtree only nodes with keys greater than the node’s key.
- AVL Trees: Self-balancing binary search trees where the heights of the two child subtrees of any node differ by at most one.
- Red-Black Trees: Another type of self-balancing binary search tree with additional properties that ensure the tree remains balanced after insertions and deletions.
- N-ary Trees: Trees where nodes can have any number of children.
Tree Representation in Code
In most programming languages, trees are represented using custom node classes or structures. Here’s a simple example of a binary tree node in Python:
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
This representation allows us to create and manipulate tree structures easily.
2. Recursion Basics: The Foundation of Tree Traversal
Recursion is a programming technique where a function calls itself to solve a problem. It’s particularly well-suited for tree problems due to the recursive nature of tree structures themselves.
The Anatomy of a Recursive Function
A typical recursive function consists of two main parts:
- Base case: The condition that stops the recursion.
- Recursive case: The part where the function calls itself with a modified input.
Here’s a simple example of a recursive function that calculates the factorial of a number:
def factorial(n):
# Base case
if n == 0 or n == 1:
return 1
# Recursive case
return n * factorial(n - 1)
Tree Traversal Using Recursion
Recursion is particularly useful for traversing trees. There are three main types of depth-first traversals:
- Inorder Traversal: Left subtree, Root, Right subtree
- Preorder Traversal: Root, Left subtree, Right subtree
- Postorder Traversal: Left subtree, Right subtree, Root
Here’s an example of an inorder traversal using recursion:
def inorder_traversal(root):
if root is None:
return
inorder_traversal(root.left)
print(root.val)
inorder_traversal(root.right)
This simple function demonstrates the power of recursion in handling tree structures. It naturally follows the tree’s hierarchical structure, making the code concise and easy to understand.
3. Common Recursive Tree Problems
Now that we’ve covered the basics, let’s explore some common tree problems that can be solved using recursion.
Problem 1: Maximum Depth of a Binary Tree
Finding the maximum depth (or height) of a binary tree is a classic problem that can be elegantly solved using recursion.
def max_depth(root):
if root is None:
return 0
left_depth = max_depth(root.left)
right_depth = max_depth(root.right)
return max(left_depth, right_depth) + 1
This function recursively calculates the depth of the left and right subtrees and returns the maximum of the two, plus one for the current node.
Problem 2: Check if a Binary Tree is Balanced
A balanced binary tree is one where the heights of the two subtrees of any node never differ by more than one. Here’s a recursive solution:
def is_balanced(root):
def check_balance(node):
if node is None:
return 0
left = check_balance(node.left)
right = check_balance(node.right)
if left == -1 or right == -1 or abs(left - right) > 1:
return -1
return max(left, right) + 1
return check_balance(root) != -1
This solution uses a helper function that returns -1 if the tree is unbalanced, and the height of the tree otherwise.
Problem 3: Lowest Common Ancestor in a Binary Tree
Finding the lowest common ancestor (LCA) of two nodes in a binary tree is another problem that lends itself well to a recursive solution.
def lowest_common_ancestor(root, p, q):
if root is None or root == p or root == q:
return root
left = lowest_common_ancestor(root.left, p, q)
right = lowest_common_ancestor(root.right, p, q)
if left and right:
return root
return left if left else right
This function recursively searches for the nodes p and q in the left and right subtrees. The node where both p and q are found in different subtrees (or one is the node itself) is the LCA.
4. Advanced Techniques for Complex Tree Problems
As we delve deeper into tree problems, we encounter more complex scenarios that require advanced recursive techniques.
Path Sum Problems
Path sum problems involve finding paths in a tree that sum to a particular value. These problems often require maintaining state during recursion.
def has_path_sum(root, target_sum):
if root is None:
return False
if root.left is None and root.right is None:
return root.val == target_sum
return has_path_sum(root.left, target_sum - root.val) or \
has_path_sum(root.right, target_sum - root.val)
This function checks if there’s a root-to-leaf path with a sum equal to the target sum.
Tree Serialization and Deserialization
Serialization is the process of converting a data structure into a format that can be easily stored or transmitted. Deserialization is the reverse process. These operations on trees often involve recursive algorithms.
class Codec:
def serialize(self, root):
if not root:
return "null"
return f"{root.val},{self.serialize(root.left)},{self.serialize(root.right)}"
def deserialize(self, data):
def dfs():
val = next(values)
if val == "null":
return None
node = TreeNode(int(val))
node.left = dfs()
node.right = dfs()
return node
values = iter(data.split(','))
return dfs()
This code demonstrates how to serialize a binary tree into a string and then deserialize it back into a tree structure.
