How to Handle Queue-based Level Order Traversal: A Comprehensive Guide
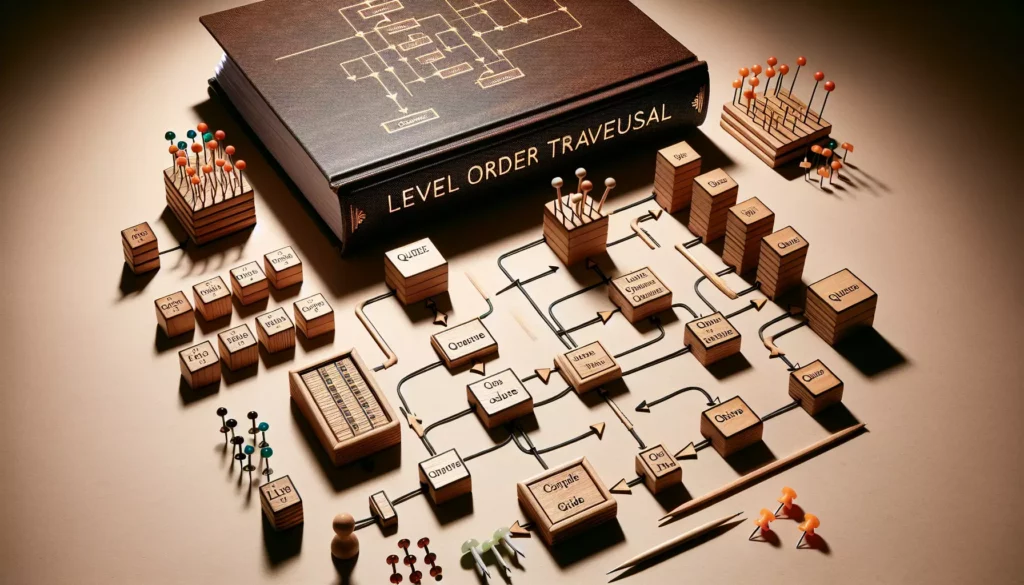
In the world of computer science and programming, tree data structures play a crucial role in organizing and representing hierarchical information. One of the fundamental operations performed on trees is traversal, which involves visiting each node in the tree in a specific order. Among the various traversal techniques, level order traversal stands out as a particularly useful method, especially when dealing with binary trees or more general tree structures.
In this comprehensive guide, we’ll dive deep into the concept of queue-based level order traversal, exploring its implementation, applications, and various problem-solving scenarios. Whether you’re a beginner looking to understand tree traversals or an experienced programmer preparing for technical interviews at top tech companies, this article will provide valuable insights and practical knowledge to enhance your coding skills.
Table of Contents
- Understanding Level Order Traversal
- The Queue-based Approach
- Implementing Queue-based Level Order Traversal
- Variations and Advanced Techniques
- Real-world Applications
- Common Interview Questions
- Optimization Tips and Best Practices
- Conclusion
1. Understanding Level Order Traversal
Before we delve into the queue-based approach, let’s first understand what level order traversal is and why it’s important.
What is Level Order Traversal?
Level order traversal, also known as breadth-first traversal, is a method of visiting all nodes in a tree structure level by level, from left to right. This means we visit all nodes at depth 1, then all nodes at depth 2, and so on, until we’ve covered all levels of the tree.
For example, consider the following binary tree:
1
/ \
2 3
/ \ / \
4 5 6 7
The level order traversal of this tree would be: 1, 2, 3, 4, 5, 6, 7
Why is Level Order Traversal Important?
Level order traversal is crucial for several reasons:
- Hierarchical Processing: It allows us to process tree data in a hierarchical manner, which is useful in many real-world scenarios like organization charts or file systems.
- Shortest Path Problems: In graph theory, level order traversal (or breadth-first search) is used to find the shortest path between two nodes.
- Tree Serialization: It’s often used in serializing and deserializing tree structures, which is important for data storage and transmission.
