How to Handle Overlapping Subproblems: Mastering Dynamic Programming
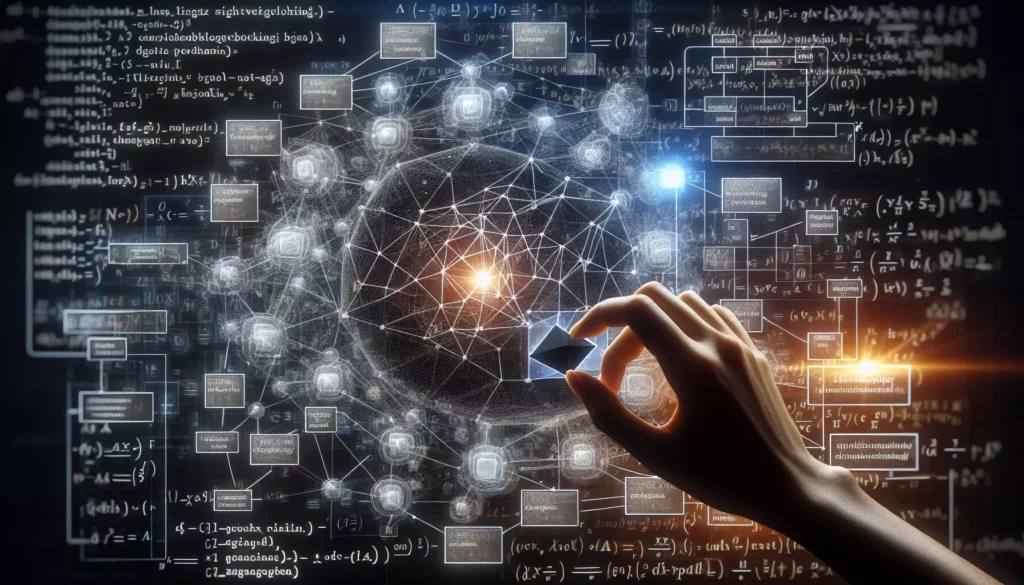
In the world of algorithmic problem-solving, efficiency is key. As aspiring programmers and seasoned developers alike strive to optimize their code, they often encounter a powerful technique known as dynamic programming. At the heart of this approach lies the concept of overlapping subproblems, a phenomenon that, when properly addressed, can lead to significant performance improvements in your algorithms. In this comprehensive guide, we’ll dive deep into the world of overlapping subproblems, exploring what they are, why they matter, and most importantly, how to handle them effectively.
Understanding Overlapping Subproblems
Before we delve into the techniques for handling overlapping subproblems, it’s crucial to understand what they are and why they occur. In essence, overlapping subproblems are a characteristic of certain computational problems where the same smaller instances are encountered multiple times during the problem-solving process.
What Are Overlapping Subproblems?
Overlapping subproblems occur when a recursive algorithm repeatedly solves the same smaller subproblems as part of solving the larger problem. This repetition can lead to unnecessary computations, significantly impacting the efficiency of your algorithm.
To illustrate this concept, let’s consider the classic example of calculating Fibonacci numbers:
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n-1) + fibonacci(n-2)
In this naive recursive implementation, as we calculate higher Fibonacci numbers, we end up recomputing the same values multiple times. For instance, to calculate fibonacci(5), we need to calculate fibonacci(4) and fibonacci(3). But to calculate fibonacci(4), we again need fibonacci(3) and fibonacci(2), leading to redundant calculations.
Why Do Overlapping Subproblems Matter?
The presence of overlapping subproblems can have a significant impact on the time complexity of your algorithm. In the Fibonacci example above, the time complexity grows exponentially with n, making it highly inefficient for larger inputs. By recognizing and addressing overlapping subproblems, we can dramatically improve the performance of our algorithms, often reducing time complexity from exponential to polynomial.
