How to Handle Array Rotation Problems: A Comprehensive Guide
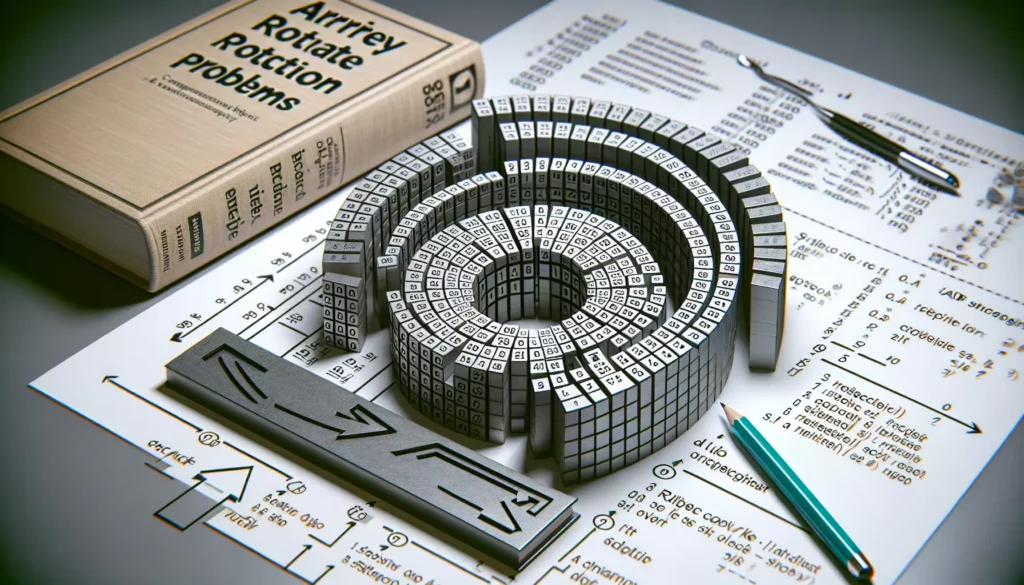
Array rotation is a fundamental concept in computer programming and a common topic in coding interviews, especially for positions at major tech companies. Understanding how to efficiently rotate arrays is crucial for developing strong algorithmic thinking and problem-solving skills. In this comprehensive guide, we’ll explore various approaches to array rotation, their time and space complexities, and provide practical examples to help you master this important concept.
Table of Contents
- Introduction to Array Rotation
- Basic Approach: Using a Temporary Array
- The Juggling Algorithm
- The Reversal Algorithm
- Block Swap Algorithm
- Optimization Techniques
- Interview Tips and Tricks
- Practice Problems
- Conclusion
1. Introduction to Array Rotation
Array rotation is the process of shifting elements in an array by a certain number of positions, either to the left or right. It’s a common operation in various programming scenarios and is often used as a building block for more complex algorithms.
There are two types of array rotations:
- Left Rotation: Elements are shifted to the left, and the elements that “fall off” the left end are moved to the right end.
- Right Rotation: Elements are shifted to the right, and the elements that “fall off” the right end are moved to the left end.
For example, let’s consider an array [1, 2, 3, 4, 5] and rotate it 2 positions to the left:
- Original array: [1, 2, 3, 4, 5]
- After rotation: [3, 4, 5, 1, 2]
Now that we understand the basics, let’s dive into different approaches to solve array rotation problems.
2. Basic Approach: Using a Temporary Array
The most straightforward approach to array rotation is using a temporary array to store the rotated elements. Here’s how it works:
- Create a temporary array of the same size as the original array.
- Copy the elements to the temporary array in their rotated positions.
- Copy the elements back to the original array.
Here’s a Python implementation of this approach:
def rotate_array(arr, k):
n = len(arr)
k = k % n # Handle cases where k > n
temp = [0] * n
# Copy elements to temp array in rotated order
for i in range(n):
temp[(i + k) % n] = arr[i]
# Copy elements back to original array
for i in range(n):
arr[i] = temp[i]
return arr
# Example usage
arr = [1, 2, 3, 4, 5]
k = 2
rotated_arr = rotate_array(arr, k)
print(f"Original array: {arr}")
print(f"Rotated array: {rotated_arr}")
Time Complexity: O(n), where n is the length of the array
Space Complexity: O(n) for the temporary array
While this approach is simple and easy to understand, it requires additional space proportional to the size of the input array. In interviews or real-world scenarios where memory usage is a concern, you might need to consider more space-efficient algorithms.
