How to Get Started with Machine Learning Using Scikit-Learn
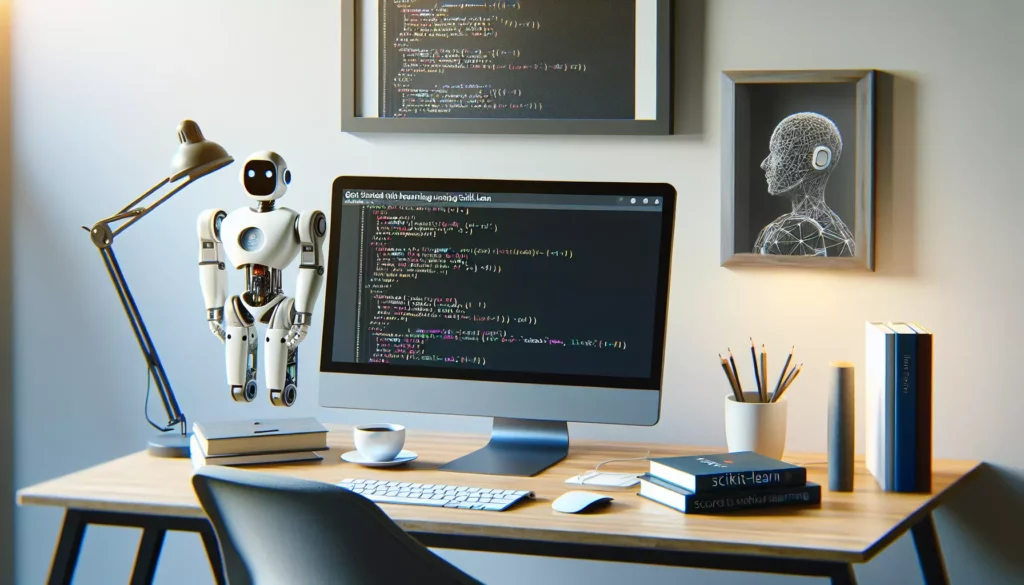
Machine learning has become an integral part of modern technology, powering everything from recommendation systems to autonomous vehicles. For aspiring data scientists and programmers looking to dive into this exciting field, Scikit-Learn provides an excellent starting point. In this comprehensive guide, we’ll walk you through the process of getting started with machine learning using Scikit-Learn, a powerful and user-friendly library for Python.
Table of Contents
- Introduction to Machine Learning and Scikit-Learn
- Installing Scikit-Learn and Required Dependencies
- Data Preparation and Preprocessing
- Choosing the Right Machine Learning Model
- Training Your First Machine Learning Model
- Model Evaluation and Metrics
- Hyperparameter Tuning and Model Optimization
- Deploying Your Machine Learning Model
- Advanced Topics and Next Steps
- Conclusion
1. Introduction to Machine Learning and Scikit-Learn
Machine learning is a subset of artificial intelligence that focuses on creating algorithms and statistical models that enable computer systems to improve their performance on a specific task through experience. It’s all about teaching computers to learn from data, identify patterns, and make decisions with minimal human intervention.
Scikit-Learn is an open-source machine learning library for Python that provides a wide range of supervised and unsupervised learning algorithms. It’s built on NumPy, SciPy, and matplotlib, making it an essential tool for data scientists and machine learning practitioners. Some key features of Scikit-Learn include:
- Simple and efficient tools for data mining and data analysis
- Accessible to everybody and reusable in various contexts
- Built on NumPy, SciPy, and matplotlib
- Open source, commercially usable – BSD license
2. Installing Scikit-Learn and Required Dependencies
Before we dive into machine learning with Scikit-Learn, let’s set up our development environment. You’ll need Python installed on your system, preferably version 3.6 or later. Here’s how to install Scikit-Learn and its dependencies:
Using pip
If you have Python and pip installed, you can simply run:
pip install scikit-learn
This command will install Scikit-Learn along with its required dependencies (NumPy and SciPy).
Using Anaconda
If you’re using Anaconda, which is recommended for data science projects, you can install Scikit-Learn using:
conda install scikit-learn
Once installed, you can verify the installation by opening a Python interpreter and running:
import sklearn
print(sklearn.__version__)
This should print the version of Scikit-Learn installed on your system.
3. Data Preparation and Preprocessing
Before we can start building machine learning models, we need to prepare our data. Data preparation is a crucial step in the machine learning pipeline, as the quality and format of your data significantly impact the performance of your models.
Loading Data
Scikit-Learn provides built-in datasets that are great for learning and practicing. Let’s start with the iris dataset, a classic dataset in machine learning:
from sklearn import datasets
iris = datasets.load_iris()
X = iris.data
y = iris.target
Here, X
contains the feature data, and y
contains the target labels.
