How to Handle Exceptions and Write Robust Code
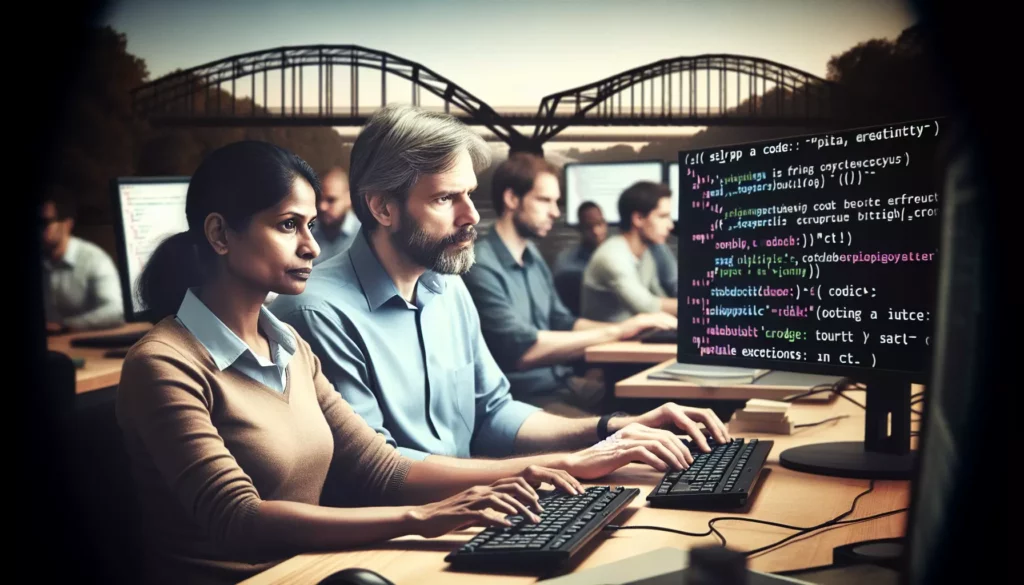
In the world of programming, errors and unexpected situations are inevitable. As developers, it’s our responsibility to anticipate these issues and write code that can gracefully handle them. This is where exception handling comes into play. By mastering exception handling techniques, you can create more robust, reliable, and user-friendly applications. In this comprehensive guide, we’ll explore the ins and outs of exception handling and provide you with practical strategies to write more resilient code.
Understanding Exceptions
Before diving into exception handling techniques, it’s crucial to understand what exceptions are and why they occur. An exception is an event that disrupts the normal flow of a program’s execution. These can be caused by various factors, such as:
- Invalid user input
- Hardware failures
- Network issues
- Programming errors
- Resource constraints
When an exception occurs, it creates an object containing information about the error, including its type and the state of the program when the error occurred. If not properly handled, exceptions can cause your program to crash or produce unexpected results.
The Importance of Exception Handling
Exception handling is a programming construct designed to handle runtime errors gracefully. It allows you to:
- Separate error-handling code from regular code
- Propagate errors up the call stack
- Group and differentiate error types
- Create meaningful error messages for users or logging systems
By implementing proper exception handling, you can improve the reliability, maintainability, and user experience of your applications.
Basic Exception Handling Techniques
1. Try-Catch Blocks
The most common method of handling exceptions is using try-catch blocks. Here’s a basic structure:
try {
// Code that might throw an exception
} catch (ExceptionType e) {
// Code to handle the exception
}
The code inside the try block is executed, and if an exception occurs, it’s caught by the appropriate catch block. You can have multiple catch blocks to handle different types of exceptions.
2. Finally Block
The finally block is used to execute code regardless of whether an exception was thrown or not. It’s often used for cleanup operations:
try {
// Code that might throw an exception
} catch (ExceptionType e) {
// Code to handle the exception
} finally {
// Code that will always execute
}
3. Throwing Exceptions
You can also throw exceptions explicitly using the throw keyword:
if (someCondition) {
throw new ExceptionType("Error message");
}
This is useful when you want to signal an error condition in your own code.
