How to Handle Circular Dependencies: A Comprehensive Guide
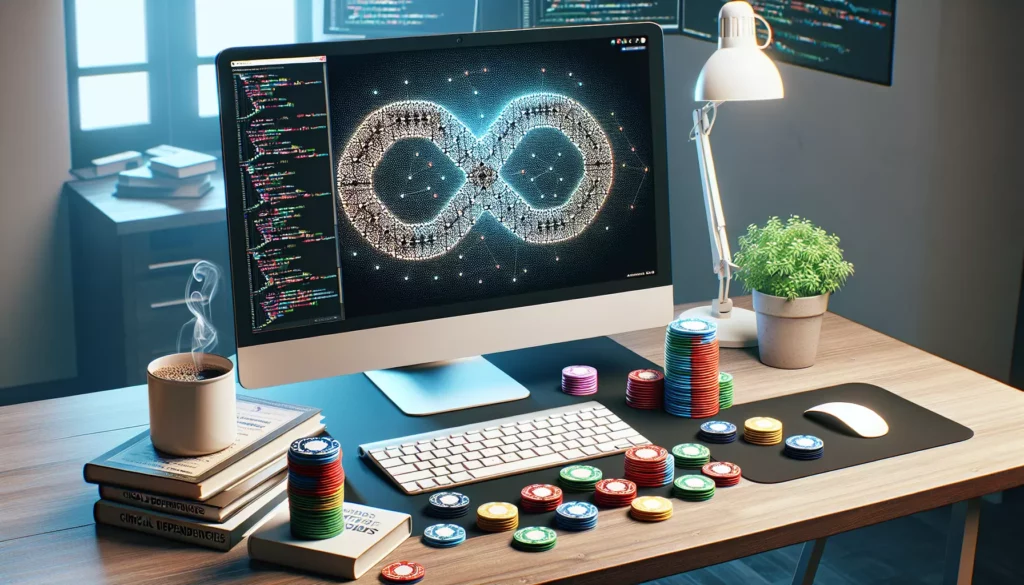
Circular dependencies are a common challenge in software development that can lead to complex issues if not properly managed. In this comprehensive guide, we’ll explore what circular dependencies are, why they’re problematic, and most importantly, how to handle them effectively. Whether you’re a beginner programmer or preparing for technical interviews at major tech companies, understanding circular dependencies is crucial for writing clean, maintainable code.
What Are Circular Dependencies?
Before we dive into solutions, let’s clearly define what we mean by circular dependencies:
A circular dependency occurs when two or more modules or components in a software system depend on each other, either directly or indirectly. This creates a cycle in the dependency graph, where Module A depends on Module B, which in turn depends on Module A.
For example:
// Module A
import B from './moduleB';
function doSomethingA() {
B.doSomethingB();
}
// Module B
import A from './moduleA';
function doSomethingB() {
A.doSomethingA();
}
In this simple example, Module A depends on Module B, and Module B depends on Module A, creating a circular dependency.
Why Are Circular Dependencies Problematic?
Circular dependencies can cause several issues in your codebase:
- Initialization Problems: In languages that use static initialization, circular dependencies can lead to undefined behavior or runtime errors.
- Tight Coupling: Circular dependencies often indicate that modules are too tightly coupled, making the code harder to maintain and test.
- Reduced Reusability: Modules involved in circular dependencies are difficult to reuse independently.
- Compilation Issues: In compiled languages, circular dependencies can cause compilation errors or unexpected behavior.
- Difficulty in Understanding: Circular dependencies make the codebase harder to understand and reason about.
- Performance Impact: In some cases, circular dependencies can lead to performance issues, especially in interpreted languages.
Strategies for Handling Circular Dependencies
Now that we understand the problems circular dependencies can cause, let’s explore strategies to handle them:
1. Redesign the Architecture
The most effective way to handle circular dependencies is to prevent them in the first place through proper design. This often involves rethinking your architecture:
- Identify Common Functionality: Look for shared functionality that can be extracted into a separate module.
