How to Handle Matrix Rotation: A Comprehensive Guide
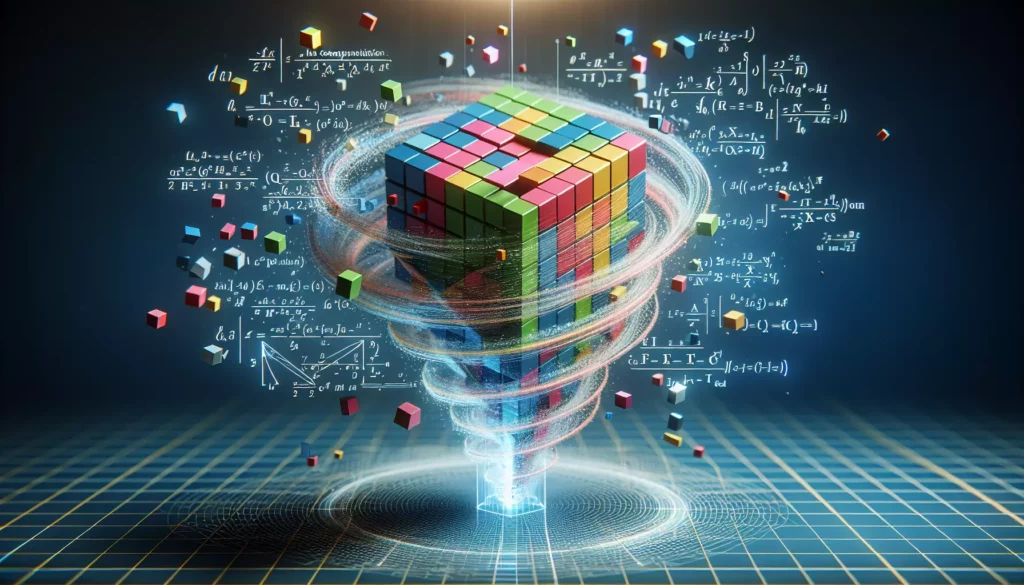
Matrix rotation is a fundamental operation in computer science and mathematics, with applications ranging from image processing to game development. In this comprehensive guide, we’ll explore various techniques for rotating matrices, their implementations, and real-world applications. Whether you’re preparing for a technical interview or simply want to improve your coding skills, understanding matrix rotation is essential for any aspiring programmer.
Table of Contents
- Introduction to Matrix Rotation
- Types of Matrix Rotation
- Clockwise Rotation
- Counterclockwise Rotation
- In-Place Rotation
- Rotation using Transposition
- Real-World Applications
- Optimization Techniques
- Common Challenges and Solutions
- Conclusion
1. Introduction to Matrix Rotation
Matrix rotation is the process of changing the orientation of elements in a 2D array or matrix. It’s a crucial operation in various fields, including computer graphics, image processing, and algorithms. Understanding how to efficiently rotate matrices is not only important for solving coding problems but also for developing practical applications.
Before diving into the specifics of matrix rotation, let’s refresh our understanding of what a matrix is:
A matrix is a rectangular array of numbers, symbols, or expressions arranged in rows and columns. For example:
[1 2 3]
[4 5 6]
[7 8 9]
This is a 3x3 matrix.
When we talk about rotating a matrix, we’re referring to changing the position of these elements in a systematic way, either clockwise or counterclockwise.
2. Types of Matrix Rotation
There are two primary types of matrix rotation:
- Clockwise Rotation: Elements move in a clockwise direction.
- Counterclockwise Rotation: Elements move in a counterclockwise direction.
Both types can be performed for any degree of rotation, but the most common rotations are 90°, 180°, and 270°. In this guide, we’ll focus primarily on 90° rotations, as they form the basis for other rotation angles.
