How to Handle Linked List Reversal: A Comprehensive Guide
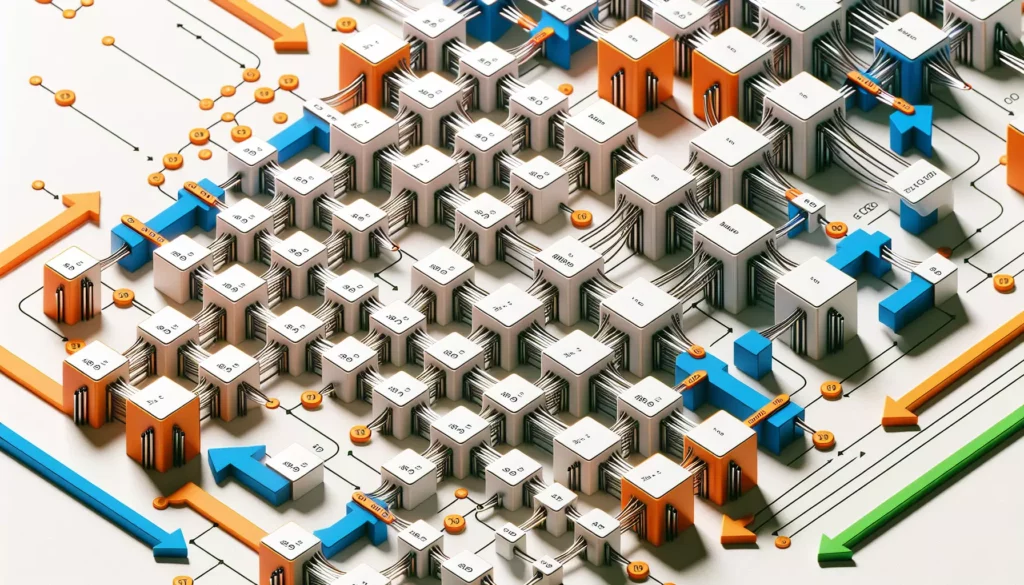
Linked list reversal is a fundamental problem in computer science and a common topic in coding interviews, especially for tech giants like FAANG (Facebook, Amazon, Apple, Netflix, Google). Understanding how to reverse a linked list is crucial for developing strong algorithmic thinking and problem-solving skills. In this comprehensive guide, we’ll explore various approaches to handle linked list reversal, from the basic iterative method to more advanced recursive techniques.
Table of Contents
- Introduction to Linked Lists
- Why Reverse a Linked List?
- Iterative Approach to Linked List Reversal
- Recursive Approach to Linked List Reversal
- In-Place Reversal of a Linked List
- Reversing a Sublist within a Linked List
- Reversing Linked List in K-Groups
- Time and Space Complexity Analysis
- Common Mistakes and How to Avoid Them
- Practice Problems and Solutions
- Conclusion
1. Introduction to Linked Lists
Before diving into the reversal techniques, let’s briefly review what a linked list is. A linked list is a linear data structure where elements are stored in nodes. Each node contains a data field and a reference (or link) to the next node in the sequence.
Here’s a simple implementation of a linked list node in Python:
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
This structure allows for efficient insertion and deletion of elements, as only the links need to be changed, not the physical location of the elements in memory.
2. Why Reverse a Linked List?
Reversing a linked list is a common operation that serves several purposes:
- Interview practice: It’s a classic problem that tests a candidate’s understanding of linked list manipulation and pointer operations.
- Algorithm building block: Reversing a linked list is often used as a subroutine in more complex algorithms.
- Data processing: In some applications, data might need to be processed in reverse order.
- Palindrome checking: Reversing half of a linked list can be used to check if it’s a palindrome.
3. Iterative Approach to Linked List Reversal
The iterative approach is often the most intuitive way to reverse a linked list. It involves traversing the list once while changing the next pointers of each node to point to the previous node.
Here’s the Python code for the iterative approach:
def reverseList(head):
prev = None
current = head
while current is not None:
next_temp = current.next
current.next = prev
prev = current
current = next_temp
return prev
Let’s break down this algorithm:
- We start with three pointers:
prev
(initially None),current
(pointing to the head), andnext_temp
. - We iterate through the list, at each step:
- Save the next node in
next_temp
- Reverse the link of the current node to point to the previous node
- Move
prev
andcurrent
one step forward
- Save the next node in
- After the loop,
prev
will be pointing to the last node, which is now the new head of the reversed list.
4. Recursive Approach to Linked List Reversal
The recursive approach to reversing a linked list can be more elegant but might be less intuitive at first. It leverages the call stack to reverse the links.
Here’s the Python code for the recursive approach:
def reverseList(head):
if not head or not head.next:
return head
new_head = reverseList(head.next)
head.next.next = head
head.next = None
return new_head
Let’s analyze this recursive solution:
- The base case is when we reach the last node or an empty list.
- We recursively call
reverseList
on the rest of the list (head.next). - After the recursive calls are done, we need to reverse the link between the current node and the next node.
- We set the next node’s next pointer to the current node:
head.next.next = head
- We set the current node’s next pointer to None:
head.next = None
- We return the new head, which is the last node of the original list.
5. In-Place Reversal of a Linked List
In-place reversal means reversing the linked list without using any extra space. Both the iterative and recursive approaches we’ve seen are in-place reversals. However, the recursive approach uses implicit extra space in the form of the call stack.
The iterative approach is truly in-place as it only uses a constant amount of extra space regardless of the size of the input. This makes it more space-efficient, especially for very large lists.
