How to Handle Matrix Multiplication: A Comprehensive Guide
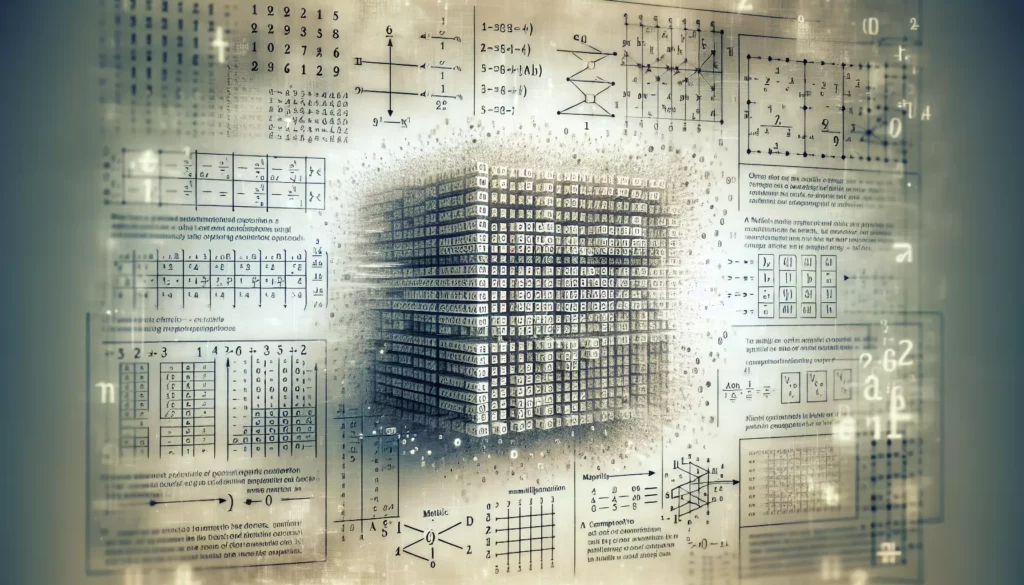
Matrix multiplication is a fundamental operation in linear algebra with widespread applications in computer science, data analysis, and machine learning. Understanding how to perform matrix multiplication efficiently is crucial for aspiring programmers and data scientists. In this comprehensive guide, we’ll explore the concept of matrix multiplication, its importance, and how to implement it in various programming languages.
Table of Contents
- What is Matrix Multiplication?
- Importance of Matrix Multiplication
- Rules of Matrix Multiplication
- Implementing Matrix Multiplication
- Optimizing Matrix Multiplication
- Applications of Matrix Multiplication
- Common Mistakes and Pitfalls
- Conclusion
What is Matrix Multiplication?
Matrix multiplication is an operation that produces a new matrix by combining two input matrices according to specific rules. The resulting matrix contains elements that are the sum of the products of corresponding rows from the first matrix and columns from the second matrix.
For two matrices A and B to be multiplied, the number of columns in matrix A must be equal to the number of rows in matrix B. The resulting matrix C will have the same number of rows as matrix A and the same number of columns as matrix B.
Importance of Matrix Multiplication
Matrix multiplication is a crucial operation in various fields:
- Computer Graphics: Used for transformations, rotations, and scaling in 2D and 3D graphics.
- Machine Learning: Essential in neural network computations and data transformations.
- Data Analysis: Used in dimensionality reduction techniques like Principal Component Analysis (PCA).
- Scientific Computing: Applied in solving systems of linear equations and simulations.
- Cryptography: Utilized in various encryption and decryption algorithms.
Rules of Matrix Multiplication
Before diving into the implementation, let’s review the rules of matrix multiplication:
- The number of columns in the first matrix must equal the number of rows in the second matrix.
- The resulting matrix will have the same number of rows as the first matrix and the same number of columns as the second matrix.
- Each element in the resulting matrix is calculated by multiplying corresponding elements from a row of the first matrix and a column of the second matrix, then summing these products.
Mathematically, for matrices A (m x n) and B (n x p), the resulting matrix C (m x p) is calculated as follows:
C[i][j] = sum(A[i][k] * B[k][j]) for k = 0 to n-1
Where i ranges from 0 to m-1, and j ranges from 0 to p-1
Implementing Matrix Multiplication
Now, let’s implement matrix multiplication in three popular programming languages: Python, Java, and C++.
Python Implementation
Python offers a concise and readable way to implement matrix multiplication:
def matrix_multiply(A, B):
# Check if matrices can be multiplied
if len(A[0]) != len(B):
raise ValueError("Number of columns in A must equal number of rows in B")
# Initialize the result matrix with zeros
result = [[0 for _ in range(len(B[0]))] for _ in range(len(A))]
# Perform matrix multiplication
for i in range(len(A)):
for j in range(len(B[0])):
for k in range(len(B)):
result[i][j] += A[i][k] * B[k][j]
return result
# Example usage
A = [[1, 2], [3, 4]]
B = [[5, 6], [7, 8]]
C = matrix_multiply(A, B)
print(C)
This implementation uses nested loops to iterate through the matrices and perform the multiplication. The time complexity of this naive implementation is O(n^3) for n x n matrices.
