How to Handle Linked List Merging: A Comprehensive Guide
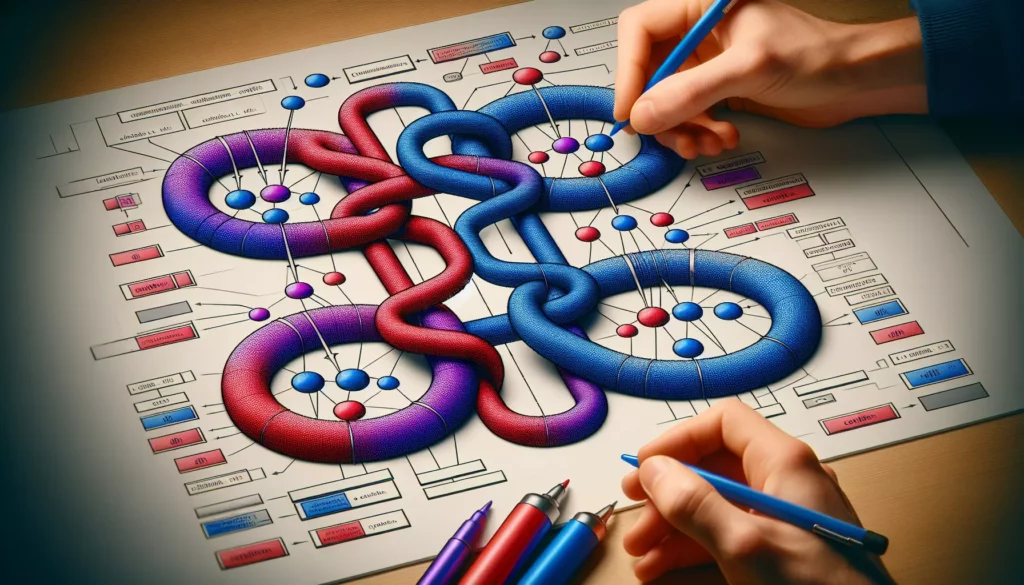
Linked list merging is a fundamental operation in computer science and a common topic in coding interviews, especially for tech giants like FAANG (Facebook, Amazon, Apple, Netflix, Google). Understanding how to merge linked lists efficiently is crucial for developing strong algorithmic thinking and problem-solving skills. In this comprehensive guide, we’ll explore various techniques for handling linked list merging, from basic concepts to advanced implementations.
Table of Contents
- Introduction to Linked Lists
- Basic Linked List Merging
- Merging Sorted Linked Lists
- K-way Merge of Sorted Linked Lists
- In-place Merging Techniques
- Optimization Strategies
- Common Pitfalls and How to Avoid Them
- Interview Tips for Linked List Merging Questions
- Advanced Topics in Linked List Merging
- Conclusion
1. Introduction to Linked Lists
Before diving into merging techniques, let’s briefly review what linked lists are and why they’re important in computer science.
What is a Linked List?
A linked list is a linear data structure where elements are stored in nodes. Each node contains a data field and a reference (or link) to the next node in the sequence. Unlike arrays, linked lists do not store elements in contiguous memory locations, which allows for efficient insertion and deletion operations.
Types of Linked Lists
- Singly Linked List: Each node points to the next node in the sequence.
- Doubly Linked List: Each node has pointers to both the next and previous nodes.
- Circular Linked List: The last node points back to the first node, creating a circle.
Basic Structure of a Linked List Node
Here’s a simple implementation of a singly linked list node in Python:
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
Understanding the basic structure and operations of linked lists is crucial before tackling merging problems.
2. Basic Linked List Merging
Let’s start with the simplest form of linked list merging: combining two unsorted linked lists.
Merging Two Unsorted Linked Lists
The basic approach to merge two unsorted linked lists is to append one list to the end of the other. Here’s a Python implementation:
def merge_unsorted_lists(list1, list2):
if not list1:
return list2
if not list2:
return list1
current = list1
while current.next:
current = current.next
current.next = list2
return list1
This method has a time complexity of O(n), where n is the length of the first list, as we need to traverse to its end. The space complexity is O(1) since we’re modifying the lists in-place.
Handling Edge Cases
When merging linked lists, always consider these edge cases:
- One or both lists are empty
- Lists have different lengths
- Circular linked lists (if applicable)
Proper handling of these cases ensures robust and error-free code.
3. Merging Sorted Linked Lists
Merging sorted linked lists is a more common and slightly more complex operation. It’s frequently asked in coding interviews and is a fundamental part of the merge sort algorithm.
Algorithm for Merging Two Sorted Linked Lists
- Create a dummy node as the start of the result list.
- Initialize a current pointer to the dummy node.
- Compare the values of nodes from both lists.
- Append the smaller value to the result list and move the pointer in that list.
- Repeat steps 3-4 until one list is exhausted.
- Append any remaining nodes from the non-empty list.
- Return the next of the dummy node (the actual head of the merged list).
Implementation in Python
def merge_sorted_lists(list1, list2):
dummy = ListNode(0)
current = dummy
while list1 and list2:
if list1.val <= list2.val:
current.next = list1
list1 = list1.next
else:
current.next = list2
list2 = list2.next
current = current.next
if list1:
current.next = list1
if list2:
current.next = list2
return dummy.next
This algorithm has a time complexity of O(n + m), where n and m are the lengths of the input lists. The space complexity is O(1) as we’re creating only a constant number of new nodes.
