How to Get Started with Web Development: A Comprehensive Guide for Beginners
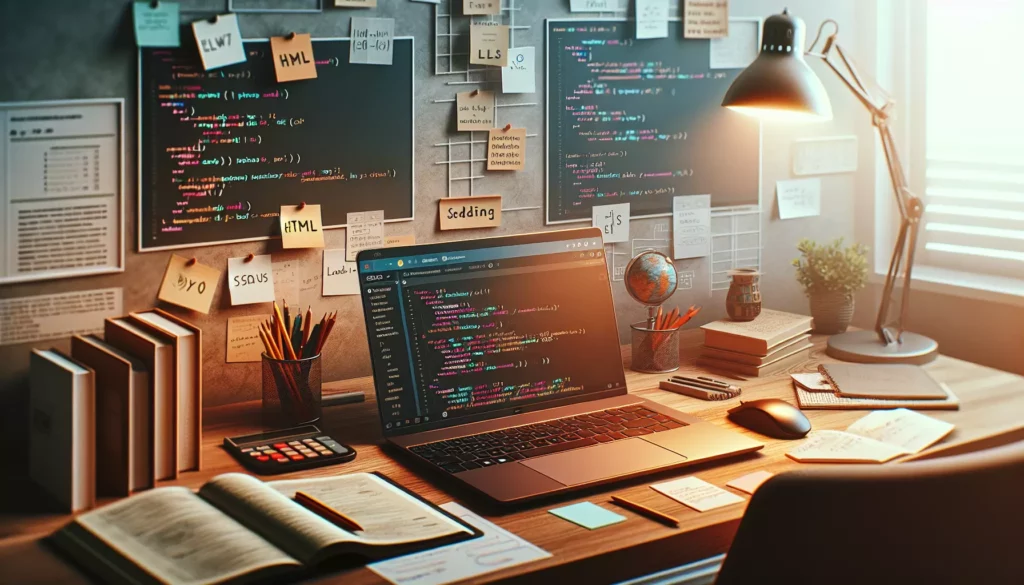
Web development is an exciting and ever-evolving field that offers countless opportunities for creativity, problem-solving, and career growth. Whether you’re looking to build your own website, start a new career, or simply understand the technology behind the web, getting started with web development can seem daunting at first. But don’t worry! This comprehensive guide will walk you through the essential steps to begin your journey in web development, from understanding the basics to choosing the right tools and resources.
Table of Contents
- Understanding the Basics of Web Development
- Learning the Essential Languages
- Choosing the Right Tools and Resources
- Building Your First Projects
- Exploring Frameworks and Libraries
- Understanding Version Control
- Mastering Responsive Design
- Diving into Backend Development
- Working with Databases
- API Integration and Development
- Testing and Debugging
- Security Best Practices
- Deployment and Hosting
- Continuous Learning and Staying Updated
- Building Your Portfolio
- Networking and Joining the Developer Community
1. Understanding the Basics of Web Development
Before diving into coding, it’s crucial to understand what web development entails. Web development is the process of building and maintaining websites. It involves several aspects:
- Frontend Development: This is what users see and interact with in their browsers. It includes the design, layout, and interactivity of a website.
- Backend Development: This involves the server-side of web development, including databases, server logic, and application integration.
- Full-Stack Development: This combines both frontend and backend development skills.
Understanding these concepts will help you decide which area you want to focus on initially. Many beginners start with frontend development as it’s more visual and provides immediate feedback.
2. Learning the Essential Languages
To get started with web development, you’ll need to learn the following fundamental languages:
HTML (HyperText Markup Language)
HTML is the backbone of web pages. It provides the structure and content of a webpage. Here’s a simple example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>My First Web Page</title>
</head>
<body>
<h1>Hello, World!</h1>
<p>This is my first web page.</p>
</body>
</html>
CSS (Cascading Style Sheets)
CSS is used to style and layout web pages. It determines how HTML elements are displayed. Here’s a basic CSS example:
body {
font-family: Arial, sans-serif;
background-color: #f0f0f0;
}
h1 {
color: #333;
text-align: center;
}
p {
line-height: 1.6;
margin-bottom: 20px;
}
JavaScript
JavaScript adds interactivity and dynamic behavior to web pages. It’s a powerful programming language that can be used for both frontend and backend development. Here’s a simple JavaScript example:
function greetUser() {
let name = prompt("What's your name?");
alert(`Hello, ${name}! Welcome to my website.`);
}
greetUser();
Start by mastering these three languages, as they form the foundation of web development. There are numerous free resources available online to learn these languages, including interactive tutorials, video courses, and documentation.
3. Choosing the Right Tools and Resources
To start coding, you’ll need some essential tools:
- Text Editor or Integrated Development Environment (IDE): Popular choices include Visual Studio Code, Sublime Text, or Atom.
- Web Browsers: Use modern browsers like Chrome, Firefox, or Edge for testing your web pages.
- Version Control System: Git is the most widely used version control system. GitHub or GitLab provide free repositories for your projects.
- Online Learning Platforms: Websites like AlgoCademy, freeCodeCamp, Codecademy, or MDN Web Docs offer comprehensive web development courses and documentation.
Choose tools that you find comfortable and start with free resources. As you progress, you can explore more advanced tools and paid courses if needed.
4. Building Your First Projects
The best way to learn web development is by doing. Start with small projects and gradually increase their complexity. Here are some ideas for beginner projects:
- Personal portfolio website
- To-do list application
- Simple calculator
- Weather app using a public API
- Blog layout
As you build these projects, you’ll encounter challenges that will push you to learn more and improve your skills. Don’t be afraid to make mistakes – they’re an essential part of the learning process.