Splitting the Data
It’s important to split your data into training and testing sets. The training set is used to teach your model, while the testing set is used to evaluate its performance on unseen data:
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
This code splits the data into 70% for training and 30% for testing.
Feature Scaling
Many machine learning algorithms perform better when features are on a similar scale. Scikit-Learn provides several scalers, including StandardScaler:
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
X_train_scaled = scaler.fit_transform(X_train)
X_test_scaled = scaler.transform(X_test)
This standardizes the features by removing the mean and scaling to unit variance.
4. Choosing the Right Machine Learning Model
Scikit-Learn offers a wide variety of machine learning algorithms. The choice of algorithm depends on your specific problem, the size and nature of your data, and your desired outcomes. Here are some common types of machine learning problems and suitable algorithms:
Classification
- Logistic Regression: For binary classification problems
- Decision Trees: For both binary and multi-class classification
- Random Forest: An ensemble method that often performs well on various datasets
- Support Vector Machines (SVM): Effective for both linear and non-linear classification
Regression
- Linear Regression: For simple linear relationships
- Polynomial Regression: When the relationship between variables is non-linear
- Random Forest Regressor: For complex relationships and feature importance
Clustering
- K-Means: For partitioning n observations into k clusters
- DBSCAN: For density-based clustering
- Hierarchical Clustering: For creating a hierarchy of clusters
For our iris dataset example, let’s use a simple yet effective algorithm: k-Nearest Neighbors (KNN).
5. Training Your First Machine Learning Model
Now that we have prepared our data and chosen a model, let’s train our first machine learning model using Scikit-Learn. We’ll use the K-Nearest Neighbors classifier for this example:
from sklearn.neighbors import KNeighborsClassifier
# Create the model
knn = KNeighborsClassifier(n_neighbors=3)
# Train the model
knn.fit(X_train_scaled, y_train)
In this code:
- We import the KNeighborsClassifier from Scikit-Learn.
- We create an instance of the classifier, specifying that we want to consider the 3 nearest neighbors for classification.
- We use the
fit
method to train our model on the scaled training data.
The fit
method is where the actual learning happens. The model analyzes the training data to learn the relationship between the features (X_train_scaled
) and the target labels (y_train
).
6. Model Evaluation and Metrics
After training your model, it’s crucial to evaluate its performance. Scikit-Learn provides various metrics and tools for model evaluation. Let’s start by making predictions on our test set and then evaluate the model’s performance:
from sklearn.metrics import accuracy_score, classification_report, confusion_matrix
# Make predictions on the test set
y_pred = knn.predict(X_test_scaled)
# Calculate accuracy
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy:.2f}")
# Print classification report
print("\nClassification Report:")
print(classification_report(y_test, y_pred, target_names=iris.target_names))
# Print confusion matrix
print("\nConfusion Matrix:")
print(confusion_matrix(y_test, y_pred))
This code does the following:
- We use the trained model to make predictions on the scaled test data.
- We calculate the accuracy of our model by comparing the predicted labels to the true labels.
- We print a classification report, which includes precision, recall, and F1-score for each class.
- We print a confusion matrix, which shows the number of correct and incorrect predictions for each class.
Understanding these metrics is crucial for assessing your model’s performance:
- Accuracy: The proportion of correct predictions among the total number of cases examined.
- Precision: The ability of the classifier not to label as positive a sample that is negative.
- Recall: The ability of the classifier to find all the positive samples.
- F1-score: The harmonic mean of precision and recall, providing a single score that balances both metrics.
- Confusion Matrix: A table that describes the performance of a classification model on a set of test data for which the true values are known.
7. Hyperparameter Tuning and Model Optimization
While our initial model might perform well, we can often improve its performance by tuning its hyperparameters. Hyperparameters are parameters that are not learned from the data but are set prior to training. For our KNN model, the number of neighbors (k) is a hyperparameter.
Scikit-Learn provides tools for automated hyperparameter tuning, such as GridSearchCV and RandomizedSearchCV. Let’s use GridSearchCV to find the optimal number of neighbors for our KNN model:
from sklearn.model_selection import GridSearchCV
# Define the parameter grid
param_grid = {'n_neighbors': [1, 3, 5, 7, 9, 11, 13, 15]}
# Create a GridSearchCV object
grid_search = GridSearchCV(KNeighborsClassifier(), param_grid, cv=5)
# Fit the GridSearchCV object to the data
grid_search.fit(X_train_scaled, y_train)
# Print the best parameters and score
print("Best parameters:", grid_search.best_params_)
print("Best cross-validation score:", grid_search.best_score_)
# Use the best model to make predictions
best_knn = grid_search.best_estimator_
y_pred_best = best_knn.predict(X_test_scaled)
# Calculate and print the accuracy of the best model
accuracy_best = accuracy_score(y_test, y_pred_best)
print(f"Accuracy of best model: {accuracy_best:.2f}")
This code does the following:
- We define a parameter grid with different values for
n_neighbors
. - We create a GridSearchCV object, which will try all combinations of parameters in the grid.
