How to Get Started with Machine Learning and AI Programming: A Comprehensive Guide
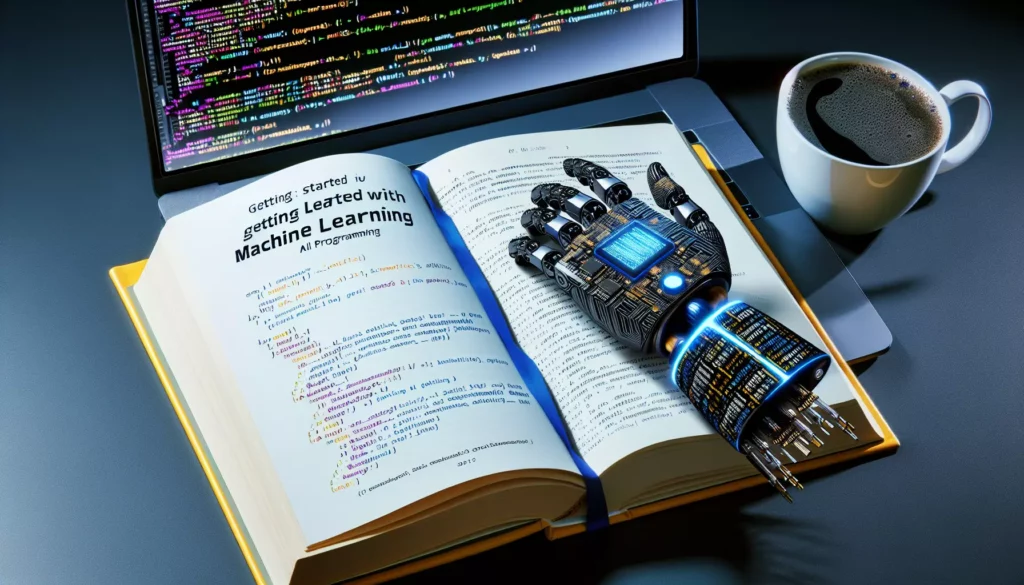
In today’s rapidly evolving technological landscape, machine learning (ML) and artificial intelligence (AI) have emerged as transformative forces across various industries. As these fields continue to grow and shape our world, there’s never been a better time to dive into ML and AI programming. This comprehensive guide will walk you through the essential steps to embark on your journey in machine learning and AI, providing you with a solid foundation to build upon.
1. Understanding the Basics
Before diving into the technical aspects of machine learning and AI programming, it’s crucial to grasp the fundamental concepts and terminology associated with these fields.
1.1 What is Machine Learning?
Machine learning is a subset of AI that focuses on creating algorithms and statistical models that enable computer systems to improve their performance on a specific task through experience, without being explicitly programmed.
1.2 What is Artificial Intelligence?
Artificial Intelligence is a broader field that encompasses machine learning and aims to create intelligent machines that can perform tasks that typically require human intelligence, such as visual perception, speech recognition, decision-making, and language translation.
1.3 Key Concepts
- Supervised Learning: Training models on labeled data
- Unsupervised Learning: Finding patterns in unlabeled data
- Reinforcement Learning: Learning through interaction with an environment
- Deep Learning: A subset of ML that uses neural networks with multiple layers
- Natural Language Processing (NLP): Processing and analyzing human language
- Computer Vision: Enabling machines to interpret and understand visual information
2. Prerequisites
To get started with machine learning and AI programming, you’ll need a strong foundation in certain areas:
2.1 Mathematics
A solid understanding of mathematics is crucial for ML and AI. Focus on:
- Linear Algebra
- Calculus
- Probability and Statistics
- Optimization
2.2 Programming Skills
While there are multiple programming languages used in ML and AI, Python has emerged as the most popular choice due to its simplicity and extensive libraries. Familiarize yourself with:
- Python programming fundamentals
- Object-oriented programming concepts
- Data structures and algorithms
2.3 Data Analysis and Visualization
Understanding how to work with data is essential. Learn:
- Data manipulation using pandas
- Data visualization with matplotlib and seaborn
- Basic statistical analysis
3. Choose Your Learning Path
There are multiple ways to learn machine learning and AI programming. Choose the path that best suits your learning style and goals:
3.1 Online Courses
Many platforms offer comprehensive courses on ML and AI:
- Coursera: “Machine Learning” by Andrew Ng
- edX: “Artificial Intelligence” by Columbia University
- Udacity: “Intro to Machine Learning” Nanodegree
- Fast.ai: Practical Deep Learning for Coders
3.2 Books
For those who prefer self-study through books:
- “Hands-On Machine Learning with Scikit-Learn, Keras, and TensorFlow” by Aurélien Géron
- “Deep Learning” by Ian Goodfellow, Yoshua Bengio, and Aaron Courville
- “Artificial Intelligence: A Modern Approach” by Stuart Russell and Peter Norvig
3.3 Coding Platforms
Interactive coding platforms can help you practice and reinforce your learning:
- AlgoCademy: Offers AI-powered assistance and step-by-step guidance for learning algorithms and data structures
- Kaggle: Provides datasets, competitions, and a community of data scientists
- LeetCode: Offers coding challenges and interview preparation resources
4. Master the Essential Tools and Libraries
Familiarize yourself with the following tools and libraries commonly used in ML and AI:
4.1 NumPy
NumPy is the fundamental package for scientific computing in Python. It provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays.
import numpy as np
# Create a 2D array
arr = np.array([[1, 2, 3], [4, 5, 6]])
print(arr)
# Perform operations
print(np.sum(arr))
print(np.mean(arr))
4.2 Pandas
Pandas is a powerful data manipulation and analysis library. It provides data structures like DataFrames, which allow you to work with structured data efficiently.
import pandas as pd
# Create a DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
print(df)
# Perform operations
print(df.describe())
print(df['A'].mean())
4.3 Scikit-learn
Scikit-learn is a machine learning library that provides simple and efficient tools for data mining and data analysis. It includes various algorithms for classification, regression, clustering, and model evaluation.