- Level-specific Operations: Some problems require performing operations on specific levels of a tree, which is easily achievable with level order traversal.
2. The Queue-based Approach
Now that we understand the concept of level order traversal, let’s explore the queue-based approach, which is the most efficient and widely used method for implementing this traversal.
Why Use a Queue?
A queue is a perfect data structure for level order traversal because of its First-In-First-Out (FIFO) property. This property ensures that we process nodes in the order they are discovered, which naturally aligns with the level-by-level traversal we want to achieve.
The Basic Algorithm
Here’s a high-level overview of the queue-based level order traversal algorithm:
- Create an empty queue and enqueue the root node.
- While the queue is not empty:
- Dequeue a node from the front of the queue.
- Process the dequeued node (e.g., print its value).
- Enqueue the left child of the dequeued node if it exists.
- Enqueue the right child of the dequeued node if it exists.
- Repeat step 2 until the queue is empty.
This algorithm ensures that we visit all nodes at a given level before moving to the next level, thus achieving the level order traversal.
3. Implementing Queue-based Level Order Traversal
Let’s implement the queue-based level order traversal in Python. We’ll start with a basic implementation and then explore some variations.
Basic Implementation
First, let’s define a simple binary tree node structure:
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
Now, let’s implement the level order traversal function:
from collections import deque
def levelOrderTraversal(root):
if not root:
return []
result = []
queue = deque([root])
while queue:
level_size = len(queue)
current_level = []
for _ in range(level_size):
node = queue.popleft()
current_level.append(node.val)
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
result.append(current_level)
return result
This implementation does the following:
- We use Python’s
deque
class for efficient queue operations. - We start by checking if the root is null. If so, we return an empty list.
- We initialize the queue with the root node.
- We use a while loop to process nodes level by level until the queue is empty.
- For each level, we process all nodes currently in the queue (which represents the current level).
- We add the children of each processed node to the queue for the next level.
- We append each level’s values to the result list.
Using the Implementation
Let’s see how to use this implementation with an example:
# Create a sample binary tree
root = TreeNode(1)
root.left = TreeNode(2)
root.right = TreeNode(3)
root.left.left = TreeNode(4)
root.left.right = TreeNode(5)
root.right.left = TreeNode(6)
root.right.right = TreeNode(7)
# Perform level order traversal
result = levelOrderTraversal(root)
print(result) # Output: [[1], [2, 3], [4, 5, 6, 7]]
This will output the level order traversal of the tree, with each inner list representing a level of the tree.
4. Variations and Advanced Techniques
The basic implementation we’ve seen is just the beginning. There are several variations and advanced techniques that build upon this concept:
Zigzag Level Order Traversal
In this variation, we traverse the tree in a zigzag order. Odd-numbered levels are traversed left to right, while even-numbered levels are traversed right to left.
def zigzagLevelOrder(root):
if not root:
return []
result = []
queue = deque([root])
left_to_right = True
while queue:
level_size = len(queue)
current_level = deque()
for _ in range(level_size):
node = queue.popleft()
if left_to_right:
current_level.append(node.val)
else:
current_level.appendleft(node.val)
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
result.append(list(current_level))
left_to_right = not left_to_right
return result
Level Order Traversal II (Bottom-Up)
This variation returns the level order traversal from bottom to top.
def levelOrderBottom(root):
if not root:
return []
result = []
queue = deque([root])
while queue:
level_size = len(queue)
current_level = []
for _ in range(level_size):
node = queue.popleft()
current_level.append(node.val)
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
result.append(current_level)
return result[::-1] # Reverse the result
Average of Levels in Binary Tree
This problem requires calculating the average value of nodes on each level.
def averageOfLevels(root):
if not root:
return []
result = []
queue = deque([root])
while queue:
level_size = len(queue)
level_sum = 0
for _ in range(level_size):
node = queue.popleft()
level_sum += node.val
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
result.append(level_sum / level_size)
return result
5. Real-world Applications
Queue-based level order traversal has numerous real-world applications across various domains of computer science and software development:
1. File System Traversal
When exploring directory structures, level order traversal can be used to list all files and subdirectories at each level of the file system hierarchy.
