How to Handle Array Partition Problems: A Comprehensive Guide
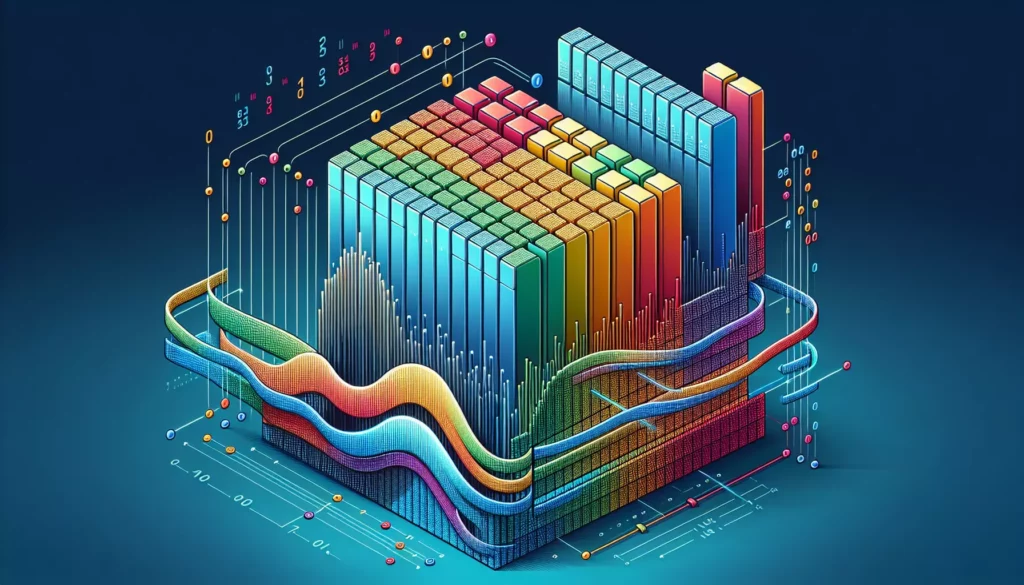
Array partition problems are a common challenge in coding interviews and algorithm-based questions. These problems typically involve dividing an array into two or more parts based on specific criteria. Understanding how to approach and solve these problems efficiently is crucial for aspiring programmers and those preparing for technical interviews, especially at major tech companies.
In this comprehensive guide, we’ll explore various array partition problems, discuss different approaches to solve them, and provide step-by-step explanations along with code examples. By the end of this article, you’ll have a solid understanding of how to tackle array partition problems and improve your problem-solving skills.
Table of Contents
- Understanding Array Partition Problems
- Common Types of Array Partition Problems
- Basic Approaches to Solving Array Partition Problems
- Advanced Techniques for Efficient Solutions
- Step-by-Step Examples
- Tips for Optimizing Your Solutions
- Practice Problems and Resources
- Conclusion
1. Understanding Array Partition Problems
Array partition problems involve dividing an array into two or more parts based on certain conditions or constraints. These problems test your ability to manipulate arrays, think logically, and implement efficient algorithms. The goal is usually to find a partition that satisfies specific criteria, such as minimizing the difference between the sums of the partitions or ensuring that each partition meets certain requirements.
Key aspects of array partition problems include:
- Identifying the partition criteria
- Determining the most efficient way to divide the array
- Handling edge cases and constraints
- Optimizing the solution for time and space complexity
2. Common Types of Array Partition Problems
There are several variations of array partition problems that you might encounter. Some common types include:
2.1. Equal Sum Partition
In this type of problem, you need to determine if it’s possible to divide the array into two parts with equal sums. For example, given the array [1, 5, 11, 5], you can partition it into [1, 5, 5] and [11], both having a sum of 11.
2.2. Minimum Difference Partition
Here, the goal is to partition the array into two subsets such that the difference between their sums is minimized. For instance, given [3, 1, 4, 2, 2, 1], you can partition it into [3, 2, 1] and [4, 2, 1], with a minimum difference of 1.
2.3. K-way Partition
This involves dividing the array into K non-empty parts, often with additional constraints such as minimizing the maximum sum of any partition or ensuring that each partition has at least a certain number of elements.