Identifying Overlapping Subproblems
The first step in handling overlapping subproblems is being able to identify them in the problems you’re trying to solve. Here are some key indicators that you might be dealing with overlapping subproblems:
- Recursive Solutions: If your initial approach to solving a problem involves recursion, there’s a good chance you’ll encounter overlapping subproblems.
- Repetitive Calculations: If you notice that your algorithm is performing the same calculations multiple times, it’s a clear sign of overlapping subproblems.
- Optimal Substructure: Problems with optimal substructure (where the optimal solution to a problem can be constructed from optimal solutions of its subproblems) often exhibit overlapping subproblems.
- State-Dependent Problems: If the solution to a subproblem depends on a small set of parameters or states, and these states are revisited multiple times, you’re likely dealing with overlapping subproblems.
Some common problems that exhibit overlapping subproblems include:
- Fibonacci Sequence
- Longest Common Subsequence
- Matrix Chain Multiplication
- Shortest Path Problems (e.g., Floyd-Warshall algorithm)
- Knapsack Problem
Techniques for Handling Overlapping Subproblems
Now that we understand what overlapping subproblems are and how to identify them, let’s explore the primary techniques for handling them effectively:
1. Memoization (Top-Down Approach)
Memoization is a top-down approach to dynamic programming where we store the results of expensive function calls and return the cached result when the same inputs occur again. This technique is particularly useful when we don’t need to solve all subproblems and can avoid unnecessary calculations.
Let’s apply memoization to our Fibonacci example:
def fibonacci_memo(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci_memo(n-1, memo) + fibonacci_memo(n-2, memo)
return memo[n]
In this implementation, we use a dictionary (memo) to store the results of previously computed Fibonacci numbers. Before calculating a number, we check if it’s already in our memo. If it is, we return the stored value, avoiding redundant calculations.
2. Tabulation (Bottom-Up Approach)
Tabulation is a bottom-up approach where we solve all related subproblems first, typically by filling up an n-dimensional table. We then use these results to solve the larger problem. This approach is usually more efficient in terms of space complexity and can be easier to implement iteratively.
Here’s how we can solve the Fibonacci problem using tabulation:
def fibonacci_tab(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
In this approach, we build up our solution iteratively, storing all intermediate results in an array. This eliminates the need for recursion and ensures that each subproblem is solved only once.
3. State Reduction
In some cases, we can optimize our solution further by reducing the state we need to keep track of. This is particularly useful when we only need a small window of previous results to compute the next one.
For the Fibonacci sequence, we can reduce our state to just two variables:
def fibonacci_optimized(n):
if n <= 1:
return n
a, b = 0, 1
for _ in range(2, n + 1):
a, b = b, a + b
return b
This approach maintains the same time complexity as the tabulation method but reduces the space complexity from O(n) to O(1).
Advanced Techniques and Considerations
While memoization and tabulation are the primary techniques for handling overlapping subproblems, there are several advanced considerations and techniques to keep in mind:
1. Space-Time Tradeoffs
When dealing with overlapping subproblems, we’re often trading space for time. By storing results of subproblems, we’re using additional memory to reduce computation time. In some cases, especially with large inputs or constrained memory environments, you might need to balance between time and space efficiency.
2. Iterative vs. Recursive Implementations
While memoization typically uses a recursive approach and tabulation an iterative one, it’s possible to implement both techniques either recursively or iteratively. The choice often depends on the specific problem and personal preference. Iterative solutions generally have better space complexity (due to lack of call stack overhead) and can be more efficient in practice.
3. Multi-dimensional Problems
Many real-world problems involve multiple parameters or dimensions. In such cases, our memoization or tabulation strategies need to account for these multiple dimensions. For example, in the Knapsack problem, we might use a 2D array to store results based on both the item index and remaining capacity.
4. State Compression
In some problems, especially those involving bitmasks or large state spaces, we can use techniques like state compression to reduce memory usage. This involves encoding the state information more efficiently, often using bitwise operations.
