How to Handle Command-Line Arguments in Your Programs
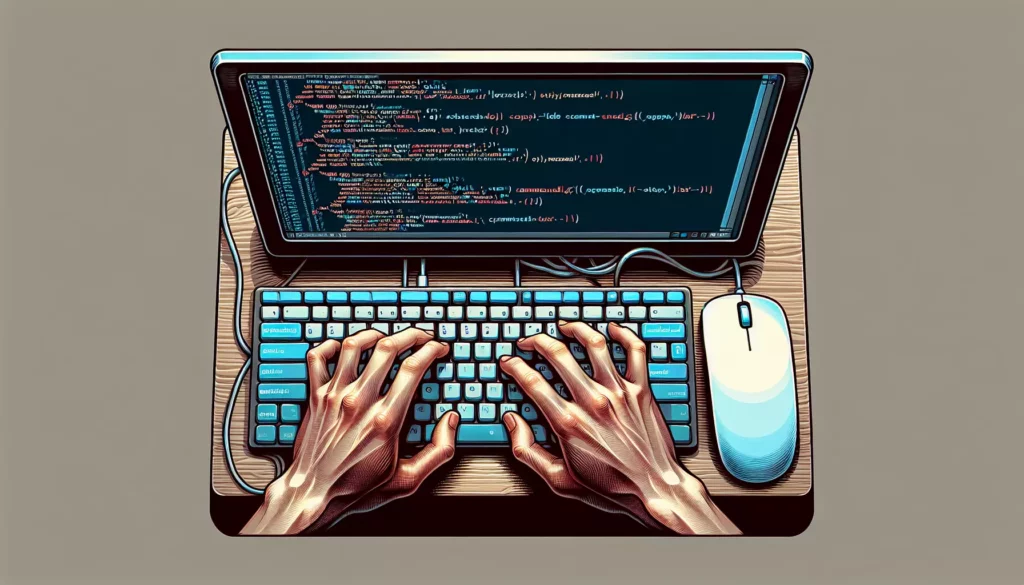
Command-line arguments are a powerful feature that allows users to pass information to a program when it starts. They provide a way to customize the behavior of a program without modifying its source code. In this comprehensive guide, we’ll explore various methods to handle command-line arguments in different programming languages, discuss best practices, and provide practical examples to help you master this essential programming skill.
Table of Contents
- Introduction to Command-Line Arguments
- Handling Command-Line Arguments in Python
- Handling Command-Line Arguments in Java
- Handling Command-Line Arguments in C++
- Handling Command-Line Arguments in JavaScript (Node.js)
- Best Practices for Handling Command-Line Arguments
- Advanced Techniques and Libraries
- Real-World Examples and Use Cases
- Conclusion
1. Introduction to Command-Line Arguments
Command-line arguments are parameters supplied to a program when it is invoked from the command line interface. They allow users to pass data or configure the program’s behavior without modifying the source code. This feature is particularly useful for creating flexible and reusable scripts or applications.
Some common use cases for command-line arguments include:
- Specifying input and output file paths
- Setting configuration options
- Enabling or disabling features
- Providing authentication credentials
- Controlling the verbosity of output
Now, let’s explore how to handle command-line arguments in various popular programming languages.
2. Handling Command-Line Arguments in Python
Python provides multiple ways to handle command-line arguments. We’ll discuss three common methods: using the sys.argv
list, the argparse
module, and the click
library.
2.1. Using sys.argv
The simplest way to access command-line arguments in Python is through the sys.argv
list. This list contains the script name as the first element, followed by any additional arguments passed to the script.
import sys
def main():
print(f"Script name: {sys.argv[0]}")
print(f"Number of arguments: {len(sys.argv) - 1}")
print(f"Arguments: {sys.argv[1:]}")
if __name__ == "__main__":
main()
To run this script with arguments, you would use:
python script.py arg1 arg2 arg3
2.2. Using the argparse module
For more complex argument parsing, Python’s built-in argparse
module provides a powerful and flexible solution.
import argparse
def main():
parser = argparse.ArgumentParser(description="A simple argument parser example")
parser.add_argument("-n", "--name", help="Your name", required=True)
parser.add_argument("-a", "--age", help="Your age", type=int)
parser.add_argument("-v", "--verbose", action="store_true", help="Increase output verbosity")
args = parser.parse_args()
if args.verbose:
print(f"Hello, {args.name}! You are {args.age} years old.")
else:
print(f"Hello, {args.name}!")
if __name__ == "__main__":
main()
You can run this script with:
python script.py --name John --age 30 --verbose
2.3. Using the click library
The click
library is a third-party package that provides a simple and intuitive way to create command-line interfaces in Python.
import click
@click.command()
@click.option("--name", prompt="Your name", help="Your name")
@click.option("--age", prompt="Your age", type=int, help="Your age")
@click.option("--verbose", is_flag=True, help="Increase output verbosity")
def greet(name, age, verbose):
if verbose:
click.echo(f"Hello, {name}! You are {age} years old.")
else:
click.echo(f"Hello, {name}!")
if __name__ == "__main__":
greet()
To use this script, you would run:
python script.py --name John --age 30 --verbose
3. Handling Command-Line Arguments in Java
In Java, command-line arguments are passed to the main
method as an array of strings.
