How to Handle Duplicate Values Efficiently: A Comprehensive Guide
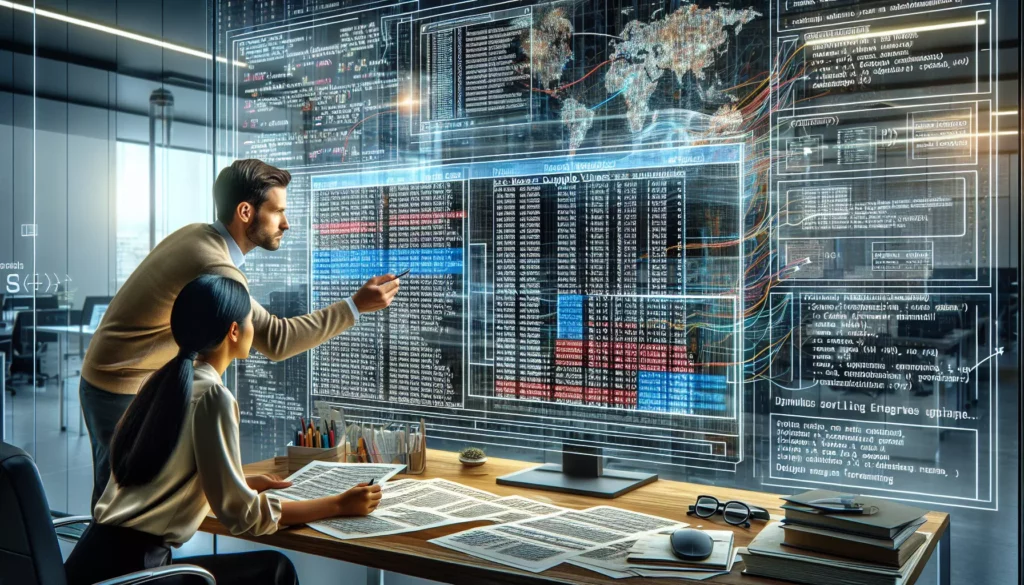
In the world of programming and data management, dealing with duplicate values is a common challenge that developers face. Whether you’re working on a small project or a large-scale application, knowing how to handle duplicate values efficiently can significantly improve your code’s performance and data integrity. In this comprehensive guide, we’ll explore various techniques and best practices for managing duplicate values across different programming languages and data structures.
Table of Contents
- Understanding Duplicate Values
- Identifying Duplicate Values
- Removing Duplicate Values
- Preventing Duplicate Values
- Handling Duplicates in Different Data Structures
- Efficient Algorithms for Handling Duplicates
- Dealing with Duplicates in Databases
- Real-World Examples and Use Cases
- Best Practices and Performance Considerations
- Conclusion
1. Understanding Duplicate Values
Before diving into the methods of handling duplicate values, it’s essential to understand what they are and why they occur. Duplicate values are instances of the same data appearing more than once in a dataset or collection. They can arise due to various reasons, such as:
- Data entry errors
- Merging datasets from different sources
- System glitches or bugs
- Intentional data redundancy for specific use cases
While some duplicates might be intentional and necessary, unintended duplicates can lead to several issues:
- Increased storage requirements
- Reduced data quality and integrity
- Inaccurate analysis and reporting
- Performance degradation in data processing
2. Identifying Duplicate Values
The first step in handling duplicate values is to identify them. Here are some common methods for detecting duplicates:
2.1. Using Sets
Sets are data structures that only allow unique elements. By converting a collection to a set and comparing the sizes, you can quickly determine if duplicates exist.
def has_duplicates(items):
return len(items) != len(set(items))
# Example usage
numbers = [1, 2, 3, 4, 2, 5]
print(has_duplicates(numbers)) # Output: True
2.2. Sorting and Comparing
For larger datasets, sorting the elements and comparing adjacent items can be an efficient way to identify duplicates.
def find_duplicates(items):
sorted_items = sorted(items)
duplicates = []
for i in range(1, len(sorted_items)):
if sorted_items[i] == sorted_items[i-1]:
duplicates.append(sorted_items[i])
return duplicates
# Example usage
numbers = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5]
print(find_duplicates(numbers)) # Output: [1, 3, 5]
2.3. Using Hash Tables
Hash tables provide a fast way to count occurrences of elements and identify duplicates.