Tree Construction from Traversals
Constructing a binary tree from its traversals is a more complex problem that requires careful use of recursion.
def build_tree(preorder, inorder):
if not preorder or not inorder:
return None
root = TreeNode(preorder[0])
mid = inorder.index(preorder[0])
root.left = build_tree(preorder[1:mid+1], inorder[:mid])
root.right = build_tree(preorder[mid+1:], inorder[mid+1:])
return root
This function builds a binary tree given its preorder and inorder traversals.
5. Optimization and Performance Considerations
While recursion is elegant and intuitive for tree problems, it’s important to consider performance and optimization techniques.
Tail Recursion
Tail recursion is a special case of recursion where the recursive call is the last operation in the function. Some compilers can optimize tail-recursive functions to use constant stack space.
def tail_recursive_factorial(n, acc=1):
if n == 0:
return acc
return tail_recursive_factorial(n - 1, n * acc)
While Python doesn’t optimize for tail recursion, languages like Scala do, making this an important technique to know.
Memoization
Memoization involves caching the results of expensive function calls to avoid redundant computations. This can significantly improve performance for certain tree problems.
from functools import lru_cache
@lru_cache(maxsize=None)
def fibonacci(n):
if n < 2:
return n
return fibonacci(n-1) + fibonacci(n-2)
The @lru_cache
decorator in Python implements memoization, dramatically improving the performance of the recursive Fibonacci function.
Iterative Solutions
In some cases, an iterative solution might be more efficient than a recursive one. For example, tree traversal can be done iteratively using a stack:
def inorder_traversal_iterative(root):
result = []
stack = []
current = root
while current or stack:
while current:
stack.append(current)
current = current.left
current = stack.pop()
result.append(current.val)
current = current.right
return result
This iterative solution avoids the overhead of multiple function calls and potential stack overflow for very deep trees.
6. Real-World Applications of Recursive Tree Algorithms
Recursive tree algorithms find applications in various domains of computer science and software development:
File System Operations
File systems are inherently tree-like structures. Operations like calculating directory size or searching for files often use recursive algorithms.
import os
def get_directory_size(path):
total = 0
for dirpath, dirnames, filenames in os.walk(path):
for f in filenames:
fp = os.path.join(dirpath, f)
total += os.path.getsize(fp)
return total
HTML DOM Manipulation
The Document Object Model (DOM) in web browsers is a tree structure. Many DOM manipulation tasks involve recursive traversal and modification of this tree.
function highlightKeywords(element, keywords) {
if (element.nodeType === Node.TEXT_NODE) {
const parent = element.parentNode;
const text = element.textContent;
const parts = text.split(new RegExp(`(${keywords.join('|')})`, 'gi'));
const fragment = document.createDocumentFragment();
parts.forEach(part => {
if (keywords.some(keyword => part.toLowerCase() === keyword.toLowerCase())) {
const span = document.createElement('span');
span.className = 'highlight';
span.textContent = part;
fragment.appendChild(span);
} else {
fragment.appendChild(document.createTextNode(part));
}
});
parent.replaceChild(fragment, element);
} else {
Array.from(element.childNodes).forEach(child => highlightKeywords(child, keywords));
}
}
Abstract Syntax Trees in Compilers
Compilers and interpreters often represent program structure as Abstract Syntax Trees (ASTs). These trees are traversed and manipulated using recursive algorithms during various stages of compilation or interpretation.
Decision Trees in Machine Learning
Decision trees, a popular machine learning model, are another example of tree structures. Training and using these models often involves recursive algorithms.
class DecisionNode:
def __init__(self, feature_index=None, threshold=None, left=None, right=None, value=None):
self.feature_index = feature_index
self.threshold = threshold
self.left = left
self.right = right
self.value = value
def predict(node, x):
if node.value is not None:
return node.value
if x[node.feature_index] <= node.threshold:
return predict(node.left, x)
else:
return predict(node.right, x)
7. Conclusion
Mastering recursive tree problems is a crucial skill for any programmer or computer scientist. The techniques and patterns we’ve explored in this guide form the foundation for solving a wide range of tree-based challenges, from basic traversals to complex tree manipulations.
Remember, while recursion often provides elegant and intuitive solutions to tree problems, it’s important to consider performance implications, especially for large trees. In some cases, iterative solutions or optimizations like memoization may be necessary.
As you continue to practice and encounter more tree problems, you’ll develop a intuition for when and how to apply recursive techniques effectively. Keep exploring, keep coding, and embrace the beauty and power of recursive tree algorithms!
Happy coding!