How to Handle Multiple Edge Cases Efficiently in Programming
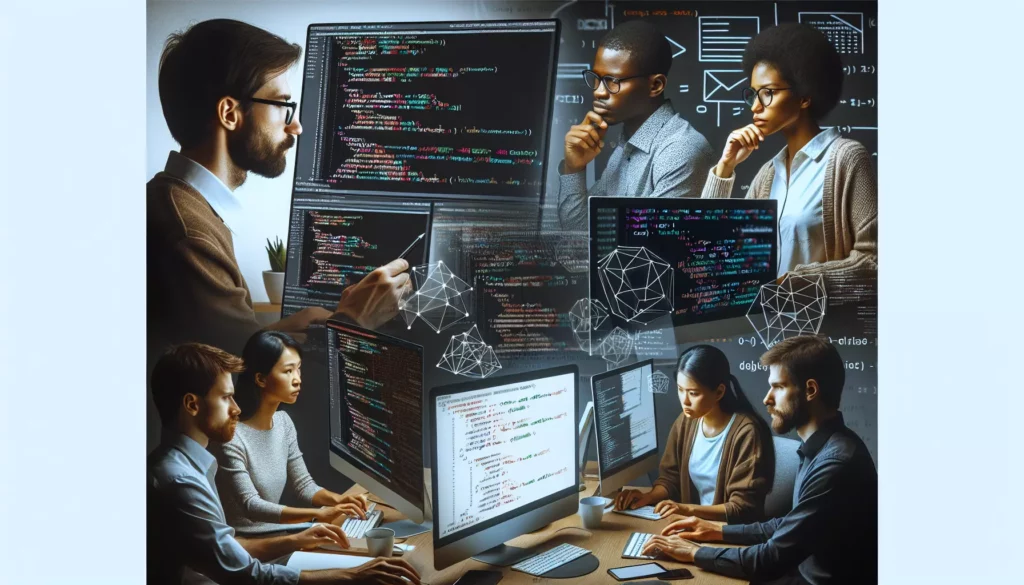
In the world of programming, handling edge cases is a crucial skill that separates novice developers from seasoned professionals. Edge cases are those unexpected or unusual situations that can occur in your code, often at the extremes of your input ranges or in scenarios you might not have initially considered. When you’re dealing with multiple edge cases, the complexity of your code can increase rapidly, potentially leading to bugs, decreased performance, and maintenance nightmares. In this comprehensive guide, we’ll explore effective strategies for handling multiple edge cases efficiently, ensuring your code is robust, maintainable, and performant.
Understanding Edge Cases
Before diving into strategies for handling multiple edge cases, it’s essential to understand what edge cases are and why they’re important. Edge cases are scenarios that occur at the extreme ends of possible inputs or in unexpected situations. These can include:
- Empty inputs or null values
- Extremely large or small numbers
- Unexpected data types
- Boundary conditions
- Rare or unusual combinations of inputs
Failing to handle edge cases properly can lead to bugs, crashes, security vulnerabilities, and poor user experiences. As your codebase grows and becomes more complex, the number of potential edge cases often increases, making it crucial to have efficient strategies for dealing with them.
Strategies for Efficient Edge Case Handling
1. Early Returns and Guard Clauses
One of the most effective ways to handle multiple edge cases is by using early returns and guard clauses. This approach involves checking for edge cases at the beginning of your function and returning early if those conditions are met. This strategy helps to:
- Reduce nesting and improve code readability
- Handle edge cases before processing the main logic
- Simplify the overall structure of your function
Here’s an example of how you might use early returns to handle multiple edge cases:
function processUserData(user) {
if (!user) {
return 'Error: User data is missing';
}
if (!user.name || typeof user.name !== 'string') {
return 'Error: Invalid user name';
}
if (!user.age || typeof user.age !== 'number' || user.age < 0) {
return 'Error: Invalid user age';
}
// Main logic here
return `Processed data for ${user.name}, age ${user.age}`;
}
In this example, we check for various edge cases (missing user data, invalid name, invalid age) before proceeding with the main logic. This approach makes the code easier to read and maintain.