Recursive Approach
We can also implement this algorithm recursively:
def merge_sorted_lists_recursive(list1, list2):
if not list1:
return list2
if not list2:
return list1
if list1.val <= list2.val:
list1.next = merge_sorted_lists_recursive(list1.next, list2)
return list1
else:
list2.next = merge_sorted_lists_recursive(list1, list2.next)
return list2
While elegant, the recursive approach may lead to stack overflow for very long lists.
4. K-way Merge of Sorted Linked Lists
K-way merging is an extension of merging two sorted lists, where we need to merge k sorted linked lists into a single sorted linked list. This problem is more challenging and often appears in advanced coding interviews.
Approaches to K-way Merging
- Brute Force: Repeatedly merge two lists at a time.
- Using a Min-Heap: More efficient approach using a priority queue.
- Divide and Conquer: Recursively merge lists in pairs.
Implementation Using a Min-Heap
Here’s an efficient implementation using a min-heap in Python:
import heapq
def merge_k_sorted_lists(lists):
heap = []
dummy = ListNode(0)
current = dummy
# Add the first node of each list to the heap
for i, lst in enumerate(lists):
if lst:
heapq.heappush(heap, (lst.val, i, lst))
while heap:
val, i, node = heapq.heappop(heap)
current.next = node
current = current.next
if node.next:
heapq.heappush(heap, (node.next.val, i, node.next))
return dummy.next
This algorithm has a time complexity of O(N log k), where N is the total number of nodes across all lists, and k is the number of lists. The space complexity is O(k) for the heap.
5. In-place Merging Techniques
In-place merging is a technique where we merge linked lists without using additional data structures, which can be crucial in memory-constrained environments.
In-place Merge of Two Sorted Lists
Here’s an in-place algorithm for merging two sorted lists:
def merge_two_lists_in_place(list1, list2):
if not list1:
return list2
if not list2:
return list1
# Ensure list1 starts with the smaller value
if list1.val > list2.val:
list1, list2 = list2, list1
current = list1
while current.next and list2:
if current.next.val > list2.val:
temp = current.next
current.next = list2
list2 = temp
current = current.next
if list2:
current.next = list2
return list1
This in-place merging technique maintains a time complexity of O(n + m) while achieving O(1) space complexity.
6. Optimization Strategies
When dealing with linked list merging, several optimization strategies can improve performance:
Tail Tracking
Keep track of the tail of the merged list to avoid traversing the entire list for each append operation.
Early Termination
In sorted list merging, if one list is exhausted, simply append the remainder of the other list instead of continuing comparisons.
Minimizing Pointer Updates
Reduce the number of pointer updates by comparing nodes in batches, especially useful in k-way merging.
Memory Management
In languages with manual memory management, efficient allocation and deallocation of nodes can significantly impact performance.
7. Common Pitfalls and How to Avoid Them
When working with linked list merging, be aware of these common pitfalls:
Losing References
Always ensure you maintain proper references to avoid losing parts of the list during merging.
Infinite Loops
Be cautious when dealing with circular linked lists or when updating pointers to avoid creating unintended cycles.
Unhandled Edge Cases
Always consider and handle edge cases like empty lists or lists of different lengths.
Inefficient Comparisons
In k-way merging, avoid comparing all list heads in each iteration. Use efficient data structures like heaps.
8. Interview Tips for Linked List Merging Questions
When faced with a linked list merging problem in an interview, consider these tips:
- Clarify the input: Are the lists sorted? How many lists are there?
- Discuss trade-offs between different approaches (e.g., recursive vs. iterative, in-place vs. using extra space).
- Start with a simple approach and then optimize.
- Think aloud and explain your thought process.
- Test your solution with various edge cases.
- Analyze the time and space complexity of your solution.
9. Advanced Topics in Linked List Merging
For those looking to deepen their understanding, consider these advanced topics:
Parallel Merging
Explore techniques for merging linked lists in parallel, leveraging multi-core processors.
External Sorting
Understand how linked list merging applies to external sorting algorithms for handling large datasets that don’t fit in memory.
Functional Programming Approaches
Investigate how linked list merging can be implemented using functional programming paradigms.
Concurrent Linked Lists
Study techniques for merging concurrent or lock-free linked lists in multi-threaded environments.
10. Conclusion
Mastering linked list merging is a crucial skill for any programmer, especially those aiming for positions at top tech companies. It combines fundamental data structure knowledge with algorithm design and optimization techniques. By understanding the various approaches to linked list merging – from basic concatenation to complex k-way merges – you’ll be well-equipped to tackle a wide range of programming challenges.
Remember, the key to excelling in linked list operations is practice. Implement these algorithms, experiment with different scenarios, and challenge yourself with variations of merging problems. As you gain proficiency, you’ll find that these skills transfer to many other areas of computer science and software development.
Keep exploring, keep coding, and never stop learning. The world of algorithms and data structures is vast and fascinating, with linked list merging being just one piece of the puzzle in your journey to becoming an expert programmer.