3.1. Basic argument handling
public class ArgumentHandler {
public static void main(String[] args) {
System.out.println("Number of arguments: " + args.length);
for (int i = 0; i < args.length; i++) {
System.out.println("Argument " + i + ": " + args[i]);
}
}
}
You can run this Java program with:
java ArgumentHandler arg1 arg2 arg3
3.2. Using Apache Commons CLI
For more advanced argument parsing in Java, you can use the Apache Commons CLI library. First, add the library to your project, then use it as follows:
import org.apache.commons.cli.*;
public class AdvancedArgumentHandler {
public static void main(String[] args) {
Options options = new Options();
Option name = new Option("n", "name", true, "Your name");
name.setRequired(true);
options.addOption(name);
Option age = new Option("a", "age", true, "Your age");
options.addOption(age);
Option verbose = new Option("v", "verbose", false, "Increase output verbosity");
options.addOption(verbose);
CommandLineParser parser = new DefaultParser();
HelpFormatter formatter = new HelpFormatter();
CommandLine cmd = null;
try {
cmd = parser.parse(options, args);
} catch (ParseException e) {
System.out.println(e.getMessage());
formatter.printHelp("AdvancedArgumentHandler", options);
System.exit(1);
}
String nameValue = cmd.getOptionValue("name");
String ageValue = cmd.getOptionValue("age");
boolean verboseMode = cmd.hasOption("verbose");
if (verboseMode) {
System.out.println("Hello, " + nameValue + "! You are " + ageValue + " years old.");
} else {
System.out.println("Hello, " + nameValue + "!");
}
}
}
You can run this Java program with:
java AdvancedArgumentHandler -n John -a 30 -v
4. Handling Command-Line Arguments in C++
In C++, command-line arguments are passed to the main
function as parameters.
4.1. Basic argument handling
#include <iostream>
int main(int argc, char* argv[]) {
std::cout << "Number of arguments: " << argc - 1 << std::endl;
for (int i = 1; i < argc; i++) {
std::cout << "Argument " << i << ": " << argv[i] << std::endl;
}
return 0;
}
Compile and run this C++ program with:
g++ argument_handler.cpp -o argument_handler
./argument_handler arg1 arg2 arg3
4.2. Using the Boost.Program_options library
For more advanced argument parsing in C++, you can use the Boost.Program_options library. First, install the Boost libraries, then use them as follows:
#include <iostream>
#include <boost/program_options.hpp>
namespace po = boost::program_options;
int main(int argc, char* argv[]) {
std::string name;
int age;
bool verbose;
po::options_description desc("Allowed options");
desc.add_options()
("help", "produce help message")
("name,n", po::value<std::string>(&name)->required(), "Your name")
("age,a", po::value<int>(&age), "Your age")
("verbose,v", po::bool_switch(&verbose), "Increase output verbosity")
;
po::variables_map vm;
try {
po::store(po::parse_command_line(argc, argv, desc), vm);
if (vm.count("help")) {
std::cout << desc << std::endl;
return 0;
}
po::notify(vm);
} catch (po::error& e) {
std::cerr << "Error: " << e.what() << std::endl;
std::cout << desc << std::endl;
return 1;
}
if (verbose) {
std::cout << "Hello, " << name << "! You are " << age << " years old." << std::endl;
} else {
std::cout << "Hello, " << name << "!" << std::endl;
}
return 0;
}
Compile and run this C++ program with:
g++ advanced_argument_handler.cpp -o advanced_argument_handler -lboost_program_options
./advanced_argument_handler --name John --age 30 --verbose
5. Handling Command-Line Arguments in JavaScript (Node.js)
In Node.js, you can access command-line arguments through the process.argv
array.
5.1. Basic argument handling
const args = process.argv.slice(2);
console.log(`Number of arguments: ${args.length}`);
args.forEach((arg, index) => {
console.log(`Argument ${index + 1}: ${arg}`);
});
Run this Node.js script with:
node script.js arg1 arg2 arg3
5.2. Using the yargs library
For more advanced argument parsing in Node.js, you can use the yargs
library. First, install it using npm:
npm install yargs
Then, use it in your script:
const yargs = require('yargs');
const argv = yargs
.option('name', {
alias: 'n',
description: 'Your name',
type: 'string',
demandOption: true
})
.option('age', {
alias: 'a',
description: 'Your age',
type: 'number'
})
.option('verbose', {
alias: 'v',
description: 'Increase output verbosity',
type: 'boolean',
default: false
})
.help()
.alias('help', 'h')
.argv;
if (argv.verbose) {
console.log(`Hello, ${argv.name}! You are ${argv.age} years old.`);
} else {
console.log(`Hello, ${argv.name}!`);
}
Run this Node.js script with:
node script.js --name John --age 30 --verbose
6. Best Practices for Handling Command-Line Arguments
When working with command-line arguments, consider the following best practices:
- Provide clear and concise help messages: Always include a help option that explains how to use your program and what each argument does.