- Use Dependency Inversion: Implement interfaces or abstract classes that both modules can depend on, rather than depending directly on each other.
- Apply the Single Responsibility Principle: Ensure each module has a single, well-defined responsibility to reduce the likelihood of circular dependencies.
Example of redesigning:
// CommonInterface.js
export interface CommonInterface {
doSomething(): void;
}
// ModuleA.js
import { CommonInterface } from './CommonInterface';
export class ModuleA implements CommonInterface {
doSomething() {
console.log('ModuleA doing something');
}
}
// ModuleB.js
import { CommonInterface } from './CommonInterface';
export class ModuleB implements CommonInterface {
doSomething() {
console.log('ModuleB doing something');
}
}
// Main.js
import { ModuleA } from './ModuleA';
import { ModuleB } from './ModuleB';
const a = new ModuleA();
const b = new ModuleB();
a.doSomething();
b.doSomething();
In this redesigned version, both ModuleA and ModuleB implement a common interface, eliminating the need for direct dependencies between them.
2. Use Dependency Injection
Dependency Injection (DI) is a design pattern that can help manage dependencies more effectively:
- Instead of modules creating their dependencies directly, dependencies are “injected” from the outside.
- This approach makes modules more loosely coupled and easier to test.
Example of using Dependency Injection:
// ModuleA.js
export class ModuleA {
constructor(moduleB) {
this.moduleB = moduleB;
}
doSomethingA() {
console.log('ModuleA doing something');
this.moduleB.doSomethingB();
}
}
// ModuleB.js
export class ModuleB {
constructor(moduleA) {
this.moduleA = moduleA;
}
doSomethingB() {
console.log('ModuleB doing something');
this.moduleA.doSomethingA();
}
}
// Main.js
const moduleA = new ModuleA(null);
const moduleB = new ModuleB(moduleA);
moduleA.moduleB = moduleB;
moduleA.doSomethingA();
In this example, dependencies are injected through constructors, allowing for more flexible and testable code.
3. Use Lazy Loading
Lazy loading can be an effective way to break circular dependencies, especially in dynamic languages:
- Instead of loading dependencies immediately, load them when they’re actually needed.
- This can help break cycles in the dependency graph.
Example of lazy loading in JavaScript:
// ModuleA.js
let ModuleB;
export const ModuleA = {
doSomethingA() {
console.log('ModuleA doing something');
if (!ModuleB) {
ModuleB = require('./ModuleB');
}
ModuleB.doSomethingB();
}
};
// ModuleB.js
let ModuleA;
export const ModuleB = {
doSomethingB() {
console.log('ModuleB doing something');
if (!ModuleA) {
ModuleA = require('./ModuleA');
}
ModuleA.doSomethingA();
}
};
Here, modules are loaded only when their functions are called, breaking the immediate circular dependency.
4. Use Mediator Pattern
The Mediator pattern can be useful for managing complex dependencies:
- Introduce a mediator object that coordinates communication between modules.
- Modules communicate through the mediator instead of directly with each other.
Example of using the Mediator pattern:
// Mediator.js
export class Mediator {
constructor() {
this.moduleA = null;
this.moduleB = null;
}
setModuleA(moduleA) {
this.moduleA = moduleA;
}
setModuleB(moduleB) {
this.moduleB = moduleB;
}
notifyA() {
this.moduleA.doSomethingA();
}
notifyB() {
this.moduleB.doSomethingB();
}
}
// ModuleA.js
export class ModuleA {
constructor(mediator) {
this.mediator = mediator;
}
doSomethingA() {
console.log('ModuleA doing something');
this.mediator.notifyB();
}
}
// ModuleB.js
export class ModuleB {
constructor(mediator) {
this.mediator = mediator;
}
doSomethingB() {
console.log('ModuleB doing something');
this.mediator.notifyA();
}
}
// Main.js
const mediator = new Mediator();
const moduleA = new ModuleA(mediator);
const moduleB = new ModuleB(mediator);
mediator.setModuleA(moduleA);
mediator.setModuleB(moduleB);
moduleA.doSomethingA();
The Mediator pattern decouples ModuleA and ModuleB, allowing them to communicate indirectly.