5. Rolling Array Technique
For problems where we only need the results from the last few states, we can use a rolling array technique. This involves using modulo arithmetic to reuse a small fixed-size array, effectively “rolling” over previous results. This can significantly reduce space complexity in certain problems.
Common Pitfalls and How to Avoid Them
As you work on mastering the handling of overlapping subproblems, be aware of these common pitfalls:
1. Incorrect Memoization Keys
When using memoization, ensure that your memoization key fully captures the state of the subproblem. Missing a crucial parameter can lead to incorrect results. Always double-check that your memoization key is unique for each distinct subproblem.
2. Unnecessary Memoization
Not all recursive problems benefit from memoization. If a problem doesn’t have overlapping subproblems, adding memoization can actually slow down your solution due to the overhead of maintaining the memo structure. Analyze the problem carefully to determine if memoization is necessary.
3. Inefficient State Representation
Choose your state representation wisely. An overly complex state can lead to excessive memory usage and slower access times. Strive for a minimal state representation that captures all necessary information.
4. Ignoring Base Cases
When implementing recursive solutions with memoization, don’t forget to handle base cases before checking the memo. Failing to do so can lead to infinite recursion or incorrect results.
5. Overcomplicating Simple Problems
While dynamic programming is a powerful technique, it’s not always the best solution. For simple problems or small input sizes, a straightforward implementation might be more readable and maintainable. Always consider the trade-offs between complexity and performance.
Real-World Applications
Understanding how to handle overlapping subproblems isn’t just an academic exercise. This skill has numerous real-world applications across various domains of computer science and software engineering:
1. Bioinformatics
Sequence alignment algorithms, crucial in genomics and proteomics, often rely on dynamic programming techniques to handle overlapping subproblems efficiently. The Smith-Waterman algorithm for local sequence alignment is a prime example.
2. Financial Modeling
Options pricing models, like the Black-Scholes model, use dynamic programming to efficiently calculate option values, handling the overlapping subproblems that arise in binomial tree models.
3. Natural Language Processing
Many NLP tasks, such as parsing and machine translation, use dynamic programming algorithms to handle the overlapping subproblems in processing language structures.
4. Computer Graphics
Certain rendering algorithms and image processing techniques use dynamic programming to efficiently compute results by avoiding redundant calculations of pixel or vertex data.
5. Network Routing
Routing algorithms in computer networks often use dynamic programming approaches to find optimal paths while handling the overlapping subproblems inherent in network traversal.
Practice Problems
To truly master the art of handling overlapping subproblems, practice is essential. Here are some problems you can work on to hone your skills:
- Longest Common Subsequence: Given two strings, find the length of their longest common subsequence.
- 0/1 Knapsack Problem: Given a set of items with weights and values, determine the most valuable combination of items that can be carried in a knapsack of fixed capacity.
- Coin Change Problem: Given a set of coin denominations and a target amount, find the minimum number of coins needed to make up that amount.
- Edit Distance: Given two strings, compute the minimum number of operations required to convert one string into the other.
- Longest Increasing Subsequence: Given an array of integers, find the length of the longest subsequence such that all elements of the subsequence are sorted in ascending order.
For each of these problems, try implementing both memoization and tabulation approaches. Analyze the time and space complexity of your solutions, and consider how you might optimize them further.
Conclusion
Mastering the art of handling overlapping subproblems is a crucial skill for any programmer looking to write efficient and optimized code. By understanding the concepts of memoization, tabulation, and state reduction, you’ll be well-equipped to tackle a wide range of algorithmic challenges.
Remember, the key to success lies not just in knowing these techniques, but in practicing them regularly and applying them judiciously. As you encounter new problems, always be on the lookout for opportunities to optimize your solutions by identifying and efficiently handling overlapping subproblems.
Whether you’re preparing for technical interviews, working on complex software systems, or simply striving to become a better programmer, your ability to handle overlapping subproblems will serve you well. So keep practicing, stay curious, and never stop optimizing!