Advanced Exception Handling Strategies
1. Custom Exceptions
Creating custom exceptions allows you to define application-specific error types. This can make your code more readable and easier to maintain:
public class CustomException extends Exception {
public CustomException(String message) {
super(message);
}
}
2. Exception Chaining
Exception chaining involves wrapping a lower-level exception within a higher-level one. This preserves the original error information while providing a more appropriate exception for the current context:
try {
// Some low-level operation
} catch (LowLevelException e) {
throw new HighLevelException("High-level operation failed", e);
}
3. Try-with-Resources
Introduced in Java 7, try-with-resources automatically closes resources that implement the AutoCloseable interface:
try (BufferedReader br = new BufferedReader(new FileReader(path))) {
// Use the resource
} catch (IOException e) {
// Handle exception
}
This ensures that resources are properly closed, even if an exception occurs.
Best Practices for Exception Handling
1. Be Specific with Exception Types
Catch the most specific exception types possible. Avoid catching generic exceptions like Exception unless absolutely necessary:
try {
// Code that might throw IOException or SQLException
} catch (IOException e) {
// Handle IOException
} catch (SQLException e) {
// Handle SQLException
}
2. Don’t Catch Exceptions You Can’t Handle
If you can’t meaningfully recover from an exception, it’s often better to let it propagate up the call stack:
public void doSomething() throws SomeException {
// Method implementation
}
3. Log Exceptions Properly
When catching exceptions, make sure to log them with sufficient context:
try {
// Some operation
} catch (SomeException e) {
logger.error("Failed to perform operation: " + e.getMessage(), e);
}
4. Avoid Empty Catch Blocks
Empty catch blocks can hide important errors. If you must catch an exception without handling it, at least log it:
try {
// Some operation
} catch (SomeException e) {
logger.warn("Ignoring exception", e);
}
5. Use Finally Blocks or Try-with-Resources for Cleanup
Ensure that resources are always properly closed, even if an exception occurs:
FileInputStream fis = null;
try {
fis = new FileInputStream("file.txt");
// Use the file
} catch (IOException e) {
// Handle exception
} finally {
if (fis != null) {
try {
fis.close();
} catch (IOException e) {
// Handle close exception
}
}
}
Exception Handling in Different Programming Languages
Java
Java has a robust exception handling mechanism with checked and unchecked exceptions:
try {
int result = someMethod();
} catch (IOException e) {
System.err.println("IO error: " + e.getMessage());
} catch (SQLException e) {
System.err.println("Database error: " + e.getMessage());
} finally {
// Cleanup code
}
Python
Python uses a try-except-finally structure for exception handling:
try:
result = some_function()
except IOError as e:
print(f"IO error occurred: {e}")
except ValueError as e:
print(f"Value error occurred: {e}")
else:
print("Operation successful")
finally:
# Cleanup code
JavaScript
JavaScript uses try-catch-finally blocks similar to Java:
try {
let result = someFunction();
} catch (error) {
console.error("An error occurred:", error.message);
} finally {
// Cleanup code
}
Handling Asynchronous Exceptions
In asynchronous programming, exception handling can be more challenging. Here are some strategies for different scenarios:
1. Promises (JavaScript)
Use .catch() to handle errors in promise chains:
fetchData()
.then(processData)
.then(displayResult)
.catch(error => console.error("Error:", error));
2. Async/Await (JavaScript, Python)
Use try-catch blocks with async functions:
async function fetchAndProcessData() {
try {
const data = await fetchData();
const result = await processData(data);
return result;
} catch (error) {
console.error("Error:", error);
}
}
3. Reactive Programming (RxJS)
Use operators like catchError to handle exceptions in observables:
observable
.pipe(
map(data => processData(data)),
catchError(error => {
console.error("Error:", error);
return of(null); // Return a default value or a new observable
})
)
.subscribe(result => console.log(result));
Testing Exception Handling
Proper testing of exception handling is crucial to ensure your code behaves correctly in error scenarios. Here are some approaches:
1. Unit Testing
Write tests that deliberately trigger exceptions and verify that they’re handled correctly:
@Test
public void testDivisionByZero() {
try {
int result = divideNumbers(10, 0);
fail("Expected ArithmeticException was not thrown");
} catch (ArithmeticException e) {
assertEquals("Cannot divide by zero", e.getMessage());
}
}
2. Mocking
Use mocking frameworks to simulate exceptions from dependencies:
@Test
public void testDatabaseConnectionFailure() {
DatabaseConnection mockConnection = mock(DatabaseConnection.class);
when(mockConnection.connect()).thenThrow(new SQLException("Connection failed"));
try {
databaseService.performOperation(mockConnection);
fail("Expected DatabaseException was not thrown");
} catch (DatabaseException e) {
assertEquals("Database operation failed: Connection failed", e.getMessage());
}
}
3. Integration Testing
Perform integration tests to ensure that exception handling works correctly across different components of your system.