2. Network Routing
In computer networks, breadth-first search (which uses level order traversal) is used in routing algorithms to find the shortest path between network nodes.
3. Web Crawling
Web crawlers often use level order traversal to explore web pages. Starting from a seed URL, they visit linked pages level by level, which can be useful for search engines or web scraping applications.
4. Game AI
In game development, level order traversal can be used in AI algorithms for pathfinding or exploring game states in strategy games.
5. Social Network Analysis
When analyzing social networks, level order traversal can be used to find connections or explore relationships between users at different degrees of separation.
6. XML/HTML Parsing
When parsing hierarchical data formats like XML or HTML, level order traversal can be useful for processing elements level by level.
6. Common Interview Questions
Level order traversal is a popular topic in technical interviews, especially for positions at top tech companies. Here are some common interview questions related to this topic:
1. Binary Tree Right Side View
Given a binary tree, imagine you’re standing on the right side of it. Return the values of the nodes you can see ordered from top to bottom.
def rightSideView(root):
if not root:
return []
result = []
queue = deque([root])
while queue:
level_size = len(queue)
for i in range(level_size):
node = queue.popleft()
if i == level_size - 1:
result.append(node.val)
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
return result
2. Populating Next Right Pointers in Each Node
You are given a perfect binary tree where all leaves are on the same level, and every parent has two children. Populate each next pointer to point to its next right node. If there is no next right node, the next pointer should be set to NULL.
def connect(root):
if not root:
return root
queue = deque([root])
while queue:
level_size = len(queue)
for i in range(level_size):
node = queue.popleft()
if i < level_size - 1:
node.next = queue[0]
else:
node.next = None
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
return root
3. Binary Tree Level Order Traversal II
Given a binary tree, return the bottom-up level order traversal of its nodes’ values. (i.e., from left to right, level by level from leaf to root).
def levelOrderBottom(root):
if not root:
return []
result = []
queue = deque([root])
while queue:
level_size = len(queue)
current_level = []
for _ in range(level_size):
node = queue.popleft()
current_level.append(node.val)
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
result.append(current_level)
return result[::-1]
7. Optimization Tips and Best Practices
When working with queue-based level order traversal, consider these optimization tips and best practices:
1. Use Efficient Queue Implementations
In Python, use collections.deque
instead of a list for queue operations. Deque provides O(1) time complexity for append and pop operations at both ends.
2. Avoid Unnecessary Queue Operations
In some cases, you can reduce the number of queue operations by processing nodes in batches (level by level) rather than one at a time.
3. Consider Space Complexity
For very large trees, consider using iterative deepening depth-first search (IDDFS) if memory is a concern, as it uses less space than a queue-based approach.
4. Optimize for Specific Problems
For problems that only require information about the last node in each level (like the right side view problem), you can optimize by only keeping track of the last node processed in each level.
5. Use Sentinel Nodes
For problems that require level separation, consider using sentinel nodes in the queue to mark the end of each level, which can simplify the logic in some cases.
6. Combine with Other Techniques
Sometimes, combining level order traversal with other techniques like hash maps or dynamic programming can lead to more efficient solutions for complex problems.
8. Conclusion
Queue-based level order traversal is a powerful technique that forms the foundation for solving many tree-related problems. Its applications span various domains in computer science and software development, making it an essential skill for any programmer, especially those preparing for technical interviews at top tech companies.
By mastering this technique and its variations, you’ll be well-equipped to tackle a wide range of problems involving tree structures. Remember to practice regularly, explore different problem variations, and always consider the specific requirements of each problem to choose the most appropriate approach.
As you continue your journey in programming and algorithm mastery, keep exploring advanced tree traversal techniques and their applications. The skills you develop in this area will serve you well in your coding career and help you stand out in technical interviews.
Happy coding, and may your tree traversals always be efficient and bug-free!