2.4. Partition Around a Pivot
In this problem, you need to rearrange the array elements around a pivot value, placing all elements smaller than the pivot to its left and all larger elements to its right. This is a key step in the QuickSort algorithm.
3. Basic Approaches to Solving Array Partition Problems
When tackling array partition problems, there are several fundamental approaches you can consider:
3.1. Brute Force
This approach involves generating all possible partitions and checking each one to see if it satisfies the given conditions. While straightforward, it’s often inefficient for large arrays due to its high time complexity.
3.2. Dynamic Programming
Dynamic programming can be very effective for many array partition problems, especially those involving sums or differences. It involves breaking down the problem into smaller subproblems and storing their solutions to avoid redundant calculations.
3.3. Two-Pointer Technique
This method uses two pointers that move through the array, often in opposite directions, to partition the array efficiently. It’s particularly useful for problems like the partition around a pivot.
3.4. Greedy Algorithms
In some cases, a greedy approach can provide an optimal solution. This involves making the locally optimal choice at each step with the hope of finding a global optimum.
4. Advanced Techniques for Efficient Solutions
To solve array partition problems more efficiently, consider these advanced techniques:
4.1. Bit Manipulation
For certain types of partition problems, especially those involving subsets, bit manipulation can lead to very efficient solutions. It allows you to represent and manipulate subsets using binary numbers.
4.2. Prefix Sums
Precomputing cumulative sums can significantly speed up calculations in problems involving sums of subarrays. This technique is particularly useful for problems where you need to frequently calculate sums of different partitions.
4.3. Binary Search
In some partition problems, especially those involving minimizing maximum values or maximizing minimum values, binary search can be applied to efficiently find the optimal partition point.
4.4. Hashing
Using hash tables can help in quickly identifying complementary partitions or checking for the existence of specific sums, which can be crucial in optimizing certain partition algorithms.
5. Step-by-Step Examples
Let’s walk through some examples to illustrate how to apply these techniques to solve array partition problems.
5.1. Equal Sum Partition
Problem: Given an array of positive integers, determine if it can be partitioned into two subsets with equal sum.
Approach: We can use dynamic programming to solve this efficiently.
def can_partition(nums):
total_sum = sum(nums)
# If the total sum is odd, it can't be partitioned into two equal subsets
if total_sum % 2 != 0:
return False
target_sum = total_sum // 2
n = len(nums)
# Create a DP table
dp = [[False] * (target_sum + 1) for _ in range(n + 1)]
# Initialize the first column as True (empty subset can always sum to 0)
for i in range(n + 1):
dp[i][0] = True
# Fill the DP table
for i in range(1, n + 1):
for j in range(1, target_sum + 1):
if j < nums[i-1]:
dp[i][j] = dp[i-1][j]
else:
dp[i][j] = dp[i-1][j] or dp[i-1][j-nums[i-1]]
return dp[n][target_sum]
# Example usage
nums = [1, 5, 11, 5]
print(can_partition(nums)) # Output: True
Explanation:
- We first check if the total sum is even. If it’s odd, it’s impossible to partition into two equal subsets.
- We create a DP table where dp[i][j] represents whether it’s possible to achieve a sum of j using the first i elements of the array.
- We fill this table iteratively, considering whether to include or exclude each element.
- The final answer is in dp[n][target_sum], which tells us if it’s possible to achieve half of the total sum using any subset of the array.
5.2. Partition Array for Maximum Sum
Problem: Given an array of integers arr and an integer k, partition the array into (contiguous) subarrays of length at most k. After partitioning, each subarray has their values changed to become the maximum value of that subarray. Return the largest sum of the given array after partitioning.
Approach: We can use dynamic programming to solve this problem efficiently.
def maxSumAfterPartitioning(arr, k):
n = len(arr)
dp = [0] * (n + 1)
for i in range(1, n + 1):
max_val = 0
for j in range(1, min(k, i) + 1):
max_val = max(max_val, arr[i - j])
dp[i] = max(dp[i], dp[i - j] + max_val * j)
return dp[n]
# Example usage
arr = [1, 15, 7, 9, 2, 5, 10]
k = 3
print(maxSumAfterPartitioning(arr, k)) # Output: 84
Explanation:
- We use a DP array where dp[i] represents the maximum sum we can achieve for the first i elements of the array.
