How to Handle Memory Constraints in Your Solutions
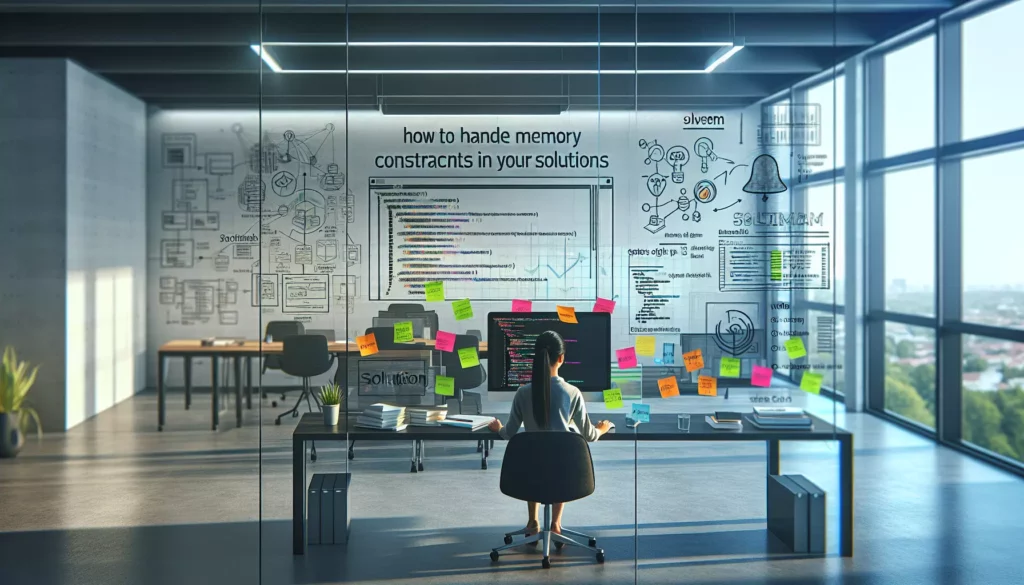
In the world of software development and algorithmic problem-solving, efficiently managing memory is a critical skill that can make or break your solutions. As you progress from basic coding exercises to more complex problems, particularly those encountered in technical interviews at major tech companies, understanding how to handle memory constraints becomes increasingly important. This comprehensive guide will explore various techniques and strategies to optimize memory usage in your code, helping you create more efficient and scalable solutions.
Understanding Memory Constraints
Before diving into specific techniques, it’s crucial to understand what memory constraints are and why they matter. In programming, memory constraints refer to limitations on the amount of available memory that your program can use. These constraints can arise from various sources:
- Hardware limitations: The physical RAM available on the machine running your code.
- System-imposed limits: Operating system restrictions on memory allocation for individual processes.
- Problem-specific constraints: Requirements set by the problem statement or interviewer, often to test your ability to optimize solutions.
Handling memory constraints effectively is important for several reasons:
- Performance: Efficient memory usage often correlates with faster execution times.
- Scalability: Solutions that manage memory well can handle larger inputs and datasets.
- Reliability: Proper memory management reduces the risk of crashes and out-of-memory errors.
- Interview success: Many technical interviews, especially at FAANG companies, assess candidates’ ability to optimize memory usage.
Techniques for Handling Memory Constraints
Now that we understand the importance of managing memory constraints, let’s explore various techniques you can employ to optimize your solutions:
1. Use Appropriate Data Structures
Choosing the right data structure can significantly impact memory usage. Consider the following options:
- Arrays: Great for fixed-size collections, but can waste memory if oversized.
- Linked Lists: Efficient for dynamic sizing, but have overhead for node pointers.
- Hash Tables: Offer fast access but can consume more memory due to unused buckets.
- Trees: Balanced trees like AVL or Red-Black trees can be memory-efficient for certain operations.
- Tries: Excellent for string-related problems, often more memory-efficient than storing full strings.
Example: Using a Trie for efficient string storage
class TrieNode:
def __init__(self):
self.children = {}
self.is_end = False
class Trie:
def __init__(self):
self.root = TrieNode()
def insert(self, word):
node = self.root
for char in word:
if char not in node.children:
node.children[char] = TrieNode()
node = node.children[char]
node.is_end = True
def search(self, word):
node = self.root
for char in word:
if char not in node.children:
return False
node = node.children[char]
return node.is_end
# Usage
trie = Trie()
trie.insert("apple")
print(trie.search("apple")) # True
print(trie.search("app")) # False
2. In-Place Algorithms
In-place algorithms modify the input data structure directly without using additional data structures. This approach can significantly reduce memory usage, especially for large inputs.
