How to Handle Invalid Input in Your Code: A Comprehensive Guide
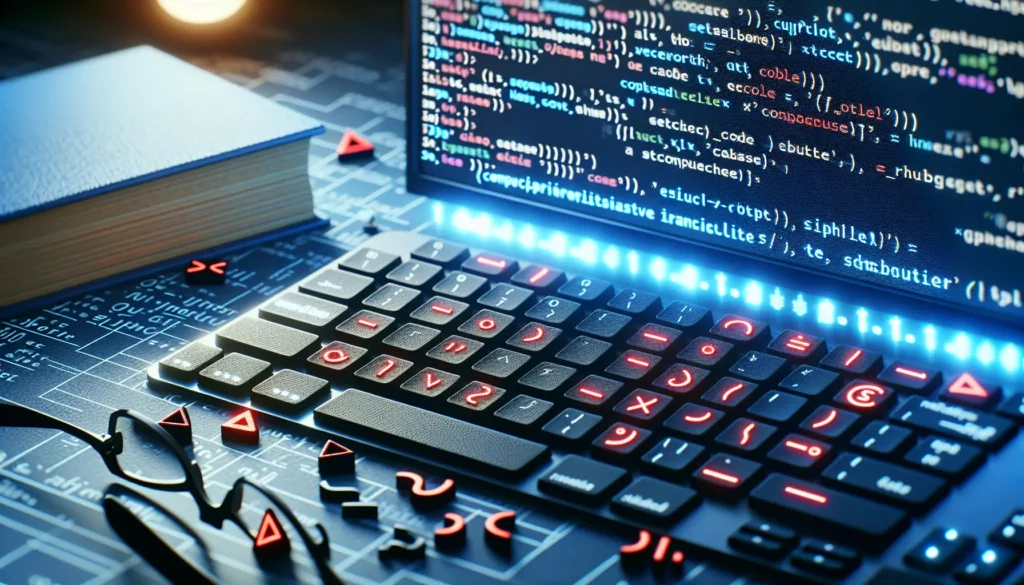
As programmers, we often focus on writing code that works perfectly when given the right input. However, in real-world applications, users don’t always provide the data we expect. This is where input validation becomes crucial. In this comprehensive guide, we’ll explore various techniques and best practices for handling invalid input in your code, ensuring your programs are robust, secure, and user-friendly.
Why Input Validation Matters
Before diving into the specifics, let’s understand why input validation is so important:
- Security: Prevents malicious input that could lead to security vulnerabilities like SQL injection or cross-site scripting (XSS).
- Reliability: Ensures your program behaves predictably, even with unexpected input.
- User Experience: Provides helpful feedback to users when they make mistakes.
- Data Integrity: Maintains the quality and consistency of data in your system.
- Performance: Avoids unnecessary processing of invalid data, potentially saving computational resources.
Types of Input Validation
Input validation can be broadly categorized into several types:
1. Type Checking
Ensures that the input is of the correct data type (e.g., integer, string, float).
2. Range Checking
Verifies that numeric input falls within an acceptable range.
3. Length Checking
Confirms that string inputs meet minimum and maximum length requirements.
4. Format Checking
Validates that input matches a specific pattern or format (e.g., email addresses, phone numbers).
5. Consistency Checking
Ensures that related pieces of information are logically consistent with each other.
Implementing Input Validation
Now, let’s explore how to implement these validation techniques in various programming languages.
Python
Python offers several built-in functions and libraries for input validation:
Type Checking
def validate_age(age):
try:
age = int(age)
if age < 0 or age > 120:
raise ValueError("Age must be between 0 and 120")
return age
except ValueError:
raise ValueError("Invalid age input. Please enter a number.")
Regular Expressions for Format Checking
import re
def validate_email(email):
pattern = r'^[\w\.-]+@[\w\.-]+\.\w+$'
if re.match(pattern, email):
return email
else:
raise ValueError("Invalid email format")
JavaScript
JavaScript provides various methods for input validation, especially useful in web development:
Form Validation
<form id="myForm" onsubmit="return validateForm()">
Name: <input type="text" id="name">
Age: <input type="number" id="age">
<input type="submit" value="Submit">
</form>
<script>
function validateForm() {
let name = document.getElementById("name").value;
let age = document.getElementById("age").value;
if (name == "") {
alert("Name must be filled out");
return false;
}
if (isNaN(age) || age < 1 || age > 120) {
alert("Age must be a number between 1 and 120");
return false;
}
return true;
}
</script>
Java
Java provides robust options for input validation:
Using Exception Handling
import java.util.Scanner;
public class InputValidation {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int age;
while (true) {
System.out.print("Enter your age: ");
try {
age = Integer.parseInt(scanner.nextLine());
if (age < 0 || age > 120) {
throw new IllegalArgumentException("Age must be between 0 and 120");
}
break;
} catch (NumberFormatException e) {
System.out.println("Invalid input. Please enter a number.");
} catch (IllegalArgumentException e) {
System.out.println(e.getMessage());
}
}
System.out.println("Your age is: " + age);
scanner.close();
}
}
Best Practices for Input Validation
To ensure effective input validation, consider the following best practices:
1. Validate on Both Client and Server Side
Client-side validation provides immediate feedback to users, while server-side validation ensures security and data integrity.
2. Use Whitelisting Over Blacklisting
Define what is allowed rather than what isn’t. This approach is generally more secure and easier to maintain.
3. Sanitize Input
Remove or encode potentially harmful characters to prevent security vulnerabilities.
4. Provide Clear Error Messages
Help users understand what went wrong and how to correct their input.