5. Use Event-Driven Architecture
An event-driven approach can help decouple modules:
- Modules emit events instead of calling each other directly.
- Other modules can subscribe to these events and react accordingly.
Example of event-driven architecture:
// EventEmitter.js
export class EventEmitter {
constructor() {
this.events = {};
}
on(event, listener) {
if (!this.events[event]) {
this.events[event] = [];
}
this.events[event].push(listener);
}
emit(event, data) {
const listeners = this.events[event];
if (listeners) {
listeners.forEach(listener => listener(data));
}
}
}
// ModuleA.js
export class ModuleA {
constructor(eventEmitter) {
this.eventEmitter = eventEmitter;
}
doSomethingA() {
console.log('ModuleA doing something');
this.eventEmitter.emit('aDidSomething');
}
}
// ModuleB.js
export class ModuleB {
constructor(eventEmitter) {
this.eventEmitter = eventEmitter;
this.eventEmitter.on('aDidSomething', () => this.doSomethingB());
}
doSomethingB() {
console.log('ModuleB doing something');
}
}
// Main.js
const eventEmitter = new EventEmitter();
const moduleA = new ModuleA(eventEmitter);
const moduleB = new ModuleB(eventEmitter);
moduleA.doSomethingA();
In this event-driven approach, ModuleA and ModuleB are decoupled and communicate through events.
Best Practices for Preventing Circular Dependencies
While the strategies above can help manage existing circular dependencies, it’s best to prevent them from occurring in the first place. Here are some best practices:
1. Follow the Single Responsibility Principle
Ensure each module has a single, well-defined responsibility. This reduces the likelihood of circular dependencies by limiting the reasons a module might need to depend on others.
2. Use Dependency Inversion Principle
Depend on abstractions rather than concrete implementations. This allows for more flexible and loosely coupled designs.
3. Implement Layered Architecture
Organize your codebase into layers (e.g., presentation, business logic, data access) and enforce a unidirectional dependency flow between these layers.
4. Regularly Analyze Dependencies
Use tools to visualize and analyze your project’s dependency graph. Many IDEs and build tools offer features to help identify circular dependencies.
5. Write Unit Tests
Comprehensive unit tests can help identify dependency issues early and ensure that modules can function independently.
6. Use Composition Over Inheritance
Favor composition over inheritance when designing your classes. This can lead to more flexible designs and reduce the likelihood of circular dependencies.
Tools for Detecting Circular Dependencies
Several tools can help you identify circular dependencies in your codebase:
- Madge: A JavaScript tool for generating a visual dependency graph of your project.
- Deptrac: A static code analysis tool for PHP that helps to enforce rules for dependencies between software layers.
- JDepend: Traverses Java class file directories and generates design quality metrics for each Java package.
- ESLint: Can be configured with plugins to detect circular dependencies in JavaScript projects.
- Go’s Dependency Tool: The Go programming language includes built-in tools for detecting import cycles.
Conclusion
Handling circular dependencies is a crucial skill for any software developer, especially those preparing for technical interviews at major tech companies. By understanding the causes and consequences of circular dependencies, and by applying the strategies and best practices outlined in this guide, you can write cleaner, more maintainable, and more efficient code.
Remember, the best approach is to prevent circular dependencies through thoughtful design and architecture. However, when faced with existing circular dependencies, techniques like dependency injection, lazy loading, and using design patterns like Mediator or event-driven architecture can help resolve the issue.
As you continue to develop your programming skills, keep these concepts in mind. They’ll not only help you write better code but also prepare you for the types of architectural challenges you might face in technical interviews and real-world software development projects.
Happy coding, and may your dependencies always flow in one direction!