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import accuracy_score
# Assuming X and y are your features and target variables
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2)
model = LogisticRegression()
model.fit(X_train, y_train)
y_pred = model.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy}")
4.4 TensorFlow and Keras
TensorFlow is an open-source library for numerical computation and large-scale machine learning. Keras is a high-level neural networks API that runs on top of TensorFlow, making it easier to build and train deep learning models.
import tensorflow as tf
from tensorflow import keras
# Define a simple neural network
model = keras.Sequential([
keras.layers.Dense(64, activation='relu', input_shape=(784,)),
keras.layers.Dense(10, activation='softmax')
])
# Compile the model
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# Train the model (assuming X_train and y_train are your training data)
model.fit(X_train, y_train, epochs=5)
4.5 PyTorch
PyTorch is another popular open-source machine learning library, known for its flexibility and dynamic computational graphs. It’s particularly favored in research and for building complex models.
import torch
import torch.nn as nn
# Define a simple neural network
class SimpleNet(nn.Module):
def __init__(self):
super(SimpleNet, self).__init__()
self.fc1 = nn.Linear(784, 64)
self.fc2 = nn.Linear(64, 10)
def forward(self, x):
x = torch.relu(self.fc1(x))
x = self.fc2(x)
return x
model = SimpleNet()
print(model)
5. Practice with Real-world Projects
Theory is important, but practical experience is crucial. Start working on projects to apply your knowledge:
5.1 Beginner Projects
- Iris Flower Classification: A classic machine learning problem for beginners
- Handwritten Digit Recognition: Using the MNIST dataset
- Sentiment Analysis: Classifying movie reviews as positive or negative
5.2 Intermediate Projects
- Image Classification: Using convolutional neural networks (CNNs)
- Stock Price Prediction: Time series forecasting with LSTM networks
- Recommendation Systems: Building a movie recommendation engine
5.3 Advanced Projects
- Natural Language Generation: Creating a chatbot or text generator
- Object Detection: Identifying and locating objects in images or video
- Reinforcement Learning: Training an agent to play games
6. Stay Updated and Engage with the Community
The field of ML and AI is rapidly evolving. Stay current with the latest developments:
6.1 Follow Research Papers
- arXiv.org: A repository of electronic preprints of scientific papers
- Papers With Code: A free resource of machine learning papers with code
6.2 Attend Conferences and Workshops
- NeurIPS (Neural Information Processing Systems)
- ICML (International Conference on Machine Learning)
- CVPR (Conference on Computer Vision and Pattern Recognition)
6.3 Join Online Communities
- Reddit: r/MachineLearning, r/artificial
- Stack Overflow: For technical questions and answers
- GitHub: Contribute to open-source projects
7. Explore Specializations
As you progress, you might want to specialize in specific areas of ML and AI:
7.1 Computer Vision
Focus on algorithms that enable computers to understand and process visual information from the world.
7.2 Natural Language Processing
Specialize in developing algorithms to process and analyze human language data.
7.3 Robotics
Combine AI with mechanical engineering to create intelligent machines that can interact with the physical world.
7.4 Reinforcement Learning
Dive deep into algorithms that learn through interaction with an environment to maximize a reward signal.
8. Ethical Considerations in AI
As you embark on your journey in ML and AI, it’s crucial to be aware of the ethical implications of these technologies:
8.1 Bias and Fairness
Understand how biases can be introduced into AI systems and learn techniques to detect and mitigate them.
8.2 Privacy and Security
Learn about the importance of protecting user data and ensuring the security of AI systems.
8.3 Transparency and Explainability
Explore methods to make AI decision-making processes more transparent and explainable to end-users.
9. Career Opportunities in ML and AI
As you develop your skills, various career paths become available:
- Machine Learning Engineer
- Data Scientist
- AI Research Scientist
- Computer Vision Engineer
- NLP Specialist
- Robotics Engineer
Conclusion
Getting started with machine learning and AI programming is an exciting journey that requires dedication, continuous learning, and practical application. By following this comprehensive guide, you’ll be well on your way to mastering these cutting-edge technologies. Remember that the field is vast and constantly evolving, so staying curious and adaptable is key to success.
As you progress in your learning journey, platforms like AlgoCademy can be invaluable resources. With its focus on algorithmic thinking, problem-solving, and practical coding skills, AlgoCademy can help you develop the strong foundation necessary for excelling in machine learning and AI programming. The platform’s AI-powered assistance and step-by-step guidance can be particularly helpful as you tackle complex algorithms and data structures, which are fundamental to many ML and AI applications.
Embrace the challenges, celebrate your progress, and don’t hesitate to engage with the vibrant ML and AI community. With persistence and passion, you’ll be well-equipped to contribute to this fascinating field and potentially shape the future of technology. Happy learning!