2. Use of Default Values and Optional Chaining
Another efficient way to handle edge cases, particularly when dealing with potentially undefined or null values, is to use default values and optional chaining. This can help you write more concise code while still accounting for edge cases.
Here’s an example using JavaScript:
function getUserInfo(user) {
const name = user?.name ?? 'Unknown';
const age = user?.age ?? 'Not specified';
const email = user?.contact?.email ?? 'No email provided';
return `Name: ${name}, Age: ${age}, Email: ${email}`;
}
In this example, we use the nullish coalescing operator (??) to provide default values and the optional chaining operator (?.) to safely access nested properties. This approach allows us to handle multiple edge cases (missing user object, missing properties, missing nested properties) in a concise and readable manner.
3. Input Validation and Type Checking
Implementing robust input validation and type checking is crucial for handling edge cases efficiently. By validating inputs early, you can catch and handle edge cases before they propagate through your code. This approach can include:
- Type checking using typeof or instanceof
- Range checking for numerical inputs
- Format validation for strings (e.g., email addresses, dates)
- Schema validation for complex objects
Here’s an example of how you might implement input validation:
function calculateArea(shape, dimensions) {
if (typeof shape !== 'string') {
throw new Error('Shape must be a string');
}
if (!Array.isArray(dimensions) || dimensions.length === 0) {
throw new Error('Dimensions must be a non-empty array');
}
if (!dimensions.every(d => typeof d === 'number' && d > 0)) {
throw new Error('All dimensions must be positive numbers');
}
switch (shape.toLowerCase()) {
case 'rectangle':
if (dimensions.length !== 2) {
throw new Error('Rectangle requires exactly 2 dimensions');
}
return dimensions[0] * dimensions[1];
case 'circle':
if (dimensions.length !== 1) {
throw new Error('Circle requires exactly 1 dimension (radius)');
}
return Math.PI * dimensions[0] ** 2;
default:
throw new Error('Unsupported shape');
}
}
This example demonstrates how to perform thorough input validation, checking for various edge cases such as invalid types, empty arrays, negative numbers, and incorrect number of dimensions for different shapes.
4. Error Handling and Custom Exceptions
When dealing with multiple edge cases, it’s often useful to implement a robust error handling system. This can include creating custom exception classes for different types of edge cases, which can make it easier to catch and handle specific issues in your code.
Here’s an example of how you might implement custom exceptions:
class ValidationError extends Error {
constructor(message) {
super(message);
this.name = 'ValidationError';
}
}
class InputMissingError extends ValidationError {
constructor(fieldName) {
super(`Required input '${fieldName}' is missing`);
this.name = 'InputMissingError';
}
}
class InputTypeError extends ValidationError {
constructor(fieldName, expectedType, receivedType) {
super(`Expected ${fieldName} to be ${expectedType}, but received ${receivedType}`);
this.name = 'InputTypeError';
}
}
function processUserInput(input) {
try {
if (!input.username) {
throw new InputMissingError('username');
}
if (typeof input.username !== 'string') {
throw new InputTypeError('username', 'string', typeof input.username);
}
if (!input.age) {
throw new InputMissingError('age');
}
if (typeof input.age !== 'number') {
throw new InputTypeError('age', 'number', typeof input.age);
}
// Process the input...
} catch (error) {
if (error instanceof ValidationError) {
console.error(`Validation failed: ${error.message}`);
// Handle validation errors...
} else {
console.error('An unexpected error occurred:', error);
// Handle other errors...
}
}
}
This approach allows you to create a hierarchy of error types, making it easier to catch and handle specific types of edge cases in a structured way.
5. Use of Design Patterns
Certain design patterns can be particularly useful when handling multiple edge cases. For example:
- Strategy Pattern: This pattern can be used to encapsulate different algorithms for handling various edge cases, allowing you to switch between them dynamically.
- Null Object Pattern: This pattern can help you handle null or undefined values more gracefully by providing a default object with neutral behavior.
- Decorator Pattern: This pattern can be used to add edge case handling behavior to existing objects without altering their structure.
