How to Handle Interviewer Feedback and Guidance During the Interview
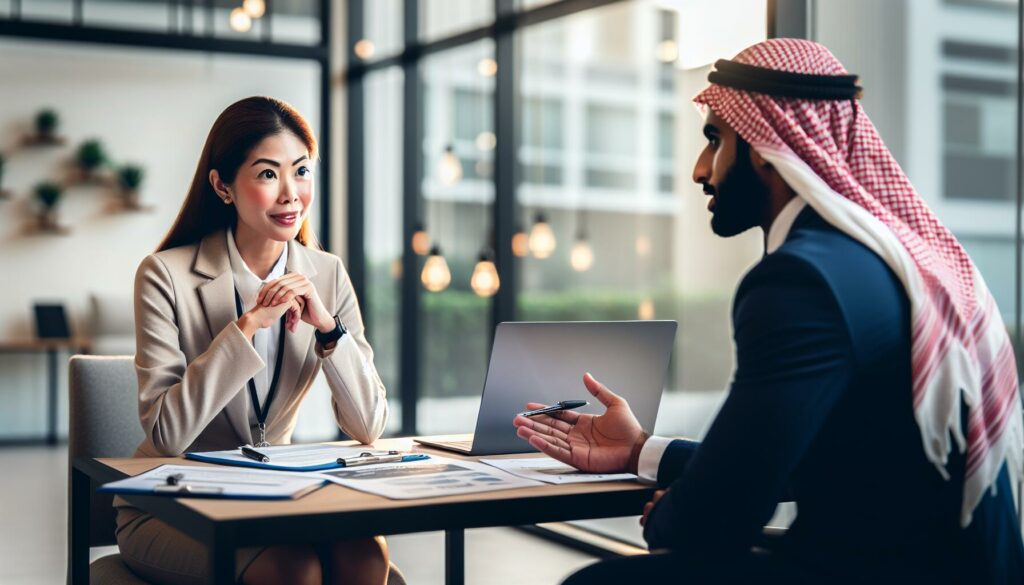
Technical interviews, especially those for prestigious companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), can be nerve-wracking experiences. One crucial aspect that can make or break your performance is how you handle feedback and guidance from your interviewer. In this comprehensive guide, we’ll explore effective strategies for responding to interviewer input, maintaining a positive attitude, and showcasing your adaptability – all essential skills for acing your coding interview.
The Importance of Interviewer Feedback
Before diving into specific strategies, it’s crucial to understand why interviewer feedback is so valuable:
- It provides insight into the interviewer’s thought process
- It helps you align your approach with the company’s expectations
- It offers an opportunity to demonstrate your ability to learn and adapt quickly
- It can guide you towards a more optimal solution
Recognizing the value of this feedback is the first step in leveraging it to your advantage during the interview process.
Strategies for Handling Interviewer Feedback
1. Active Listening
The foundation of effectively handling interviewer feedback is active listening. This involves:
- Maintaining eye contact (if in-person) or focused attention (if virtual)
- Nodding to show understanding
- Avoiding interruptions
- Taking brief notes if necessary
By demonstrating that you’re fully engaged and receptive to their input, you create a positive impression and ensure you don’t miss any crucial information.
2. Clarify When Necessary
If you’re unsure about any aspect of the feedback or guidance, don’t hesitate to ask for clarification. This shows that you’re committed to understanding and implementing their suggestions correctly. You might say something like:
“Thank you for that feedback. To make sure I understand correctly, are you suggesting that I should consider using a hash table instead of an array for this problem?”
3. Acknowledge and Appreciate
Always acknowledge the feedback you receive and express appreciation. This demonstrates professionalism and a positive attitude. For example:
“I appreciate that suggestion. It’s a perspective I hadn’t considered, and I can see how it could lead to a more efficient solution.”
4. Incorporate Feedback Thoughtfully
When you receive feedback or guidance, take a moment to consider how you can incorporate it into your approach. This might involve:
- Modifying your current solution
- Starting over with a new approach
- Asking follow-up questions to ensure you’re on the right track
Remember, the goal is to show that you can take constructive input and use it effectively.
5. Verbalize Your Thought Process
As you incorporate the feedback, explain your thought process out loud. This gives the interviewer insight into how you reason through problems and adapt to new information. For example:
“Based on your suggestion about considering space complexity, I’m thinking we could optimize this solution by using a two-pointer approach instead. This would reduce our space complexity from O(n) to O(1). Let me walk you through how that would work…”
6. Stay Calm and Positive
Receiving feedback, especially if it points out flaws in your initial approach, can be stressful. It’s crucial to maintain a calm and positive demeanor. Remember, the interviewer is not trying to trip you up – they’re assessing your problem-solving skills and how you handle challenges.
7. Use Feedback as a Learning Opportunity
View each piece of feedback as a chance to learn and improve. Even if you don’t get the job, the insights you gain from interviewer feedback can be invaluable for your future interviews and overall coding skills.
Balancing Independence and Receptiveness
While it’s important to be open to feedback, you also want to demonstrate your ability to think independently. Here are some tips for striking the right balance:
1. Start with Your Own Approach
Begin by presenting your own solution or approach to the problem. This shows initiative and independent thinking. If the interviewer then offers feedback, you can incorporate it into your existing framework.
2. Explain Your Reasoning
When you propose a solution, explain your reasoning behind it. This demonstrates your problem-solving process and gives the interviewer context for any feedback they might offer.
