How to Handle Multiple Pointers Efficiently: A Comprehensive Guide
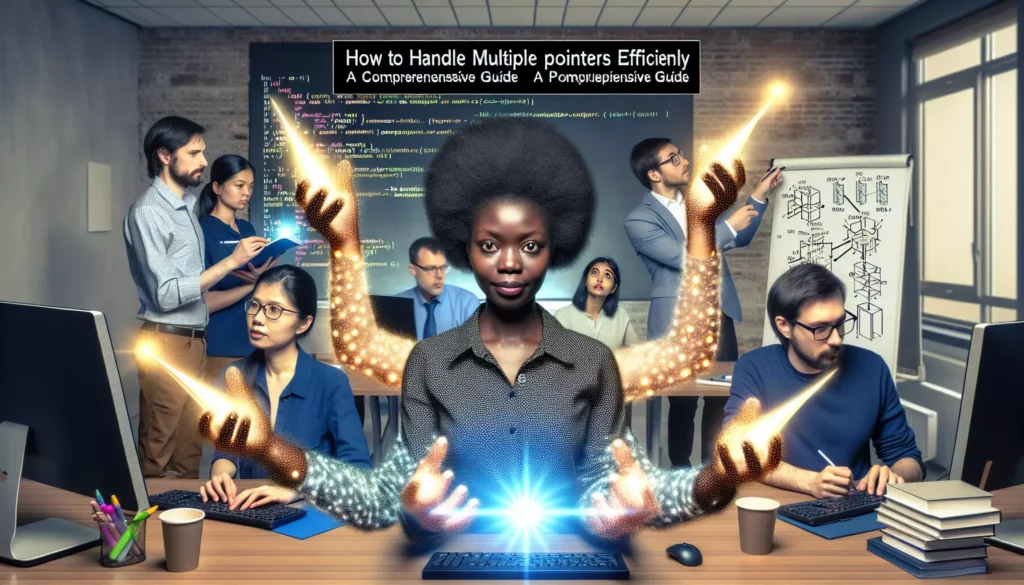
In the world of programming and algorithmic problem-solving, the ability to handle multiple pointers efficiently is a crucial skill. Whether you’re preparing for technical interviews at top tech companies or simply looking to improve your coding prowess, mastering this technique can significantly enhance your problem-solving capabilities. In this comprehensive guide, we’ll dive deep into the concept of multiple pointers, explore various scenarios where they’re useful, and provide practical examples to help you grasp this important programming paradigm.
Table of Contents
- Introduction to Multiple Pointers
- When to Use Multiple Pointers
- Common Multiple Pointer Patterns
- Practical Examples and Solutions
- Optimizing Multiple Pointer Algorithms
- Interview Tips for Multiple Pointer Questions
- Advanced Techniques and Edge Cases
- Conclusion
1. Introduction to Multiple Pointers
Multiple pointers is a technique where you use two or more pointers to traverse through a data structure, typically an array or a linked list. These pointers move through the structure at different speeds or in different directions, allowing you to solve problems more efficiently than with a single pointer or nested loops.
The key advantage of using multiple pointers is that it often allows you to solve problems in linear time (O(n)) that might otherwise require quadratic time (O(n^2)) with naive approaches. This efficiency gain is particularly important when dealing with large datasets or when optimizing for performance in technical interviews.
2. When to Use Multiple Pointers
Multiple pointers are particularly useful in the following scenarios:
- Searching for pairs in a sorted array
- Detecting cycles in linked lists
- Reversing arrays or linked lists in-place
- Finding substrings or subarrays with specific properties
- Partitioning arrays based on certain conditions
- Merging sorted arrays or linked lists
When you encounter problems that involve searching, comparing, or manipulating elements in a linear data structure, consider whether multiple pointers could provide an efficient solution.
3. Common Multiple Pointer Patterns
There are several common patterns when working with multiple pointers:
3.1. Two Pointers Moving in Opposite Directions
This pattern is often used for problems involving palindromes, reversing arrays, or finding pairs with a specific sum in a sorted array.
3.2. Fast and Slow Pointers
Also known as the “tortoise and hare” algorithm, this pattern is useful for detecting cycles in linked lists or finding the middle element of a linked list.
3.3. Two Pointers Moving at Different Speeds
This pattern can be used to find a specific element or to partition an array based on certain conditions.
3.4. Multiple Pointers for Partitioning
This pattern is often used in sorting algorithms like quicksort or for problems that require partitioning an array into multiple sections.
4. Practical Examples and Solutions
Let’s explore some practical examples of using multiple pointers to solve common coding problems.
4.1. Two Sum Problem (Sorted Array)
Given a sorted array of integers and a target sum, find two numbers such that they add up to the target sum.
def two_sum(nums, target):
left, right = 0, len(nums) - 1
while left < right:
current_sum = nums[left] + nums[right]
if current_sum == target:
return [nums[left], nums[right]]
elif current_sum < target:
left += 1
else:
right -= 1
return [] # No solution found
# Example usage
nums = [2, 7, 11, 15]
target = 9
result = two_sum(nums, target)
print(result) # Output: [2, 7]
In this example, we use two pointers starting from opposite ends of the sorted array. We move the pointers based on whether the current sum is less than, equal to, or greater than the target sum.
4.2. Detect Cycle in a Linked List
Determine if a linked list has a cycle using the fast and slow pointer technique.