from collections import Counter
def find_duplicates_with_count(items):
count = Counter(items)
return {item: freq for item, freq in count.items() if freq > 1}
# Example usage
words = ['apple', 'banana', 'apple', 'cherry', 'date', 'banana']
print(find_duplicates_with_count(words)) # Output: {'apple': 2, 'banana': 2}
3. Removing Duplicate Values
Once duplicates are identified, the next step is often to remove them. Here are some techniques for removing duplicates:
3.1. Using Sets
Converting a collection to a set and back to the original type is a quick way to remove duplicates while preserving order in Python 3.7+.
def remove_duplicates(items):
return list(dict.fromkeys(items))
# Example usage
numbers = [1, 2, 2, 3, 4, 4, 5]
print(remove_duplicates(numbers)) # Output: [1, 2, 3, 4, 5]
3.2. List Comprehension with Sets
For older Python versions, you can use a list comprehension with a set to remove duplicates while preserving order.
def remove_duplicates_preserve_order(items):
seen = set()
return [x for x in items if not (x in seen or seen.add(x))]
# Example usage
words = ['apple', 'banana', 'apple', 'cherry', 'banana', 'date']
print(remove_duplicates_preserve_order(words)) # Output: ['apple', 'banana', 'cherry', 'date']
3.3. Using Pandas for Dataframes
When working with large datasets in Python, the Pandas library offers efficient methods for removing duplicates.
import pandas as pd
def remove_duplicates_pandas(df, subset=None):
return df.drop_duplicates(subset=subset, keep='first')
# Example usage
data = {'Name': ['John', 'Jane', 'John', 'Mike'],
'Age': [25, 30, 25, 35]}
df = pd.DataFrame(data)
print(remove_duplicates_pandas(df, subset=['Name', 'Age']))
# Output:
# Name Age
# 0 John 25
# 1 Jane 30
# 3 Mike 35
4. Preventing Duplicate Values
Preventing duplicates from occurring in the first place is often more efficient than removing them later. Here are some strategies to prevent duplicates:
4.1. Using Unique Constraints in Databases
When working with databases, you can use unique constraints to prevent duplicate entries.
CREATE TABLE users (
id INT PRIMARY KEY,
email VARCHAR(255) UNIQUE,
name VARCHAR(100)
);
4.2. Implementing Custom Data Structures
You can create custom data structures that inherently prevent duplicates, such as a set-like list.
class UniqueList:
def __init__(self):
self._list = []
self._set = set()
def add(self, item):
if item not in self._set:
self._list.append(item)
self._set.add(item)
def __iter__(self):
return iter(self._list)
# Example usage
unique_list = UniqueList()
unique_list.add(1)
unique_list.add(2)
unique_list.add(1) # This won't be added
print(list(unique_list)) # Output: [1, 2]
4.3. Input Validation
Implement robust input validation to catch and prevent duplicate entries before they enter your system.