3. The Juggling Algorithm
The Juggling Algorithm is an in-place rotation algorithm that doesn’t require extra space. It works by dividing the array into sets and moving elements within these sets. Here’s how it works:
- Calculate the GCD (Greatest Common Divisor) of the array length and the rotation count.
- Divide the array into GCD sets.
- For each set, perform a cyclic movement of elements.
Here’s a Python implementation of the Juggling Algorithm:
def gcd(a, b):
while b:
a, b = b, a % b
return a
def rotate_array_juggling(arr, k):
n = len(arr)
k = k % n
# If rotation is 0 or equal to array length, return original array
if k == 0 or k == n:
return arr
sets = gcd(n, k)
for i in range(sets):
temp = arr[i]
j = i
while True:
d = (j + k) % n
if d == i:
break
arr[j] = arr[d]
j = d
arr[j] = temp
return arr
# Example usage
arr = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12]
k = 3
rotated_arr = rotate_array_juggling(arr, k)
print(f"Original array: {arr}")
print(f"Rotated array: {rotated_arr}")
Time Complexity: O(n), where n is the length of the array
Space Complexity: O(1), as it performs the rotation in-place
The Juggling Algorithm is more space-efficient than the basic approach, making it suitable for scenarios where memory usage is a concern. However, it can be more challenging to implement and understand, especially for beginners.
4. The Reversal Algorithm
The Reversal Algorithm is another in-place rotation method that’s both efficient and relatively easy to understand. It works by reversing parts of the array in a specific order. Here’s the step-by-step process for left rotation:
- Reverse the first k elements of the array.
- Reverse the remaining n-k elements.
- Reverse the entire array.
Here’s a Python implementation of the Reversal Algorithm:
def reverse_array(arr, start, end):
while start < end:
arr[start], arr[end] = arr[end], arr[start]
start += 1
end -= 1
def rotate_array_reversal(arr, k):
n = len(arr)
k = k % n
# If rotation is 0 or equal to array length, return original array
if k == 0 or k == n:
return arr
# For left rotation
reverse_array(arr, 0, k - 1)
reverse_array(arr, k, n - 1)
reverse_array(arr, 0, n - 1)
return arr
# Example usage
arr = [1, 2, 3, 4, 5, 6, 7]
k = 3
rotated_arr = rotate_array_reversal(arr, k)
print(f"Original array: {arr}")
print(f"Rotated array: {rotated_arr}")
Time Complexity: O(n), where n is the length of the array
Space Complexity: O(1), as it performs the rotation in-place
The Reversal Algorithm is both time and space efficient, making it a popular choice for array rotation problems. It’s also relatively easy to understand and implement, which can be advantageous in interview situations.
5. Block Swap Algorithm
The Block Swap Algorithm is another efficient in-place rotation method. It works by swapping blocks of elements until the entire array is rotated. Here’s how it works:
- Divide the array into two parts: A (first k elements) and B (remaining n-k elements).
- If A and B are of equal size, swap them and we’re done.
- If A is smaller, swap A with the first k elements of B, and recursively work on the right side of B.
- If B is smaller, swap B with the last n-k elements of A, and recursively work on the left side of A.
Here’s a Python implementation of the Block Swap Algorithm:
def swap(arr, fi, si, d):
for i in range(d):
arr[fi + i], arr[si + i] = arr[si + i], arr[fi + i]
def rotate_array_block_swap(arr, d):
n = len(arr)
d = d % n
i, j = d, n - d
while i != j:
if i < j:
swap(arr, d - i, d + j - i, i)
j -= i
else:
swap(arr, d - i, d, j)
i -= j
swap(arr, d - i, d, i)
return arr
# Example usage
arr = [1, 2, 3, 4, 5, 6, 7]
d = 2
rotated_arr = rotate_array_block_swap(arr, d)
print(f"Original array: {arr}")
print(f"Rotated array: {rotated_arr}")
Time Complexity: O(n), where n is the length of the array
Space Complexity: O(1), as it performs the rotation in-place
The Block Swap Algorithm is efficient and performs the rotation in-place. However, it can be more complex to implement and understand compared to some other methods, which might make it less suitable for interview situations unless you’re very comfortable with the algorithm.