- Use short and long options: Offer both short (e.g.,
-n
) and long (e.g.,--name
) options for better usability. - Implement input validation: Check that required arguments are provided and that values are within expected ranges or formats.
- Use appropriate data types: Ensure that arguments are parsed into the correct data types (e.g., integers, floats, booleans).
- Group related options: Organize related options into logical groups for better readability and understanding.
- Provide default values: When appropriate, set default values for optional arguments.
- Handle errors gracefully: Provide informative error messages when invalid arguments are supplied.
- Follow platform conventions: Adhere to common command-line conventions for your target platform (e.g., POSIX or GNU style).
- Document your command-line interface: Include documentation on how to use your program’s command-line interface in your project’s README or user manual.
7. Advanced Techniques and Libraries
As you become more proficient in handling command-line arguments, you may want to explore more advanced techniques and libraries:
7.1. Subcommands
Many modern command-line tools use subcommands to group related functionality. For example, Git uses subcommands like git commit
, git push
, and git pull
. Libraries like argparse
in Python and yargs
in Node.js support subcommands.
7.2. Configuration files
For complex applications, consider using configuration files in addition to command-line arguments. This allows users to set default options without specifying them every time they run the program.
7.3. Environment variables
Environment variables can be used alongside command-line arguments to configure your application. This is particularly useful for sensitive information like API keys or passwords.
7.4. Autocomplete
Implement command-line argument autocompletion to improve the user experience. Many argument parsing libraries provide built-in support for this feature.
7.5. Internationalization
For applications with a global audience, consider internationalizing your command-line interface, including help messages and error outputs.
8. Real-World Examples and Use Cases
Let’s explore some real-world examples of how command-line arguments are used in popular tools and applications:
8.1. ffmpeg (Media Processing)
ffmpeg is a powerful multimedia framework that uses command-line arguments extensively. Here’s an example of converting a video file:
ffmpeg -i input.mp4 -c:v libx264 -preset slow -crf 22 -c:a copy output.mp4
This command uses various arguments to specify input and output files, video codec, encoding preset, and quality settings.
8.2. npm (Package Manager)
npm, the Node.js package manager, uses command-line arguments for various operations:
npm install --save-dev jest
This command installs the Jest testing framework and saves it as a development dependency in the project.
8.3. git (Version Control)
Git uses a combination of subcommands and arguments:
git commit -m "Fix bug in login functionality"
This command creates a new commit with the specified message.
8.4. Django’s manage.py (Web Framework)
Django’s manage.py
script uses command-line arguments to perform various project management tasks:
python manage.py runserver 0.0.0.0:8000
This command starts the Django development server, binding it to all available network interfaces on port 8000.
8.5. Custom Data Processing Script
Here’s an example of a custom Python script that processes data files based on command-line arguments:
import argparse
import pandas as pd
def process_data(input_file, output_file, operation):
df = pd.read_csv(input_file)
if operation == 'sum':
result = df.sum()
elif operation == 'mean':
result = df.mean()
else:
raise ValueError(f"Unknown operation: {operation}")
result.to_csv(output_file)
def main():
parser = argparse.ArgumentParser(description="Process CSV data files")
parser.add_argument("-i", "--input", required=True, help="Input CSV file")
parser.add_argument("-o", "--output", required=True, help="Output CSV file")
parser.add_argument("-op", "--operation", choices=['sum', 'mean'], required=True, help="Operation to perform")
args = parser.parse_args()
process_data(args.input, args.output, args.operation)
print(f"Data processed. Results saved to {args.output}")
if __name__ == "__main__":
main()
This script can be run with:
python data_processor.py --input data.csv --output results.csv --operation sum
9. Conclusion
Mastering the art of handling command-line arguments is an essential skill for any programmer. It allows you to create flexible, powerful, and user-friendly applications that can be easily configured and automated. By understanding the basics of argument parsing, following best practices, and leveraging advanced techniques, you can significantly enhance the functionality and usability of your programs.
As you continue to develop your programming skills, remember that effective command-line argument handling is just one aspect of creating robust and efficient software. Keep practicing, exploring new libraries and techniques, and applying these concepts to your projects. With time and experience, you’ll become proficient in creating sophisticated command-line interfaces that rival those of professional-grade software tools.
Whether you’re building simple scripts or complex applications, the ability to handle command-line arguments effectively will serve you well throughout your programming career. It’s a fundamental skill that transcends specific languages or frameworks and is applicable across a wide range of programming domains.
Continue to experiment with different argument parsing libraries, explore real-world examples, and challenge yourself to create increasingly complex and user-friendly command-line interfaces. As you do so, you’ll not only improve your coding skills but also gain a deeper understanding of how to design intuitive and powerful software tools.