def add_user(users, new_user):
if new_user['email'] in [user['email'] for user in users]:
raise ValueError("User with this email already exists")
users.append(new_user)
# Example usage
users = [{'name': 'John', 'email': 'john@example.com'}]
try:
add_user(users, {'name': 'Jane', 'email': 'jane@example.com'})
add_user(users, {'name': 'John', 'email': 'john@example.com'}) # This will raise an error
except ValueError as e:
print(f"Error: {e}")
5. Handling Duplicates in Different Data Structures
Different data structures require different approaches to handle duplicates efficiently. Let’s explore some common data structures and how to manage duplicates in each:
5.1. Arrays and Lists
For arrays and lists, we’ve already covered some methods using sets and sorting. Here’s another approach using a dictionary for counting occurrences:
def find_duplicates_in_array(arr):
count_dict = {}
duplicates = []
for item in arr:
if item in count_dict:
if count_dict[item] == 1:
duplicates.append(item)
count_dict[item] += 1
else:
count_dict[item] = 1
return duplicates
# Example usage
numbers = [1, 2, 3, 4, 2, 5, 6, 3, 7, 8, 8]
print(find_duplicates_in_array(numbers)) # Output: [2, 3, 8]
5.2. Trees
For tree structures, you can use a depth-first search (DFS) approach to identify duplicates:
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def find_duplicates_in_tree(root):
values = {}
duplicates = []
def dfs(node):
if not node:
return
if node.val in values:
if values[node.val] == 1:
duplicates.append(node.val)
values[node.val] += 1
else:
values[node.val] = 1
dfs(node.left)
dfs(node.right)
dfs(root)
return duplicates
# Example usage
root = TreeNode(1)
root.left = TreeNode(2)
root.right = TreeNode(3)
root.left.left = TreeNode(4)
root.left.right = TreeNode(2)
root.right.right = TreeNode(4)
print(find_duplicates_in_tree(root)) # Output: [2, 4]
5.3. Graphs
For graphs, you can use a similar approach to trees, but you need to keep track of visited nodes to avoid infinite loops in cyclic graphs:
from collections import defaultdict
def find_duplicates_in_graph(graph):
values = defaultdict(int)
duplicates = []
visited = set()
def dfs(node):
if node in visited:
return
visited.add(node)
values[graph[node]['value']] += 1
if values[graph[node]['value']] == 2:
duplicates.append(graph[node]['value'])
for neighbor in graph[node]['neighbors']:
dfs(neighbor)
for node in graph:
dfs(node)
return duplicates
# Example usage
graph = {
'A': {'value': 1, 'neighbors': ['B', 'C']},
'B': {'value': 2, 'neighbors': ['D']},
'C': {'value': 3, 'neighbors': ['D']},
'D': {'value': 2, 'neighbors': []}
}
print(find_duplicates_in_graph(graph)) # Output: [2]
6. Efficient Algorithms for Handling Duplicates
When dealing with large datasets, it’s crucial to use efficient algorithms for handling duplicates. Here are some advanced algorithms that can help:
6.1. Bloom Filters
Bloom filters are probabilistic data structures that can quickly check if an element is in a set. They’re great for handling duplicates in large datasets with a small margin of error.
from bitarray import bitarray
import mmh3
class BloomFilter:
def __init__(self, size, hash_count):
self.size = size
self.hash_count = hash_count
self.bit_array = bitarray(size)
self.bit_array.setall(0)
def add(self, item):
for seed in range(self.hash_count):
index = mmh3.hash(item, seed) % self.size
self.bit_array[index] = 1
def check(self, item):
for seed in range(self.hash_count):
index = mmh3.hash(item, seed) % self.size
if self.bit_array[index] == 0:
return False
return True
# Example usage
bf = BloomFilter(1000, 3)
bf.add("apple")
bf.add("banana")
print(bf.check("apple")) # Output: True
print(bf.check("cherry")) # Output: False (probably)
6.2. Count-Min Sketch
Count-Min Sketch is another probabilistic data structure that can estimate the frequency of items in a stream of data, which is useful for identifying potential duplicates.