- For each position i, we consider partitions of length 1 to k (or i, whichever is smaller).
- We find the maximum value in each partition and update dp[i] accordingly.
- The final answer is in dp[n], which gives us the maximum sum after partitioning the entire array.
5.3. Partition Array into Three Parts with Equal Sum
Problem: Given an array of integers, return true if it can be partitioned into three non-empty parts with equal sums.
Approach: We can use a two-pointer technique to solve this problem efficiently.
def canThreePartsEqualSum(arr):
total_sum = sum(arr)
# If the total sum is not divisible by 3, it's impossible to partition
if total_sum % 3 != 0:
return False
target_sum = total_sum // 3
n = len(arr)
current_sum = 0
count = 0
for num in arr:
current_sum += num
if current_sum == target_sum:
count += 1
current_sum = 0
if count == 3:
return True
return False
# Example usage
arr = [0,2,1,-6,6,-7,9,1,2,0,1]
print(canThreePartsEqualSum(arr)) # Output: True
Explanation:
- We first check if the total sum is divisible by 3. If not, it’s impossible to partition into three equal parts.
- We then iterate through the array, keeping track of the current sum and the number of partitions we’ve found.
- Each time we reach the target sum (total_sum / 3), we increment our count and reset the current sum.
- If we find three such partitions, we return True. Otherwise, we return False.
6. Tips for Optimizing Your Solutions
When working on array partition problems, keep these optimization tips in mind:
- Analyze the problem constraints: Understanding the size of the input and any specific constraints can help you choose the most appropriate algorithm.
- Consider space-time tradeoffs: Sometimes, using extra space can significantly reduce time complexity. Evaluate whether this tradeoff is worthwhile for your specific problem.
- Look for patterns or properties: Many partition problems have underlying patterns or mathematical properties that can be exploited for more efficient solutions.
- Use appropriate data structures: Choosing the right data structure (e.g., hash tables for quick lookups) can greatly improve the efficiency of your solution.
- Precompute when possible: If certain calculations are repeated, consider precomputing them to avoid redundant work.
- Optimize your DP solutions: For dynamic programming solutions, look for opportunities to reduce the dimensionality of your DP table or use rolling arrays to save space.
- Consider bitwise operations: For problems involving subsets or combinations, bitwise operations can often lead to very efficient solutions.
7. Practice Problems and Resources
To further improve your skills in solving array partition problems, here are some practice problems and resources:
Practice Problems:
- LeetCode 416: Partition Equal Subset Sum
- LeetCode 698: Partition to K Equal Sum Subsets
- LeetCode 1043: Partition Array for Maximum Sum
- LeetCode 1013: Partition Array Into Three Parts With Equal Sum
- LeetCode 131: Palindrome Partitioning
- LeetCode 2035: Partition Array Into Two Arrays to Minimize Sum Difference
Resources:
- AlgoCademy’s interactive coding tutorials on array manipulation and dynamic programming
- “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein – for a deep dive into algorithmic techniques
- “Competitive Programmer’s Handbook” by Antti Laaksonen – offers excellent explanations of various algorithmic techniques
- GeeksforGeeks articles on array partitioning and related topics
- YouTube channels like “Back To Back SWE” and “Abdul Bari” for visual explanations of algorithms
8. Conclusion
Array partition problems are a crucial aspect of algorithmic problem-solving and are frequently encountered in coding interviews, especially at major tech companies. By understanding the various types of partition problems and mastering the techniques to solve them efficiently, you can significantly improve your problem-solving skills and coding abilities.
Remember that the key to excelling at these problems is practice. Start with simpler problems and gradually move to more complex ones. As you solve more problems, you’ll start recognizing patterns and developing intuitions that will help you tackle even the most challenging array partition problems.
Keep in mind that while efficiency is important, clarity and correctness should always be your first priority. Always start with a clear, correct solution before optimizing. With consistent practice and a solid understanding of the underlying principles, you’ll be well-equipped to handle array partition problems in your coding interviews and beyond.
Happy coding, and may your arrays always be perfectly partitioned!