Performance Considerations
While exception handling is crucial for robust code, it can impact performance if not used judiciously:
1. Avoid Using Exceptions for Control Flow
Exceptions should be used for exceptional circumstances, not for regular control flow. Using exceptions for expected scenarios can significantly slow down your application.
2. Be Mindful of Exception Creation Cost
Creating exception objects and capturing stack traces can be expensive. In performance-critical sections, consider alternatives like returning error codes for expected error conditions.
3. Use Custom Exceptions Wisely
While custom exceptions can improve code readability, creating too many exception classes can lead to increased memory usage and longer class loading times.
Debugging with Exceptions
Exceptions can be valuable tools for debugging:
1. Stack Traces
Exception stack traces provide valuable information about where and why an error occurred. Learn to read and interpret them effectively.
2. Logging
Implement comprehensive logging in your exception handling code to aid in troubleshooting:
try {
// Some operation
} catch (Exception e) {
logger.error("Operation failed", e);
throw new CustomException("Operation failed", e);
}
3. Debugging Tools
Familiarize yourself with your IDE’s debugging tools, which often provide features for setting exception breakpoints and analyzing exception objects.
Exception Handling in Design Patterns
Many design patterns incorporate exception handling as part of their implementation:
1. Factory Method
Use exceptions to signal creation failures in factory methods:
public static Connection createConnection(String type) throws ConnectionException {
switch (type) {
case "mysql":
return new MySQLConnection();
case "postgresql":
return new PostgreSQLConnection();
default:
throw new ConnectionException("Unsupported database type: " + type);
}
}
2. Template Method
Handle exceptions in the template method to provide consistent error handling across different implementations:
public abstract class DataProcessor {
public final void processData() {
try {
readData();
analyzeData();
writeResults();
} catch (IOException e) {
handleIOException(e);
} catch (DataFormatException e) {
handleDataFormatException(e);
}
}
protected abstract void readData() throws IOException;
protected abstract void analyzeData() throws DataFormatException;
protected abstract void writeResults() throws IOException;
protected void handleIOException(IOException e) {
System.err.println("IO error: " + e.getMessage());
}
protected void handleDataFormatException(DataFormatException e) {
System.err.println("Data format error: " + e.getMessage());
}
}
Exception Handling in Multithreaded Environments
Handling exceptions in multithreaded applications presents unique challenges:
1. Uncaught Exception Handlers
Set up uncaught exception handlers to deal with exceptions that occur in separate threads:
Thread.setDefaultUncaughtExceptionHandler((thread, throwable) -> {
System.err.println("Uncaught exception in thread " + thread.getName() + ": " + throwable.getMessage());
// Log the exception, notify administrators, etc.
});
2. Future and CompletableFuture (Java)
When working with Future or CompletableFuture, use methods like get() or join() carefully, as they can throw exceptions:
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> {
// Some operation that might throw an exception
return "Result";
});
try {
String result = future.get();
} catch (InterruptedException | ExecutionException e) {
System.err.println("Error in async operation: " + e.getMessage());
}
3. ExecutorService
When using ExecutorService, be aware that exceptions in submitted tasks won’t automatically propagate to the calling thread:
ExecutorService executor = Executors.newFixedThreadPool(4);
Future<String> future = executor.submit(() -> {
if (someCondition) {
throw new RuntimeException("Task failed");
}
return "Task completed";
});
try {
String result = future.get();
} catch (InterruptedException | ExecutionException e) {
System.err.println("Task execution failed: " + e.getMessage());
}
Conclusion
Exception handling is a critical skill for writing robust, reliable code. By understanding the principles and best practices outlined in this guide, you’ll be better equipped to handle errors gracefully, improve the user experience of your applications, and write code that’s easier to maintain and debug.
Remember that effective exception handling is about more than just preventing crashes. It’s about creating resilient systems that can recover from errors, provide meaningful feedback, and maintain data integrity even in the face of unexpected issues.
As you continue to develop your programming skills, make exception handling an integral part of your coding practice. Regularly review and refine your error handling strategies, and always consider how your code might fail and how you can gracefully handle those failures.
By mastering exception handling, you’ll not only write better code but also be better prepared for the challenges of real-world software development, where dealing with errors and unexpected situations is a daily occurrence.