import numpy as np
import mmh3
class CountMinSketch:
def __init__(self, width, depth):
self.width = width
self.depth = depth
self.sketch = np.zeros((depth, width), dtype=int)
def add(self, item, count=1):
for i in range(self.depth):
j = mmh3.hash(item, i) % self.width
self.sketch[i, j] += count
def estimate(self, item):
return min(self.sketch[i, mmh3.hash(item, i) % self.width] for i in range(self.depth))
# Example usage
cms = CountMinSketch(1000, 5)
cms.add("apple", 3)
cms.add("banana", 2)
cms.add("apple", 1)
print(cms.estimate("apple")) # Output: ~4 (approximate count)
print(cms.estimate("cherry")) # Output: ~0 (approximate count)
6.3. Two-Pointer Technique
For sorted arrays, the two-pointer technique can be an efficient way to remove duplicates in-place:
def remove_duplicates_sorted(arr):
if not arr:
return 0
write_pointer = 1
for read_pointer in range(1, len(arr)):
if arr[read_pointer] != arr[read_pointer - 1]:
arr[write_pointer] = arr[read_pointer]
write_pointer += 1
return write_pointer
# Example usage
numbers = [1, 1, 2, 2, 3, 4, 4, 5]
new_length = remove_duplicates_sorted(numbers)
print(numbers[:new_length]) # Output: [1, 2, 3, 4, 5]
7. Dealing with Duplicates in Databases
When working with databases, handling duplicates becomes even more critical. Here are some techniques for managing duplicates in database systems:
7.1. SQL Queries for Identifying Duplicates
You can use SQL queries to identify duplicate records in a database table:
SELECT column1, column2, COUNT(*)
FROM table_name
GROUP BY column1, column2
HAVING COUNT(*) > 1;
7.2. Removing Duplicates with SQL
To remove duplicates while keeping one instance, you can use a subquery or CTE (Common Table Expression):
WITH cte AS (
SELECT *,
ROW_NUMBER() OVER (PARTITION BY column1, column2 ORDER BY id) AS row_num
FROM table_name
)
DELETE FROM cte WHERE row_num > 1;
7.3. Preventing Duplicates with Unique Constraints
As mentioned earlier, using unique constraints is an effective way to prevent duplicates:
ALTER TABLE table_name
ADD CONSTRAINT unique_constraint_name UNIQUE (column1, column2);
7.4. Handling Duplicates in Database Migrations
When migrating data between databases, you may encounter duplicates. Here’s a Python script using SQLAlchemy to handle this scenario:
from sqlalchemy import create_engine, MetaData, Table
from sqlalchemy.orm import sessionmaker
def migrate_data_without_duplicates(source_db_url, target_db_url, table_name):
source_engine = create_engine(source_db_url)
target_engine = create_engine(target_db_url)
metadata = MetaData()
source_table = Table(table_name, metadata, autoload_with=source_engine)
target_table = Table(table_name, metadata, autoload_with=target_engine)
Source_session = sessionmaker(bind=source_engine)
Target_session = sessionmaker(bind=target_engine)
with Source_session() as source_session, Target_session() as target_session:
# Get all data from source
source_data = source_session.query(source_table).all()
# Get existing data from target
existing_data = {tuple(row) for row in target_session.query(target_table).all()}
# Insert only new data
new_data = [row for row in source_data if tuple(row) not in existing_data]
if new_data:
target_session.bulk_insert_mappings(target_table, new_data)
target_session.commit()
print(f"Migrated {len(new_data)} new records to {table_name}")
# Example usage
source_db_url = "postgresql://user:password@localhost:5432/source_db"
target_db_url = "postgresql://user:password@localhost:5432/target_db"
migrate_data_without_duplicates(source_db_url, target_db_url, "users")
8. Real-World Examples and Use Cases
Let’s explore some real-world scenarios where handling duplicate values is crucial:
8.1. Customer Data Management
In customer relationship management (CRM) systems, preventing duplicate customer records is essential for maintaining data integrity and providing a unified customer view.
def merge_customer_records(existing_record, new_record):
merged_record = existing_record.copy()
for key, value in new_record.items():
if value and (key not in existing_record or not existing_record[key]):
merged_record[key] = value
return merged_record
def update_customer_data(customers, new_customer):
for i, customer in enumerate(customers):
if customer['email'] == new_customer['email']:
customers[i] = merge_customer_records(customer, new_customer)
return
customers.append(new_customer)
# Example usage
customers = [
{'id': 1, 'name': 'John Doe', 'email': 'john@example.com', 'phone': '123-456-7890'},
{'id': 2, 'name': 'Jane Smith', 'email': 'jane@example.com', 'phone': ''}
]
new_customer = {'name': 'John D.', 'email': 'john@example.com', 'phone': '987-654-3210'}
update_customer_data(customers, new_customer)
print(customers)
# Output: [
# {'id': 1, 'name': 'John Doe', 'email': 'john@example.com', 'phone': '987-654-3210'},
# {'id': 2, 'name': 'Jane Smith', 'email': 'jane@example.com', 'phone': ''}
# ]
8.2. Data Deduplication in File Systems
Data deduplication is a technique used in file systems and backup solutions to eliminate duplicate copies of repeating data. Here’s a simple example of how this might work:
import hashlib
class DedupFileSystem:
def __init__(self):
self.files = {}
self.chunks = {}
def add_file(self, filename, content):
file_chunks = []
for i in range(0, len(content), 1024): # 1KB chunks
chunk = content[i:i+1024]
chunk_hash = hashlib.md5(chunk.encode()).hexdigest()
if chunk_hash not in self.chunks:
self.chunks[chunk_hash] = chunk
file_chunks.append(chunk_hash)
self.files[filename] = file_chunks
def get_file(self, filename):
if filename not in self.files:
return None
return ''.join(self.chunks[chunk_hash] for chunk_hash in self.files[filename])
def get_total_storage(self):
return sum(len(chunk) for chunk in self.chunks.values())
# Example usage
fs = DedupFileSystem()
fs.add_file("file1.txt", "Hello, world! " * 1000)
fs.add_file("file2.txt", "Hello, world! " * 500 + "Goodbye, world! " * 500)
print(f"Total storage used: {fs.get_total_storage()} bytes")
print(f"Content of file1.txt: {fs.get_file('file1.txt')[:20]}...")