6. Optimization Techniques
When dealing with array rotation problems, there are several optimization techniques you can consider:
6.1 Modulo Arithmetic
Using modulo arithmetic can help handle cases where the rotation count is greater than the array length. Instead of rotating the array multiple times, you can use:
effective_rotation = k % n
Where k is the rotation count and n is the array length. This ensures that you’re always working with the minimum number of rotations needed.
6.2 Handling Edge Cases
Always consider and handle edge cases in your implementation:
- Empty array
- Array with one element
- Rotation count equal to 0 or array length
- Negative rotation count (which can be treated as a positive rotation in the opposite direction)
6.3 Using Built-in Functions
In some languages, you can use built-in functions or methods to perform array rotations more efficiently. For example, in Python:
def rotate_array_slice(arr, k):
n = len(arr)
k = k % n
return arr[k:] + arr[:k]
# Example usage
arr = [1, 2, 3, 4, 5]
k = 2
rotated_arr = rotate_array_slice(arr, k)
print(f"Original array: {arr}")
print(f"Rotated array: {rotated_arr}")
While this method creates a new array and might not be suitable for in-place rotation requirements, it’s concise and can be useful in certain scenarios.
7. Interview Tips and Tricks
When tackling array rotation problems in interviews, keep these tips in mind:
- Clarify the problem: Ask whether the rotation should be left or right, and if it needs to be done in-place.
- Consider constraints: Ask about the size of the array and the range of rotation counts to help choose the most appropriate algorithm.
- Start simple: Begin with the basic approach using a temporary array, then optimize if needed.
- Explain your thought process: Talk through your approach, discussing trade-offs between time and space complexity.
- Handle edge cases: Don’t forget to consider and handle special cases like empty arrays or rotations equal to the array length.
- Optimize incrementally: If your initial solution isn’t optimal, explain how you would improve it step by step.
- Practice implementation: Be prepared to code your solution efficiently and without major errors.
8. Practice Problems
To solidify your understanding of array rotation, try these practice problems:
- Rotate Image: You are given an n x n 2D matrix representing an image. Rotate the image by 90 degrees (clockwise).
- Search in Rotated Sorted Array: Given a sorted array that has been rotated at some unknown pivot, find a target element in the array.
- Rotation Count in Rotated Sorted array: Given a sorted and rotated array, find the number of times the array has been rotated.
- Pair with given sum in sorted and rotated array: Given a sorted and rotated array, find if there’s a pair with a given sum.
- Find maximum value of Sum( i*arr[i] ) with only rotations on given array allowed: Given an array, find the maximum value of sum of index multiplied by array element, with only rotations allowed.
These problems will help you apply the concepts of array rotation in different contexts and improve your problem-solving skills.
9. Conclusion
Array rotation is a fundamental concept in computer programming that plays a crucial role in many algorithms and data structure operations. By mastering various approaches to array rotation, from the basic temporary array method to more advanced algorithms like the Juggling, Reversal, and Block Swap methods, you’ll be well-equipped to handle a wide range of related problems in both interviews and real-world programming scenarios.
Remember that the key to success in tackling array rotation problems lies not just in knowing the algorithms, but in understanding when and why to apply each approach. Consider factors such as time and space complexity, the size of the input, and any specific constraints of the problem at hand.
As you continue to practice and refine your skills, you’ll develop a intuitive sense for which approach is best suited to each situation. This deep understanding of array manipulation will serve you well in your journey as a programmer, whether you’re preparing for technical interviews at major tech companies or working on complex software projects.
Keep practicing, stay curious, and don’t hesitate to explore new and creative solutions to array rotation problems. Happy coding!