Java Implementation
Here’s how we can implement matrix multiplication in Java:
public class MatrixMultiplication {
public static int[][] matrixMultiply(int[][] A, int[][] B) {
if (A[0].length != B.length) {
throw new IllegalArgumentException("Number of columns in A must equal number of rows in B");
}
int[][] result = new int[A.length][B[0].length];
for (int i = 0; i < A.length; i++) {
for (int j = 0; j < B[0].length; j++) {
for (int k = 0; k < B.length; k++) {
result[i][j] += A[i][k] * B[k][j];
}
}
}
return result;
}
public static void main(String[] args) {
int[][] A = {{1, 2}, {3, 4}};
int[][] B = {{5, 6}, {7, 8}};
int[][] C = matrixMultiply(A, B);
// Print the result
for (int[] row : C) {
for (int element : row) {
System.out.print(element + " ");
}
System.out.println();
}
}
}
The Java implementation follows a similar structure to the Python version, using nested loops to perform the multiplication.
C++ Implementation
Here’s an implementation of matrix multiplication in C++:
#include <iostream>
#include <vector>
#include <stdexcept>
std::vector<std::vector<int>> matrixMultiply(const std::vector<std::vector<int>>& A,
const std::vector<std::vector<int>>& B) {
if (A[0].size() != B.size()) {
throw std::invalid_argument("Number of columns in A must equal number of rows in B");
}
std::vector<std::vector<int>> result(A.size(), std::vector<int>(B[0].size(), 0));
for (size_t i = 0; i < A.size(); ++i) {
for (size_t j = 0; j < B[0].size(); ++j) {
for (size_t k = 0; k < B.size(); ++k) {
result[i][j] += A[i][k] * B[k][j];
}
}
}
return result;
}
int main() {
std::vector<std::vector<int>> A = {{1, 2}, {3, 4}};
std::vector<std::vector<int>> B = {{5, 6}, {7, 8}};
std::vector<std::vector<int>> C = matrixMultiply(A, B);
// Print the result
for (const auto& row : C) {
for (int element : row) {
std::cout << element << " ";
}
std::cout << std::endl;
}
return 0;
}
The C++ implementation uses vectors to represent matrices and follows the same multiplication algorithm as the Python and Java versions.
Optimizing Matrix Multiplication
While the naive implementation works well for small matrices, it becomes inefficient for large matrices. Here are some optimization techniques:
- Cache Optimization: Improve cache utilization by transposing one of the matrices before multiplication.
- Strassen’s Algorithm: Reduces the number of multiplications for large matrices, with a time complexity of O(n^2.8) for n x n matrices.
- Parallel Processing: Utilize multi-threading or GPU acceleration to perform calculations concurrently.
- Block Matrix Multiplication: Divide matrices into smaller blocks to improve cache performance.
- Libraries and Frameworks: Use optimized libraries like NumPy for Python, BLAS for C/C++, or Eigen for C++.
Here’s an example of how to use NumPy for efficient matrix multiplication in Python:
import numpy as np
A = np.array([[1, 2], [3, 4]])
B = np.array([[5, 6], [7, 8]])
C = np.dot(A, B)
print(C)
NumPy’s dot
function is highly optimized and can be much faster than the naive implementation, especially for large matrices.
Applications of Matrix Multiplication
Matrix multiplication has numerous practical applications across various fields:
- Image Processing: Used in applying filters and transformations to images.
- Graph Theory: Calculating the number of paths between nodes in a graph.
- Recommender Systems: Collaborative filtering algorithms often use matrix multiplication.
- Natural Language Processing: Word embedding and language models utilize matrix operations.
- Financial Modeling: Portfolio optimization and risk assessment calculations.
- Quantum Mechanics: Representing and manipulating quantum states.
Common Mistakes and Pitfalls
When working with matrix multiplication, be aware of these common issues:
- Dimension Mismatch: Ensure the number of columns in the first matrix matches the number of rows in the second matrix.
- Order of Multiplication: Matrix multiplication is not commutative (A * B ≠B * A).
- Precision Loss: Be cautious of floating-point precision issues when working with large matrices or performing many operations.
- Memory Management: For large matrices, be mindful of memory usage and consider using sparse matrix representations when appropriate.
- Performance Bottlenecks: Naive implementations can be slow for large matrices; use optimized libraries or algorithms for better performance.
Conclusion
Matrix multiplication is a fundamental operation with wide-ranging applications in computer science and beyond. By understanding the principles behind matrix multiplication and learning how to implement it efficiently, you’ll be better equipped to tackle complex problems in areas such as machine learning, computer graphics, and data analysis.
As you continue your journey in programming and algorithm development, remember that matrix multiplication is just one of many important concepts you’ll encounter. Keep practicing, exploring different implementations, and considering optimization techniques to improve your skills and the efficiency of your code.
Whether you’re preparing for technical interviews at top tech companies or working on your own projects, a solid understanding of matrix multiplication and its applications will serve you well in your programming career.