Example: In-place array reversal
def reverse_array_in_place(arr):
left, right = 0, len(arr) - 1
while left < right:
arr[left], arr[right] = arr[right], arr[left]
left += 1
right -= 1
return arr
# Usage
array = [1, 2, 3, 4, 5]
reversed_array = reverse_array_in_place(array)
print(reversed_array) # [5, 4, 3, 2, 1]
3. Space-Time Tradeoffs
Sometimes, you can trade time complexity for space complexity or vice versa. Consider using techniques like:
- Memoization: Caching results of expensive function calls.
- Dynamic Programming: Building solutions to larger problems from solutions to smaller subproblems.
- Two-pointer technique: Using two pointers to traverse data structures, often reducing the need for additional storage.
Example: Two-pointer technique for finding a pair with a given sum
def find_pair_with_sum(arr, target_sum):
left, right = 0, len(arr) - 1
while left < right:
current_sum = arr[left] + arr[right]
if current_sum == target_sum:
return arr[left], arr[right]
elif current_sum < target_sum:
left += 1
else:
right -= 1
return None
# Usage
sorted_array = [1, 2, 3, 4, 5, 6, 7, 8, 9]
result = find_pair_with_sum(sorted_array, 10)
print(result) # (1, 9)
4. Bit Manipulation
Using bit manipulation techniques can significantly reduce memory usage, especially when dealing with large sets of boolean flags or small integer values.
Example: Using bitwise operations to check if a number is even or odd
def is_even(num):
return not (num & 1)
# Usage
print(is_even(4)) # True
print(is_even(7)) # False
5. Streaming and Iterative Processing
For large datasets that don’t fit entirely in memory, consider processing data in chunks or streams. This approach allows you to handle massive amounts of data with limited memory.
Example: Processing a large file line by line
def process_large_file(filename):
with open(filename, 'r') as file:
for line in file:
# Process each line
processed_line = line.strip().upper()
print(processed_line)
# Usage
process_large_file('large_data.txt')
6. Memory-Efficient Data Compression
When dealing with large amounts of data, consider using compression techniques to reduce memory footprint. This can include:
- Run-length encoding for repetitive data
- Huffman coding for variable-length encoding
- Delta encoding for sequences with small changes
Example: Simple run-length encoding
def run_length_encode(s):
if not s:
return ""
result = []
count = 1
for i in range(1, len(s)):
if s[i] == s[i-1]:
count += 1
else:
result.append(f"{count}{s[i-1]}")
count = 1
result.append(f"{count}{s[-1]}")
return ''.join(result)
# Usage
original = "AABBBCCCC"
encoded = run_length_encode(original)
print(encoded) # "2A3B4C"
Advanced Techniques for Handling Memory Constraints
As you progress to more complex problems and larger-scale applications, you may need to employ more advanced techniques to handle memory constraints effectively:
7. External Sorting
When dealing with datasets too large to fit in memory, external sorting algorithms can be used. These algorithms sort data that resides in external storage (like hard drives) and only bring small chunks into memory at a time.
Example: Simplified external merge sort (conceptual)
def external_merge_sort(input_file, output_file, chunk_size):
# Step 1: Split the file into sorted chunks
chunks = split_and_sort(input_file, chunk_size)
# Step 2: Merge the sorted chunks
merge_chunks(chunks, output_file)
def split_and_sort(input_file, chunk_size):
chunks = []
with open(input_file, 'r') as f:
while True:
chunk = f.readlines(chunk_size)
if not chunk:
break
chunk.sort()
temp_file = f"temp_{len(chunks)}.txt"
with open(temp_file, 'w') as temp:
temp.writelines(chunk)
chunks.append(temp_file)
return chunks
def merge_chunks(chunks, output_file):
# Implementation of merging chunks
# This would involve opening all chunk files and performing a multi-way merge
pass
# Usage
external_merge_sort("large_input.txt", "sorted_output.txt", 1000000)
8. Memory-Mapped Files
Memory-mapped files allow you to treat a file as if it were in memory, providing efficient random access without loading the entire file into RAM. This technique is particularly useful for working with very large files.