3. Clockwise Rotation
Let’s start with a 90° clockwise rotation. For a square matrix, this means that:
- The first row becomes the last column
- The second row becomes the second-to-last column
- And so on…
Here’s a Python implementation of a 90° clockwise rotation:
def rotate_90_clockwise(matrix):
n = len(matrix)
rotated = [[0 for _ in range(n)] for _ in range(n)]
for i in range(n):
for j in range(n):
rotated[j][n-1-i] = matrix[i][j]
return rotated
# Example usage
matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
rotated = rotate_90_clockwise(matrix)
for row in rotated:
print(row)
This function creates a new matrix to store the rotated values. The time complexity of this approach is O(n^2), where n is the number of rows (or columns) in the square matrix.
4. Counterclockwise Rotation
For a 90° counterclockwise rotation, the process is similar, but the mapping of elements changes:
- The first column becomes the first row
- The second column becomes the second row
- And so on…
Here’s a Python implementation for counterclockwise rotation:
def rotate_90_counterclockwise(matrix):
n = len(matrix)
rotated = [[0 for _ in range(n)] for _ in range(n)]
for i in range(n):
for j in range(n):
rotated[n-1-j][i] = matrix[i][j]
return rotated
# Example usage
matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
rotated = rotate_90_counterclockwise(matrix)
for row in rotated:
print(row)
The time and space complexity for this approach is also O(n^2).
5. In-Place Rotation
While the previous methods create a new matrix for the rotated result, it’s often desirable to perform the rotation in-place, especially when dealing with large matrices or memory-constrained environments. In-place rotation modifies the original matrix without using additional space.
Here’s an implementation of in-place 90° clockwise rotation:
def rotate_90_clockwise_inplace(matrix):
n = len(matrix)
# Transpose the matrix
for i in range(n):
for j in range(i, n):
matrix[i][j], matrix[j][i] = matrix[j][i], matrix[i][j]
# Reverse each row
for i in range(n):
matrix[i] = matrix[i][::-1]
# Example usage
matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
rotate_90_clockwise_inplace(matrix)
for row in matrix:
print(row)
This in-place rotation method first transposes the matrix (swaps elements across the main diagonal) and then reverses each row. The time complexity remains O(n^2), but the space complexity is reduced to O(1) as we’re not using any additional data structures.
6. Rotation using Transposition
As we saw in the in-place rotation method, transposition plays a crucial role in matrix rotation. Let’s break down this process:
- Transposition: Swap elements across the main diagonal (from top-left to bottom-right).
- Reversal: Depending on the direction of rotation, reverse either rows or columns.
Here’s how you can implement matrix transposition:
def transpose(matrix):
n = len(matrix)
for i in range(n):
for j in range(i, n):
matrix[i][j], matrix[j][i] = matrix[j][i], matrix[i][j]
# Example usage
matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
transpose(matrix)
for row in matrix:
print(row)
After transposition, to achieve a 90° clockwise rotation, you would reverse each row. For a 90° counterclockwise rotation, you would reverse each column instead.
7. Real-World Applications
Matrix rotation finds applications in various fields:
- Image Processing: Rotating images is a common operation in photo editing software and computer vision applications.
- Computer Graphics: In 3D rendering and game development, matrix rotations are used to change the orientation of objects.
- Data Visualization: Rotating data representations can provide new perspectives on complex datasets.
- Cryptography: Some encryption algorithms use matrix rotations as part of their operations.
- Scientific Simulations: In physics and engineering simulations, matrix rotations can represent changes in orientation or coordinate systems.
Let’s look at a simple example of how matrix rotation could be used in image processing:
from PIL import Image
import numpy as np
def rotate_image(image_path, degrees):
# Open the image
img = Image.open(image_path)
# Convert to numpy array
img_array = np.array(img)
# Rotate the image
if degrees == 90:
rotated_array = np.rot90(img_array, k=1, axes=(1, 0))
elif degrees == 180:
rotated_array = np.rot90(img_array, k=2, axes=(1, 0))
elif degrees == 270:
rotated_array = np.rot90(img_array, k=3, axes=(1, 0))
else:
raise ValueError("Rotation must be 90, 180, or 270 degrees")
# Convert back to image
rotated_img = Image.fromarray(rotated_array)
return rotated_img
# Example usage
rotated = rotate_image("path/to/your/image.jpg", 90)
rotated.save("rotated_image.jpg")
This example uses the Python Imaging Library (PIL) and NumPy to rotate an image. The image is converted to a NumPy array, rotated using the np.rot90()
function, and then converted back to an image.