6. Reversing a Sublist within a Linked List
Sometimes, you might need to reverse only a portion of a linked list. This is a more complex problem but builds on the basic reversal technique.
Here’s a Python implementation to reverse a sublist from position m to n:
def reverseBetween(head, m, n):
if not head or m == n:
return head
dummy = ListNode(0)
dummy.next = head
prev = dummy
for _ in range(m - 1):
prev = prev.next
start = prev.next
then = start.next
for _ in range(n - m):
start.next = then.next
then.next = prev.next
prev.next = then
then = start.next
return dummy.next
This algorithm works as follows:
- We use a dummy node to handle edge cases where the head might change.
- We move to the node just before the sublist starts.
- We then use a variation of the iterative reversal algorithm to reverse only the sublist.
- Finally, we return the head of the modified list.
7. Reversing Linked List in K-Groups
An even more challenging variation is reversing the linked list in groups of k nodes. This problem is often asked in advanced coding interviews.
Here’s a Python implementation:
def reverseKGroup(head, k):
dummy = ListNode(0)
dummy.next = head
prev_group_end = dummy
while head:
group_start = head
group_end = getGroupEnd(head, k)
if not group_end:
return dummy.next
next_group_start = group_end.next
reverseGroup(group_start, next_group_start)
prev_group_end.next = group_end
group_start.next = next_group_start
prev_group_end = group_start
head = next_group_start
return dummy.next
def getGroupEnd(head, k):
while head and k > 1:
head = head.next
k -= 1
return head
def reverseGroup(start, end):
prev = end
while start != end:
temp = start.next
start.next = prev
prev = start
start = temp
This algorithm does the following:
- We use a dummy node to handle changes to the head.
- We iterate through the list, reversing groups of k nodes at a time.
- For each group, we find the end node, reverse the group, and update the connections.
- If we can’t form a complete group of k nodes, we leave the remaining nodes as they are.
8. Time and Space Complexity Analysis
Understanding the time and space complexity of these algorithms is crucial for optimizing your code and performing well in interviews.
Iterative Reversal:
- Time Complexity: O(n), where n is the number of nodes in the list.
- Space Complexity: O(1), as we only use a constant amount of extra space.
Recursive Reversal:
- Time Complexity: O(n), where n is the number of nodes in the list.
- Space Complexity: O(n) due to the recursive call stack.
Reversing a Sublist:
- Time Complexity: O(n), as we might need to traverse the entire list.
- Space Complexity: O(1), as we only use a constant amount of extra space.
Reversing in K-Groups:
- Time Complexity: O(n), where n is the number of nodes in the list.
- Space Complexity: O(1), as we reverse in-place.
9. Common Mistakes and How to Avoid Them
When working with linked list reversal, there are several common pitfalls to watch out for:
- Losing the reference to the next node: Always save the reference to the next node before changing links.
- Not handling edge cases: Consider empty lists, single-node lists, and lists with only two nodes.
- Infinite loops: Ensure that you’re properly updating all pointers to avoid creating cycles in the list.
- Off-by-one errors: Be careful with the start and end positions when reversing sublists.
- Not updating the head: Remember to return the new head of the reversed list.
To avoid these mistakes:
- Always draw out the linked list and walk through your algorithm step-by-step.
- Test your code with various edge cases.
- Use a dummy node when the head of the list might change.
- Double-check your loop conditions and pointer updates.
10. Practice Problems and Solutions
To solidify your understanding of linked list reversal, try these practice problems:
- Reverse Linked List II (LeetCode 92): Reverse a linked list from position m to n.
- Palindrome Linked List (LeetCode 234): Check if a linked list is a palindrome.
- Reverse Nodes in k-Group (LeetCode 25): Reverse the nodes of a linked list k at a time.
- Swap Nodes in Pairs (LeetCode 24): Swap every two adjacent nodes in a linked list.
- Reorder List (LeetCode 143): Reorder the list to be L0 → Ln → L1 → Ln-1 → L2 → Ln-2 → …
Here’s a solution to the “Palindrome Linked List” problem as an example:
def isPalindrome(head):
if not head or not head.next:
return True
# Find the middle of the linked list
slow = fast = head
while fast.next and fast.next.next:
slow = slow.next
fast = fast.next.next
# Reverse the second half of the linked list
second_half = reverseList(slow.next)
# Compare the first half with the reversed second half
first_half = head
while second_half:
if first_half.val != second_half.val:
return False
first_half = first_half.next
second_half = second_half.next
return True
def reverseList(head):
prev = None
current = head
while current:
next_temp = current.next
current.next = prev
prev = current
current = next_temp
return prev
This solution uses the concept of reversing a linked list to efficiently check if the list is a palindrome.
11. Conclusion
Mastering linked list reversal is a crucial skill for any programmer, especially those preparing for technical interviews at top tech companies. The techniques we’ve covered – from basic iterative and recursive approaches to more complex operations like reversing sublists and k-groups – form the foundation for solving a wide range of linked list problems.
Remember, the key to becoming proficient with linked list operations is practice. Work through the problems we’ve discussed, implement the solutions yourself, and don’t hesitate to draw out the steps when you’re stuck. With time and effort, you’ll find that these operations become second nature, allowing you to tackle even more complex data structure and algorithm challenges.
As you continue your journey in programming and interview preparation, keep in mind that linked list reversal is just one of many important topics. Explore other data structures, algorithms, and problem-solving techniques to build a well-rounded skill set. Happy coding!