3. Ask Thoughtful Questions
Instead of immediately asking for help or guidance, try to work through challenges on your own first. If you do need assistance, ask specific, well-thought-out questions that show you’ve put effort into solving the problem.
4. Propose Alternatives
If the interviewer suggests an approach, and you see potential issues or have ideas for improvement, respectfully propose your alternatives. This shows critical thinking and confidence in your abilities.
Practical Examples
Let’s look at some practical examples of handling interviewer feedback during a coding interview:
Example 1: Optimizing a Solution
Interviewer: “Your current solution works, but it has a time complexity of O(n^2). Can you think of a way to optimize it?”
Good Response: “Thank you for that feedback. You’re right, the nested loops are causing the O(n^2) complexity. Let me think about this for a moment… We could potentially use a hash map to reduce the time complexity to O(n). Would you like me to explain how we could implement that?”
Example 2: Addressing an Edge Case
Interviewer: “Your solution looks good, but have you considered what would happen if the input array is empty?”
Good Response: “That’s an excellent point, thank you for bringing it up. I should have considered that edge case. Let me modify my code to handle an empty array input. We could add a check at the beginning of the function like this:”
if (arr.length === 0) {
return null; // or any appropriate value for an empty input
}
“This ensures our function handles empty inputs gracefully. Is there any other edge case you think I should consider?”
Example 3: Guidance on Approach
Interviewer: “For this problem, have you considered using a stack data structure?”
Good Response: “I appreciate that suggestion. I hadn’t considered using a stack, but I can see how it could be beneficial for this problem. A stack could help us keep track of the elements in a last-in-first-out order, which seems particularly useful for this scenario. Would you mind if I take a moment to think about how we could implement this using a stack?”
Showcasing Adaptability
Adaptability is a crucial skill in the fast-paced tech industry, and interviewers often look for candidates who can quickly adjust their approach based on new information or changing requirements. Here are some ways to showcase your adaptability during the interview:
1. Embrace New Approaches
If the interviewer suggests a different method or algorithm, show enthusiasm for learning and applying this new approach. This demonstrates your willingness to expand your knowledge and try new things.
2. Quickly Pivot When Necessary
If your initial solution isn’t optimal or doesn’t meet all the requirements, be ready to pivot quickly. Explain your new thought process as you make changes, showing how you’re incorporating the new information or constraints.
3. Connect New Concepts to Prior Knowledge
When presented with a new idea or approach, try to connect it to concepts you’re already familiar with. This shows that you can integrate new information with your existing knowledge base.
4. Demonstrate Learning from Mistakes
If you make a mistake or overlook something, acknowledge it openly and show how you’re learning from it. This could involve explaining how you’d prevent similar mistakes in the future or how this new understanding changes your approach to problem-solving.
The Role of Soft Skills
While technical skills are crucial in coding interviews, soft skills play an equally important role, especially when it comes to handling feedback and guidance. Here are some key soft skills to focus on:
1. Communication
Clear and effective communication is essential when receiving and responding to feedback. Practice explaining your thoughts concisely and asking clear, specific questions.
2. Emotional Intelligence
Being able to read the interviewer’s tone and adjust your responses accordingly is a valuable skill. This includes recognizing when the interviewer is trying to guide you versus when they’re assessing your independent problem-solving skills.
3. Resilience
Coding interviews can be challenging, and you may receive critical feedback. Demonstrating resilience by maintaining a positive attitude and continuing to put forth your best effort is crucial.
4. Teamwork
Even though you’re the one being interviewed, the process of receiving and incorporating feedback is a form of collaboration. Show that you can work effectively with others by being receptive to input and building upon shared ideas.
Preparing for Feedback Scenarios
While you can’t predict exactly what feedback you’ll receive in an interview, you can prepare yourself to handle various scenarios effectively:
1. Practice with Mock Interviews
Engage in mock interviews where you receive real-time feedback. This can help you get comfortable with the process of receiving and acting on input during a high-pressure situation.
2. Review Common Feedback Points
Familiarize yourself with common types of feedback in coding interviews, such as:
- Optimization suggestions
- Requests to consider edge cases
- Guidance on different data structures or algorithms
- Feedback on code style or best practices
Understanding these common feedback points can help you respond more effectively when you encounter them.
3. Develop a Feedback Mindset
Cultivate a mindset that views all feedback as an opportunity for growth. This positive attitude will shine through in your interviews and make you more receptive to guidance.
Conclusion
Handling interviewer feedback and guidance effectively is a crucial skill for success in coding interviews, especially for prestigious companies like FAANG. By actively listening, thoughtfully incorporating feedback, and demonstrating adaptability, you can showcase not only your technical skills but also your ability to learn, collaborate, and thrive in a dynamic work environment.
Remember, the interviewer’s feedback is not just about assessing your current knowledge – it’s also an opportunity to demonstrate your potential for growth and your ability to work well with others. Approach each piece of feedback as a chance to improve and shine, and you’ll be well on your way to impressing your interviewers and landing your dream job in tech.
As you continue to prepare for your coding interviews, consider using platforms like AlgoCademy to hone your skills. With interactive coding tutorials, AI-powered assistance, and a focus on algorithmic thinking and problem-solving, AlgoCademy can help you build the confidence and competence you need to excel in even the most challenging technical interviews.