8. Optimization Techniques
While the basic implementations we’ve discussed work well for small to medium-sized matrices, there are several optimization techniques that can be employed for larger matrices or when performance is critical:
- Vectorization: Using libraries like NumPy can significantly speed up matrix operations by leveraging optimized, low-level implementations.
- Parallel Processing: For very large matrices, you can split the matrix into smaller submatrices and rotate them in parallel.
- Cache Optimization: Arranging your data access patterns to maximize cache hits can improve performance, especially for in-place rotations.
- Bit Manipulation: For certain types of matrices (e.g., binary matrices), bit manipulation techniques can be used for extremely fast rotations.
Here’s an example of using NumPy for efficient matrix rotation:
import numpy as np
def rotate_90_clockwise_numpy(matrix):
return np.rot90(matrix, k=-1)
def rotate_90_counterclockwise_numpy(matrix):
return np.rot90(matrix, k=1)
# Example usage
matrix = np.array([
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
])
rotated_cw = rotate_90_clockwise_numpy(matrix)
rotated_ccw = rotate_90_counterclockwise_numpy(matrix)
print("Clockwise rotation:")
print(rotated_cw)
print("\nCounterclockwise rotation:")
print(rotated_ccw)
NumPy’s rot90()
function is highly optimized and can handle large matrices efficiently.
9. Common Challenges and Solutions
When working with matrix rotations, you might encounter several challenges. Here are some common ones and their solutions:
- Challenge: Rotating non-square matrices.
Solution: Implement a function that can handle matrices of any dimension by adjusting the indexing accordingly. - Challenge: Dealing with large matrices that don’t fit in memory.
Solution: Implement a chunking strategy where you rotate the matrix in smaller, manageable pieces. - Challenge: Maintaining the integrity of the original data.
Solution: If you need to preserve the original matrix, always work on a copy unless in-place rotation is specifically required. - Challenge: Handling rotations of angles other than 90°, 180°, or 270°.
Solution: For arbitrary angles, you might need to use more complex algorithms involving trigonometric functions and interpolation.
Here’s an example of a function that can rotate non-square matrices:
def rotate_90_clockwise_any_size(matrix):
rows = len(matrix)
cols = len(matrix[0]) if rows > 0 else 0
rotated = [[0 for _ in range(rows)] for _ in range(cols)]
for i in range(rows):
for j in range(cols):
rotated[j][rows-1-i] = matrix[i][j]
return rotated
# Example usage
matrix = [
[1, 2, 3, 4],
[5, 6, 7, 8],
[9, 10, 11, 12]
]
rotated = rotate_90_clockwise_any_size(matrix)
for row in rotated:
print(row)
This function can handle matrices of any size, not just square matrices.
10. Conclusion
Matrix rotation is a fundamental operation in computer science with wide-ranging applications. We’ve explored various methods for rotating matrices, including clockwise and counterclockwise rotations, in-place rotations, and optimizations using libraries like NumPy. We’ve also discussed real-world applications and common challenges you might face when working with matrix rotations.
As you continue to develop your programming skills, remember that understanding matrix operations like rotation is crucial for many advanced algorithms and applications. Practice implementing these rotations in different programming languages and try to optimize your solutions for various scenarios.
Whether you’re preparing for a technical interview at a major tech company or working on a complex computer graphics project, the ability to efficiently manipulate matrices will serve you well. Keep exploring, practicing, and pushing the boundaries of your understanding. Happy coding!