5. Handle Edge Cases
Consider extreme values, empty inputs, and other edge cases in your validation logic.
6. Use Built-in Validation Functions
Many programming languages and frameworks offer built-in validation functions. Use these when available for efficiency and reliability.
Advanced Input Validation Techniques
As you become more proficient in handling invalid input, consider these advanced techniques:
1. Data Normalization
Standardize input data to a consistent format before validation. For example, converting all text to lowercase or removing extra whitespace.
def normalize_email(email):
return email.strip().lower()
2. Cross-Field Validation
Validate related fields together to ensure logical consistency.
def validate_date_range(start_date, end_date):
if start_date > end_date:
raise ValueError("Start date must be before end date")
3. Asynchronous Validation
For web applications, perform certain validations asynchronously to improve user experience.
async function checkUsernameAvailability(username) {
const response = await fetch(`/api/check-username?username=${username}`);
const data = await response.json();
return data.available;
}
4. Custom Validation Rules
Implement domain-specific validation rules that go beyond basic type and format checking.
def validate_product_code(code):
if not code.startswith('PRD-'):
raise ValueError("Product code must start with 'PRD-'")
if len(code) != 10:
raise ValueError("Product code must be 10 characters long")
# Additional checks specific to your product coding system
Handling Invalid Input in Different Contexts
The approach to handling invalid input can vary depending on the context of your application. Let’s explore some specific scenarios:
Command-Line Applications
For command-line tools, clear and concise error messages are crucial. Consider using a library like Python’s argparse
for robust argument parsing and validation.
import argparse
parser = argparse.ArgumentParser(description='Process some integers.')
parser.add_argument('integers', metavar='N', type=int, nargs='+',
help='an integer for the accumulator')
parser.add_argument('--sum', dest='accumulate', action='store_const',
const=sum, default=max,
help='sum the integers (default: find the max)')
args = parser.parse_args()
print(args.accumulate(args.integers))
Web Applications
In web applications, consider using a combination of client-side and server-side validation. Many web frameworks provide built-in validation features:
Django (Python)
from django import forms
class UserForm(forms.Form):
username = forms.CharField(max_length=100)
email = forms.EmailField()
age = forms.IntegerField(min_value=0, max_value=120)
def clean_username(self):
username = self.cleaned_data['username']
if User.objects.filter(username=username).exists():
raise forms.ValidationError("Username already exists")
return username
Express (Node.js)
const { body, validationResult } = require('express-validator');
app.post('/user',
body('username').isLength({ min: 5 }),
body('email').isEmail(),
body('age').isInt({ min: 0, max: 120 }),
(req, res) => {
const errors = validationResult(req);
if (!errors.isEmpty()) {
return res.status(400).json({ errors: errors.array() });
}
// Process valid input
});
Database Interactions
When working with databases, it’s crucial to validate input to prevent SQL injection and ensure data integrity:
import mysql.connector
from mysql.connector import Error
def insert_user(username, email, age):
try:
connection = mysql.connector.connect(host='localhost',
database='users_db',
user='user',
password='password')
cursor = connection.cursor(prepared=True)
# Using parameterized query to prevent SQL injection
sql_insert_query = """INSERT INTO users (username, email, age)
VALUES (%s, %s, %s)"""
# Validate input before insertion
if not username or len(username) > 100:
raise ValueError("Invalid username")
if not '@' in email or len(email) > 255:
raise ValueError("Invalid email")
if not isinstance(age, int) or age < 0 or age > 120:
raise ValueError("Invalid age")
input_data = (username, email, age)
cursor.execute(sql_insert_query, input_data)
connection.commit()
print("User inserted successfully")
except Error as e:
print(f"Error: {e}")
finally:
if connection.is_connected():
cursor.close()
connection.close()
Testing Input Validation
Thorough testing is essential to ensure your input validation is working correctly. Here are some approaches:
Unit Testing
Write unit tests to check various scenarios, including valid inputs, edge cases, and invalid inputs.
import unittest
class TestInputValidation(unittest.TestCase):
def test_validate_age_valid(self):
self.assertEqual(validate_age("25"), 25)
def test_validate_age_invalid_type(self):
with self.assertRaises(ValueError):
validate_age("twenty-five")
def test_validate_age_out_of_range(self):
with self.assertRaises(ValueError):
validate_age("150")
if __name__ == '__main__':
unittest.main()
Fuzz Testing
Use fuzz testing to generate random, unexpected inputs and ensure your validation handles them gracefully.
Integration Testing
Test input validation as part of larger system tests to ensure it works correctly in the context of your entire application.
Common Pitfalls in Input Validation
Be aware of these common mistakes when implementing input validation:
1. Trusting Client-Side Validation Alone
Always implement server-side validation, as client-side checks can be bypassed.
2. Overreliance on Type Conversion
Don’t assume type conversion will always work or produce the expected result.
3. Neglecting to Handle Unicode
Ensure your validation can handle non-ASCII characters and different encodings.
4. Insufficient Error Handling
Provide clear, specific error messages to guide users in correcting their input.
5. Not Considering Performance
Overly complex validation can impact performance, especially with large datasets.
Conclusion
Handling invalid input is a critical aspect of writing robust, secure, and user-friendly code. By implementing thorough input validation, you can prevent errors, enhance security, and improve the overall quality of your software. Remember to validate input on both client and server sides, use appropriate techniques for different types of data, and always consider the specific requirements of your application.
As you continue to develop your programming skills, make input validation an integral part of your coding practice. It’s not just about preventing errors; it’s about creating software that users can trust and rely on. Keep exploring new validation techniques and stay updated on best practices to ensure your applications remain secure and efficient in an ever-evolving digital landscape.