Example: Using memory-mapped files in Python
import mmap
def sum_integers_in_file(filename):
with open(filename, "r+b") as f:
# Memory-map the file
mmapped_file = mmap.mmap(f.fileno(), 0)
total = 0
# Read 4-byte integers from the file
for i in range(0, len(mmapped_file), 4):
integer = int.from_bytes(mmapped_file[i:i+4], byteorder='little')
total += integer
mmapped_file.close()
return total
# Usage
result = sum_integers_in_file("large_integers.bin")
print(f"Sum of integers: {result}")
9. Probabilistic Data Structures
Probabilistic data structures trade perfect accuracy for significantly reduced memory usage. These structures are particularly useful when dealing with large-scale data processing and analytics.
- Bloom Filters: For membership testing in a set
- Count-Min Sketch: For frequency estimation of events in a stream
- HyperLogLog: For cardinality estimation
Example: Simple Bloom Filter implementation
import mmh3
from bitarray import bitarray
class BloomFilter:
def __init__(self, size, hash_count):
self.size = size
self.hash_count = hash_count
self.bit_array = bitarray(size)
self.bit_array.setall(0)
def add(self, item):
for seed in range(self.hash_count):
index = mmh3.hash(item, seed) % self.size
self.bit_array[index] = 1
def check(self, item):
for seed in range(self.hash_count):
index = mmh3.hash(item, seed) % self.size
if not self.bit_array[index]:
return False
return True
# Usage
bloom_filter = BloomFilter(100, 3)
bloom_filter.add("apple")
bloom_filter.add("banana")
print(bloom_filter.check("apple")) # True
print(bloom_filter.check("orange")) # False (probably)
10. Garbage Collection Optimization
In languages with automatic memory management, understanding and optimizing garbage collection can significantly improve memory usage and performance.
- Minimize object creation and destruction
- Use object pools for frequently created and destroyed objects
- Be aware of reference cycles and break them when possible
Example: Object pool in Python
class ReusableObject:
def __init__(self):
self.value = None
def set_value(self, value):
self.value = value
def reset(self):
self.value = None
class ObjectPool:
def __init__(self, size):
self.size = size
self.objects = [ReusableObject() for _ in range(size)]
self.available = set(range(size))
def get_object(self):
if not self.available:
raise Exception("No objects available in the pool")
index = self.available.pop()
return self.objects[index]
def return_object(self, obj):
obj.reset()
index = self.objects.index(obj)
self.available.add(index)
# Usage
pool = ObjectPool(5)
obj1 = pool.get_object()
obj1.set_value(42)
print(obj1.value) # 42
pool.return_object(obj1)
obj2 = pool.get_object()
print(obj2.value) # None
Best Practices for Handling Memory Constraints
To effectively handle memory constraints in your solutions, consider adopting these best practices:
- Profile your code: Use memory profiling tools to identify memory bottlenecks and optimize accordingly.
- Lazy evaluation: Compute values only when needed, especially for large or complex calculations.
- Use generators: In Python and similar languages, use generators to create iterables without storing all values in memory.
- Avoid unnecessary copies: Pass references or pointers when possible instead of creating copies of large data structures.
- Clean up resources: Properly close files, database connections, and other resources when they’re no longer needed.
- Use appropriate data types: Choose the smallest data type that can represent your data (e.g., using a byte instead of an integer for small values).
- Understand language-specific memory management: Be aware of how your programming language handles memory allocation and deallocation.
Conclusion
Handling memory constraints is a crucial skill for any programmer, especially when preparing for technical interviews at top tech companies. By understanding and applying the techniques and best practices outlined in this guide, you’ll be better equipped to create efficient, scalable solutions to complex problems.
Remember that optimizing for memory often involves trade-offs with time complexity or code readability. Always consider the specific requirements of your problem and the constraints of your environment when choosing which techniques to apply.
As you continue to practice and refine your skills, you’ll develop an intuition for when and how to apply these memory optimization techniques. This expertise will not only help you succeed in technical interviews but also make you a more effective and resourceful programmer in your professional career.
Keep coding, keep optimizing, and never stop learning!