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def has_cycle(head):
if not head or not head.next:
return False
slow = head
fast = head.next
while slow != fast:
if not fast or not fast.next:
return False
slow = slow.next
fast = fast.next.next
return True
# Example usage
# Create a linked list with a cycle
node1 = ListNode(1)
node2 = ListNode(2)
node3 = ListNode(3)
node4 = ListNode(4)
node1.next = node2
node2.next = node3
node3.next = node4
node4.next = node2 # Create a cycle
result = has_cycle(node1)
print(result) # Output: True
This algorithm uses the “tortoise and hare” approach, where the slow pointer moves one step at a time, and the fast pointer moves two steps. If there’s a cycle, the fast pointer will eventually catch up to the slow pointer.
4.3. Remove Duplicates from Sorted Array
Remove duplicates from a sorted array in-place and return the new length.
def remove_duplicates(nums):
if not nums:
return 0
# Pointer for the position to place the next unique element
write_pointer = 1
# Pointer for iterating through the array
for read_pointer in range(1, len(nums)):
if nums[read_pointer] != nums[read_pointer - 1]:
nums[write_pointer] = nums[read_pointer]
write_pointer += 1
return write_pointer
# Example usage
nums = [1, 1, 2, 2, 3, 4, 4, 5]
new_length = remove_duplicates(nums)
print(new_length) # Output: 5
print(nums[:new_length]) # Output: [1, 2, 3, 4, 5]
In this example, we use two pointers: a write pointer to keep track of where to place the next unique element, and a read pointer to iterate through the array.
5. Optimizing Multiple Pointer Algorithms
When working with multiple pointers, consider the following optimization techniques:
5.1. Minimize Pointer Movement
Try to move pointers as little as possible. In some cases, you can achieve the desired result by moving only one pointer at a time.
5.2. Use Appropriate Data Structures
Choose the right data structure for your problem. For example, if you need constant-time access to elements, an array might be better than a linked list.
5.3. Avoid Unnecessary Comparisons
Look for opportunities to reduce the number of comparisons or operations performed in each iteration.
5.4. Consider Edge Cases
Always consider edge cases, such as empty arrays, single-element arrays, or when pointers meet at the ends of the data structure.
6. Interview Tips for Multiple Pointer Questions
When tackling multiple pointer problems in technical interviews, keep these tips in mind:
- Clarify the problem and constraints before starting
- Think out loud and explain your thought process
- Start with a simple, brute-force approach if you’re stuck
- Analyze the time and space complexity of your solution
- Test your solution with various input cases, including edge cases
- Be prepared to optimize your initial solution
7. Advanced Techniques and Edge Cases
As you become more comfortable with multiple pointers, you can explore more advanced techniques and tackle more complex problems.
7.1. Three Pointers
Some problems may require the use of three pointers. For example, the “3Sum” problem, where you need to find triplets in an array that sum to a target value.
def three_sum(nums, target):
nums.sort()
result = []
for i in range(len(nums) - 2):
if i > 0 and nums[i] == nums[i - 1]:
continue
left, right = i + 1, len(nums) - 1
while left < right:
current_sum = nums[i] + nums[left] + nums[right]
if current_sum == target:
result.append([nums[i], nums[left], nums[right]])
while left < right and nums[left] == nums[left + 1]:
left += 1
while left < right and nums[right] == nums[right - 1]:
right -= 1
left += 1
right -= 1
elif current_sum < target:
left += 1
else:
right -= 1
return result
# Example usage
nums = [-1, 0, 1, 2, -1, -4]
target = 0
result = three_sum(nums, target)
print(result) # Output: [[-1, -1, 2], [-1, 0, 1]]
7.2. Sliding Window Technique
The sliding window technique is a variation of the multiple pointer approach, often used for problems involving subarrays or substrings.
def max_sum_subarray(nums, k):
if len(nums) < k:
return None
window_sum = sum(nums[:k])
max_sum = window_sum
for i in range(k, len(nums)):
window_sum = window_sum - nums[i - k] + nums[i]
max_sum = max(max_sum, window_sum)
return max_sum
# Example usage
nums = [1, 4, 2, 10, 23, 3, 1, 0, 20]
k = 4
result = max_sum_subarray(nums, k)
print(result) # Output: 39
7.3. Dealing with Circular Arrays
When working with circular arrays, you may need to use the modulo operator to wrap around the array.
def find_rotation_point(arr):
left, right = 0, len(arr) - 1
while left <= right:
if arr[left] <= arr[right]:
return left
mid = (left + right) // 2
next_mid = (mid + 1) % len(arr)
if arr[mid] > arr[next_mid]:
return next_mid
elif arr[left] <= arr[mid]:
left = mid + 1
else:
right = mid - 1
return 0 # Array is not rotated
# Example usage
rotated_array = [4, 5, 6, 7, 0, 1, 2]
rotation_point = find_rotation_point(rotated_array)
print(rotation_point) # Output: 4
8. Conclusion
Mastering the multiple pointer technique is essential for efficient problem-solving in programming. By understanding when and how to apply this technique, you can significantly improve your ability to tackle a wide range of algorithmic challenges.
Remember that practice is key to becoming proficient with multiple pointers. As you work through more problems, you’ll develop an intuition for when to use this technique and how to implement it effectively. Keep challenging yourself with diverse problems, and don’t hesitate to revisit and optimize your solutions.
Whether you’re preparing for technical interviews at top tech companies or simply aiming to enhance your coding skills, the multiple pointer technique will serve as a powerful tool in your programming toolkit. Happy coding!