print(f"Content of file2.txt: {fs.get_file('file2.txt')[:20]}...")
8.3. Duplicate Detection in Plagiarism Checkers
Plagiarism detection tools need to efficiently identify duplicate or similar text across large document sets. Here’s a simplified example using the Jaccard similarity:
def tokenize(text):
return set(text.lower().split())
def jaccard_similarity(set1, set2):
intersection = len(set1.intersection(set2))
union = len(set1.union(set2))
return intersection / union if union != 0 else 0
def check_plagiarism(documents, threshold=0.8):
suspicious_pairs = []
doc_tokens = [tokenize(doc) for doc in documents]
for i in range(len(documents)):
for j in range(i+1, len(documents)):
similarity = jaccard_similarity(doc_tokens[i], doc_tokens[j])
if similarity >= threshold:
suspicious_pairs.append((i, j, similarity))
return suspicious_pairs
# Example usage
documents = [
"The quick brown fox jumps over the lazy dog",
"A quick brown fox leaps over a lazy dog",
"An entirely different sentence with no similarity",
"The fast brown fox jumps over the sleepy dog"
]
suspicious = check_plagiarism(documents, threshold=0.7)
for i, j, sim in suspicious:
print(f"Documents {i} and {j} are suspiciously similar (similarity: {sim:.2f})")
9. Best Practices and Performance Considerations
When dealing with duplicate values, keep these best practices and performance considerations in mind:
- Choose the right data structure: Use sets for fast lookup and uniqueness checks, sorted lists for efficient searching, and hash tables for quick counting and access.
- Consider memory usage: For very large datasets, consider using streaming algorithms or disk-based solutions to avoid loading everything into memory.
- Optimize database queries: Use indexes on columns prone to duplicates and write efficient queries to handle duplicates.
- Use appropriate algorithms: Choose algorithms based on your data size and structure. For example, use Bloom filters for approximate membership queries on large datasets.
- Implement early detection: Catch duplicates as early as possible in your data pipeline to minimize downstream effects.
- Regular maintenance: Periodically clean and deduplicate your data to maintain data quality over time.
- Benchmark and profile: Measure the performance of your duplicate handling methods and optimize as needed.
- Consider parallelization: For large-scale deduplication tasks, consider using parallel processing techniques or distributed computing frameworks.
10. Conclusion
Handling duplicate values efficiently is a crucial skill for any programmer or data scientist. By understanding the various techniques and best practices outlined in this guide, you’ll be well-equipped to tackle duplicate-related challenges in your projects.
Remember that the best approach for handling duplicates often depends on your specific use case, data structure, and performance requirements. Always consider the trade-offs between time complexity, space complexity, and accuracy when choosing a method to handle duplicates.
As you continue to work with data and build applications, you’ll encounter many situations where efficient duplicate handling is essential. By mastering these techniques, you’ll be able to write more robust, efficient, and maintainable code.
Keep practicing and experimenting with different approaches to handling duplicates, and don’t hesitate to explore more advanced techniques as you encounter more complex scenarios in your programming journey.