Here’s an example of how you might use the Strategy pattern to handle different edge cases:
class DefaultStrategy {
handle(input) {
// Default handling logic
}
}
class NullInputStrategy {
handle(input) {
// Handle null input
}
}
class NegativeNumberStrategy {
handle(input) {
// Handle negative numbers
}
}
class EdgeCaseHandler {
constructor() {
this.strategy = new DefaultStrategy();
}
setStrategy(strategy) {
this.strategy = strategy;
}
handleInput(input) {
return this.strategy.handle(input);
}
}
function processInput(input) {
const handler = new EdgeCaseHandler();
if (input === null || input === undefined) {
handler.setStrategy(new NullInputStrategy());
} else if (typeof input === 'number' && input < 0) {
handler.setStrategy(new NegativeNumberStrategy());
}
return handler.handleInput(input);
}
This approach allows you to encapsulate different edge case handling strategies and switch between them based on the input, making your code more flexible and easier to extend.
6. Comprehensive Testing
While not a direct strategy for handling edge cases in your code, comprehensive testing is crucial for ensuring that your edge case handling is effective. This includes:
- Unit tests for individual functions, focusing on edge cases
- Integration tests to ensure edge cases are handled correctly in the context of the larger system
- Fuzz testing to identify unexpected edge cases
- Property-based testing to verify that your code behaves correctly for a wide range of inputs
Here’s an example of how you might write unit tests to cover edge cases:
function divide(a, b) {
if (typeof a !== 'number' || typeof b !== 'number') {
throw new Error('Both arguments must be numbers');
}
if (b === 0) {
throw new Error('Cannot divide by zero');
}
return a / b;
}
// Unit tests
describe('divide function', () => {
test('divides two positive numbers', () => {
expect(divide(10, 2)).toBe(5);
});
test('divides a positive and a negative number', () => {
expect(divide(10, -2)).toBe(-5);
});
test('divides two negative numbers', () => {
expect(divide(-10, -2)).toBe(5);
});
test('throws error when dividing by zero', () => {
expect(() => divide(10, 0)).toThrow('Cannot divide by zero');
});
test('throws error when arguments are not numbers', () => {
expect(() => divide('10', 2)).toThrow('Both arguments must be numbers');
expect(() => divide(10, '2')).toThrow('Both arguments must be numbers');
expect(() => divide(null, undefined)).toThrow('Both arguments must be numbers');
});
});
These tests cover various edge cases, including division by zero, negative numbers, and invalid input types.
Best Practices for Handling Multiple Edge Cases
When implementing strategies to handle multiple edge cases, keep these best practices in mind:
- Prioritize edge cases: Focus on the most critical and likely edge cases first. Not all edge cases are equally important or likely to occur.
- Document your assumptions: Clearly document the edge cases you’re handling and any assumptions you’re making about the input or system state.
- Use consistent error messages: When throwing errors or returning error messages for edge cases, use consistent and informative language to help with debugging and user experience.
- Consider performance: While it’s important to handle edge cases, be mindful of the performance impact of your error checking code, especially in performance-critical sections.
- Regularly review and update: As your system evolves, new edge cases may emerge. Regularly review and update your edge case handling strategies.
- Use logging and monitoring: Implement logging for edge cases to help with debugging and to identify patterns in how often certain edge cases occur in production.
Conclusion
Efficiently handling multiple edge cases is a critical skill for any programmer. By employing strategies such as early returns, input validation, custom exceptions, and appropriate design patterns, you can create more robust, maintainable, and reliable code. Remember that handling edge cases is not just about preventing errors—it’s about creating a better user experience, improving the reliability of your system, and making your code easier to understand and maintain.
As you develop your skills in handling edge cases, you’ll find that you become better at anticipating potential issues, leading to more thoughtful and comprehensive code design. This proactive approach to edge case handling will serve you well as you tackle increasingly complex programming challenges and build more sophisticated systems.
Keep practicing, stay curious about potential edge cases in your code, and always strive to balance thoroughness with efficiency in your approach to handling these important scenarios. With time and experience, handling multiple edge cases efficiently will become second nature, elevating the quality and reliability of your code.