- We fit the GridSearchCV object to our training data.
- We print the best parameters found and the corresponding cross-validation score.
- We use the best model to make predictions on our test set and calculate its accuracy.
By using GridSearchCV, we can systematically explore different hyperparameter combinations and choose the one that performs best on our data.
8. Deploying Your Machine Learning Model
Once you’ve trained and optimized your model, the next step is to deploy it so it can make predictions on new, unseen data. While the specifics of deployment can vary depending on your use case, here are some general steps and considerations:
Saving the Model
Scikit-Learn provides a simple way to save your trained model using the joblib library:
from joblib import dump, load
# Save the model
dump(best_knn, 'best_knn_model.joblib')
# Later, you can load the model like this:
# loaded_model = load('best_knn_model.joblib')
Creating a Prediction Function
You’ll typically want to create a function that takes in new data, preprocesses it in the same way as your training data, and returns predictions:
def predict_iris(features):
# Ensure features are in the correct format
features = np.array(features).reshape(1, -1)
# Scale the features
scaled_features = scaler.transform(features)
# Make prediction
prediction = best_knn.predict(scaled_features)
# Return the predicted class name
return iris.target_names[prediction[0]]
# Example usage
new_flower = [5.1, 3.5, 1.4, 0.2]
print(f"Predicted class: {predict_iris(new_flower)}")
Deployment Options
Depending on your needs, you might deploy your model in various ways:
- As part of a web application: You could use a web framework like Flask or Django to create an API that serves predictions.
- As a standalone script: For batch predictions or integration into other systems.
- Using cloud services: Platforms like AWS SageMaker, Google Cloud AI Platform, or Azure Machine Learning can host and serve your model.
Monitoring and Updating
Once deployed, it’s important to monitor your model’s performance over time. You may need to retrain your model periodically with new data to maintain its accuracy.
9. Advanced Topics and Next Steps
Congratulations! You’ve now gone through the basics of machine learning with Scikit-Learn. As you continue your journey, here are some advanced topics to explore:
Ensemble Methods
Ensemble methods combine multiple models to create a more powerful predictor. Scikit-Learn offers several ensemble methods:
- Random Forests
- Gradient Boosting (e.g., XGBoost, LightGBM)
- Voting Classifiers
Feature Selection and Engineering
Learn techniques to select the most important features and create new features to improve your model’s performance:
- Principal Component Analysis (PCA)
- Feature importance with Random Forests
- Polynomial features
Handling Imbalanced Data
Many real-world datasets are imbalanced, where one class is much more frequent than others. Techniques to handle this include:
- Oversampling (e.g., SMOTE)
- Undersampling
- Adjusting class weights
Cross-Validation Strategies
Learn more advanced cross-validation techniques:
- Stratified K-Fold
- Leave-One-Out Cross-Validation
- Time Series Cross-Validation
Pipelines
Scikit-Learn’s Pipeline class allows you to chain multiple steps that can be cross-validated together while setting different parameters.
Explainable AI
As models become more complex, understanding their decisions becomes crucial. Explore techniques for model interpretability:
- SHAP (SHapley Additive exPlanations) values
- LIME (Local Interpretable Model-agnostic Explanations)
- Partial Dependence Plots
10. Conclusion
In this comprehensive guide, we’ve walked through the process of getting started with machine learning using Scikit-Learn. We’ve covered everything from installation and data preparation to model training, evaluation, and deployment. Remember, machine learning is a vast field, and this guide is just the beginning of your journey.
As you continue to learn and grow in your machine learning journey, keep these key points in mind:
- Practice regularly with different datasets and problems
- Stay updated with the latest developments in the field
- Participate in machine learning competitions on platforms like Kaggle
- Collaborate with others and share your knowledge
- Always consider the ethical implications of your machine learning models
With Scikit-Learn as your foundation, you’re well-equipped to tackle a wide range of machine learning problems. As you gain more experience, you may want to explore other libraries and frameworks like TensorFlow or PyTorch for deep learning tasks.
Remember, the key to mastering machine learning is continuous learning and practice. Keep experimenting, stay curious, and don’t be afraid to tackle complex problems. Happy learning!