5. Exploring Frameworks and Libraries
Once you’re comfortable with the basics, you can start exploring popular frameworks and libraries that make web development more efficient:
Frontend Frameworks/Libraries:
- React.js
- Vue.js
- Angular
CSS Frameworks:
- Bootstrap
- Tailwind CSS
- Foundation
These tools can significantly speed up your development process and introduce you to best practices in web development. However, it’s important to have a solid understanding of the fundamentals before diving into frameworks.
6. Understanding Version Control
Version control is crucial for managing your code, especially when working on larger projects or collaborating with others. Git is the most popular version control system. Here are some basic Git commands to get you started:
git init # Initialize a new Git repository
git add . # Add all files to staging area
git commit -m "Message" # Commit changes with a descriptive message
git push origin main # Push changes to remote repository
git pull origin main # Pull latest changes from remote repository
Learning Git will help you track changes, collaborate with others, and manage your projects more effectively.
7. Mastering Responsive Design
With the variety of devices used to access the web, responsive design is crucial. It ensures that your website looks good and functions well on all screen sizes. Key concepts to learn include:
- Flexible grids and layouts
- CSS media queries
- Flexible images and media
- Mobile-first design approach
Here’s a simple example of a media query in CSS:
@media screen and (max-width: 600px) {
body {
font-size: 14px;
}
.container {
width: 100%;
}
}
Practice creating layouts that adapt to different screen sizes to ensure your websites are accessible to all users.
8. Diving into Backend Development
If you’re interested in full-stack development, you’ll need to learn backend technologies. Popular backend languages and frameworks include:
- Node.js with Express.js
- Python with Django or Flask
- Ruby on Rails
- PHP with Laravel
- Java with Spring
Backend development involves handling server-side logic, databases, and APIs. Start with one language and framework, and build simple server applications to understand how the backend works.
9. Working with Databases
Databases are essential for storing and managing data in web applications. Learn about different types of databases:
- Relational Databases: MySQL, PostgreSQL
- NoSQL Databases: MongoDB, Redis
Understanding how to design database schemas, write queries, and integrate databases with your backend is crucial for building dynamic web applications.
10. API Integration and Development
APIs (Application Programming Interfaces) allow different software systems to communicate with each other. Learn how to:
- Consume third-party APIs in your applications
- Design and build your own RESTful APIs
- Use API testing tools like Postman
APIs are fundamental in modern web development, enabling features like social media integration, payment processing, and data retrieval from external sources.
11. Testing and Debugging
Writing clean, bug-free code is essential. Learn about:
- Different types of testing (unit, integration, end-to-end)
- Testing frameworks like Jest for JavaScript
- Debugging techniques and tools in browsers and IDEs
Regular testing and efficient debugging will improve the quality of your code and save time in the long run.
12. Security Best Practices
Web security is crucial to protect your applications and users’ data. Learn about common security vulnerabilities and how to prevent them:
- Cross-Site Scripting (XSS)
- SQL Injection
- Cross-Site Request Forgery (CSRF)
- Secure authentication and authorization
- HTTPS implementation
Implementing security best practices from the start will help you build safer, more robust web applications.
13. Deployment and Hosting
Learn how to deploy your web applications to make them accessible on the internet. Familiarize yourself with:
- Web hosting services (e.g., Netlify, Heroku, AWS, DigitalOcean)
- Domain name registration and DNS configuration
- Continuous Integration/Continuous Deployment (CI/CD) pipelines
Understanding deployment processes will allow you to share your projects with the world and gain practical experience in managing live applications.
14. Continuous Learning and Staying Updated
Web development is a field that evolves rapidly. To stay relevant:
- Follow web development blogs and news sites
- Participate in online coding communities (e.g., Stack Overflow, GitHub)
- Attend web development conferences and meetups
- Experiment with new technologies and tools
Continuous learning is key to growing as a web developer and staying ahead in the field.
15. Building Your Portfolio
As you learn and create projects, compile them into a portfolio website. Your portfolio should:
- Showcase your best work
- Demonstrate your skills and technologies you’ve mastered
- Include project descriptions and links to live demos or GitHub repositories
- Highlight your problem-solving abilities and creativity
A strong portfolio is essential for landing jobs or freelance work in web development.
16. Networking and Joining the Developer Community
Building connections in the web development community can provide valuable learning opportunities and potential career prospects:
- Join local tech meetups or coding groups
- Participate in online forums and discussion boards
- Contribute to open-source projects on GitHub
- Attend hackathons and coding competitions
- Network with other developers on social media platforms like Twitter or LinkedIn
Engaging with the community can lead to mentorship opportunities, collaborations, and a deeper understanding of the industry.
Conclusion
Getting started with web development is an exciting journey that opens up a world of possibilities. Remember that learning to code is a gradual process that requires patience, practice, and persistence. Start with the basics, build projects, and continuously challenge yourself to learn new things.
As you progress, you’ll find that web development is not just about writing code – it’s about problem-solving, creativity, and continuous learning. Embrace the challenges, celebrate your progress, and don’t be afraid to ask for help when you need it.
With dedication and the right resources, you can build a rewarding career in web development. Whether you aim to work for a tech giant, start your own business, or contribute to meaningful projects, the skills you learn in web development will be invaluable.
So, are you ready to start your web development journey? Pick a starting point from this guide, dive in, and start coding. The web is waiting for your contributions!